PHP如何使用MongoDB實作資料的加密和解密
PHP如何使用MongoDB實作資料的加密和解密
在Web開發中,資料的加密和解密是一個非常重要的安全措施。 MongoDB是一種流行的資料庫,它提供了強大的功能和靈活性,可以實現資料的加密和解密。本文將介紹如何在PHP中使用MongoDB實作資料的加密和解密,並提供對應的程式碼範例。
- 安裝MongoDB和PHP擴充
首先,你需要安裝MongoDB資料庫和PHP的MongoDB擴充。你可以透過官方網站下載MongoDB資料庫,並遵循它們的安裝指南。安裝PHP的MongoDB擴充可以使用PECL指令:pecl install mongodb
。安裝完成後,你需要在php.ini檔案中加入extension=mongodb.so
以啟用擴充。 - 連接MongoDB資料庫
使用PHP連接MongoDB資料庫非常簡單。你只需要使用MongoDB類別的建構函式建立一個MongoDB客戶端對象,然後選擇你想要連接的資料庫。以下是一個範例程式碼:
<?php $mongoClient = new MongoDBClient("mongodb://localhost:27017"); $database = $mongoClient->mydatabase; ?>
- 加密資料
MongoDB提供了對稱加密和雜湊加密兩種方式。以下將分別介紹這兩種加密方式的範例程式碼。
3.1 對稱加密
對稱加密是一種使用相同的金鑰進行加密和解密的加密方式。以下是使用MongoDB對稱加密的範例程式碼:
<?php $collection = $database->mycollection; $encryptionData = [ 'name' => 'John Smith', 'email' => 'john@example.com', 'phone' => '1234567890' ]; $encryptionOptions = [ 'keyVaultNamespace' => 'encryption.__keyVault', 'kmsProviders' => [ 'local' => [ 'key' => base64_encode(openssl_random_pseudo_bytes(96)), ], ], 'algorithm' => 'AEAD_AES_256_CBC_HMAC_SHA_512-Random', 'header' => ['keyId' => base64_encode(openssl_random_pseudo_bytes(96))], ]; $command = [ 'encrypt' => $collection->getCollectionName(), 'bsonType' => 'object', 'value' => $encryptionData, 'algorithm' => 'AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic', ]; $encryptedData = $database->command(['encrypt' => [$command, $encryptionOptions]])->toArray()[0]; $collection->insertOne($encryptedData); ?>
3.2 雜湊加密
雜湊加密是一種單向加密方式,它將資料轉換為唯一的雜湊值。以下是使用MongoDB雜湊加密的範例程式碼:
<?php $collection = $database->mycollection; $encryptionData = [ 'name' => 'John Smith', 'email' => 'john@example.com', 'phone' => '1234567890' ]; $command = [ 'hash' => $collection->getCollectionName(), 'value' => $encryptionData, 'algorithm' => 'bcrypt' ]; $hashedData = $database->command($command)->toArray()[0]; $collection->insertOne($hashedData); ?>
- 解密資料
解密加密資料也很簡單。以下是對稱加密和雜湊加密資料解密的範例程式碼:
4.1 對稱解密
對稱解密需要指定金鑰。以下是對稱解密的範例程式碼:
<?php $collection = $database->mycollection; $decryptionOptions = [ 'keyVaultNamespace' => 'encryption.__keyVault', 'kmsProviders' => [ 'local' => [ 'key' => $encryptionOptions['kmsProviders']['local']['key'], ], ], ]; $command = [ 'decrypt' => $collection->getCollectionName(), 'bsonType' => 'object', 'value' => $encryptedData, 'algorithm' => 'AEAD_AES_256_CBC_HMAC_SHA_512-Deterministic', ]; $decryptedData = $database->command(['decrypt' => [$command, $decryptionOptions]])->toArray()[0]; ?>
4.2 雜湊解密
雜湊加密是一種單向加密方式,它不支援解密。如果你需要驗證雜湊加密的數據,你可以使用資料庫提供的驗證功能。
<?php $collection = $database->mycollection; $hashedData = $collection->findOne(['_id' => $documentId]); if (password_verify($inputPassword, $hashedData->password)) { // 密码验证成功,执行相应操作 } else { // 密码验证失败,执行相应操作 } ?>
綜上所述,本文介紹了使用MongoDB實作資料加密和解密的方法,並提供了對稱加密和雜湊加密的程式碼範例。透過合理地應用這些技術,你可以保護你的敏感數據,增強系統的安全性。希望本文對你有幫助!
以上是PHP如何使用MongoDB實作資料的加密和解密的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

本文介紹如何在Debian系統上配置MongoDB實現自動擴容,主要步驟包括MongoDB副本集的設置和磁盤空間監控。一、MongoDB安裝首先,確保已在Debian系統上安裝MongoDB。使用以下命令安裝:sudoaptupdatesudoaptinstall-ymongodb-org二、配置MongoDB副本集MongoDB副本集確保高可用性和數據冗餘,是實現自動擴容的基礎。啟動MongoDB服務:sudosystemctlstartmongodsudosys
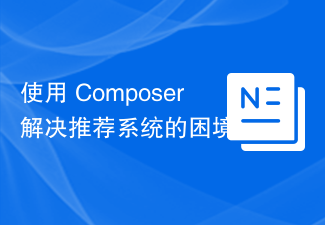
在開發一個電商網站時,我遇到了一個棘手的問題:如何為用戶提供個性化的商品推薦。最初,我嘗試了一些簡單的推薦算法,但效果並不理想,用戶的滿意度也因此受到影響。為了提升推薦系統的精度和效率,我決定採用更專業的解決方案。最終,我通過Composer安裝了andres-montanez/recommendations-bundle,這不僅解決了我的問題,還大大提升了推薦系統的性能。可以通過一下地址學習composer:學習地址
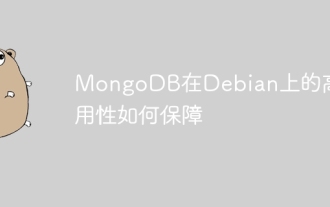
本文介紹如何在Debian系統上構建高可用性的MongoDB數據庫。我們將探討多種方法,確保數據安全和服務持續運行。關鍵策略:副本集(ReplicaSet):利用副本集實現數據冗餘和自動故障轉移。當主節點出現故障時,副本集會自動選舉新的主節點,保證服務的持續可用性。數據備份與恢復:定期使用mongodump命令進行數據庫備份,並製定有效的恢復策略,以應對數據丟失風險。監控與報警:部署監控工具(如Prometheus、Grafana)實時監控MongoDB的運行狀態,並
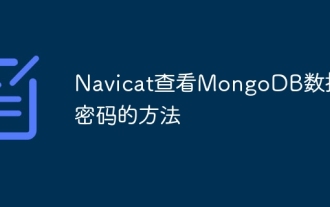
直接通過 Navicat 查看 MongoDB 密碼是不可能的,因為它以哈希值形式存儲。取回丟失密碼的方法:1. 重置密碼;2. 檢查配置文件(可能包含哈希值);3. 檢查代碼(可能硬編碼密碼)。
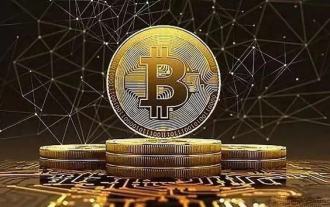
2025年數字虛擬貨幣十大交易平台排行榜:1. OKX,2. Binance,3. Gate.io,4. Kraken,5. Huobi Global,6. Coinbase,7. KuCoin,8. Crypto.com,9. Bitfinex,10. MEXC Global。這些平台因其交易量、用戶體驗、安全性和幣種豐富度等因素被評選為最佳,選擇時需考慮交易費用、平台安全性
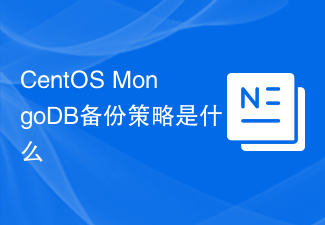
CentOS系統下MongoDB高效備份策略詳解本文將詳細介紹在CentOS系統上實施MongoDB備份的多種策略,以確保數據安全和業務連續性。我們將涵蓋手動備份、定時備份、自動化腳本備份以及Docker容器環境下的備份方法,並提供備份文件管理的最佳實踐。手動備份:利用mongodump命令進行手動全量備份,例如:mongodump-hlocalhost:27017-u用戶名-p密碼-d數據庫名稱-o/備份目錄此命令會將指定數據庫的數據及元數據導出到指定的備份目錄。
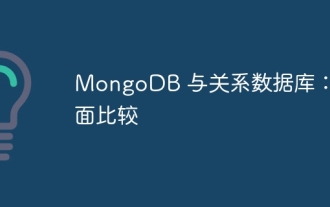
MongoDB與關係型數據庫:深度對比本文將深入探討NoSQL數據庫MongoDB與傳統關係型數據庫(如MySQL和SQLServer)的差異。關係型數據庫採用行和列的表格結構組織數據,而MongoDB則使用靈活的面向文檔模型,更適應現代應用的需求。主要區別數據結構:關係型數據庫使用預定義模式的表格存儲數據,表間關係通過主鍵和外鍵建立;MongoDB使用類似JSON的BSON文檔存儲在集合中,每個文檔結構可獨立變化,實現無模式設計。架構設計:關係型數據庫需要預先定義固定的模式;MongoDB支持
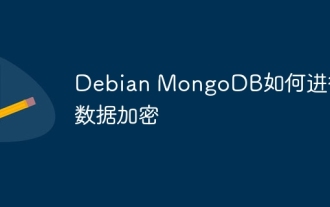
在Debian系統上為MongoDB數據庫加密,需要遵循以下步驟:第一步:安裝MongoDB首先,確保您的Debian系統已安裝MongoDB。如果沒有,請參考MongoDB官方文檔進行安裝:https://docs.mongodb.com/manual/tutorial/install-mongodb-on-debian/第二步:生成加密密鑰文件創建一個包含加密密鑰的文件,並設置正確的權限:ddif=/dev/urandomof=/etc/mongodb-keyfilebs=512
