如何透過PHP物件導向簡單工廠模式實現物件的封裝與隱藏
如何透過PHP物件導向簡單工廠模式實現物件的封裝與隱藏
簡介:
在PHP物件導向程式設計中,封裝是一種實作數據隱藏的重要概念。封裝可以將資料和相關的操作封裝在一個類別中,並透過對外暴露的公共介面來操作資料。而簡單工廠模式則是一種常用的創建物件的設計模式,它將物件的創建與使用分離,使得系統更加靈活,易於擴展。本文將結合範例程式碼,介紹如何透過PHP物件導向簡單工廠模式來實現物件的封裝和隱藏。
實作步驟:
- 建立一個抽象類別或接口,用於定義物件的公共接口。
- 建立具體的類,實作抽象類別或接口,並進行資料封裝和隱藏。
- 建立一個簡單工廠類,在其中根據條件來建立具體的類別的實例。
程式碼範例:
- 建立抽象類別或介面。
<?php abstract class Shape { protected $color; abstract public function draw(); } ?>
- 建立具體類,並進行資料封裝和隱藏。
<?php class Circle extends Shape { private $radius; public function __construct($radius, $color) { $this->radius = $radius; $this->color = $color; } public function draw() { echo "Drawing a ".$this->color." circle with radius ".$this->radius.".<br>"; } } class Square extends Shape { private $length; public function __construct($length, $color) { $this->length = $length; $this->color = $color; } public function draw() { echo "Drawing a ".$this->color." square with length ".$this->length.".<br>"; } } ?>
- 建立簡單工廠類別。
<?php class ShapeFactory { public static function create($type, $params) { switch ($type) { case 'circle': return new Circle($params['radius'], $params['color']); case 'square': return new Square($params['length'], $params['color']); default: throw new Exception('Invalid shape type.'); } } } ?>
使用範例:
<?php $params = [ 'radius' => 5, 'color' => 'red' ]; $circle = ShapeFactory::create('circle', $params); $circle->draw(); $params = [ 'length' => 10, 'color' => 'blue' ]; $square = ShapeFactory::create('square', $params); $square->draw(); ?>
透過上述程式碼範例,我們可以看到透過PHP物件導向簡單工廠模式的方式,能夠實現物件的封裝和隱藏。具體類別中的屬性被封裝為私有屬性,只能透過建構方法來指定,並透過抽象類別或介面的公共方法進行存取。而簡單工廠類別則負責根據條件來建立特定類別的實例,將物件的建立與使用分離,實現了物件的隱藏。
結論:
透過PHP物件導向簡單工廠模式,我們可以輕鬆實現物件的封裝與隱藏。這種方式能夠提高程式碼的可維護性和可擴展性,使系統更加靈活。在實際開發中,可以根據具體需求來選擇合適的設計模式,以提高程式碼的品質和效率。
以上是如何透過PHP物件導向簡單工廠模式實現物件的封裝與隱藏的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
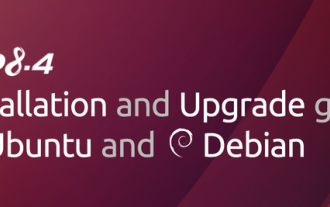
PHP 8.4 帶來了多項新功能、安全性改進和效能改進,同時棄用和刪除了大量功能。 本指南介紹如何在 Ubuntu、Debian 或其衍生版本上安裝 PHP 8.4 或升級到 PHP 8.4
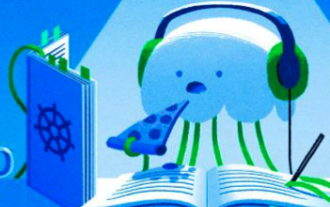
Visual Studio Code,也稱為 VS Code,是一個免費的原始碼編輯器 - 或整合開發環境 (IDE) - 可用於所有主要作業系統。 VS Code 擁有大量針對多種程式語言的擴展,可以輕鬆編寫
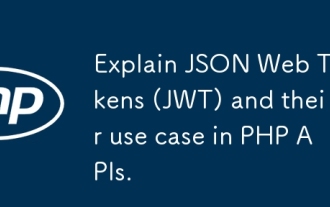
JWT是一種基於JSON的開放標準,用於在各方之間安全地傳輸信息,主要用於身份驗證和信息交換。 1.JWT由Header、Payload和Signature三部分組成。 2.JWT的工作原理包括生成JWT、驗證JWT和解析Payload三個步驟。 3.在PHP中使用JWT進行身份驗證時,可以生成和驗證JWT,並在高級用法中包含用戶角色和權限信息。 4.常見錯誤包括簽名驗證失敗、令牌過期和Payload過大,調試技巧包括使用調試工具和日誌記錄。 5.性能優化和最佳實踐包括使用合適的簽名算法、合理設置有效期、
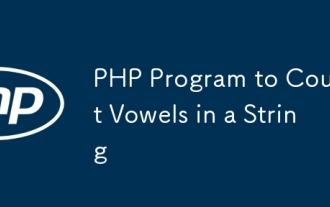
字符串是由字符組成的序列,包括字母、數字和符號。本教程將學習如何使用不同的方法在PHP中計算給定字符串中元音的數量。英語中的元音是a、e、i、o、u,它們可以是大寫或小寫。 什麼是元音? 元音是代表特定語音的字母字符。英語中共有五個元音,包括大寫和小寫: a, e, i, o, u 示例 1 輸入:字符串 = "Tutorialspoint" 輸出:6 解釋 字符串 "Tutorialspoint" 中的元音是 u、o、i、a、o、i。總共有 6 個元
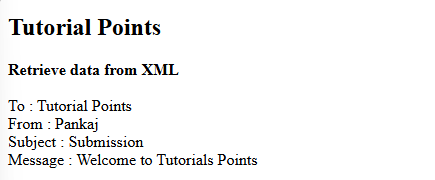
本教程演示瞭如何使用PHP有效地處理XML文檔。 XML(可擴展的標記語言)是一種用於人類可讀性和機器解析的多功能文本標記語言。它通常用於數據存儲
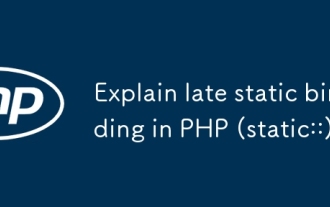
靜態綁定(static::)在PHP中實現晚期靜態綁定(LSB),允許在靜態上下文中引用調用類而非定義類。 1)解析過程在運行時進行,2)在繼承關係中向上查找調用類,3)可能帶來性能開銷。

PHP的魔法方法有哪些? PHP的魔法方法包括:1.\_\_construct,用於初始化對象;2.\_\_destruct,用於清理資源;3.\_\_call,處理不存在的方法調用;4.\_\_get,實現動態屬性訪問;5.\_\_set,實現動態屬性設置。這些方法在特定情況下自動調用,提升代碼的靈活性和效率。
