PHP中的蟻群演算法詳解
PHP中的蟻群演算法詳解
引言:
蟻群演算法(Ant Colony Optimization,ACO)是模擬自然界中螞蟻覓食行為的啟發式演算法。它是以螞蟻找到食物的路徑尋優行為為基礎,透過模擬螞蟻在路徑選擇過程中釋放信息素和感知信息素的行為,尋找問題的最優解。本文將詳細介紹如何使用PHP實作蟻群演算法,並給出對應的程式碼範例。
- 演算法原理
蟻群演算法的基本原理是透過模擬螞蟻在尋找食物的過程中釋放信息素和感知信息素的行為來尋找最優路徑。螞蟻在搜尋食物時,會在路徑上釋放一種稱為費洛蒙的化學物質,其濃度會隨著時間的推移而增加或減少。螞蟻在路徑選擇時,會根據信息素的濃度和距離來判斷,較濃度高的路徑和較短的路徑更容易被選擇。當螞蟻找到食物並返回巢穴時,會在該路徑上釋放更多的信息素,進一步增加該路徑被選擇的機率,以便其他螞蟻也能找到這條路徑。 - PHP實作蟻群演算法
以下是一個簡單的PHP蟻群演算法範例程式碼:
class Ant { public $path; public $visitedCities; public $currentCity; public function __construct($startCity) { $this->path = []; $this->visitedCities = []; $this->currentCity = $startCity; $this->visitedCities[] = $startCity; $this->path[] = $startCity; } public function chooseNextCity($pheromones, $distances) { // 根据信息素和距离计算下一步要选择的城市 // ... } public function updatePath($city) { // 更新路径和访问过的城市列表 // ... } } class AntColonyAlgorithm { public $pheromones; public $distances; public $ants; public $bestPath; public $bestDistance; public function __construct($pheromones, $distances) { $this->pheromones = $pheromones; $this->distances = $distances; $this->ants = []; $this->bestPath = []; $this->bestDistance = PHP_INT_MAX; } public function start($startCity, $numAnts, $iterations) { // 初始化蚂蚁群 // ... for ($i = 0; $i < $iterations; $i++) { // 每个蚂蚁进行路径选择 // ... // 更新信息素 // ... // 更新全局最优解 // ... } return [$this->bestPath, $this->bestDistance]; } public function evaporatePheromones() { // 信息素蒸发 // ... } public function depositPheromones() { // 信息素沉积 // ... } } // 初始化信息素和距离 $pheromones = [ [0, 0.5, 0.2], [0.5, 0, 0.7], [0.2, 0.7, 0] ]; $distances = [ [0, 10, 20], [10, 0, 5], [20, 5, 0] ]; // 创建蚁群算法实例 $aco = new AntColonyAlgorithm($pheromones, $distances); // 启动算法 $startCity = 0; $numAnts = 5; $iterations = 10; list($bestPath, $bestDistance) = $aco->start($startCity, $numAnts, $iterations); // 输出结果 echo "最优路径: ".implode(" -> ", $bestPath)."<br>"; echo "最优解: ".$bestDistance;
以上程式碼是一個簡單的蟻群演算法範例,其中Ant類表示螞蟻對象,AntColonyAlgorithm類別表示蟻群演算法實例。在演算法中,首先需要初始化信息素和距離,然後建立蟻群演算法實例並啟動演算法。演算法會迭代指定次數,每次迭代中,螞蟻會選擇下一步要前往的城市,並根據資訊素更新路徑和造訪過的城市清單。隨著迭代的進行,全域最優解會逐漸更新,最終獲得最佳解。
結論:
蟻群演算法是一種基於螞蟻覓食行為的啟發式演算法,透過模擬螞蟻在路徑選擇過程中釋放信息素和感知信息素的行為,實現尋找最優解的目標。本文中給出了一個簡單的PHP實作蟻群演算法的範例程式碼,供讀者參考學習。希望讀者透過學習蟻群演算法,能夠應用於解決實際問題,並在最佳化問題過程中取得理想的效果。
以上是PHP中的蟻群演算法詳解的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

熱門話題
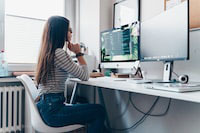
這篇文章將為大家詳細講解有關PHP將行格式化為CSV並寫入文件指針,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。將行格式化為CSV並寫入檔案指標步驟1:開啟檔案指標$file=fopen("path/to/file.csv","w");步驟2:將行轉換為CSV字串使用fputcsv( )函數將行轉換為CSV字串。此函數接受以下參數:$file:檔案指標$fields:作為陣列的CSV欄位$delimiter:欄位分隔符號(可選)$enclosure:欄位引號(
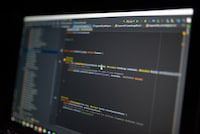
這篇文章將為大家詳細講解有關PHP改變當前的umask,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP更改目前的umask概述umask是一個用於設定新建立的檔案和目錄的預設檔案權限的php函數。它接受一個參數,這是一個八進制數字,表示要阻止的權限。例如,要阻止對新建立的檔案進行寫入權限,可以使用002。更改umask的方法有兩種方法可以更改PHP中的目前umask:使用umask()函數:umask()函數直接變更目前umask。其語法為:intumas
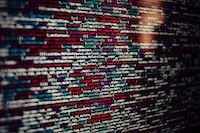
這篇文章將為大家詳細講解有關PHP建立一個具有唯一文件名的文件,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。在PHP中建立唯一檔案名稱的檔案簡介在php中建立具有唯一檔案名稱的檔案對於組織和管理檔案系統至關重要。唯一文件名稱可確保不會覆蓋現有文件,並便於尋找和檢索特定文件。本指南將介紹在PHP中產生唯一檔案名稱的幾種方法。方法1:使用uniqid()函數uniqid()函數產生一個基於當前時間和微秒的唯一字串。此字串可以作為檔案名稱的基礎。
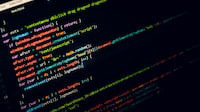
這篇文章將為大家詳細講解有關PHP計算文件的MD5散列,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP計算檔案的MD5雜湊MD5(MessageDigest5)是一種單向加密演算法,可將任意長度的訊息轉換為固定長度的128位元雜湊值。它廣泛用於確保文件完整性、驗證資料真實性和建立數位簽章。在PHP中計算檔案的MD5雜湊php提供了多種方法來計算檔案的MD5雜湊:使用md5_file()函數md5_file()函數直接計算檔案的MD5雜湊值,傳回一個32個字元的
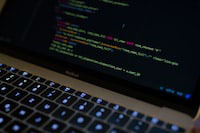
這篇文章將為大家詳細講解有關PHP返回一個鍵值翻轉後的數組,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP鍵值翻轉數組鍵值翻轉是一種對數組進行的操作,它將數組中的鍵和值進行交換,產生一個新的數組,其中原始鍵作為值,原始值作為鍵。實作方法在php中,可以透過以下方法對陣列進行鍵值翻轉:array_flip()函數:array_flip()函數專門用於鍵值翻轉操作。它接收一個數組作為參數,並傳回一個新的數組,其中鍵和值已交換。 $original_array=[
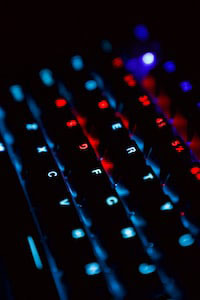
這篇文章將為大家詳細講解有關PHP將文件截斷到給定的長度,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP檔案截斷簡介php中的file_put_contents()函數可用來將檔案截斷到指定長度。截斷是指刪除檔案末端的部分內容,從而縮短檔案長度。語法file_put_contents($filename,$data,SEEK_SET,$offset);$filename:要截斷的檔案路徑。 $data:要寫入檔案的空字串。 SEEK_SET:指定為檔案開始處
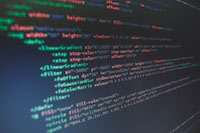
這篇文章將為大家詳細講解有關PHP判斷某個數組中是否存在指定的key,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP判斷某個陣列中是否存在指定的key:在php中,判斷某個陣列中是否存在指定的key的方法有多種:1.使用isset()函數:isset($array["key"])此函數傳回布林值,如果指定的key存在,則傳回true,否則傳回false。 2.使用array_key_exists()函數:array_key_exists("key",$arr

這篇文章將為大家詳細講解有關PHP返回上一個Mysql操作中的錯誤訊息的數字編碼,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。利用PHP回傳MySQL錯誤訊息數字編碼引言在處理mysql查詢時,可能會遇到錯誤。為了有效處理這些錯誤,了解錯誤訊息數字編碼至關重要。本文將指導您使用php取得Mysql錯誤訊息數字編碼。取得錯誤訊息數字編碼的方法1.mysqli_errno()mysqli_errno()函數傳回目前MySQL連線的最近錯誤號碼。文法如下:$erro
