如何使用Python中的裝飾器函數
如何使用Python中的裝飾函數
在Python程式設計中,裝飾器(decorators)是一種非常有用的工具。它允許我們在不修改原始函數程式碼的情況下,對函數進行額外的功能擴展。裝飾器函數可以在函數執行前後自動執行一些操作,例如記錄日誌、計時、驗證權限等。本文將介紹裝飾器函數的基本概念,並提供一些具體的程式碼範例。
一、裝飾器函數的定義
裝飾函數是一個接受函數作為參數並傳回一個新函數的函數。它可以使用@語法將其應用於函數。以下是一個簡單的裝飾器函數定義的範例:
def decorator_function(original_function): def wrapper_function(*args, **kwargs): # 在函数执行前的操作 print("Before executing the function") # 执行原始函数 result = original_function(*args, **kwargs) # 在函数执行后的操作 print("After executing the function") return result return wrapper_function
二、裝飾器函數的應用
裝飾函數可以在函數執行前後執行一些額外的操作。以下是使用裝飾器函數實作計時功能的範例:
import time def timer_decorator(original_function): def wrapper_function(*args, **kwargs): start_time = time.time() result = original_function(*args, **kwargs) end_time = time.time() execution_time = end_time - start_time print(f"Execution time: {execution_time} seconds") return result return wrapper_function @timer_decorator def my_function(): time.sleep(1) print("Function executed") my_function()
在上面的範例中,timer_decorator裝飾函數被應用於my_function函數。當呼叫my_function時,裝飾器會在函數執行前記錄開始時間,在函數執行後計算結束時間,並計算函數的執行時間。
三、裝飾器函數的參數
裝飾函數可以接受參數,以便對不同的函數套用不同的裝飾器。以下是一個帶有參數的裝飾器函數的範例:
def prefix_decorator(prefix): def decorator_function(original_function): def wrapper_function(*args, **kwargs): print(f"{prefix}: Before executing the function") result = original_function(*args, **kwargs) print(f"{prefix}: After executing the function") return result return wrapper_function return decorator_function @prefix_decorator("LOG") def my_function(): print("Function executed") my_function()
在上面的範例中,prefix_decorator函數傳回一個裝飾器函數,該函數在函數執行前後分別列印帶有前綴的日誌資訊。
四、多個裝飾器的應用
可以將多個裝飾器函數應用於同一個函數,形成裝飾器的堆疊效果。下面是一個應用多個裝飾器的範例:
def decorator1(original_function): def wrapper_function(*args, **kwargs): print("Decorator 1: Before executing the function") result = original_function(*args, **kwargs) print("Decorator 1: After executing the function") return result return wrapper_function def decorator2(original_function): def wrapper_function(*args, **kwargs): print("Decorator 2: Before executing the function") result = original_function(*args, **kwargs) print("Decorator 2: After executing the function") return result return wrapper_function @decorator1 @decorator2 def my_function(): print("Function executed") my_function()
在上面的範例中,decorator1和decorator2裝飾器函數依序應用於my_function函數。當呼叫my_function時,裝飾器2會在裝飾器1之後執行。
總結:
裝飾器函數是Python中非常有用的工具,它可以在不修改原始函數程式碼的情況下,對函數進行額外的功能擴展。透過提供一些具體程式碼範例,本文希望能夠幫助讀者理解裝飾器函數的基本概念以及如何使用它們。使用裝飾器函數可以減少程式碼重複,並使程式碼更加模組化和易於維護。
以上是如何使用Python中的裝飾器函數的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
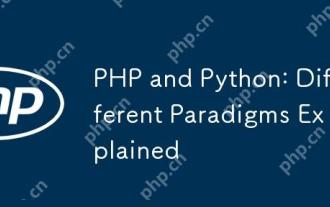
PHP主要是過程式編程,但也支持面向對象編程(OOP);Python支持多種範式,包括OOP、函數式和過程式編程。 PHP適合web開發,Python適用於多種應用,如數據分析和機器學習。
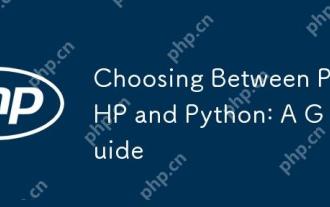
PHP適合網頁開發和快速原型開發,Python適用於數據科學和機器學習。 1.PHP用於動態網頁開發,語法簡單,適合快速開發。 2.Python語法簡潔,適用於多領域,庫生態系統強大。
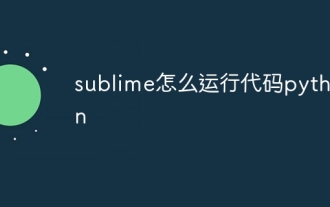
在 Sublime Text 中運行 Python 代碼,需先安裝 Python 插件,再創建 .py 文件並編寫代碼,最後按 Ctrl B 運行代碼,輸出會在控制台中顯示。
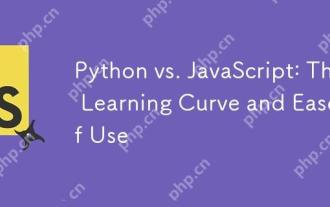
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
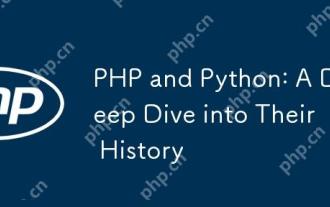
PHP起源於1994年,由RasmusLerdorf開發,最初用於跟踪網站訪問者,逐漸演變為服務器端腳本語言,廣泛應用於網頁開發。 Python由GuidovanRossum於1980年代末開發,1991年首次發布,強調代碼可讀性和簡潔性,適用於科學計算、數據分析等領域。
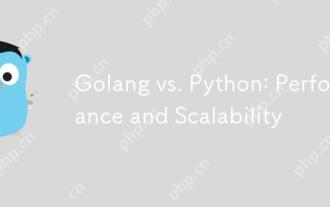
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
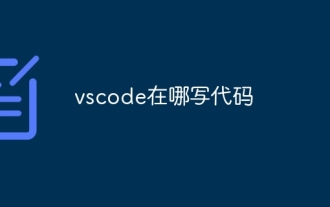
在 Visual Studio Code(VSCode)中編寫代碼簡單易行,只需安裝 VSCode、創建項目、選擇語言、創建文件、編寫代碼、保存並運行即可。 VSCode 的優點包括跨平台、免費開源、強大功能、擴展豐富,以及輕量快速。
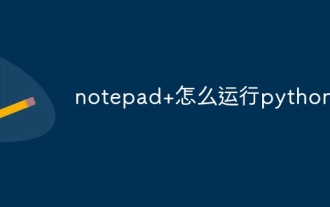
在 Notepad 中運行 Python 代碼需要安裝 Python 可執行文件和 NppExec 插件。安裝 Python 並為其添加 PATH 後,在 NppExec 插件中配置命令為“python”、參數為“{CURRENT_DIRECTORY}{FILE_NAME}”,即可在 Notepad 中通過快捷鍵“F6”運行 Python 代碼。
