Java 與 C#
Java is a dynamic, secured and class based high level object oriented programming language developed by Oracle Corporation. On the other hand; C# is a .Net Framework object ori Corporation. On the other hand; C# is a 。 .
Java和C#都是通用的程式設計範式,或基本上被稱為命令式程式設計環境。而且這兩種語言都能夠提供一些高階結果作為輸出。
In a broad view there are many differences between these two OOPs −
Java Runtime Environment旨在執行Java程式碼,而C#運行在CLR環境(公共語言執行時期)。
Java和C#都是物件導向的程式語言。但是在特定的方式上,C#是一種功能和元件導向的強型別編碼語言。這種語言提供了多重重載的特性,而這是Java所沒有的。
這兩者的Array特性也不相同。對Java來說,Object是直接的專門化,而對C#來說,Array是系統的專門化。
Use of C# -
Web app. Development
#Windows application development.
#Gaming application.
使用Java -
Web項目,大數據應用程式
Server-side programming
Embedded systems
Android應用程式
#Java和C#語言的工作原理:
Java
In a software designing environment, it is important to have a runtime platform. The runtime platform provides the access to main memory and other important features of a system to run a code.
Java執行環境(Java Runtime Environment)是一種基本的後端技術,它在Java建置程式碼和作業系統之間建立並建立通訊管道。簡單來說,JRE是一個執行時間工具,它提供了編寫Java程式碼並執行以獲得所需結果的所有資源。
There are two Java components −
JDK – Java開發工具包
JVM – Java Virtual Machine
A collection of software development tools to develop an application using Java. You can get many JDK version in matching with its Java version. Like, Java SE needs JDK Java SE.
JVM逐行執行Java程式碼。當Java應用程式運行時,開發人員配置設定。它還透過使用運行時檢查正在運行的Java應用程式的內部儲存。
C
#的中文翻譯為:C
#Basically, the .NET build codes compile into a Microsoft Intermediate Language aka MSIL by using Just in Time (JIT) compiler. Clearly, the output will be a machine code (written by a set of class libraries and be a machine code (written by a set of class libraries) and class libraries) and class libraries beit it. produced by a machine processor.
The compiler and the CLR format the C# code to an executable code. Here we get an understanding complex machine environment in .NET. The executable codes can be saved as .exe and 。
A Java Code Algorithm:-的中文翻譯為:
一個Java程式碼演算法:- 第一步 - 在IDE中編寫原始程式碼。
- Step 2 − Put It Into The Compiler.
- 步驟3 - 將其轉換為字節碼。
- 步驟 4 − JVM(Windows,MacOS,Linux)。
- Step 5 − Converted Machine Code.
- #步驟 6 − 終止進程
- Step 1 − Start.
- Step 2 − Select The Documents.
- #第三步 - 指令部分。
- Step 4 − Select Interface.
- #第五步 - 選擇課程。
- 第6步 - Main()方法宣告。
- 步驟 7 - 沒有頭文件,匯入 .dll 檔案。
- 第8步 - 輸入反射
public class Main {
public static void main (String[] args) {
System.out.println ("THE STATEMENT");
}
}
登入後複製
Syntaxpublic class Main { public static void main (String[] args) { System.out.println ("THE STATEMENT"); } }
using System;
namespace Tutorialspoint {
class Program{
static void Main(string[] args){
Console.WriteLine("Hello Student");
}
}
}
登入後複製
The class using system declares the system namespace. The namespace organises the code as a container. Every line of the written code that runs, must be stay inside a class in C# language.using System; namespace Tutorialspoint { class Program{ static void Main(string[] args){ Console.WriteLine("Hello Student"); } } }
Approach
- Approach 1: A General Example of a Java Code.
- 方法二:C#程式碼的通用範例。
Example 1
public class TableofMultiplication {
public static void main(String[] args) {
int num = 18, j = 1;
while(j <= 20){
System.out.printf("%d * %d = %d \n", num, j, num * j);j++;
}
}
}
登入後複製
輸出public class TableofMultiplication { public static void main(String[] args) { int num = 18, j = 1; while(j <= 20){ System.out.printf("%d * %d = %d \n", num, j, num * j);j++; } } }
18 * 2 = 36
18 * 3 = 54
18 * 4 = 72
18 * 5 = 90
18 * 6 = 108
18 * 7 = 126
18 * 8 = 144
18 * 9 = 162
18 * 10 = 180
18 * 11 = 198
18 * 12 = 216
18 * 13 = 234
18 * 14 = 252
18 * 15 = 270
18 * 16 = 288
18 * 17 = 306
18 * 18 = 324
18 * 19 = 342
18 * 20 = 360
登入後複製
A General Example of A C# Code#Example 118 * 2 = 36 18 * 3 = 54 18 * 4 = 72 18 * 5 = 90 18 * 6 = 108 18 * 7 = 126 18 * 8 = 144 18 * 9 = 162 18 * 10 = 180 18 * 11 = 198 18 * 12 = 216 18 * 13 = 234 18 * 14 = 252 18 * 15 = 270 18 * 16 = 288 18 * 17 = 306 18 * 18 = 324 18 * 19 = 342 18 * 20 = 360
using System;
public class Exercise6 {
public static void Main() {
int i,n;
Console.Write("\n\n");
Console.Write("Display the multiplication table of the number:\n");
Console.Write("-----------------------------------");
Console.Write("\n\n");
Console.Write("Input the number you need to do multiplication: ");
n= Convert.ToInt32(Console.ReadLine());
Console.Write("\n");
for(i=1;i<=100;i++){
Console.Write("{0} X {1} = {2} \n",n,i,n*i);
}
}
}
登入後複製
輸出using System; public class Exercise6 { public static void Main() { int i,n; Console.Write("\n\n"); Console.Write("Display the multiplication table of the number:\n"); Console.Write("-----------------------------------"); Console.Write("\n\n"); Console.Write("Input the number you need to do multiplication: "); n= Convert.ToInt32(Console.ReadLine()); Console.Write("\n"); for(i=1;i<=100;i++){ Console.Write("{0} X {1} = {2} \n",n,i,n*i); } } }
Display the multiplication table of the number:
-----------------------------------
Input the number you need to do multiplication:
0 X 1 = 0
0 X 2 = 0
0 X 3 = 0
0 X 4 = 0
0 X 5 = 0
0 X 6 = 0
0 X 7 = 0
0 X 8 = 0
0 X 9 = 0
0 X 10 = 0
0 X 11 = 0
0 X 12 = 0
0 X 13 = 0
0 X 14 = 0
0 X 15 = 0
0 X 16 = 0
0 X 17 = 0
0 X 18 = 0
0 X 19 = 0
0 X 20 = 0
0 X 21 = 0
0 X 22 = 0
0 X 23 = 0
0 X 24 = 0
0 X 25 = 0
0 X 26 = 0
0 X 27 = 0
0 X 28 = 0
0 X 29 = 0
0 X 30 = 0
0 X 31 = 0
0 X 32 = 0
0 X 33 = 0
0 X 34 = 0
0 X 35 = 0
0 X 36 = 0
0 X 37 = 0
0 X 38 = 0
0 X 39 = 0
0 X 40 = 0
0 X 41 = 0
0 X 42 = 0
0 X 43 = 0
0 X 44 = 0
0 X 45 = 0
0 X 46 = 0
0 X 47 = 0
0 X 48 = 0
0 X 49 = 0
0 X 50 = 0
0 X 51 = 0
0 X 52 = 0
0 X 53 = 0
0 X 54 = 0
0 X 55 = 0
0 X 56 = 0
0 X 57 = 0
0 X 58 = 0
0 X 59 = 0
0 X 60 = 0
0 X 61 = 0
0 X 62 = 0
0 X 63 = 0
0 X 64 = 0
0 X 65 = 0
0 X 66 = 0
0 X 67 = 0
0 X 68 = 0
0 X 69 = 0
0 X 70 = 0
0 X 71 = 0
0 X 72 = 0
0 X 73 = 0
0 X 74 = 0
0 X 75 = 0
0 X 76 = 0
0 X 77 = 0
0 X 78 = 0
0 X 79 = 0
0 X 80 = 0
0 X 81 = 0
0 X 82 = 0
0 X 83 = 0
0 X 84 = 0
0 X 85 = 0
0 X 86 = 0
0 X 87 = 0
0 X 88 = 0
0 X 89 = 0
0 X 90 = 0
0 X 91 = 0
0 X 92 = 0
0 X 93 = 0
0 X 94 = 0
0 X 95 = 0
0 X 96 = 0
0 X 97 = 0
0 X 98 = 0
0 X 99 = 0
0 X 100 = 0
登入後複製
ConclusionDisplay the multiplication table of the number: ----------------------------------- Input the number you need to do multiplication: 0 X 1 = 0 0 X 2 = 0 0 X 3 = 0 0 X 4 = 0 0 X 5 = 0 0 X 6 = 0 0 X 7 = 0 0 X 8 = 0 0 X 9 = 0 0 X 10 = 0 0 X 11 = 0 0 X 12 = 0 0 X 13 = 0 0 X 14 = 0 0 X 15 = 0 0 X 16 = 0 0 X 17 = 0 0 X 18 = 0 0 X 19 = 0 0 X 20 = 0 0 X 21 = 0 0 X 22 = 0 0 X 23 = 0 0 X 24 = 0 0 X 25 = 0 0 X 26 = 0 0 X 27 = 0 0 X 28 = 0 0 X 29 = 0 0 X 30 = 0 0 X 31 = 0 0 X 32 = 0 0 X 33 = 0 0 X 34 = 0 0 X 35 = 0 0 X 36 = 0 0 X 37 = 0 0 X 38 = 0 0 X 39 = 0 0 X 40 = 0 0 X 41 = 0 0 X 42 = 0 0 X 43 = 0 0 X 44 = 0 0 X 45 = 0 0 X 46 = 0 0 X 47 = 0 0 X 48 = 0 0 X 49 = 0 0 X 50 = 0 0 X 51 = 0 0 X 52 = 0 0 X 53 = 0 0 X 54 = 0 0 X 55 = 0 0 X 56 = 0 0 X 57 = 0 0 X 58 = 0 0 X 59 = 0 0 X 60 = 0 0 X 61 = 0 0 X 62 = 0 0 X 63 = 0 0 X 64 = 0 0 X 65 = 0 0 X 66 = 0 0 X 67 = 0 0 X 68 = 0 0 X 69 = 0 0 X 70 = 0 0 X 71 = 0 0 X 72 = 0 0 X 73 = 0 0 X 74 = 0 0 X 75 = 0 0 X 76 = 0 0 X 77 = 0 0 X 78 = 0 0 X 79 = 0 0 X 80 = 0 0 X 81 = 0 0 X 82 = 0 0 X 83 = 0 0 X 84 = 0 0 X 85 = 0 0 X 86 = 0 0 X 87 = 0 0 X 88 = 0 0 X 89 = 0 0 X 90 = 0 0 X 91 = 0 0 X 92 = 0 0 X 93 = 0 0 X 94 = 0 0 X 95 = 0 0 X 96 = 0 0 X 97 = 0 0 X 98 = 0 0 X 99 = 0 0 X 100 = 0
在本文中,我們將Java與C#進行了詳細比較。最好找到適合您專案的語言。在這裡,我們透過使用不同的演算法和建立程式碼來更好地理解這兩種語言。
以上是Java 與 C#的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
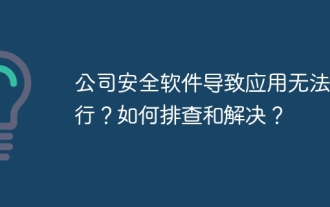
公司安全軟件導致部分應用無法正常運行的排查與解決方法許多公司為了保障內部網絡安全,會部署安全軟件。 ...
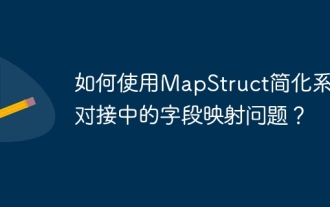
系統對接中的字段映射處理在進行系統對接時,常常會遇到一個棘手的問題:如何將A系統的接口字段有效地映�...
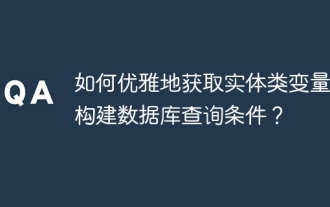
在使用MyBatis-Plus或其他ORM框架進行數據庫操作時,經常需要根據實體類的屬性名構造查詢條件。如果每次都手動...
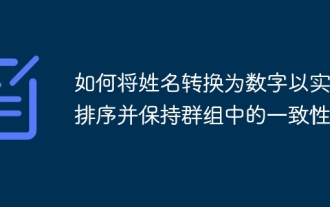
將姓名轉換為數字以實現排序的解決方案在許多應用場景中,用戶可能需要在群組中進行排序,尤其是在一個用...
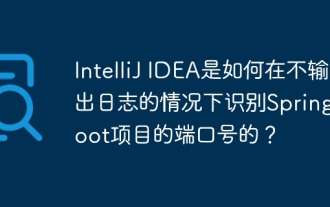
在使用IntelliJIDEAUltimate版本啟動Spring...
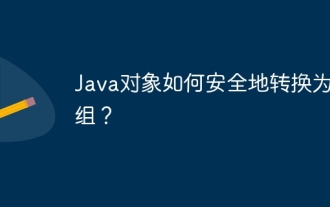
Java對象與數組的轉換:深入探討強制類型轉換的風險與正確方法很多Java初學者會遇到將一個對象轉換成數組的�...
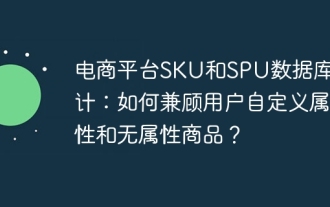
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
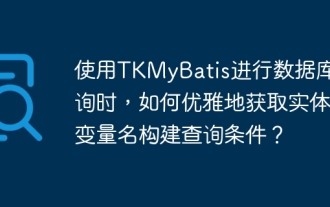
在使用TKMyBatis進行數據庫查詢時,如何優雅地獲取實體類變量名以構建查詢條件,是一個常見的難題。本文將針...
