Java程式以左對角線排序2D數組
在資料結構領域中,vector是特定物件的可增長的類別數組。 vector類屬於遺留類,與集合完全相容。在java.util套件中,List介面可以使用這裡列出的所有方法。這裡的初始容量是10,一般方法是−
Vector<E> v = new Vector<E>();
compare()方法接受兩個參數,然後使用Java環境邏輯進行比較。
對2D數組依左對角線排序的演算法
Here is the particular algorithm to sort the 2D array across left diagonal.
第一步 - 開始。
步驟 2 - 逐一遍歷所有左對角線。
Step 3 − Add elements on that left diagonal in the vector.
第四步 - 處理這些向量。
第五步 - 再次進行排序。
Step 6 − Push them back from vector to left diagonal.
第7步 - 刪除所有向量,使集合為空。
第8步 - 再次開始全新的排序。
第九步 - 再次重複所有步驟。
第10步 - 逐步完成所有左對角線。
第11步 - 終止進程。
在左對角線上對2D陣列進行排序的語法
在這裡,我們有一些特定的語法來沿著左對角線拍攝一些2D數組,如下所示:
A. removeAll():
Syntax:
Vector.removeAll(Vectors As Value) It is used to remove all the elements from the vector.
B. Collections.sort():
Syntax:
Collections.sort(Vectors As Value) This method is used to sort the vector in a process.
C. add():
Syntax:
Vector.add(Value as the integer value) It is used to add some elements in the vector.
D. get():
Syntax:
Vector.get(3); This method used to store the vector element at a pricular index.
在這些特定的語法中,我們嘗試將一些2D陣列進行沿左對角線排序。
對2D陣列進行左對角線排序的方法
方法1 - Java程式對2D陣列依照左對角線排序
方法2 - Java程式以對角線遞減順序對2D矩陣進行排序
#方法3 - Java程式對2D矩陣進行對角線排序並取得其總和
#Java程式:將2D陣列依照左對角線進行排序
In this Java code we have tried to show how to sort a 2D array across the left diagonal in a general manner.
Example 1
的中文翻譯為:範例1
#import java.io.*; import java.lang.*; import java.util.*; public class ARBRDD { public static void main(String[] args) throws java.lang.Exception{ int[][] arr = { { 5, 2, 0, 7, 1 }, { 3, 4, 2, 9, 14 }, { 5, 1, 3, 5, 2 }, { 4, 2, 6, 2, 1 }, { 0, 6, 3, 5, 1 }, { 1, 4, 7, 2, 8 } }; System.out.println("Matrix without sorting data is here ----> \n"); for (int i = 0; i < 5; i++) { for (int j = 0; j < 5; j++) { System.out.print(arr[i][j] + " "); } System.out.println(); } Vector<Integer> v = new Vector<>(); for (int i = 0; i < 5; i++) { v.add(arr[i][i]); } Collections.sort(v); for (int j = 0; j < 5; j++) { arr[j][j] = v.get(j); } System.out.println("Matrix after sorting data is here ----> \n"); for (int i = 0; i < 5; i++) { for (int j = 0; j < 5; j++) { System.out.print(arr[i][j] + " "); } System.out.println(); } } }
輸出
Matrix without sorting data is here ----> 5 2 0 7 1 3 4 2 9 14 5 1 3 5 2 4 2 6 2 1 0 6 3 5 1 Matrix after sorting data is here ----> 1 2 0 7 1 3 2 2 9 14 5 1 3 5 2 4 2 6 4 1 0 6 3 5 5
Java程式以對角線遞減順序對2D矩陣進行排序
在這段Java程式碼中,我們嘗試展示如何以遞減的方式對一個2D數組矩陣沿著左對角線進行排序。
Example 2
的中文翻譯為:範例2
import java.io.*; import java.util.*; public class ARBRDD { public static void diagonalSort(ArrayList<ArrayList<Integer> > mat){ int row = mat.size(); int col = mat.get(0).size(); ArrayList<ArrayList<Integer> > Neg = new ArrayList<ArrayList<Integer> >(); ArrayList<ArrayList<Integer> > Pos = new ArrayList<ArrayList<Integer> >(); int i, j; for (i = 0; i < row; i++) { ArrayList<Integer> temp = new ArrayList<Integer>(); Neg.add(temp); } for (j = 0; j < col; j++) { ArrayList<Integer> temp = new ArrayList<Integer>(); Pos.add(temp); } for (i = 0; i < row; i++) { for (j = 0; j < col; j++) { if (j < i) { Neg.get(i - j).add(mat.get(i).get(j)); } else if (i < j) { Pos.get(j - i).add(mat.get(i).get(j)); } else { Pos.get(0).add(mat.get(i).get(j)); } } } for (i = 0; i < row; i++) { Collections.sort(Neg.get(i)); ; } for (i = 0; i < col; i++) { Collections.sort(Pos.get(i)); ; } for (i = 0; i < row; i++) { for (j = 0; j < col; j++) { if (j < i) { int d = i - j; int l = Neg.get(d).size(); mat.get(i).set(j, Neg.get(d).get(l - 1)); Neg.get(d).remove(l - 1); } else if (i < j) { int d = j - i; int l = Pos.get(d).size(); mat.get(i).set(j, Pos.get(d).get(l - 1)); Pos.get(d).remove(l - 1); } else { int l = Pos.get(0).size(); mat.get(i).set(j, Pos.get(0).get(l - 1)); Pos.get(0).remove(l - 1); } } } for (i = 0; i < row; i++) { for (j = 0; j < col; j++) { System.out.print(mat.get(i).get(j) + " "); } System.out.println(); } } public static void main(String[] args){ ArrayList<ArrayList<Integer> > arr = new ArrayList<ArrayList<Integer> >(); ArrayList<Integer> row1 = new ArrayList<Integer>(); row1.add(10); row1.add(2); row1.add(3); arr.add(row1); ArrayList<Integer> row2 = new ArrayList<Integer>(); row2.add(4); row2.add(5); row2.add(6); arr.add(row2); ArrayList<Integer> row3 = new ArrayList<Integer>(); row3.add(7); row3.add(8); row3.add(9); arr.add(row3); diagonalSort(arr); } }
輸出
10 6 3 8 9 2 7 4 5
Java程式對2D矩陣進行對角線排序並取得其總和
在這段Java程式碼中,我們嘗試展示如何對一個2D數組矩陣沿著左對角線進行排序,並得到其總和。
Example 3
import java.util.*; public class ARBRDD{ public static void main(String args[]){ Scanner sc = new Scanner(System.in); int i,j,row,col,sum=0; System.out.println("Enter the number of rows ---->:"); row = sc.nextInt(); System.out.println("Enter the number of columns---->:"); col = sc.nextInt(); int[][] mat = new int[row][col]; System.out.println("Enter the elements of the matrix: -----@") ; for(i=0;i<row;i++){ for(j=0;j<col;j++){ mat[i][j] = sc.nextInt(); } } System.out.println("####The elements of the matrix we get####") ; for(i=0;i<row;i++){ for(j=0;j<col;j++){ System.out.print(mat[i][j]+"\t"); } System.out.println(""); } for(i=0;i<row;i++){ for(j=0;j<col;j++){ if(i==j) { sum = sum + mat[i][j]; } } } System.out.printf("Sum of the diagonal elements of the matrix is here = "+sum) ; } }
輸出
Enter the number of rows ---->: 3 Enter the number of columns---->: 3 Enter the elements of the matrix: -----@ 1 2 3 4 5 6 7 8 9 ####The elements of the matrix#### 1 2 3 4 5 6 7 8 9 Sum of the diagonal elements of the matrix is here = 15
結論
在本文中,我們詳細討論了對2D數組進行排序的問題。今天我們在上述語法和演算法的基礎上使用了各種排序方法來解決這個問題。希望透過本文,您能夠對如何在Java環境中透過使用左對角線對2D數組進行排序問題有廣泛的了解。
以上是Java程式以左對角線排序2D數組的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
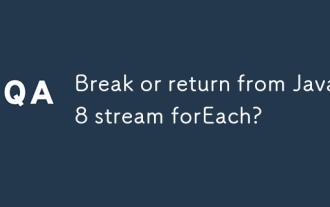
Java 8引入了Stream API,提供了一種強大且表達力豐富的處理數據集合的方式。然而,使用Stream時,一個常見問題是:如何從forEach操作中中斷或返回? 傳統循環允許提前中斷或返回,但Stream的forEach方法並不直接支持這種方式。本文將解釋原因,並探討在Stream處理系統中實現提前終止的替代方法。 延伸閱讀: Java Stream API改進 理解Stream forEach forEach方法是一個終端操作,它對Stream中的每個元素執行一個操作。它的設計意圖是處
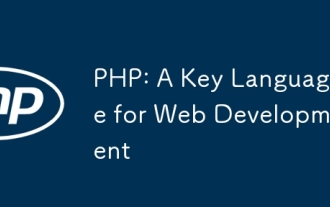
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
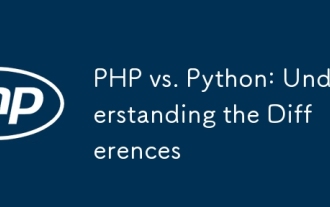
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
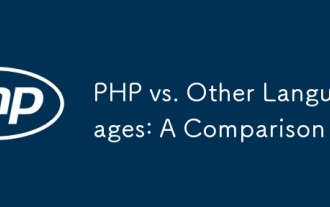
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
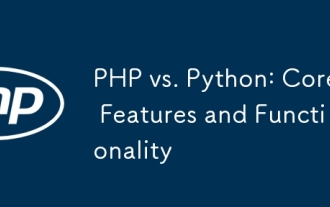
PHP和Python各有優勢,適合不同場景。 1.PHP適用於web開發,提供內置web服務器和豐富函數庫。 2.Python適合數據科學和機器學習,語法簡潔且有強大標準庫。選擇時應根據項目需求決定。
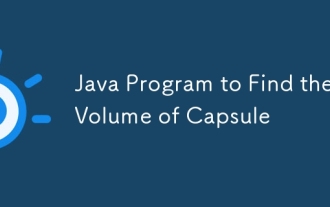
膠囊是一種三維幾何圖形,由一個圓柱體和兩端各一個半球體組成。膠囊的體積可以通過將圓柱體的體積和兩端半球體的體積相加來計算。本教程將討論如何使用不同的方法在Java中計算給定膠囊的體積。 膠囊體積公式 膠囊體積的公式如下: 膠囊體積 = 圓柱體體積 兩個半球體體積 其中, r: 半球體的半徑。 h: 圓柱體的高度(不包括半球體)。 例子 1 輸入 半徑 = 5 單位 高度 = 10 單位 輸出 體積 = 1570.8 立方單位 解釋 使用公式計算體積: 體積 = π × r2 × h (4
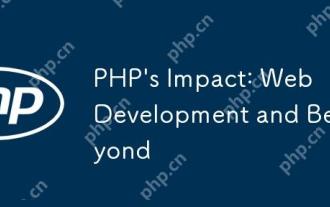
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
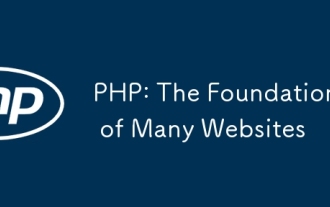
PHP成為許多網站首選技術棧的原因包括其易用性、強大社區支持和廣泛應用。 1)易於學習和使用,適合初學者。 2)擁有龐大的開發者社區,資源豐富。 3)廣泛應用於WordPress、Drupal等平台。 4)與Web服務器緊密集成,簡化開發部署。
