在C語言中,列印給定層級的葉節點
The task involves printing leaf nodes of a binary tree at given level k which is specified by the user.
Leaf nodes are the end nodes whose left and right pointer is NULL whichpointer that partic node that a parent node.
Example
的中文翻譯為:範例
Input : 11 22 33 66 44 88 77 Output : 88 77
在這裡,k代表需要列印的樹的層級。這裡使用的方法是遍歷每個節點,並檢查節點是否有任何指標。即使只有一個指針,表示左側或右側或兩者都有,那個特定的節點也不能是葉節點。
使用層次遍歷技術遞歸遍歷每個節點,從左側開始,然後是根節點,最後是右側。
下面的程式碼展示了給定演算法的C語言實作
演算法
START Step 1 -> create node variable of type structure Declare int data Declare pointer of type node using *left, *right Step 2 -> create function for inserting node with parameter as new_data Declare temp variable of node using malloc Set temp->data = new_data Set temp->left = temp->right = NULL return temp Step 3 -> declare Function void leaf(struct node* root, int level) IF root = NULL Exit End IF level = 1 IF root->left == NULL && root->right == NULL Print root->data End End ELSE IF level>1 Call leaf(root->left, level - 1) Call leaf(root->right, level - 1) End Step 4-> In main() Set level = 4 Call New passing value user want to insert as struct node* root = New(1) Call leaf(root,level) STOP
Example
的中文翻譯為:範例
include<stdio.h> #include<stdlib.h> //structre of a node defined struct node { struct node* left; struct node* right; int data; }; //structure to create a new node struct node* New(int data) { struct node* temp = (struct node*)malloc(sizeof(struct node)); temp->data = data; temp->left = NULL; temp->right = NULL; return temp; } //function to found leaf node void leaf(struct node* root, int level) { if (root == NULL) return; if (level == 1) { if (root->left == NULL && root->right == NULL) printf("%d</p><p>",root->data); } else if (level > 1) { leaf(root->left, level - 1); leaf(root->right, level - 1); } } int main() { printf("leaf nodes are: "); struct node* root = New(11); root->left = New(22); root->right = New(33); root->left->left = New(66); root->right->right = New(44); root->left->left->left = New(88); root->left->left->right = New(77); int level = 4; leaf(root, level); return 0; }
輸出
如果我們執行上述程序,它將產生以下輸出。
leaf nodes are: 88 77
以上是在C語言中,列印給定層級的葉節點的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
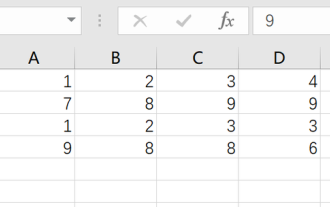
如果在開啟一份需要列印的文件時,在列印預覽裡我們會發現表格框線不知為何消失不見了,遇到這樣的情況,我們就要及時進行處理,如果你的列印文件裡也出現了此類的問題,那麼就和小編一起來學習下邊的課程吧:excel列印表格框線消失怎麼辦? 1.開啟一份需要列印的文件,如下圖所示。 2、選取所有需要的內容區域,如下圖所示。 3、按滑鼠右鍵,選擇「設定儲存格格式」選項,如下圖所示。 4、點選視窗上方的「邊框」選項,如下圖所示。 5、在左側的線條樣式中選擇細實線圖樣,如下圖所示。 6、選擇“外邊框”
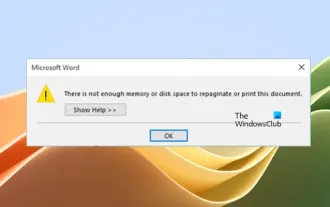
本文將介紹如何解決MicrosoftWord中出現的記憶體或磁碟空間不足以重新分頁或列印文件的問題。這種錯誤通常會在使用者嘗試列印Word文件時出現。如果您遇到類似的錯誤,請參考本文提供的建議來解決。記憶體或磁碟空間不足,無法重新分頁或列印此文件Word錯誤解決MicrosoftWord列印錯誤「沒有足夠記憶體或磁碟空間重新分頁或列印文件」的方法。更新MicrosoftOffice關閉佔用記憶體的應用程式更改您的預設印表機在安全模式下啟動Word重命名NorMal.dotm檔案將Word檔案儲存為另一
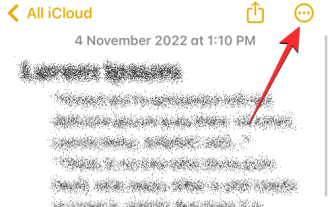
在這個數位化的世界中,列印頁面的需求並沒有消失。儘管您可能認為在電腦上保存內容並直接發送到印表機更為便捷,但是您同樣可以在iPhone上完成相同的操作。透過iPhone的相機,您可以拍攝照片或文檔,並且還可以直接儲存文件以便隨時列印。這樣一來,您可以快速方便地將您所需的資訊實體化,並將其保存在紙本文件中。無論是在工作還是日常生活中,iPhone為您提供了一個便攜式的列印解決方案。以下貼文將幫助您了解如果您希望使用iPhone在印表機上列印頁面,您需要了解的所有資訊。從iPhone列印:要求蘋
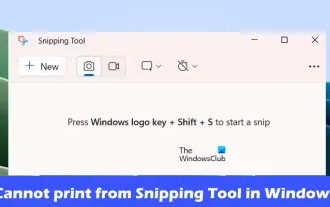
如果您在Windows11/10中無法使用截圖工具進行列印,可能是由於系統檔案損壞或驅動程式問題導致的。本文將為您提供解決此問題的方法。在Windows11/10中無法從截圖工具列印如果您無法從Windows11/10中的SnippingTool列印,請使用這些修復程式:重新啟動PC印表機清除列印佇列更新印表機和顯示卡驅動程式修復或重設剪裁工具執行SFC和DISM掃描使用PowerShell指令卸載並重新安裝截圖工具。我們開始吧。 1]重新啟動您的PC和印表機重新啟動電腦和印表機有助於消除暫時的故障
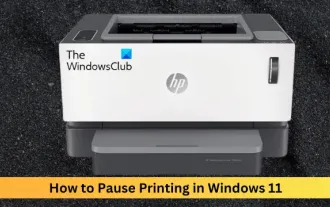
錯誤地列印了一個大文件?需要停止或暫停列印以節省墨水和紙張嗎?在許多情況下,您可能需要暫停Windows11裝置上正在進行的列印作業。如何在Windows11中暫停列印?在Windows11中,暫停列印會暫停列印作業,但並不會取消列印任務。這為用戶提供了更靈活的控制權。有三種方法可以實現這一點:使用工作列暫停列印使用Windows設定暫停列印使用控制面板列印現在,讓我們來詳細看看這些。 1]使用工作列列印右鍵點選工作列上的列印佇列通知。按一下開啟所有活動印表機選項。在這裡,右鍵列印作業並選擇全部暫停
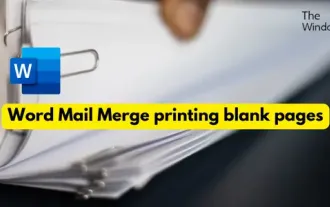
如果您發現在使用Word列印郵件合併文件時出現空白頁,這篇文章將對您有所幫助。郵件合併是一項便捷的功能,讓您能夠輕鬆建立個人化文件並傳送給多個收件者。在MicrosoftWord中,郵件合併功能備受推崇,因為它能夠幫助使用者節省手動為每個收件者複製相同內容的時間。為了列印郵件合併文檔,您可以轉到郵件標籤。但有些Word使用者反映,在嘗試列印郵件合併文件時,印表機會列印空白頁或完全不列印。這可能是由於格式設定不正確或印表機設定問題。嘗試檢查文檔和印表機設置,確保列印前預覽文檔,以確保內容正確。如果
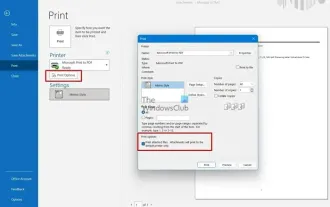
Outlook是功能最豐富的電子郵件用戶端之一,已成為專業交流不可或缺的工具。其中一個挑戰是在Outlook中同時列印所有附件。通常需要逐個下載附件才能列印,但如果想一次列印所有內容,這就是大多數人遇到的問題。如何列印Outlook中的所有附件儘管大部分資訊是在Outlook應用程式中線上維護的,但有時需要將資訊列印出來備份。必須親自簽署文件,以滿足合約、政府表格或家庭作業等法律要求。有幾種方法可以讓您一次點擊列印Outlook中的所有附件,而不是逐一列印。讓我們詳細地看看每一個。 Outloo
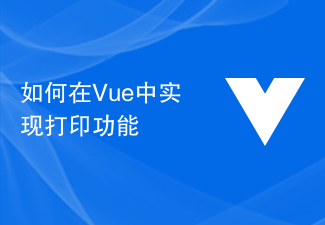
如何在Vue中實現列印功能,需要具體程式碼範例Vue.js是一種用於建立使用者介面的漸進式JavaScript框架。在許多Web應用程式中,列印功能是非常重要的一部分。本文將介紹如何在Vue中實現列印功能,並提供具體的程式碼範例。在Vue中實現列印功能,首先要明確列印的內容是什麼。通常,我們會將要列印的內容放在一個HTML元素中,例如一個div。然後,透過Jav
