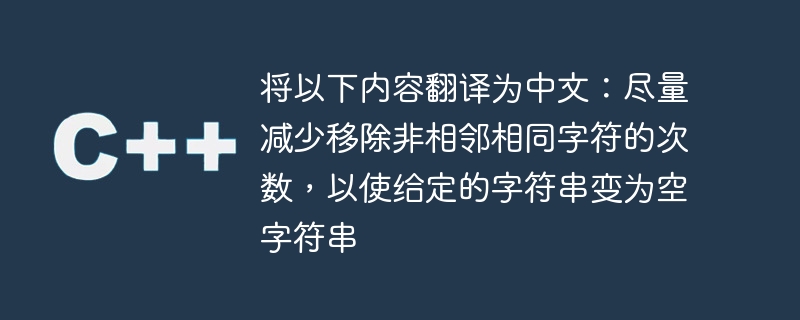
在本文中,我們將深入探討C 中一個引人入勝的字串操作問題。問題陳述是“最小化刪除非相鄰字元以使給定字串為空”。這個問題是提升你對字串、字元刪除和演算法思維的理解的絕佳方式。
Problem Statement
Given a string, the task is to minimize the number of removal operations of non-equal adjacent characters required to make the given string empty. In one operation, you can remove any two adjacent characters that areare 是#nnot.
C Solution Approach
解決這個問題的方法是使用堆疊資料結構。我們遍歷字串的字符,對於每個字符,如果堆疊不為空且堆疊的頂部字符不等於當前字符,則從堆疊中彈出頂部字符。否則,將當前字元推入堆疊。所需的操作次數是最後堆疊中剩餘字元的數量。
Example
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int minimizeRemovals(string str) {
stack<char> s;
for (char c : str) {
if (!s.empty() && s.top() != c) {
s.pop();
} else {
s.push(c);
}
}
return s.size();
}
int main() {
string str = "abba";
int operations = minimizeRemovals(str);
cout << "The minimum number of removal operations is: " << operations << endl;
return 0;
}
登入後複製
Output
#
The minimum number of removal operations is: 0
登入後複製
Explanation with a Test Case
當我們將這個字串傳遞給minimizeRemovals函數時,它會迭代字串的字元。過程如下圖所示−
-
- 它將 'a' 推入堆疊。
- 然後它將 'b' 推入堆疊,因為 'b' 不等於堆疊頂部的元素 ('a')。
- When the next 'b' is encountered, it sees that the top of the stack is also 'b', so it doesn't perform a remove operation, and 'b' is pushed onto the stack.
- Now the top of the stack is 'b', and the next character is 'a'. Since 'a' is not equal to 'b', it performs a remove operation by popping the top of the stack . Now the top of the stack is 'b'.
- 最後,在字串中遇到了與棧頂元素('b')不相等的字元'a'。因此,它執行了一個移除操作,彈出了棧頂元素。
At the end of the function, there are no characters left in the stack, indicating that all non-equal adjacent characters have been removed from the string. Hence, the function returns 0, which is the minved from the string. Hence, the function returns 0, which is the minimum number of removal to make the given string empty.
Conclusion
這個問題為使用堆疊資料結構進行字串操作提供了絕佳的機會。這是一個很好的問題,可以練習你的C 編碼技巧,並理解如何使用堆疊解決問題。
以上是將以下內容翻譯為中文:盡量減少移除非相鄰相同字元的次數,以使給定的字串變為空字串的詳細內容。更多資訊請關注PHP中文網其他相關文章!