如何使用MySQL在Ruby on Rails中實作資料模型關聯功能
如何使用MySQL在Ruby on Rails中實作資料模型關聯功能
在Ruby on Rails開發中,資料庫的設計和關聯是非常重要的一環。而MySQL是一種常用的關聯式資料庫,它具有強大的功能和靈活的查詢語言,是Ruby on Rails中常用的資料庫之一。本文將詳細介紹如何使用MySQL在Ruby on Rails中實作資料模型關聯功能,並提供程式碼範例。
- 資料模型設計
在使用MySQL實作資料模型關聯功能之前,我們首先需要設計好資料庫的表格結構和模型之間的關係。在MySQL中,常用的關聯關係有一對一、一對多、多對多三種。
- 一對一關聯:兩個表之間只存在一個對應關係,例如使用者(User)和身分證(IDCard),一個使用者只對應一個身分證,一個身分證也只對應一個用戶。
- 一對多關聯:一個表中的記錄可以對應另一個表中的多個記錄,例如使用者(User)和訂單(Order),一個使用者可以擁有多個訂單,但一個訂單只能屬於一個使用者。
- 多對多重關聯:兩個表之間存在多個對應關係,例如使用者(User)和角色(Role),一個使用者可以擁有多個角色,一個角色也可以被多個使用者擁有。
- 建立模型和資料庫遷移
在Ruby on Rails中,我們使用命令列建立模型和資料庫遷移來定義和建立資料庫表和模型。以下是如何建立三種關聯關係的模型和資料庫遷移的範例程式碼:
- 一對一關聯:
# 创建用户模型 rails generate model User name:string # 创建身份证模型 rails generate model IDCard number:integer # 编辑迁移文件 class CreateIDCards < ActiveRecord::Migration[6.1] def change create_table :id_cards do |t| t.integer :number t.references :user # 添加用户外键 t.timestamps end end end # 运行数据库迁移 rails db:migrate # 编辑用户模型 class User < ApplicationRecord has_one :id_card # 声明一对一关联关系 end # 编辑身份证模型 class IDCard < ApplicationRecord belongs_to :user # 声明一对一关联关系 end
- 一對一關聯:
# 创建用户模型 rails generate model User name:string # 创建订单模型 rails generate model Order number:integer user:references # 编辑迁移文件 class CreateOrders < ActiveRecord::Migration[6.1] def change create_table :orders do |t| t.integer :number t.references :user # 添加用户外键 t.timestamps end end end # 运行数据库迁移 rails db:migrate # 编辑用户模型 class User < ApplicationRecord has_many :orders # 声明一对多关联关系 end # 编辑订单模型 class Order < ApplicationRecord belongs_to :user # 声明一对多关联关系 end
- 多重對多重關聯:
# 创建用户模型 rails generate model User name:string # 创建角色模型 rails generate model Role name:string # 编辑迁移文件 class CreateRolesUsers < ActiveRecord::Migration[6.1] def change create_table :roles_users, id: false do |t| t.references :role t.references :user end end end # 运行数据库迁移 rails db:migrate # 编辑用户模型 class User < ApplicationRecord has_and_belongs_to_many :roles # 声明多对多关联关系 end # 编辑角色模型 class Role < ApplicationRecord has_and_belongs_to_many :users # 声明多对多关联关系 end
- #資料關聯操作
在資料庫的關聯關係建立之後,我們可以進行資料的關聯操作,例如建立關聯資料、查詢關聯資料、更新關聯資料等等。以下是對三種關聯關係進行操作的範例程式碼:
- 一對一關聯:
# 创建用户和身份证 user = User.create(name: "John") id_card = IDCard.create(number: 123456, user: user) # 查询用户的身份证 user.id_card # 查询身份证的用户 id_card.user
- 一對一關聯:
# 创建用户和订单 user = User.create(name: "John") order1 = Order.create(number: 1, user: user) order2 = Order.create(number: 2, user: user) # 查询用户的订单 user.orders # 查询订单的用户 order1.user order2.user
# 创建用户和角色 user1 = User.create(name: "John") user2 = User.create(name: "Tom") role1 = Role.create(name: "Admin") role2 = Role.create(name: "User") # 建立用户和角色的关联 user1.roles << role1 user1.roles << role2 user2.roles << role2 # 查询用户的角色 user1.roles user2.roles # 查询角色的用户 role1.users role2.users
以上是如何使用MySQL在Ruby on Rails中實作資料模型關聯功能的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

熱門話題
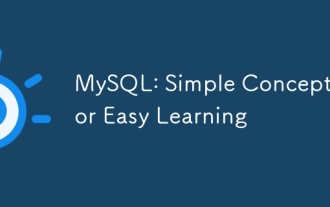
MySQL是一個開源的關係型數據庫管理系統。 1)創建數據庫和表:使用CREATEDATABASE和CREATETABLE命令。 2)基本操作:INSERT、UPDATE、DELETE和SELECT。 3)高級操作:JOIN、子查詢和事務處理。 4)調試技巧:檢查語法、數據類型和權限。 5)優化建議:使用索引、避免SELECT*和使用事務。
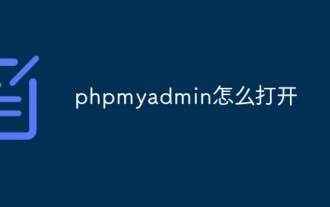
可以通過以下步驟打開 phpMyAdmin:1. 登錄網站控制面板;2. 找到並點擊 phpMyAdmin 圖標;3. 輸入 MySQL 憑據;4. 點擊 "登錄"。
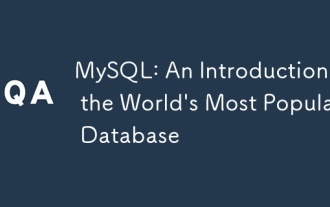
MySQL是一種開源的關係型數據庫管理系統,主要用於快速、可靠地存儲和檢索數據。其工作原理包括客戶端請求、查詢解析、執行查詢和返回結果。使用示例包括創建表、插入和查詢數據,以及高級功能如JOIN操作。常見錯誤涉及SQL語法、數據類型和權限問題,優化建議包括使用索引、優化查詢和分錶分區。
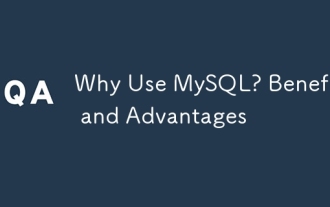
選擇MySQL的原因是其性能、可靠性、易用性和社區支持。 1.MySQL提供高效的數據存儲和檢索功能,支持多種數據類型和高級查詢操作。 2.採用客戶端-服務器架構和多種存儲引擎,支持事務和查詢優化。 3.易於使用,支持多種操作系統和編程語言。 4.擁有強大的社區支持,提供豐富的資源和解決方案。

Redis 使用單線程架構,以提供高性能、簡單性和一致性。它利用 I/O 多路復用、事件循環、非阻塞 I/O 和共享內存來提高並發性,但同時存在並發性受限、單點故障和不適合寫密集型工作負載的局限性。
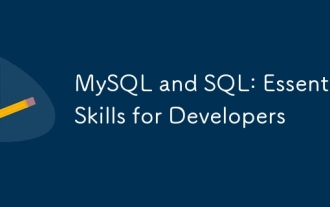
MySQL和SQL是開發者必備技能。 1.MySQL是開源的關係型數據庫管理系統,SQL是用於管理和操作數據庫的標準語言。 2.MySQL通過高效的數據存儲和檢索功能支持多種存儲引擎,SQL通過簡單語句完成複雜數據操作。 3.使用示例包括基本查詢和高級查詢,如按條件過濾和排序。 4.常見錯誤包括語法錯誤和性能問題,可通過檢查SQL語句和使用EXPLAIN命令優化。 5.性能優化技巧包括使用索引、避免全表掃描、優化JOIN操作和提升代碼可讀性。
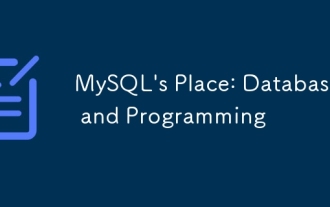
MySQL在數據庫和編程中的地位非常重要,它是一個開源的關係型數據庫管理系統,廣泛應用於各種應用場景。 1)MySQL提供高效的數據存儲、組織和檢索功能,支持Web、移動和企業級系統。 2)它使用客戶端-服務器架構,支持多種存儲引擎和索引優化。 3)基本用法包括創建表和插入數據,高級用法涉及多表JOIN和復雜查詢。 4)常見問題如SQL語法錯誤和性能問題可以通過EXPLAIN命令和慢查詢日誌調試。 5)性能優化方法包括合理使用索引、優化查詢和使用緩存,最佳實踐包括使用事務和PreparedStatemen
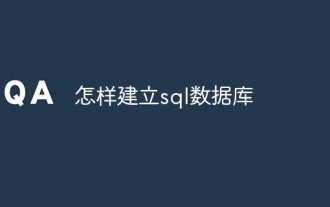
構建 SQL 數據庫涉及 10 個步驟:選擇 DBMS;安裝 DBMS;創建數據庫;創建表;插入數據;檢索數據;更新數據;刪除數據;管理用戶;備份數據庫。
