Vue 中如何實作彈出層及模態框?
Vue是一種基於JavaScript的前端框架,提供了許多方便的工具與元件,用於建立單頁應用(SPA)的介面和使用者互動。其中,彈出層(modal)和模態框(popover)是常見的UI元件,在Vue中也可以很方便地實現。本文將介紹Vue中如何實作彈出層及模態框。
一、彈出層
彈出層一般用於提示訊息、展示選單或操作面板,通常需要覆蓋整個頁面或部分區域。 Vue中實作彈出層需要用到動態元件和slot(插槽)。
- 建立彈出層元件
首先,我們需要建立一個彈出層元件。在此,我們建立一個名為Modal的彈出層元件,並包含一個插槽(slot),用於動態載入需要顯示的內容。
<template> <div class="modal-container" v-show="show"> <div class="modal-content"> <slot></slot> </div> </div> </template> <script> export default { name: 'Modal', props: { show: { type: Boolean, default: false } } } </script> <style scoped> .modal-container { position: fixed; top: 0; left: 0; width: 100%; height: 100%; z-index: 9999; background-color: rgba(0, 0, 0, 0.5); display: flex; justify-content: center; align-items: center; } .modal-content { background-color: #fff; padding: 20px; } </style>
在上面的程式碼中,我們首先定義了一個名為Modal的元件,並傳入了一個名為show的 props,該屬性用於控制彈出層是否顯示。在元件模板中,我們使用了動態插槽(slot)來展示彈出層中需要顯示的內容。然後,我們設定了一些樣式,使彈出層能夠居中顯示,並添加半透明的背景色。
- 在需要顯示彈出層的元件中使用Modal元件
接下來,我們需要在需要顯示彈出層的元件中使用Modal元件。在此,我們建立一個名為App的根元件,並在該元件中新增一個按鈕,用於觸發顯示彈出層。
<template> <div class="app"> <button @click="showModal = !showModal">显示弹出层</button> <modal v-bind:show="showModal"> <p>这是弹出层中的内容</p> </modal> </div> </template> <script> import Modal from './components/Modal.vue' export default { name: 'App', components: { Modal }, data() { return { showModal: false } } } </script> <style> .app { padding: 20px; } </style>
在上面的程式碼中,我們首先匯入了先前定義的Modal元件,並在元件模板中加入了一個按鈕,用於觸發顯示彈出層。然後,我們使用v-bind指令將showModal屬性綁定到Modal元件的show屬性上。最後,我們將需要在彈出層中展示的內容放置在Modal元件的插槽中。
二、模態框
模態框通常用於提示使用者需要進行確認或選擇,同時防止使用者在進行操作之前進行其他操作。與彈出層類似,Vue中實作模態框也需要用到動態元件和slot。
- 建立模態框元件
首先,我們需要建立一個模態框元件。在此,我們建立一個名為Confirmation的模態框元件,並包含兩個插槽(slot),一個用於展示提示訊息,另一個用於展示確認和取消按鈕。
<template> <div class="modal-container" v-show="show"> <div class="modal-content"> <div class="modal-body"> <slot name="body"></slot> </div> <div class="modal-footer"> <slot name="footer"> <button @click="cancel">取消</button> <button @click="confirm">确认</button> </slot> </div> </div> </div> </template> <script> export default { name: 'Confirmation', props: { show: { type: Boolean, default: false }, onCancel: Function, onConfirm: Function }, methods: { cancel() { this.onCancel && this.onCancel() }, confirm() { this.onConfirm && this.onConfirm() } } } </script> <style scoped> .modal-container { position: fixed; top: 0; left: 0; width: 100%; height: 100%; z-index: 9999; background-color: rgba(0, 0, 0, 0.5); display: flex; justify-content: center; align-items: center; } .modal-content { background-color: #fff; padding: 20px; } .modal-footer { display: flex; justify-content: flex-end; margin-top: 20px; } .modal-footer button { margin-left: 10px; } </style>
在上面的程式碼中,我們建立了一個名為Confirmation的模態方塊元件,並傳入了名為show、onCancel和onConfirm的屬性,分別用於控制模態方塊是否顯示、取消操作和確認操作。在組件模板中,我們使用了兩個插槽(slot),一個用於展示提示訊息,一個用於展示確認和取消按鈕。在方法中,我們定義了cancel和confirm方法用於處理取消和確認操作,並在這些方法中觸發父元件傳遞的回呼函數。
- 在需要顯示模態框的元件中使用Confirmation元件
#接下來,我們需要在需要顯示模態框的元件中使用Confirmation元件。在此,我們建立一個名為App的根元件,並在該元件中新增一個按鈕,用於觸發Confirmation元件顯示模態方塊。
<template> <div class="app"> <button @click="showModal = !showModal">显示模态框</button> <confirmation v-bind:show="showModal" v-bind:onCancel="cancel" v-bind:onConfirm="confirm" > <template v-slot:body> <p>确定要删除吗?</p> </template> </confirmation> </div> </template> <script> import Confirmation from './components/Confirmation.vue' export default { name: 'App', components: { Confirmation }, data() { return { showModal: false } }, methods: { cancel() { this.showModal = false }, confirm() { alert('删除成功!') this.showModal = false } } } </script> <style> .app { padding: 20px; } </style>
在上面的程式碼中,我們將Confirmation元件當作模態方塊元件使用,並將showModal屬性、cancel方法和confirm方法綁定到Confirmation元件的屬性上。在Confirmation元件的插槽中,我們展示了要顯示的提示訊息。在Vue的範本中,我們使用v-slot指令來定義在Confirmation元件中使用的插槽,以及插槽的名稱(body)。在父元件中,我們定義了cancel方法和confirm方法用於處理取消和確認操作,並在確認操作中提示使用者刪除成功。
總結
在Vue中實作彈出層和模態框需要使用動態元件和slot。透過將元件作為彈出層或模態框使用,我們可以輕鬆實現這些常見的UI元件。同時,透過將回呼函數傳遞給子元件,我們可以輕鬆地處理子元件中的使用者操作,並將結果回饋給父元件。
以上是Vue 中如何實作彈出層及模態框?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
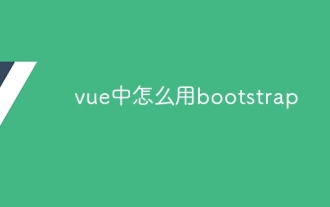
在 Vue.js 中使用 Bootstrap 分為五個步驟:安裝 Bootstrap。在 main.js 中導入 Bootstrap。直接在模板中使用 Bootstrap 組件。可選:自定義樣式。可選:使用插件。
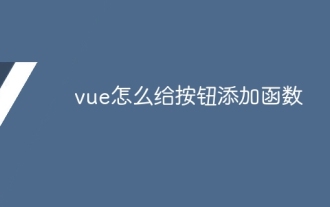
可以通過以下步驟為 Vue 按鈕添加函數:將 HTML 模板中的按鈕綁定到一個方法。在 Vue 實例中定義該方法並編寫函數邏輯。
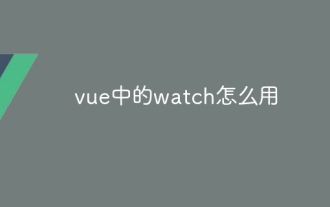
Vue.js 中的 watch 選項允許開發者監聽特定數據的變化。當數據發生變化時,watch 會觸發一個回調函數,用於執行更新視圖或其他任務。其配置選項包括 immediate,用於指定是否立即執行回調,以及 deep,用於指定是否遞歸監聽對像或數組的更改。
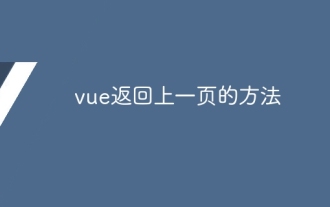
Vue.js 返回上一頁有四種方法:$router.go(-1)$router.back()使用 <router-link to="/"> 組件window.history.back(),方法選擇取決於場景。
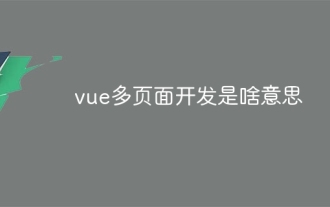
Vue 多頁面開發是一種使用 Vue.js 框架構建應用程序的方法,其中應用程序被劃分為獨立的頁面:代碼維護性:將應用程序拆分為多個頁面可以使代碼更易於管理和維護。模塊化:每個頁面都可以作為獨立的模塊,便於重用和替換。路由簡單:頁面之間的導航可以通過簡單的路由配置來管理。 SEO 優化:每個頁面都有自己的 URL,這有助於搜索引擎優化。

NetflixusesAcustomFrameworkcalled“ Gibbon” BuiltonReact,notReactorVuedIrectly.1)TeamSperience:selectBasedonFamiliarity.2)ProjectComplexity:vueforsimplerprojects:reactforforforproproject,reactforforforcompleplexones.3)cocatizationneedneeds:reactoffipicatizationneedneedneedneedneedneeds:reactoffersizationneedneedneedneedneeds:reactoffersizatization needefersmoreflexibleise.4)
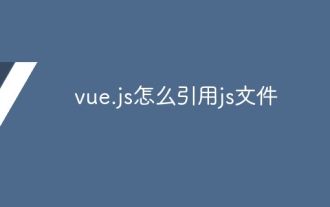
在 Vue.js 中引用 JS 文件的方法有三種:直接使用 <script> 標籤指定路徑;利用 mounted() 生命週期鉤子動態導入;通過 Vuex 狀態管理庫進行導入。
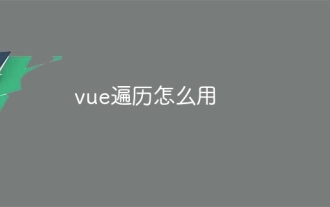
Vue.js 遍歷數組和對像有三種常見方法:v-for 指令用於遍歷每個元素並渲染模板;v-bind 指令可與 v-for 一起使用,為每個元素動態設置屬性值;.map 方法可將數組元素轉換為新數組。
