在C程式中,將以下內容翻譯為中文:找出鍊錶倒數第n個節點的程式
給定 n 個節點,任務是列印鍊錶末端的第 n 個節點。程式不得更改清單中節點的順序,而應僅列印鍊錶最後一個節點中的第 n 個節點。
範例
Input -: 10 20 30 40 50 60 N=3 Output -: 40
在上面的範例中,從第一個節點開始,遍歷到count-n 個節點,即10,20 30,40, 50,60,所以倒數第三個節點是40。
而不是如此有效率地遍歷整個列表可以遵循的方法-
- 取得一個臨時指針,比如說,節點類型的temp
- 將此暫存指標設定為指向的第一個節點頭指標
- 將計數器設定為清單中的節點數
- 將temp 移到temp → 下一個直到count -n
- 顯示temp → 資料
如果我們使用這個方法,計數將為5,程式將迭代循環直到5-3,即2,因此從0th 位置上的10 開始,直到20結果是在第1 個位置,第30 個位置在第二個位置。因此,透過這種方法,不需要遍歷整個清單直到結束,這將節省空間和記憶體。
演算法
Start Step 1 -> create structure of a node and temp, next and head as pointer to a structure node struct node int data struct node *next, *head, *temp End Step 2 -> declare function to insert a node in a list void insert(int val) struct node* newnode = (struct node*)malloc(sizeof(struct node)) newnode->data = val IF head= NULL set head = newnode set head->next = NULL End Else Set temp=head Loop While temp->next!=NULL Set temp=temp->next End Set newnode->next=NULL Set temp->next=newnode End Step 3 -> Declare a function to display list void display() IF head=NULL Print no node End Else Set temp=head Loop While temp!=NULL Print temp->data Set temp=temp->next End End Step 4 -> declare a function to find nth node from last of a linked list void last(int n) declare int product=1, i Set temp=head Loop For i=0 and i<count-n and i++ Set temp=temp->next End Print temp->data Step 5 -> in main() Create nodes using struct node* head = NULL Declare variable n as nth to 3 Call function insert(10) to insert a node Call display() to display the list Call last(n) to find nth node from last of a list Stop
範例
現場示範
#include<stdio.h> #include<stdlib.h> //structure of a node struct node{ int data; struct node *next; }*head,*temp; int count=0; //function for inserting nodes into a list void insert(int val){ struct node* newnode = (struct node*)malloc(sizeof(struct node)); newnode->data = val; newnode->next = NULL; if(head == NULL){ head = newnode; temp = head; count++; } else { temp->next=newnode; temp=temp->next; count++; } } //function for displaying a list void display(){ if(head==NULL) printf("no node "); else { temp=head; while(temp!=NULL) { printf("%d ",temp->data); temp=temp->next; } } } //function for finding 3rd node from the last of a linked list void last(int n){ int i; temp=head; for(i=0;i<count-n;i++){ temp=temp->next; } printf("</p><p>%drd node from the end of linked list is : %d" ,n,temp->data); } int main(){ //creating list struct node* head = NULL; int n=3; //inserting elements into a list insert(1); insert(2); insert(3); insert(4); insert(5); insert(6); //displaying the list printf("</p><p>linked list is : "); display(); //calling function for finding nth element in a list from last last(n); return 0; }
輸出
linked list is : 1 2 3 4 5 6 3rd node from the end of linked list is : 4
以上是在C程式中,將以下內容翻譯為中文:找出鍊錶倒數第n個節點的程式的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

熱門話題
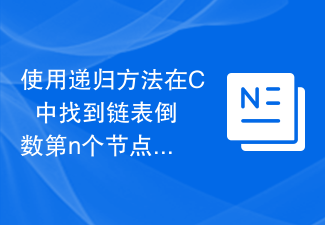
給定一個單鍊錶和正整數N作為輸入。目標是使用遞歸找到給定列表中從末尾算起的第N個節點。如果輸入清單有節點a→b→c→d→e→f且N為4,那麼倒數第4個節點將會是c。我們將首先遍歷直到列表中的最後一個節點以及從遞歸(回溯)增量計數返回。當count等於N時,則傳回指向目前節點的指標作為結果。讓我們來看看此的各種輸入輸出場景-輸入-List:-1→5→7→12→2→96→33N=3輸出−倒數第N個節點為:2解釋−第三個節點是2 。輸入−列表:-12→53→8→19→20→96→33N=8輸出-節點不存
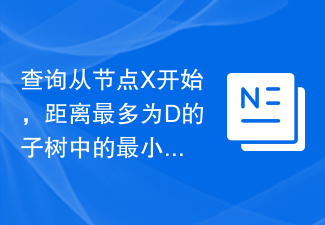
在進行電腦程式設計時,有時需要求出源自特定節點的子樹的最小權重,條件是該子樹不能包含距離指定節點超過D個單位的節點。這個問題出現在各個領域和應用中,包括圖論、基於樹的演算法和網路最佳化。子樹是較大樹結構的子集,指定的節點作為子樹的根節點。子樹包含根節點的所有後代及其連接邊。節點的權重是指分配給該節點的特定值,可以表示其重要性、重要性或其他相關指標。在這個問題中,目標是找到子樹中所有節點中的最小權重,同時將子樹限制在距離根節點最多D個單位的節點。在下面的文章中,我們將深入研究從子樹中挖掘最小權重的複雜性
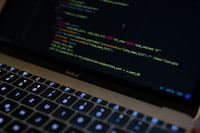
PHPSPL資料結構庫概述PHPSPL(標準php庫)資料結構庫包含一組類別和接口,用於儲存和操作各種資料結構。這些資料結構包括數組、鍊錶、堆疊、佇列和集合,每個資料結構都提供了一組特定的方法和屬性,用於操縱資料。數組在PHP中,數組是儲存一系列元素的有序集合。 SPL數組類別提供了對原生的PHP數組進行加強的功能,包括排序、過濾和映射。以下是使用SPL陣列類別的範例:useSplArrayObject;$array=newArrayObject(["foo","bar","baz"]);$array
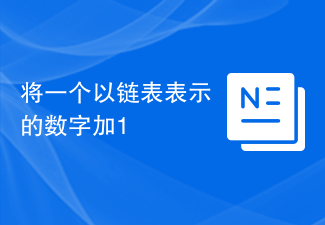
數字的鍊錶表示是這樣提供的:鍊錶的所有節點都被視為數字的一位數字。節點儲存數字,使得鍊錶的第一個元素保存數字的最高有效位,鍊錶的最後一個元素保存數字的最低有效位。例如,數字202345在鍊錶中表示為(2->0->2->3->4->5)。要為這個表示數字的鍊錶加1,我們必須檢查清單中最低有效位的值。如果小於9就可以了,否則程式碼將更改下一個數字,依此類推。現在讓我們看一個範例來了解如何做到這一點,1999表示為(1->9->9->9)並添加1應該將其
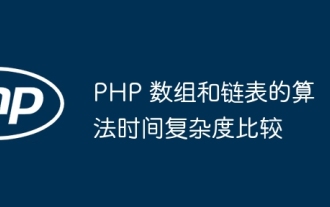
陣列與鍊錶的演算法時間複雜度比較:存取陣列O(1),鍊錶O(n);插入陣列O(1),鍊錶O(1)/O(n);刪除陣列O(1),鍊錶O (n);搜尋數組O(n),鍊錶O(n)。
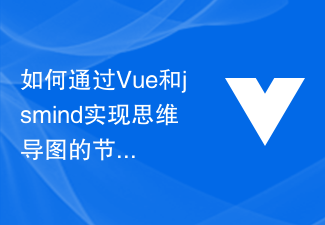
如何透過Vue和jsmind實現心智圖的節點複製和剪切功能?心智圖是一種常見的思考工具,能夠幫助我們整理想法、梳理思考邏輯。而節點複製和剪切功能是心智圖中常用的操作,能讓我們更方便重複利用現有的節點,提高思考整理的效率。在本文中,我們將使用Vue和jsmind這兩個工具來實現心智圖的節點複製和剪切功能。首先,我們需要安裝Vue和jsmind,並創建
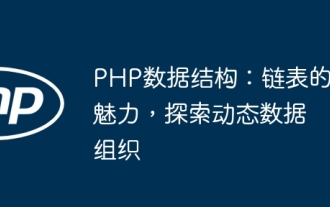
鍊錶是一種資料結構,採用一系列帶有資料和指標的節點組織元素,特別適合處理大型資料集和頻繁的插入/刪除操作。它的基本組成部分包括節點(資料和指向下一個節點的指標)和頭節點(指向鍊錶中第一個節點)。常見鍊錶操作包括:新增(尾部插入)、刪除(特定值)和遍歷。

在Python中,鍊錶是一種線性資料結構,它由一系列節點組成,每個節點包含一個值和對鍊錶中下一個節點的引用。在本文中,我們將討論如何在Python中將元素新增至鍊錶的第一個和最後一個位置。 LinkedListinPython鍊錶是一種引用資料結構,用於儲存一組元素。它在某種程度上類似於數組,但是在數組中,資料儲存在連續的記憶體位置中,而在鍊錶中,資料不受此條件限制。這意味著資料不是按順序存儲,而是以隨機的方式儲存在記憶體中。 Thisraisesonequestionthatis,howwecanac
