使用 Spring Cloud 釋放微服務的力量
微服務架構的興起改變了開發人員建置和部署應用程式的方式。 Spring Cloud是 Spring 生態系統的一部分,旨在簡化開發和管理微服務的複雜性。 在本綜合指南中,我們將探索 Spring Cloud 及其功能,並透過建立簡單的微服務應用程式來展示其功能。
什麼是Spring Cloud?
Spring Cloud 是一組工具和函式庫,為分散式系統中的常見模式和挑戰提供解決方案,例如組態管理、服務發現、斷路器和分散式跟踪。 它是基於 Spring Boot 構建,可以輕鬆創建可擴展、容錯的微服務。
#Spring Cloud 的主要特性
- 設定管理: Spring Cloud Config為分散式應用程式提供集中設定管理。
- 服務發現: Spring Cloud Netflix Eureka 支援服務註冊和發現,以實現更好的負載平衡和容錯能力。
- 斷路器: Spring Cloud Netflix Hystrix 透過隔離服務之間的存取點來協助防止連鎖故障。
- 分散式追蹤: Spring Cloud Sleuth 和 Zipkin 支援跨多個服務追蹤請求,以實現更好的可觀察性和除錯。
使用Spring Cloud 建立簡單的微服務應用程式
在此範例中,我們將建立一個簡單的微服務應用程序,其中包含兩個服務: auser-service
和an order-service
。 我們也將使用 Spring Cloud Config 和 Eureka 進行集中配置和服務發現。
先決條件
請確保您的電腦上安裝了以下軟體:
- Java 8 或更高版本
- Maven 或Gradle
- 您選擇的IDE
依賴關係
#
<!-- maven --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-config-server</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-config</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
或
//Gradle implementation 'org.springframework.cloud:spring-cloud-config-server' implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-client' implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-server' implementation 'org.springframework.cloud:spring-cloud-starter-config' implementation 'org.springframework.cloud:spring-cloud-starter-netflix-eureka-client' implementation 'org.springframework.boot:spring-boot-starter-web'
#第1步:設定Spring Cloud配置伺服器
使用Spring Initializr (https://start.spring.io/) 建立一個新的Spring Boot 專案並新增Config Server
和Eureka Discovery
依賴項。 為專案命名config-server
。
將下列屬性新增至您的application.yml
檔案:
##
server: port: 8888 spring: application: name: config-server cloud: config: server: git: uri: https://github.com/your-username/config-repo.git # Replace with your Git repository URL eureka: client: serviceUrl: defaultZone: http://localhost:8761/eureka/
通过将以下注释添加到您的主类中来启用 和Config Server
:Eureka Client
import org.springframework.cloud.config.server.EnableConfigServer; import org.springframework.cloud.netflix.eureka.EnableEurekaClient; @EnableConfigServer @EnableEurekaClient @SpringBootApplication public class ConfigServerApplication { public static void main(String[] args) { SpringApplication.run(ConfigServerApplication.class, args); }
第2步:设置Spring Cloud Eureka服务器
使用Spring Initializr创建一个新的 Spring Boot 项目并添加Eureka Server
依赖项。为项目命名eureka-server
。
将以下属性添加到您的application.yml
文件中:
server: port: 8761 spring: application: name: eureka-server eureka: client: registerWithEureka: false fetchRegistry: false
通过将以下注释添加到主类来启用 Eureka Server:
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer; @EnableEurekaServer @SpringBootApplication public class EurekaServerApplication { public static void main(String[] args) { SpringApplication.run(EurekaServerApplication.class, args); } }
第三步:创建用户服务
使用 Spring Initializr 创建一个新的 Spring Boot 项目并添加Config Client
、Eureka Discovery
和Web
依赖项。为项目命名user-service
。
将以下属性添加到您的bootstrap.yml
文件中:
spring: application: name: user-service cloud: config: uri: http://localhost:8888 eureka: client: serviceUrl: defaultZone: http://localhost:8761/eureka/
创建一个简单REST controller
的User Service
:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { @GetMapping("/users/{id}") public String getUser(@PathVariable("id") String id) { return "User with ID: " + id; } }
第四步:创建订单服务
使用 Spring Initializr 创建一个新的 Spring Boot 项目并添加Config Client
、Eureka Discovery
和Web
依赖项。为项目命名order-service
。
将以下属性添加到您的bootstrap.yml
文件中:
spring: application: name: order-service cloud: config: uri: http://localhost:8888 eureka: client: serviceUrl: defaultZone: http://localhost:8761/eureka/
创建一个简单REST controller
的Order Service
:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; @RestController public class OrderController { @GetMapping("/orders/{id}") public String getOrder(@PathVariable("id") String id) { return "Order with ID: " + id; } }
第 5 步:运行应用程序
按以下顺序启动config-server
、eureka-server
、user-service
和应用程序。order-service
所有服务运行后,您可以访问 Eureka 仪表板http://localhost:8761
并查看已注册的服务。
您现在可以访问用户服务http://localhost:<user-service-port>/users/1
和订单服务http://localhost:<order-service-port>/orders/1
。
结论
在本综合指南中,我们探索了 Spring Cloud 及其功能,并通过构建简单的微服务应用程序展示了其功能。通过利用 Spring Cloud 的强大功能,您可以简化微服务的开发和管理,使它们更具弹性、可扩展性并且更易于维护。使用 Spring Cloud 拥抱微服务世界,将您的应用程序提升到新的高度。
以上是使用 Spring Cloud 釋放微服務的力量的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
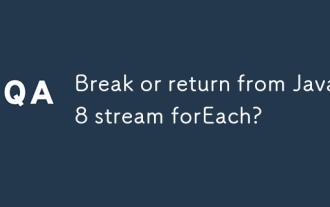
Java 8引入了Stream API,提供了一種強大且表達力豐富的處理數據集合的方式。然而,使用Stream時,一個常見問題是:如何從forEach操作中中斷或返回? 傳統循環允許提前中斷或返回,但Stream的forEach方法並不直接支持這種方式。本文將解釋原因,並探討在Stream處理系統中實現提前終止的替代方法。 延伸閱讀: Java Stream API改進 理解Stream forEach forEach方法是一個終端操作,它對Stream中的每個元素執行一個操作。它的設計意圖是處
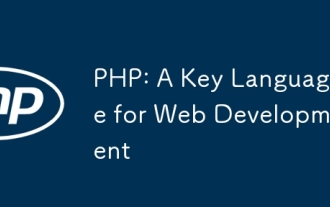
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
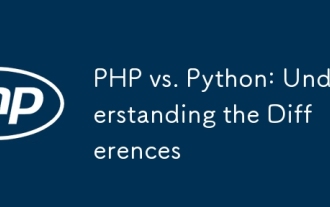
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
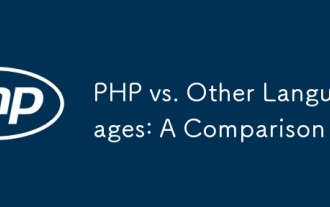
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
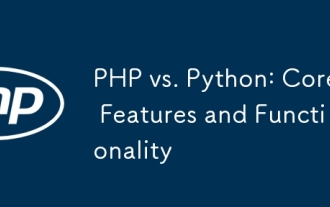
PHP和Python各有優勢,適合不同場景。 1.PHP適用於web開發,提供內置web服務器和豐富函數庫。 2.Python適合數據科學和機器學習,語法簡潔且有強大標準庫。選擇時應根據項目需求決定。
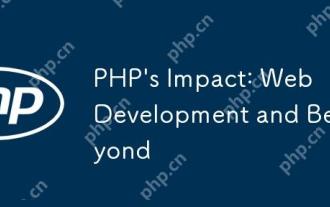
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
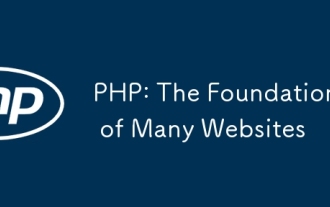
PHP成為許多網站首選技術棧的原因包括其易用性、強大社區支持和廣泛應用。 1)易於學習和使用,適合初學者。 2)擁有龐大的開發者社區,資源豐富。 3)廣泛應用於WordPress、Drupal等平台。 4)與Web服務器緊密集成,簡化開發部署。
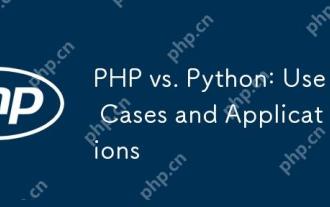
PHP適用於Web開發和內容管理系統,Python適合數據科學、機器學習和自動化腳本。 1.PHP在構建快速、可擴展的網站和應用程序方面表現出色,常用於WordPress等CMS。 2.Python在數據科學和機器學習領域表現卓越,擁有豐富的庫如NumPy和TensorFlow。
