使一個字串等於另一個字串所需刪除的最長子字串的長度
在本文中,我們將討論找到需要刪除的最長子字串的長度以使一個字串等於另一個字串的問題。我們將首先理解問題陳述,然後探索解決問題的簡單和有效的方法,以及它們各自的演算法和時間複雜度。最後,我們將用 C 實作該解決方案。
問題陳述
給定兩個字串 A 和 B,確定需要從字串 A 中刪除的最長子字串的長度,使其等於字串 B。
天真的方法
最簡單的方法是產生字串 A 的所有可能的子字串,將它們一一刪除,然後檢查結果字串是否等於字串 B。如果是,我們將儲存刪除的子字串的長度。最後,我們將傳回所有刪除的子字串中的最大長度。
演算法(樸素)
將 maxLength 初始化為 0。
產生字串A的所有可能的子字串
對於每個子字串,將其從字串 A 中刪除,並檢查結果字串是否等於字串 B。
如果是,則將maxLength更新為maxLength與刪除子字串長度中的最大值。
傳回最大長度。
C 程式碼(樸素)
範例
#include <iostream> #include <string> #include <algorithm> int longestSubstringToDelete(std::string A, std::string B) { int maxLength = 0; for (int i = 0; i < A.length(); i++) { for (int j = i; j < A.length(); j++) { std::string temp = A; temp.erase(i, j - i + 1); if (temp == B) { maxLength = std::max(maxLength, j - i + 1); } } } return maxLength; } int main() { std::string A = "abcde"; std::string B = "acde"; std::cout << "Length of longest substring to be deleted: " << longestSubstringToDelete(A, B) << std::endl; return 0; }
輸出
Length of longest substring to be deleted: 1
時間複雜度(樸素) - O(n^3),其中 n 是字串 A 的長度。
高效的方法
解決這個問題的有效方法是找到兩個字串的最長公共子序列(LCS)。字串A中需要刪除的最長子字串的長度,使其等於字串B,其長度就是字串A的長度與LCS長度的差。
演算法(高效)
找出字串 A 和字串 B 的最長公共子序列 (LCS)。
傳回字串A的長度與LCS的長度之間的差。
C 程式碼(高效率)
#include <iostream> #include <string> #include <vector> #include <algorithm> int longestCommonSubsequence(std::string A, std::string B) { int m = A.length(); int n = B.length(); std::vector<std::vector<int>> dp(m + 1, std::vector<int>(n + 1, 0)); for (int i = 1; i <= m; i++) { for (int j = 1; j <= n; j++) { if (A[i - 1] == B[j - 1]) { dp[i][j] = 1 + dp[i - 1][j - 1]; } else { dp[i][j] = std::max(dp[i - 1][j], dp[i][j - 1]); } } } return dp[m][n]; } int longestSubstringToDelete(std::string A, std::string B) { int lcsLength = longestCommonSubsequence(A, B); return A.length() - lcsLength; } int main() { std::string A = "abcde"; std::string B = "acde"; std::cout << "Length of longest substring to be deleted: " << longestSubstringToDelete(A, B) << std::endl; return 0; }
輸出
Length of longest substring to be deleted: 1
時間複雜度(高效) - O(m * n),其中 m 是字串 A 的長度,n 是字串 B 的長度。
結論
在本文中,我們探討了尋找需要刪除的最長子字串的長度以使一個字串等於另一個字串的問題。我們討論了解決這個問題的簡單而有效的方法,以及它們的演算法和時間複雜度。高效方法利用最長公共子序列概念,與樸素方法相比,時間複雜度有了顯著提高。
以上是使一個字串等於另一個字串所需刪除的最長子字串的長度的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
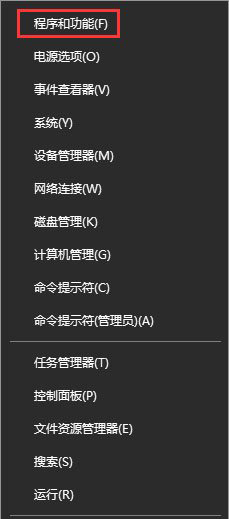
電腦C盤發現有個inetpub資料夾佔用極大的內存,這個inetpub是什麼資料夾?可以直接刪除嗎?其實inetpub是IIS服務端的一個資料夾,IIS全名為InternetInformationServices,也就網路資訊服務,是可以搭建網站、調試網站用的,如果不需要的話,可以將其卸載掉。 具體方法如下: 1、右鍵點選開始選單,選擇「程式與功能」。 2、開啟後點選「啟用或關閉Windows功能」。 3、在Windows功能清單中,取消勾選II
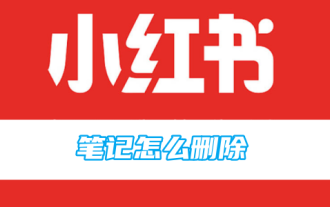
小紅書筆記怎麼刪除?在小紅書APP中是可以編輯筆記的,多數的用戶不知道小紅書筆記如何的刪除,接下來就是小編為用戶帶來的小紅書筆記刪除方法圖文教程,有興趣的用戶快來一起看看吧!小紅書使用教學小紅書筆記怎麼刪除1、先打開小紅書APP進入到主頁面,選擇右下角【我】進入到專區;2、之後在我的專區,點擊下圖所示的筆記頁面,選擇要刪除的筆記;3、進入到筆記頁面,右上角【三個點】;4、最後下方會展開功能欄,點選【刪除】即可完成。
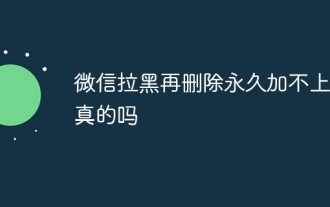
1.首先,拉黑再刪除永久加不上是假的,拉黑刪除後想要再加對方,只要對方同意即可。 2.如果用戶將某人封鎖,對方將無法向用戶發送訊息、查看用戶的朋友圈、與用戶通話。 3.封鎖並不意味著將對方從用戶的微信聯絡人清單中刪除。 4.如果用戶在封鎖後又將對方從用戶的微信聯絡人清單中刪除,那麼在刪除後是沒有辦法恢復的。 5.如果用戶想再加入對方為好友,需要對方同意並重新新增使用者。
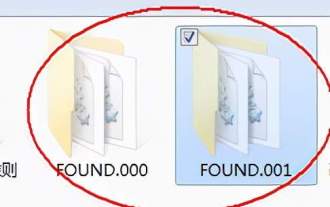
日常使用電腦的過程中,可能會收到found.000檔案遺失損壞的錯誤提示,這個found.000是什麼資料夾?如果沒有用的話,可以刪除嗎?既然有這麼多人不認識這個文件,下面小編就來跟大家仔細說說found.000文件夾吧~ 一、found.000是什麼文件夾當電腦出現因非法關機導致的文件部分或全部丟失時,可以在位於系統分割區中指定目錄下找到名為「found.000」的特殊資料夾及其內部所包含的以「.chk」為副檔名的檔案。 這個「fo
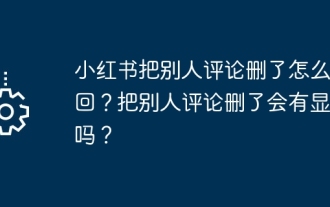
小紅書作為一款熱門的社群電商平台,用戶之間的互動評論是平台中不可或缺的溝通方式。有時候,我們可能會發現自己的評論被其他人刪除,這種情況可能會讓我們感到困惑。一、小紅書把別人評論刪了怎麼找回來?當發現自己的評論被刪除時,首先可以嘗試在平台上直接搜尋相關的貼文或商品,查看是否還能找到該評論。如果評論被刪除後仍然顯示,那麼可能是被原帖主刪除的,這時候可以嘗試聯絡原帖主,詢問其刪除評論的原因,並要求恢復評論。如果評論已經被完全刪除且無法在原始貼文上找到,那麼在平台上恢復評論的機會相對較小。可以嘗試使用其他途徑
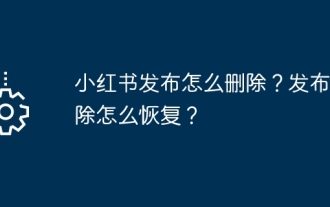
小紅書作為一個受歡迎的社群電商平台,吸引了大量用戶分享生活點滴和購物心得。有時候我們可能會不經意發布一些不合適的內容,這時候需要及時刪除,這樣可以更好地維護個人形像或遵守平台規定。一、小紅書發布怎麼刪除? 1.登入小紅書帳號,進入個人首頁。 2.在個人主頁下方,找到「我的創作」選項,點選進入。 3.在「我的創作」頁面,你可以看到所有發布的內容,包括筆記、影片等。 4.找到需要刪除的內容,點選右側的「...」按鈕。 5.在彈出的選單中,選擇“刪除”選項。 6.確認刪除後,該條內容將從你的個人主頁和公開頁面消失
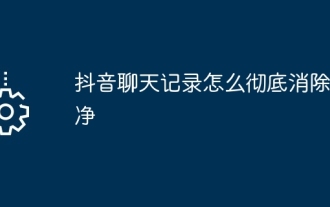
1.開啟抖音app,點選介面底部的【訊息】,點選需要刪除的聊天對話入口。 2.長按任一聊天記錄,點選【多選】,勾選想要刪除的聊天記錄。 3.點選右下角的【刪除】按鈕,在彈出的視窗中選擇【確認刪除】即可將這些記錄永久刪除。

微信的文件傳輸助手是每個用戶都有的,有些用戶將它當做成了備忘錄,會記錄一些東西。那麼微信檔案傳輸助手要怎麼徹底刪除呢?下面就讓小編為大家詳細介紹一下吧。微信檔案傳輸助手怎麼徹底刪除答案:【微信】-【長按檔案傳輸助手】-【刪除該聊天】。具體步驟:1、先開啟微信軟體,進入到首頁後我們找到【檔案傳輸助理】長按;2、然後會彈出標為未讀、置頂該聊天、不顯示該聊天、刪除該聊天,在這裡我們點選【刪除該聊天】即可;
