借助Go的SectionReader模組,如何處理檔案指定部分的並發讀取與寫入?
借助Go的SectionReader模組,如何處理檔案指定部分的並發讀取與寫入?
在處理大檔案時,我們可能需要同時讀取和寫入檔案的不同部分。 Go語言中的SectionReader模組可以幫助我們進行指定部分的讀取操作。同時,Go語言的goroutine和channel機制,使得並發讀取與寫入變得簡單有效率。本文將介紹如何利用SectionReader模組以及goroutine和channel來實現文件指定部分的並發讀取與寫入。
首先,我們要先了解SectionReader模組的基本用法。 SectionReader是一個根據給定的io.ReaderAt介面(一般為文件)和指定範圍(offset和limit)創建的結構體。此結構體可以實現對檔案指定部分的讀取操作。下面是一個範例程式碼:
package main import ( "fmt" "io" "os" ) func main() { file, err := os.Open("example.txt") if err != nil { fmt.Println("Open file error:", err) return } defer file.Close() section := io.NewSectionReader(file, 10, 20) // 从第10个字节开始,读取20个字节 buffer := make([]byte, 20) n, err := section.Read(buffer) if err != nil { fmt.Println("Read error:", err) return } fmt.Printf("Read %d bytes: %s ", n, buffer[:n]) }
在上述程式碼中,我們先開啟了一個名為example.txt的文件,並使用NewSectionReader函數建立了一個SectionReader實例。此實例指定了從檔案的第10個位元組開始,並讀取20個位元組。然後,我們建立了一個20位元組大小的緩衝區,透過Read方法從SectionReader讀取數據,並列印到控制台上。
接下來,我們將利用goroutine和channel來實現檔案指定部分的並發讀取與寫入。假設我們有一個1000位元組大小的文件,我們希望同時從文件的前半部分和後半部分讀取數據,並將其寫入到兩個不同的文件中。以下是範例程式碼:
package main import ( "fmt" "io" "os" "sync" ) func main() { file, err := os.Open("example.txt") if err != nil { fmt.Println("Open file error:", err) return } defer file.Close() var wg sync.WaitGroup wg.Add(2) buffer1 := make(chan []byte) buffer2 := make(chan []byte) go func() { defer wg.Done() section := io.NewSectionReader(file, 0, 500) data := make([]byte, 500) _, err := section.Read(data) if err != nil { fmt.Println("Read error:", err) return } buffer1 <- data }() go func() { defer wg.Done() section := io.NewSectionReader(file, 500, 500) data := make([]byte, 500) _, err := section.Read(data) if err != nil { fmt.Println("Read error:", err) return } buffer2 <- data }() go func() { file1, err := os.Create("output1.txt") if err != nil { fmt.Println("Create file1 error:", err) return } defer file1.Close() data := <-buffer1 file1.Write(data) }() go func() { file2, err := os.Create("output2.txt") if err != nil { fmt.Println("Create file2 error:", err) return } defer file2.Close() data := <-buffer2 file2.Write(data) }() wg.Wait() }
在上述程式碼中,我們先開啟了一個名為example.txt的文件,並使用兩個SectionReader實例分別指定前半部分和後半部分的範圍。然後,我們創建了兩個用於儲存資料的channel,並使用兩個goroutine來同時讀取檔案的不同部分。每個goroutine讀取資料後,將資料透過對應的channel傳遞給寫入檔案的goroutine。寫入檔案的goroutine再從channel中取得數據,並將其寫入到對應的檔案中。
透過上述範例程式碼,我們可以實現對檔案指定部分的並發讀取與寫入。利用SectionReader模組和goroutine與channel機制,我們可以有效率地處理大檔案的讀取與寫入操作。在實際的應用中,我們可以根據需求進行靈活的調整,並結合其他處理模組來滿足具體需求。
以上是借助Go的SectionReader模組,如何處理檔案指定部分的並發讀取與寫入?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

熱門話題
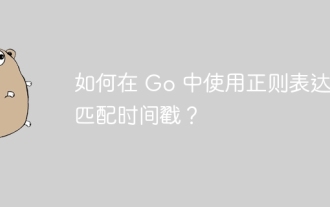
在Go中,可以使用正規表示式比對時間戳記:編譯正規表示式字串,例如用於匹配ISO8601時間戳記的表達式:^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ 。使用regexp.MatchString函數檢查字串是否與正規表示式相符。
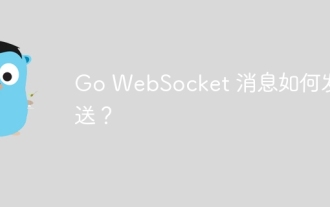
在Go中,可以使用gorilla/websocket包發送WebSocket訊息。具體步驟:建立WebSocket連線。傳送文字訊息:呼叫WriteMessage(websocket.TextMessage,[]byte("訊息"))。發送二進位訊息:呼叫WriteMessage(websocket.BinaryMessage,[]byte{1,2,3})。
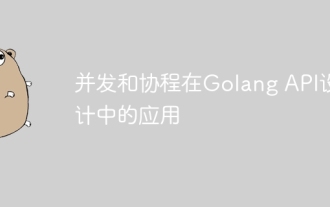
並發和協程在GoAPI設計中可用於:高效能處理:同時處理多個請求以提高效能。非同步處理:使用協程非同步處理任務(例如傳送電子郵件),釋放主執行緒。流處理:使用協程高效處理資料流(例如資料庫讀取)。
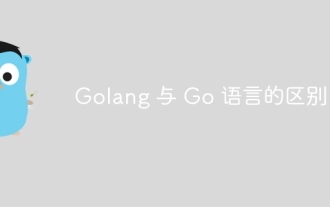
Go和Go語言是不同的實體,具有不同的特性。 Go(又稱Golang)以其並發性、編譯速度快、記憶體管理和跨平台優點而聞名。 Go語言的缺點包括生態系統不如其他語言豐富、文法更嚴格、缺乏動態類型。
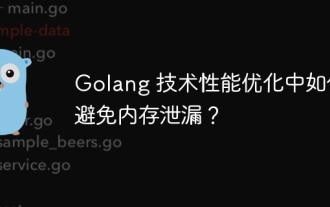
記憶體洩漏會導致Go程式記憶體不斷增加,可通過:關閉不再使用的資源,如檔案、網路連線和資料庫連線。使用弱引用防止記憶體洩漏,當物件不再被強引用時將其作為垃圾回收目標。利用go協程,協程棧記憶體會在退出時自動釋放,避免記憶體洩漏。
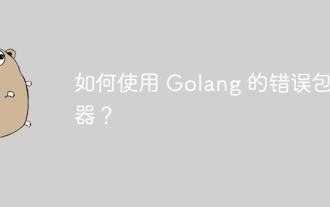
在Golang中,錯誤包裝器允許你在原始錯誤上追加上下文訊息,從而創建新錯誤。這可用於統一不同程式庫或元件拋出的錯誤類型,簡化偵錯和錯誤處理。步驟如下:使用errors.Wrap函數將原有錯誤包裝成新錯誤。新錯誤包含原始錯誤的上下文資訊。使用fmt.Printf輸出包裝後的錯誤,提供更多上下文和可操作性。在處理不同類型的錯誤時,使用errors.Wrap函數統一錯誤類型。
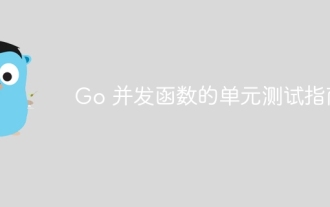
對並發函數進行單元測試至關重要,因為這有助於確保其在並發環境中的正確行為。測試並發函數時必須考慮互斥、同步和隔離等基本原理。可以透過模擬、測試競爭條件和驗證結果等方法對並發函數進行單元測試。
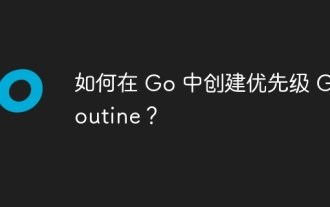
在Go語言中建立優先權Goroutine有兩步驟:註冊自訂Goroutine建立函數(步驟1)並指定優先權值(步驟2)。這樣,您可以建立不同優先順序的Goroutine,優化資源分配並提高執行效率。
