如何使用 JavaScript 交換 JSON 元素的鍵和值?
在這裡,我們將學習使用 JavaScript 交換 JSON 元素的鍵和值。在 JavaScript 中,物件儲存鍵值對。因此,我們可以像交換兩個普通變數一樣交換鍵和值。
在本教學中,我們將學習使用 JavaScript 交換 JSON 元素的所有鍵值的不同方法。
使用for迴圈
我們可以使用 for 迴圈來遍歷 JSON 物件的鍵。之後,我們可以使用鍵從物件存取值。因此,我們可以交換物件中的每個鍵和值。
此外,我們需要建立一個新的空對象,並在交換後儲存該對象的鍵和值。
文法
使用者可以依照以下語法使用 for 迴圈交換 JSON 元素中的鍵和值。
for (let key in object) { let value = object[key]; new_obj[value] = key; }
在上面的語法中,我們使用鍵從物件中存取其值,對於new_obj,我們使用值作為鍵,鍵作為值。
範例 1
在下面的範例中,我們建立了包含唯一鍵值對的物件。此外,我們建立了空的 new_obj 物件來儲存交換後的鍵和值。之後,我們使用 for 迴圈來迭代物件的鍵。在 for 迴圈中,我們從 JSON 元素存取特定鍵的值。
我們將物件中的鍵和值加入到 new_obj 物件中,但我們使用值作為鍵,使用鍵作為要交換的值。在輸出中,使用者可以觀察到被交換的 new_obj 的鍵值對。
<html> <body> <h2>Using the <i> for loop </i> to swap keys and values of JSON elements</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); let object = { "key1": true, "key2": 10, "key3": "hello", "key4": 40 } let new_obj = {}; for (let key in object) { let value = object[key]; new_obj[value] = key; } output.innerHTML += "The old object is " + JSON.stringify(object) + "<br/>"; output.innerHTML += "The new object after swapping the key and values is " + JSON.stringify(new_obj) + "<br/>"; </script> </body> </html>
使用 Object.keys() 和 forEach() 方法
我們可以使用Object.keys()方法以陣列格式取得物件的鍵。之後,我們可以使用 forEach() 方法來迭代鍵數組。
在遍歷所有鍵時,我們可以存取特定鍵的值並將其用作新物件的鍵。
文法
使用者可以依照下面的語法使用Object.keys()和forEach()方法來交換JSON物件的所有鍵和值。
Object.keys(student_obj).forEach((objectKey) => { swapped_obj[ student_obj[objectKey] ] = objectKey; })
在上面的語法中,我們使用「student_obj[objectKey]」從物件中取得值,它作為 swapped_obj 物件的鍵。
範例 2
在這個範例中,我們建立了student_obj對象,它包含了student的各種屬性及其值。之後,我們使用 Object.entries() 和 forEach() 方法交換物件的所有鍵和值並將其儲存到 swapped_obj 物件中。
<html> <body> <h2>Using the <i> Object.keys() and forEach() methods </i> to swap keys and values of JSON elements.</h2> <div id = "output"> </div> <script> let output = document.getElementById('output'); let student_obj = { "name": "Shubham", "age": 22, "hobby": "Writing", "Above18": true, "id": "waewr34fg7y657" } let swapped_obj = {}; Object.keys(student_obj).forEach((objectKey) => { let keyvalue = student_obj[objectKey]; swapped_obj[keyvalue] = objectKey; }) output.innerHTML += "The old object is " + JSON.stringify(student_obj) + "<br/>"; output.innerHTML += "The new object after swapping the key and values is " + JSON.stringify(swapped_obj) + "<br/>"; </script> </body> </html>
使用Object.entries()和map()方法
我們可以使用Object.entries()方法以陣列格式取得物件的所有鍵和值。交換舊的鍵值對後,我們可以使用map()方法來映射新的鍵值對。
我們可以使用陣列解構屬性來交換每個鍵和值對。
文法
使用者可以依照下面的語法使用Object.entries()和map()方法來交換JSON元素的鍵和值。
const result = Object.entries(table).map( ([prop, propValue]) => { return [propValue, prop]; } ); let newObject = Object.fromEntries(result);
我們顛倒了上述語法中每個鍵和值對的順序。因此,鍵變成了值,而值變成了鍵。此外,我們使用 Object.fromEntries() 方法從鍵和值的條目建立一個物件。
範例 3
在此範例中,我們定義了包含表格屬性的表格物件。當使用者點擊該按鈕時,它會呼叫 swapKeyValues() 函數。
在 swapKeyValues() 函數中,我們交換物件的每個鍵和值對並將它們儲存在 FinalObject 陣列中。之後,我們使用Object.fromEntries()方法將finalObject數組轉換為JSON對象,並將其儲存在newObject變數中
<html> <body> <h2>Using the <i> Object.entries() and map() methods </i> to swap keys and values of JSON elements.</h2> <div id = "output"></div> <button onclick = "swapKeyValues()"> Swap Object key values</button> <script> let output = document.getElementById('output'); let table = { "id": "1234", "color": "blue", "size": "6 feet", "legs": "6", "chairs": "8", } function swapKeyValues() { output.innerHTML += "The initial object is " + JSON.stringify(table) + "<br/>"; let objectEntries = Object.entries(table); const finalObject = objectEntries.map( ([prop, propValue]) => { return [propValue, prop]; } ); let newObject = Object.fromEntries(finalObject); output.innerHTML += "The new object after swapping the key and values is " + JSON.stringify(newObject) + "<br/>"; return; } </script> </body> </html>
我們學習了三種交換 JSON 元素的鍵和值的方法。另外,我們可以使用 array.reduce() 方法和 Object.entries() 方法來交換物件的鍵和值。
以上是如何使用 JavaScript 交換 JSON 元素的鍵和值?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
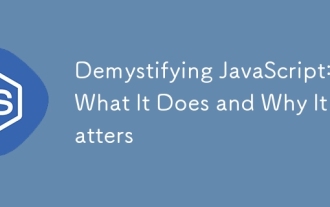
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
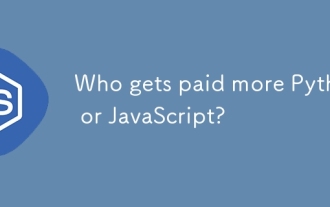
Python和JavaScript開發者的薪資沒有絕對的高低,具體取決於技能和行業需求。 1.Python在數據科學和機器學習領域可能薪資更高。 2.JavaScript在前端和全棧開發中需求大,薪資也可觀。 3.影響因素包括經驗、地理位置、公司規模和特定技能。
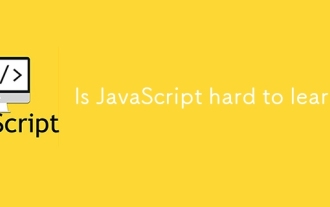
學習JavaScript不難,但有挑戰。 1)理解基礎概念如變量、數據類型、函數等。 2)掌握異步編程,通過事件循環實現。 3)使用DOM操作和Promise處理異步請求。 4)避免常見錯誤,使用調試技巧。 5)優化性能,遵循最佳實踐。
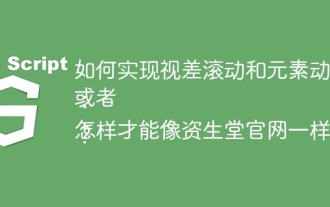
實現視差滾動和元素動畫效果的探討本文將探討如何實現類似資生堂官網(https://www.shiseido.co.jp/sb/wonderland/)中�...
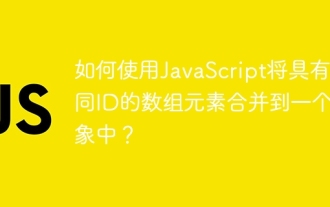
如何在JavaScript中將具有相同ID的數組元素合併到一個對像中?在處理數據時,我們常常會遇到需要將具有相同ID�...
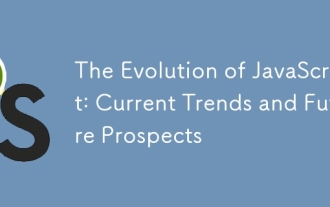
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
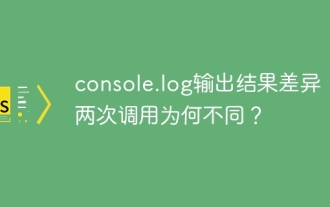
深入探討console.log輸出差異的根源本文將分析一段代碼中console.log函數輸出結果的差異,並解釋其背後的原因。 �...
