PHP 實作知識問答網站中的標籤雲和話題聚合功能。
PHP 實作知識問答網站中的標籤雲和話題聚合功能
在知識問答網站中,標籤雲和話題聚合是兩個重要的功能。標籤雲可以幫助使用者快速了解網站上的熱門話題和常見標籤,方便使用者瀏覽和搜尋相關的問題和答案。而話題聚合則可以將相同標籤的問題和答案集中在一起,提供使用者更方便的檢視和討論方式。下面我們將使用 PHP 來實作這兩個功能。
首先,我們需要建立一個資料庫來儲存問題、答案和標籤的資料。可以建立一個名為 "qa" 的資料庫,其中包含三個表:questions、answers 和 tags。
questions 表的結構如下:
CREATE TABLE `questions` ( `id` int(11) NOT NULL AUTO_INCREMENT, `title` varchar(255) DEFAULT '', `content` text, `date` datetime DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
answers 表的結構如下:
CREATE TABLE `answers` ( `id` int(11) NOT NULL AUTO_INCREMENT, `question_id` int(11) DEFAULT NULL, `content` text, `date` datetime DEFAULT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
tags 表的結構如下:
CREATE TABLE `tags` ( `id` int(11) NOT NULL AUTO_INCREMENT, `title` varchar(255) DEFAULT '', PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
接下來,我們需要在知識問答網站的提問頁面和回答頁面上新增標籤選擇框,並將使用者選擇的標籤與問題或答案關聯起來。
<!-- 提问页面 --> <form action="post_question.php" method="post"> <label for="title">问题标题:</label> <input type="text" name="title" id="title"><br> <label for="content">问题内容:</label> <textarea name="content" id="content"></textarea><br> <label for="tags">标签:</label> <select name="tags[]" id="tags" multiple> <option value="php">PHP</option> <option value="javascript">JavaScript</option> <option value="html">HTML</option> <!-- 其他标签选项 --> </select><br> <input type="submit" value="提交问题"> </form> <!-- 回答页面 --> <form action="post_answer.php" method="post"> <label for="content">回答内容:</label> <textarea name="content" id="content"></textarea><br> <label for="tags">标签:</label> <select name="tags[]" id="tags" multiple> <option value="php">PHP</option> <option value="javascript">JavaScript</option> <option value="html">HTML</option> <!-- 其他标签选项 --> </select><br> <input type="submit" value="提交回答"> </form>
然後,我們需要在背景的問題提交處理程序(post_question.php)和回答提交處理程序(post_answer.php)中,將問題或答案關聯的標籤資料存入資料庫。
// post_question.php // 获取用户提交的问题数据 $title = $_POST['title']; $content = $_POST['content']; $tags = $_POST['tags']; // 插入问题数据到 questions 表中 // 获取最后插入的问题的id $question_id = mysqli_insert_id($conn); // 插入标签数据到 tags 表中 foreach ($tags as $tag) { $sql = "INSERT INTO tags (title) VALUES ('$tag')"; mysqli_query($conn, $sql); // 获取最后插入的标签的id $tag_id = mysqli_insert_id($conn); // 插入问题和标签的关联到 question_tag 表中 $sql = "INSERT INTO question_tag (question_id, tag_id) VALUES ($question_id, $tag_id)"; mysqli_query($conn, $sql); } // post_answer.php // 获取用户提交的回答数据 $content = $_POST['content']; $tags = $_POST['tags']; // 插入回答数据到 answers 表中 // 获取最后插入的回答的id $answer_id = mysqli_insert_id($conn); // 插入标签数据到 tags 表中 foreach ($tags as $tag) { $sql = "INSERT INTO tags (title) VALUES ('$tag')"; mysqli_query($conn, $sql); // 获取最后插入的标签的id $tag_id = mysqli_insert_id($conn); // 插入回答和标签的关联到 answer_tag 表中 $sql = "INSERT INTO answer_tag (answer_id, tag_id) VALUES ($answer_id, $tag_id)"; mysqli_query($conn, $sql); }
現在,我們已經成功將使用者選擇的標籤與問題和答案關聯起來了。接下來,我們將實作標籤雲和話題聚合的功能。
首先,我們需要寫一個 PHP 函數來取得所有標籤及其出現次數。
function getTagsCloud() { global $conn; // 查询所有标签及其出现次数 $sql = "SELECT title, COUNT(*) AS count FROM tags GROUP BY title"; $result = mysqli_query($conn, $sql); $tagsCloud = array(); while ($row = mysqli_fetch_assoc($result)) { $tagsCloud[$row['title']] = $row['count']; } return $tagsCloud; }
然後,我們可以在前端頁面上使用該函數來展示標籤雲。
<?php // 获取标签云数据 $tagsCloud = getTagsCloud(); ?> <!-- 标签云 --> <div class="tag-cloud"> <?php foreach ($tagsCloud as $tag => $count): ?> <a href="search.php?tag=<?php echo $tag; ?>"><?php echo $tag; ?></a> <?php endforeach; ?> </div>
最後,我們可以實作話題聚合的功能,也就是根據標籤查詢相關的問題和答案。
function getQuestionsByTag($tag) { global $conn; // 根据标签获取问题 $sql = "SELECT q.id, q.title, q.date, COUNT(*) AS answersCount FROM questions q INNER JOIN question_tag qt ON q.id = qt.question_id INNER JOIN tags t ON t.id = qt.tag_id WHERE t.title = '$tag' GROUP BY q.id"; $result = mysqli_query($conn, $sql); $questions = array(); while ($row = mysqli_fetch_assoc($result)) { $questions[] = $row; } return $questions; } function getAnswersByTag($tag) { global $conn; // 根据标签获取回答 $sql = "SELECT a.id, a.content, a.date, q.title FROM answers a INNER JOIN answer_tag at ON a.id = at.answer_id INNER JOIN tags t ON t.id = at.tag_id INNER JOIN questions q ON q.id = a.question_id WHERE t.title = '$tag'"; $result = mysqli_query($conn, $sql); $answers = array(); while ($row = mysqli_fetch_assoc($result)) { $answers[] = $row; } return $answers; }
我們可以在主題詳情頁面上使用上述兩個函數來展示相關的問題和答案。
<?php // 获取标签名称 $tag = $_GET['tag']; // 获取话题相关的问题和回答数据 $questions = getQuestionsByTag($tag); $answers = getAnswersByTag($tag); ?> <!-- 话题相关的问题 --> <?php foreach ($questions as $question): ?> <div class="question"> <h3><?php echo $question['title']; ?></h3> <p>回答数量: <?php echo $question['answersCount']; ?></p> <!-- 其他问题信息展示 --> </div> <?php endforeach; ?> <!-- 话题相关的回答 --> <?php foreach ($answers as $answer): ?> <div class="answer"> <h4><?php echo $answer['title']; ?></h4> <p><?php echo $answer['content']; ?></p> <!-- 其他回答信息展示 --> </div> <?php endforeach; ?>
透過上述程式碼範例,我們成功實現了知識問答網站中的標籤雲和話題聚合功能,用戶可以輕鬆瀏覽熱門話題和相關的問題和答案。同時,我們也提供了對應的資料庫操作範例,讓讀者更能理解程式碼的實作思路。希望本文對有需要的讀者有幫助。
以上是PHP 實作知識問答網站中的標籤雲和話題聚合功能。的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
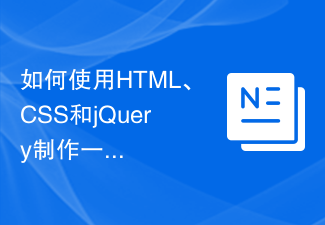
如何使用HTML、CSS和jQuery製作一個響應式的標籤雲標籤雲是一種常見的網頁元素,用於展示各種關鍵字或標籤。它通常以不同的字體大小或顏色來展示關鍵字的重要性。在本文中,將介紹如何使用HTML、CSS和jQuery來製作一個響應式的標籤雲,並給出具體的程式碼範例。在建立HTML結構首先,我們需要在HTML中建立標籤雲的基本結構。可以使用一個無序列表來表示標籤
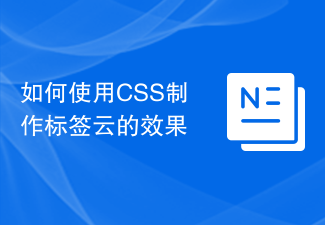
如何使用CSS製作標籤雲的效果標籤雲是常見的網頁設計元素,它以不同大小和顏色的標籤組成,用於展示關鍵字或標籤的熱門程度。在本文中,我們將介紹如何使用CSS來製作標籤雲的效果,並提供具體的程式碼範例。 HTML結構首先,我們需要在HTML中建立一個容器元素,用於包裹標籤雲的內容。可以使用一個無序列表(ul)或一個帶有一組連結元素(a)的容器。 <ul
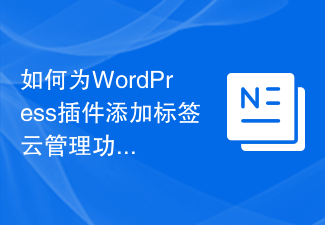
如何為WordPress外掛程式新增標籤雲端管理功能引言:WordPress是一款功能強大且易於使用的開源內容管理系統。它透過插件提供了豐富的擴展功能,使用戶能夠根據自己的需求輕鬆定製網站。其中,標籤雲(TagCloud)是一種常見的功能,可以讓使用者以雲狀的形式顯示不同標籤的熱度或依照字母順序排列。本文將向您介紹如何為WordPress外掛程式新增標籤雲管理功能,
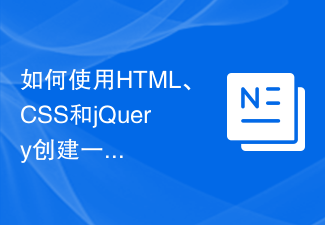
如何使用HTML、CSS和jQuery創建一個動態的標籤雲標籤雲是一種常見的Web設計元素,它常用於展示網站的標籤或關鍵字,以便用戶快速瀏覽並選擇感興趣的內容。本文將介紹如何使用HTML、CSS和jQuery建立一個動態的標籤雲,並提供具體的程式碼範例。 HTML結構首先,我們需要建立一個基本的HTML結構來容納標籤雲。通常,標籤雲是透過一個包含多個標籤的鏈
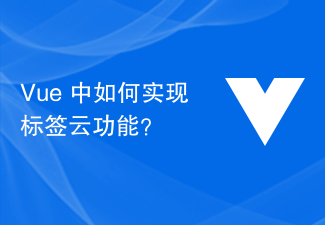
Vue是一個流行的漸進式JavaScript框架,廣泛用於Web開發。對於許多網站,標籤雲是常見的元素,可以顯示網站上的標籤或關鍵字。在本文中,我們將討論如何使用Vue實作標籤雲功能。建立標籤雲元件首先,我們需要建立一個元件來顯示標籤雲。可以使用以下程式碼開始:<template><divclass="ta
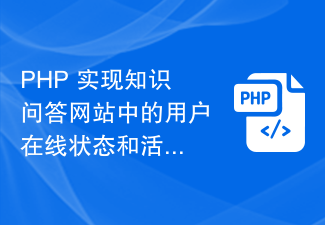
PHP實現知識問答網站中的使用者線上狀態和活動追蹤功能隨著網路的快速發展,知識問答網站逐漸成為人們獲取資訊和分享知識的重要平台。身為知識問答網站的管理員或開發者,你可能會考慮實現使用者線上狀態和活動追蹤功能,以便隨時了解和監控使用者的活動狀況。本文將介紹如何利用PHP來實現這些功能。用戶在線狀態功能實現用戶在線狀態是指用戶目前是否處於網站上活躍狀態。為了實現這
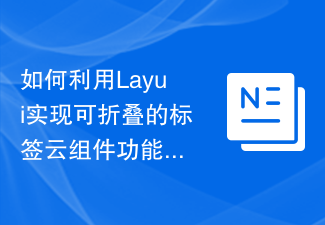
如何利用Layui實現可折疊的標籤雲組件功能概述:標籤雲是一種常見的網頁元素,它可以將標籤按照不同的風格呈現在頁面上,使用戶可以快速瀏覽和選擇感興趣的標籤。而將標籤雲進行可折疊處理,可有效利用頁面空間,增強使用者體驗。在本文中,將介紹如何利用Layui框架實現可折疊的標籤雲組件功能,並提供詳細的程式碼範例。步驟一:匯入Layui框架的相關資源檔案首先,確保你
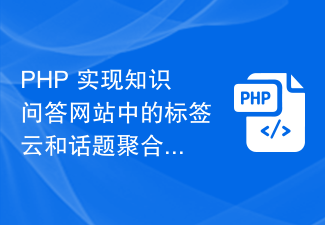
PHP實現知識問答網站中的標籤雲和話題聚合功能在知識問答網站中,標籤雲和話題聚合是兩個重要的功能。標籤雲可以幫助使用者快速了解網站上的熱門話題和常見標籤,方便使用者瀏覽和搜尋相關的問題和答案。而話題聚合則可以將相同標籤的問題和答案集中在一起,提供使用者更方便的檢視和討論方式。下面我們將使用PHP來實現這兩個功能。首先,我們需要建立一個資料庫來儲存問題、
