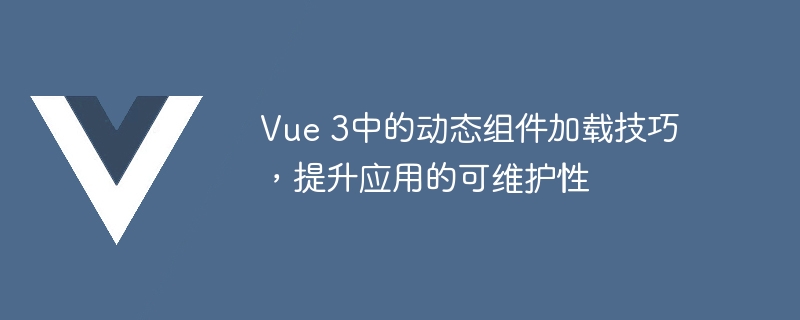
Vue 3中的動態元件載入技巧,提升應用程式的可維護性
引言:
在Vue 3中,動態元件載入是一種常見的技術,可以根據不同的條件加載不同的組件。這項技術在實際開發中非常有用,可以提升應用的可維護性和靈活性。本文將介紹一些在Vue 3中實作動態元件載入的技巧,並結合程式碼範例詳細說明。
一、使用v-if指令
v-if指令是Vue中用於條件性地渲染元件的一種方法。可以根據某個條件的真假來判斷是否渲染元件。以下是一個簡單的範例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | <template>
<div>
<button @click= "showComponent1 = !showComponent1" >Toggle Component 1</button>
<button @click= "showComponent2 = !showComponent2" >Toggle Component 2</button>
<div v- if = "showComponent1" >
<Component1 />
</div>
<div v- if = "showComponent2" >
<Component2 />
</div>
</div>
</template>
<script>
import Component1 from './Component1.vue' ;
import Component2 from './Component2.vue' ;
export default {
components: {
Component1,
Component2
},
data() {
return {
showComponent1: false,
showComponent2: false
};
}
};
</script>
|
登入後複製
在這個範例中,有兩個按鈕,分別用來切換元件1和元件2的顯示和隱藏。透過v-if指令,根據showComponent1和showComponent2的值來決定是否要渲染對應的元件。
二、使用動態元件
動態元件是Vue中另一個常用的載入元件的方法。它可以根據一個特定的屬性的值來動態地渲染不同的元件。下面是一個範例程式碼:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | <template>
<div>
<button @click= "currentComponent = 'Component1'" >Load Component 1</button>
<button @click= "currentComponent = 'Component2'" >Load Component 2</button>
<component :is= "currentComponent" />
</div>
</template>
<script>
import Component1 from './Component1.vue' ;
import Component2 from './Component2.vue' ;
export default {
components: {
Component1,
Component2
},
data() {
return {
currentComponent: null
};
}
};
</script>
|
登入後複製
在這個範例中,有兩個按鈕,分別用來載入元件1和元件2。透過給component 元件的:is屬性綁定一個變數currentComponent,根據currentComponent的值來動態渲染對應的元件。
三、使用非同步元件
在某些情況下,我們可能希望在需要的時候才載入元件,而不是一開始就載入所有的元件。 Vue 3中提供了非同步組件的機制。下面是一個範例程式碼:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | <template>
<div>
<button @click= "loadComponent1" >Load Component 1</button>
<button @click= "loadComponent2" >Load Component 2</button>
<component :is= "currentComponent" v- if = "currentComponent" />
</div>
</template>
<script>
export default {
data() {
return {
currentComponent: null
};
},
methods: {
loadComponent1() {
import( './Component1.vue' ).then(component => {
this.currentComponent = component. default ;
});
},
loadComponent2() {
import( './Component2.vue' ).then(component => {
this.currentComponent = component. default ;
});
}
}
};
</script>
|
登入後複製
在這個範例中,透過使用import函數動態地載入元件。當點擊按鈕時,會非同步載入對應的元件,並透過設定currentComponent變數來渲染元件。
總結:
本文介紹了在Vue 3中實作動態元件載入的幾種常見技巧,並結合程式碼範例進行了詳細說明。透過使用這些技巧,我們可以根據不同的條件靈活地加載不同的組件,提升應用的可維護性和靈活性。希望本文對您在Vue 3中使用動態元件載入有所幫助。
以上是Vue 3中的動態元件載入技巧,提升應用程式的可維護性的詳細內容。更多資訊請關注PHP中文網其他相關文章!