重新排列一個數組,以使連續一對元素的乘積總和最小,並使用C++編寫
我們有一個正整數型別的數組,假設是arr[],大小任意。任務是重新排列數組,使得當我們將一個元素與其相鄰元素相乘,然後將所有結果元素相加時,會傳回最小的和。
讓我們來看看不同的輸入輸出狀況:
輸入 - int arr[] = {2, 5, 1, 7, 5, 0, 1, 0}
輸出 - 重新排列陣列以最小化和,即連續一對元素的乘積為:7 0 5 0 5 1 2 1
#解釋 - 我們有一個大小為8的整數陣列。現在,我們將重新排列數組,即7 0 5 0 5 1 2 1。我們將檢查是否回傳最小和,即7 * 0 5 * 0 5 * 1 2 * 1 = 0 0 5 2 = 7。
輸入 - int arr[] = {1, 3, 7, 2, 4, 3}
輸出 - 重新排列陣列以最小化和,即連續一對元素的乘積為:7 1 4 2 3 3
#解釋 - 我們有一個大小為6的整數陣列。現在,我們將重新排列數組,即7 1 4 2 3 3。我們將檢查是否回傳最小和,即7 * 1 4 * 2 3 * 3 = 7 8 9 = 24。
下面程式中使用的方法如下:
輸入一個整數類型的陣列並計算陣列的大小。
使用C STL的sort方法對陣列進行排序,將陣列和陣列的大小傳遞給sort函數。
宣告一個整數變量,並將其設定為呼叫函數的回傳值。
Inside the function Rearrange_min_sum(arr, size)
Create a variable, let's say, 'even' and 'odd' type of type vector which stores integer variables.
#Declare a variable as temp and total and initialise it with 0.
#StartStart loop FOR from i to 0 till i less than size. Inside the loop, check IF i is less than size/2 then push arr[i] to odd vector ELSE, push arr[i] to even vector
#Call the sort method by passing even.begin(), even.end() and greater
(). -
Start loop FOR from i to 0 till i less than even.size(). Inside the loop, set arr[temp ] to even[j], arr[temp ] to odd[j] and total to total even[j] * odd[j]
Return total
#Print the result.
Example
#include <bits/stdc++.h> using namespace std; int Rearrange_min_sum(int arr[], int size){ vector<int> even, odd; int temp = 0; int total = 0; for(int i = 0; i < size; i++){ if (i < size/2){ odd.push_back(arr[i]); } else{ even.push_back(arr[i]); } } sort(even.begin(), even.end(), greater<int>()); for(int j = 0; j < even.size(); j++){ arr[temp++] = even[j]; arr[temp++] = odd[j]; total += even[j] * odd[j]; } return total; } int main(){ int arr[] = { 2, 5, 1, 7, 5, 0, 1, 0}; int size = sizeof(arr)/sizeof(arr[0]); //sort an array sort(arr, arr + size); //call function int total = Rearrange_min_sum(arr, size); cout<<"Rearrangement of an array to minimize sum i.e. "<<total<<" of product of consecutive pair elements is: "; for(int i = 0; i < size; i++){ cout << arr[i] << " "; } return 0; }
輸出
如果我們執行上面的程式碼,它將產生以下輸出
Rearrangement of an array to minimize sum i.e. 7 of product of consecutive pair elements is: 7 0 5 0 5 1 2 1
以上是重新排列一個數組,以使連續一對元素的乘積總和最小,並使用C++編寫的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

熱門話題
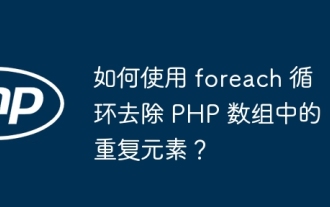
使用foreach循環移除PHP數組中重複元素的方法如下:遍歷數組,若元素已存在且當前位置不是第一個出現的位置,則刪除它。舉例而言,若資料庫查詢結果有重複記錄,可使用此方法移除,得到不含重複記錄的結果。
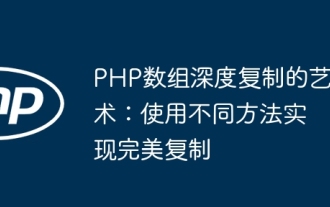
PHP中深度複製數組的方法包括:使用json_decode和json_encode進行JSON編碼和解碼。使用array_map和clone進行深度複製鍵和值的副本。使用serialize和unserialize進行序列化和反序列化。

PHP數組鍵值翻轉方法效能比較顯示:array_flip()函數在大型數組(超過100萬個元素)下比for迴圈效能更優,耗時更短。手動翻轉鍵值的for迴圈方法耗時相對較長。
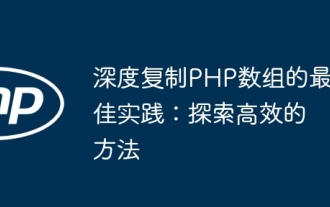
在PHP中執行陣列深度複製的最佳實踐是:使用json_decode(json_encode($arr))將陣列轉換為JSON字串,然後再轉換回陣列。使用unserialize(serialize($arr))將陣列序列化為字串,然後將其反序列化為新陣列。使用RecursiveIteratorIterator迭代器對多維數組進行遞歸遍歷。
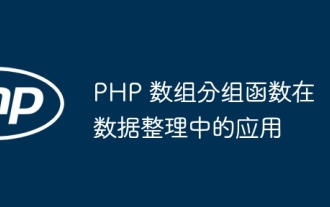
PHP的array_group_by函數可依鍵或閉包函數將陣列中的元素分組,傳回關聯數組,其中鍵為組名,值是屬於該組的元素數組。
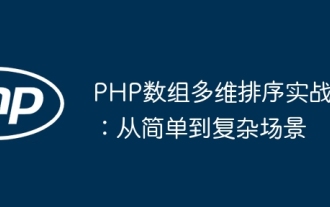
多維數組排序可分為單列排序和嵌套排序。單列排序可使用array_multisort()函數依列排序;巢狀排序需要遞歸函數遍歷陣列並排序。實戰案例包括按產品名稱排序和按銷售量和價格複合排序。
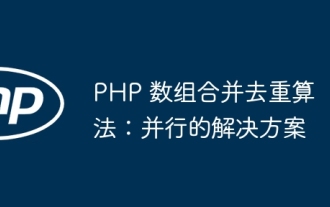
PHP數組合併去重演算法提供了平行的解決方案,將原始陣列分成小塊並行處理,主進程合併區塊的結果去重。演算法步驟:分割原始數組為均等分配的小塊。並行處理每個區塊去重。合併區塊結果並再次去重。
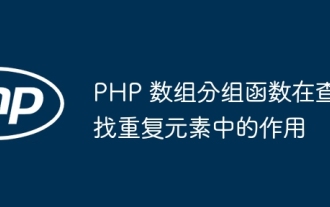
PHP的array_group()函數可用來按指定鍵對陣列進行分組,以尋找重複元素。函數透過以下步驟運作:使用key_callback指定分組鍵。可選地使用value_callback確定分組值。對分組元素進行計數並識別重複項。因此,array_group()函數對於尋找和處理重複元素非常有用。
