Python程式旋轉列表元素
在Python中,可以使用清單來在單一變數中維護多個項目。清單是Python的四種內建資料類型之一,用於儲存資料集合。另外三種類型,元組、集合和字典,各自有不同的功能。列表使用方括號進行建構。由於清單不必是同質的,它們是Python中最有用的工具。一個列表可以包含字串、物件和整數等資料類型。列表可以在生成後進行修改,因為它們是可變的。
本文的重點是速記和用一句話或一個字來表達這個概念的許多捷徑。這個操作對程式設計師來說非常重要,可以完成許多工作。我們將使用Python來展示四種不同的方法來完成這個任務。
Using The List Comprehension
在使用這種方法時,我們只需要在特定位置旋轉後重新分配清單中每個元素的索引。由於其較小的實現,這種方法在完成任務中起著重要作用。
Algorithm
- Defining a list first.
- #Use the list comprehension.
- #For applying two different sides right(i-index) and left(i index).
- Print the output list.
文法
#左旋轉
list_1 = [list_1[(i + 3) % len(list_1)]
list_1 = [list_1[(i - 3) % len(list_1)]
登入後複製
Examplelist_1 = [list_1[(i - 3) % len(list_1)]
Here, in this code we have used the list comprehension to rotate the elements in a list that is the right and left rotate. For loop is used to iterate through the list of elements.
list_1 = [10, 14, 26, 37, 42]
print (" Primary list : " + str(list_1))
list_1 = [list_1[(i + 3) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after left rotate by 3 : " + str(list_1))
list_1 = [list_1[(i - 3) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after right rotate by 3(back to primary list) : "+str(list_1))
list_1 = [list_1[(i + 2) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after left rotate by 2 : " + str(list_1))
list_1 = [list_1[(i - 2) % len(list_1)]
for i, x in enumerate(list_1)]
print ("Output of the list after right rotate by 2 : "+ str(list_1))
登入後複製
輸出list_1 = [10, 14, 26, 37, 42] print (" Primary list : " + str(list_1)) list_1 = [list_1[(i + 3) % len(list_1)] for i, x in enumerate(list_1)] print ("Output of the list after left rotate by 3 : " + str(list_1)) list_1 = [list_1[(i - 3) % len(list_1)] for i, x in enumerate(list_1)] print ("Output of the list after right rotate by 3(back to primary list) : "+str(list_1)) list_1 = [list_1[(i + 2) % len(list_1)] for i, x in enumerate(list_1)] print ("Output of the list after left rotate by 2 : " + str(list_1)) list_1 = [list_1[(i - 2) % len(list_1)] for i, x in enumerate(list_1)] print ("Output of the list after right rotate by 2 : "+ str(list_1))
Primary list : [10, 14, 26, 37, 42] Output of the list after left rotate by 3 : [37, 42, 10, 14, 26] Output of the list after right rotate by 3(back to primary list) : [10, 14, 26, 37, 42] Output of the list after left rotate by 2 : [26, 37, 42, 10, 14] Output of the list after right rotate by 2 : [10, 14, 26, 37, 42]
使用切片
This specific technique is the standard technique. With the rotation number, it simply joins the later-sliced component to the earlier-sliced part.
Algorithm
- Defining a list first.
- #Use slicing method.
- #在右旋和左旋後列印每個清單。
文法
FOR SLICING
#左旋轉 -
list_1 = list_1[3:] + list_1[:3]
list_1 = list_1[-3:] + list_1[:-3]
登入後複製
Examplelist_1 = list_1[-3:] + list_1[:-3]
The following program rearranges the elements of a list. The original list is [11, 34, 26, 57, 92]. First rotate 3 units to the left. That is, the first three elements are moved to the end, the first three elements are moved to the end, resulting in [57, 92, 11, 34, 26]. Then rotate right by 3 so the last three elements move back and forth to their original positions [11,34,26,57,92].
接著向右旋轉2次,使最後兩個元素向前移動,得到 [26, 57, 92, 11, 34]。最後向左旋轉1次,將一個元素從開頭移到結尾,得到 [57, 92, 11, 34, 26]。
list_1 = [11, 34, 26, 57, 92]
print (" Primary list : " + str(list_1))
list_1 = list_1[3:] + list_1[:3]
print ("Output of the list after left rotate by 3 : " + str(list_1))
list_1 = list_1[-3:] + list_1[:-3]
print ("Output of the list after right rotate by 3(back to Primary list) : "+str(list_1))
list_1 = list_1[-2:] + list_1[:-2]
print ("Output of the list after right rotate by 2 : "+ str(list_1))
list_1 = list_1[1:] + list_1[:1]
print ("Output of the list after left rotate by 1 : " + str(list_1))
登入後複製
輸出list_1 = [11, 34, 26, 57, 92] print (" Primary list : " + str(list_1)) list_1 = list_1[3:] + list_1[:3] print ("Output of the list after left rotate by 3 : " + str(list_1)) list_1 = list_1[-3:] + list_1[:-3] print ("Output of the list after right rotate by 3(back to Primary list) : "+str(list_1)) list_1 = list_1[-2:] + list_1[:-2] print ("Output of the list after right rotate by 2 : "+ str(list_1)) list_1 = list_1[1:] + list_1[:1] print ("Output of the list after left rotate by 1 : " + str(list_1))
Primary list : [11, 34, 26, 57, 92]
Output of the list after left rotate by 3 : [57, 92, 11, 34, 26]
Output of the list after right rotate by 3(back to Primary list) : [11, 34, 26, 57, 92]
Output of the list after right rotate by 2 : [57, 92, 11, 34, 26]
Output of the list after left rotate by 1 : [92, 11, 34, 26, 57]
登入後複製
Using The Numpy ModulePrimary list : [11, 34, 26, 57, 92] Output of the list after left rotate by 3 : [57, 92, 11, 34, 26] Output of the list after right rotate by 3(back to Primary list) : [11, 34, 26, 57, 92] Output of the list after right rotate by 2 : [57, 92, 11, 34, 26] Output of the list after left rotate by 1 : [92, 11, 34, 26, 57]
使用給定的軸,我們也可以使用python中的numpy.roll模組來旋轉清單中的元素。輸入數組的元素會因此被移動。如果一個元素從第一個位置移動到最後一個位置,它會回到初始位置。
Algorithm
- 導入numpy.roll模組
- Define the list and give the particular index.
- Print the output list.
Example
A list 'number' is created and assigned the values 1, 2, 4, 10, 18 and 83. The variable i is set to 1. The np.roll()
function from the NumPy library is then used on the list number with an argument of i which shifts each element in the list by 1 index position (the first element becomes last).
import numpy as np
if __name__ == '__main__':
number = [1, 2, 4, 10, 18, 83]
i = 1
x = np.roll(number, i)
print(x)
登入後複製
輸出import numpy as np if __name__ == '__main__': number = [1, 2, 4, 10, 18, 83] i = 1 x = np.roll(number, i) print(x)
[83 1 2 4 10 18]
登入後複製
Usingcollections.deque.rotate()[83 1 2 4 10 18]
rotate()函數是由collections模組中的deque類別提供的內建函數,用於實現旋轉操作。儘管不太為人所知,但這個函數更加實用。
Algorithm
- 首先從collection模組匯入deque類別。
- 定義一個列表
- #列印主要清單
- #使用rotate()函數旋轉元素
- Print the output.
Example
The following program uses the deque data structure from the collections module to rotate a list. The original list is printed, then it rotates left by 3 and prints out the new rotated list. It then rotates right to position ) by 3 and prints out the resulting list.
from collections import deque
list_1 = [31, 84, 76, 97, 82]
print ("Primary list : " + str(list_1))
list_1 = deque(list_1)
list_1.rotate(-3)
list_1 = list(list_1)
print ("Output list after left rotate by 3 : " + str(list_1))
list_1 = deque(list_1)
list_1.rotate(3)
list_1 = list(list_1)
print ("Output list after right rotate by 3(back to primary list) : "+ str(list_1))
登入後複製
輸出from collections import deque list_1 = [31, 84, 76, 97, 82] print ("Primary list : " + str(list_1)) list_1 = deque(list_1) list_1.rotate(-3) list_1 = list(list_1) print ("Output list after left rotate by 3 : " + str(list_1)) list_1 = deque(list_1) list_1.rotate(3) list_1 = list(list_1) print ("Output list after right rotate by 3(back to primary list) : "+ str(list_1))
Primary list : [31, 84, 76, 97, 82]
Output list after left rotate by 3 : [97, 82, 31, 84, 76]
Output list after right rotate by 3(back to primary list) : [31, 84, 76, 97, 82]
登入後複製
結論
Primary list : [31, 84, 76, 97, 82] Output list after left rotate by 3 : [97, 82, 31, 84, 76] Output list after right rotate by 3(back to primary list) : [31, 84, 76, 97, 82]
在本文中,我們簡要地解釋了四種不同的方法來旋轉清單中的元素。 ###
以上是Python程式旋轉列表元素的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

如何使用Python的count()函數計算清單中某個元素的數量,需要具體程式碼範例Python作為一種強大且易學的程式語言,提供了許多內建函數來處理不同的資料結構。其中之一就是count()函數,它可以用來計算清單中某個元素的數量。在本文中,我們將詳細介紹如何使用count()函數,並提供具體的程式碼範例。 count()函數是Python的內建函數,用來計算某
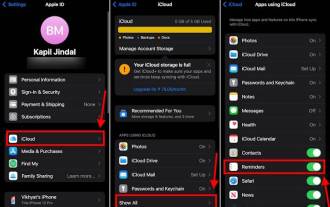
如何在iOS17中的iPhone上製作GroceryList在「提醒事項」應用程式中建立GroceryList非常簡單。你只需添加一個列表,然後用你的項目填充它。該應用程式會自動將您的商品分類,您甚至可以與您的伴侶或扁平夥伴合作,列出您需要從商店購買的東西。以下是執行此操作的完整步驟:步驟1:開啟iCloud提醒事項聽起來很奇怪,蘋果表示您需要啟用來自iCloud的提醒才能在iOS17上建立GroceryList。以下是它的步驟:前往iPhone上的「設定」應用,然後點擊[您的姓名]。接下來,選擇i
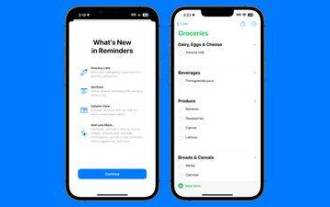
在iOS17中,Apple在提醒應用程式中添加了一個方便的小清單功能,以便在您外出購買雜貨時為您提供幫助。繼續閱讀以了解如何使用它並縮短您的商店之旅。當您使用新的「雜貨」清單類型(在美國以外名為「購物」)建立清單時,您可以輸入各種食品和雜物,並按類別自動組織它們。該組織使您在雜貨店或外出購物時更容易找到您需要的東西。提醒中可用的類別類型包括農產品、麵包和穀物、冷凍食品、零食和糖果、肉類、乳製品、雞蛋和奶酪、烘焙食品、烘焙食品、家居用品、個人護理和健康以及葡萄酒、啤酒和烈酒。以下是在iOS17創
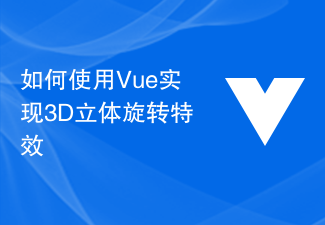
如何使用Vue實現3D立體旋轉特效作為一種流行的前端框架,Vue.js在開發動態網頁和應用程式中扮演著重要的角色。它提供了一種直覺、高效的方式來建立互動式介面,並且易於整合和擴展。本文將介紹如何使用Vue.js實作一個令人驚嘆的3D立體旋轉特效,並提供具體的程式碼範例。在開始之前,請確保您已經安裝了Vue.js,並且對Vue.js的基本用法有一定的了解。如果您還
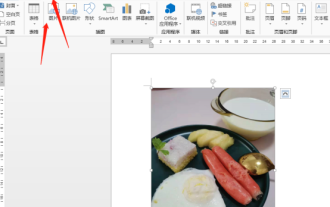
我們在使用Word辦公室軟體進行文件處理的時候,經常需要在文件裡插入一些圖片之類的素材,但是,為了排版美觀的需要,我們還需要將圖片進行一些特殊的排版,其中旋轉處理是最基本的排版處理,但是,對於一些剛接觸Word辦公室軟體的職場新人來講,可能還不太會在Word文檔裡處理圖片。下邊,我們就分享一下Word圖片怎麼旋轉的方法,希望對你有幫助和啟發。 1.首先,我們打開一個Word文檔,隨後,我們選單列點擊插入-圖片按鈕,電腦中隨意找一張圖片插入,以便於我們操作演示使用。 2、如果我們要將圖片旋轉,接著需
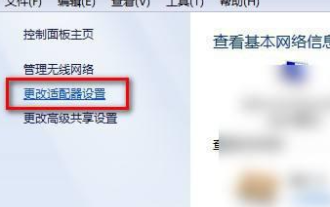
為了方便很多人移動辦公,很多筆記本都帶有無線網的功能,但有些人的電腦上無法顯示WiFi列表,現在就給大家帶來win7系統下遇到這種問題的處理方法,一起來看一下吧。 win7無線網路清單顯示不出來1、右鍵你電腦右下角的網路圖標,選擇“開啟網路和共用中心”,開啟後再點擊左邊的「變更轉接器設定」2、開啟後滑鼠右鍵選擇無線網路轉接器,選擇「診斷」3、等待診斷,如果系統診斷出問題就修復它。 4.修復完成之後,就可以看到WiFi清單了。
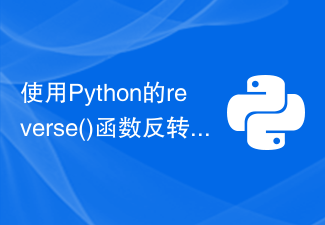
使用Python的reverse()函數反轉列表,需要具體程式碼範例在Python中,我們經常需要在程式設計中對列表進行操作,其中反轉列表是常見的一種需求,這時候我們可以使用Python內建的reverse ()函數來實現。 reverse()函數的作用是反轉列表中的元素順序,即將列表中第一個元素變為最後一個元素,第二個元素變為倒數第二個元素,以此類推。下面是使用Py
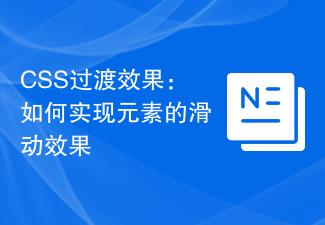
CSS過渡效果:如何實現元素的滑動效果引言:在網頁設計中,元素的動態效果能夠提升使用者體驗,其中滑動效果是常見而又受歡迎的過渡效果。透過CSS的過渡屬性,我們可以輕鬆實現元素的滑動動畫效果。本文將介紹如何使用CSS過渡屬性來實現元素的滑動效果,並提供具體的程式碼範例,以幫助讀者更好地理解和應用。一、CSS過渡屬性transition的簡介CSS過渡屬性tra
