Vue專案中的TypeError: Cannot read property 'XXX' of undefined,該如何處理?
在Vue 專案中開發中,偶爾會遇到TypeError: Cannot read property 'XXX' of undefined 這樣的錯誤,在頁面中表現為頁面無法渲染,無法取得到數據等問題。這種錯誤可能會出現在使用 Vue 框架時,資料傳輸、元件通訊、非同步請求等多種場景下。本文將詳細介紹這種錯誤的產生原因和解決方法。
一、產生原因
TypeError: Cannot read property 'XXX' of undefined 的原因多種多樣,以下是常見的幾種情況:
- #訪問未定義的物件屬性
這種情況下,既可以是在頁面渲染時遇到,也可以在頁面載入時就被偵測到。
例如:
const obj = {}; console.log(obj.xxx); //TypeError: Cannot read property 'xxx' of undefined
這個錯誤表示嘗試存取一個未定義的屬性 xxx。
- 取得非同步請求的資料出錯
在請求資料時,由於各種原因,可能出現請求傳回的資料為空或 undefined。
例如:
axios.get('/api/data').then(res => { const data = res.data; console.log(data.xxx); //TypeError: Cannot read property 'xxx' of undefined }).catch(err => { console.log(err); })
在這種情況下,如果伺服器傳回的資料為空或 undefined,嘗試存取其屬性時就會報錯。
- 存取已經銷毀的元件
在 Vue 元件中,如果在元件被銷毀時還在存取該元件的屬性或方法,就會出現該錯誤。
例如:
export default { data () { return { list: [] } }, created () { this.timer = setTimeout(() => { this.list = [1, 2, 3]; }, 1000); }, beforeDestroy () { clearTimeout(this.timer); } }
在這個元件中,建立了一個計時器,1 秒鐘後修改了元件中的 data 中的 list 屬性。在定時器沒有執行前,如果銷毀了該元件,就會出現該錯誤。
- 父元件未傳遞props 屬性
在Vue 元件中,如果需要透過父元件傳遞props 屬性時,如果父元件中沒有傳遞該屬性,就會出現該錯誤。
例如:
<template> <my-component :list="list"></my-component> </template> <script> import MyComponent from './MyComponent.vue'; export default { components: { MyComponent } } </script>
在這個範例中,父元件中沒有定義 list 屬性,但是卻將 list 屬性透過 props 綁定到了子元件中,這將會出現該錯誤。
二、解決方法
- 檢查程式碼中是否有未定義的屬性
在出現TypeError: Cannot read property 'XXX' of undefined 這種錯誤時,第一步應該檢查程式碼中是否有存取未定義的屬性。可以透過新增斷點、列印錯誤物件等方式找到具體出錯位置。
- 處理非同步請求的資料
在進行非同步請求時,需要對傳回的資料進行校驗,確保其不是 undefined。
例如:
axios.get('/api/data').then(res => { const data = res.data; if (data) { console.log(data.xxx); } else { console.log('返回的数据为空或者为 undefined'); } }).catch(err => { console.log(err); });
透過在取得資料之前先對傳回的資料進行校驗,來避免存取 undefined 引發錯誤。
- 加入元件生命週期鉤子
在元件週期鉤子中,可以控制元件的建立和銷毀,從而避免存取已經銷毀的元件。
例如:
export default { data () { return { list: [] } }, created () { this.timer = setTimeout(() => { if (!this._isDestroyed) { this.list = [1, 2, 3]; } }, 1000); }, beforeDestroy () { clearTimeout(this.timer); this._isDestroyed = true; } }
透過新增 _isDestroyed 屬性,來防止在元件已經銷毀的情況下存取該元件的屬性。
- 設定預設值
在使用 props 屬性時,應該設定預設值,避免在父元件中未定義的情況下引發錯誤。
例如:
export default { props: { list: { type: Array, default: () => [] } } }
當父元件未傳遞 list 屬性時,使用預設空數組。
總之,在開發 Vue 專案時,出現 TypeError: Cannot read property 'XXX' of undefined 這種錯誤時,需要先找到出錯原因,然後再採取適當的解決策略。這些解決方法不僅可以解決該問題,還可以幫助我們更好地理解 Vue 的資料流和元件通訊等機制。
以上是Vue專案中的TypeError: Cannot read property 'XXX' of undefined,該如何處理?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
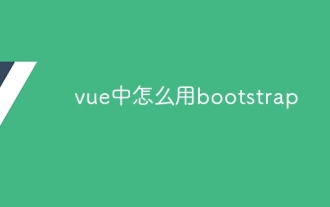
在 Vue.js 中使用 Bootstrap 分為五個步驟:安裝 Bootstrap。在 main.js 中導入 Bootstrap。直接在模板中使用 Bootstrap 組件。可選:自定義樣式。可選:使用插件。
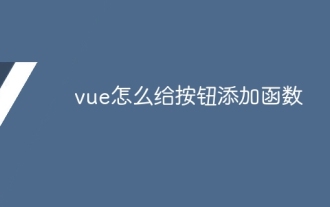
可以通過以下步驟為 Vue 按鈕添加函數:將 HTML 模板中的按鈕綁定到一個方法。在 Vue 實例中定義該方法並編寫函數邏輯。
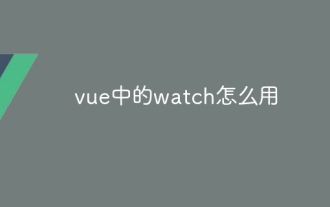
Vue.js 中的 watch 選項允許開發者監聽特定數據的變化。當數據發生變化時,watch 會觸發一個回調函數,用於執行更新視圖或其他任務。其配置選項包括 immediate,用於指定是否立即執行回調,以及 deep,用於指定是否遞歸監聽對像或數組的更改。
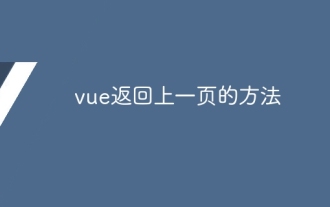
Vue.js 返回上一頁有四種方法:$router.go(-1)$router.back()使用 <router-link to="/"> 組件window.history.back(),方法選擇取決於場景。
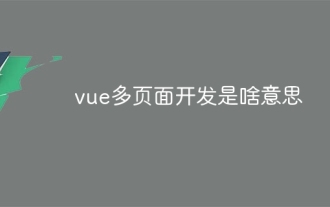
Vue 多頁面開發是一種使用 Vue.js 框架構建應用程序的方法,其中應用程序被劃分為獨立的頁面:代碼維護性:將應用程序拆分為多個頁面可以使代碼更易於管理和維護。模塊化:每個頁面都可以作為獨立的模塊,便於重用和替換。路由簡單:頁面之間的導航可以通過簡單的路由配置來管理。 SEO 優化:每個頁面都有自己的 URL,這有助於搜索引擎優化。

NetflixusesAcustomFrameworkcalled“ Gibbon” BuiltonReact,notReactorVuedIrectly.1)TeamSperience:selectBasedonFamiliarity.2)ProjectComplexity:vueforsimplerprojects:reactforforforproproject,reactforforforcompleplexones.3)cocatizationneedneeds:reactoffipicatizationneedneedneedneedneedneeds:reactoffersizationneedneedneedneedneeds:reactoffersizatization needefersmoreflexibleise.4)
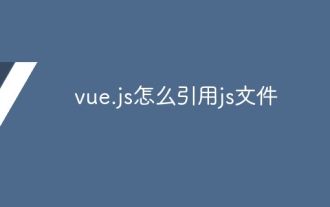
在 Vue.js 中引用 JS 文件的方法有三種:直接使用 <script> 標籤指定路徑;利用 mounted() 生命週期鉤子動態導入;通過 Vuex 狀態管理庫進行導入。
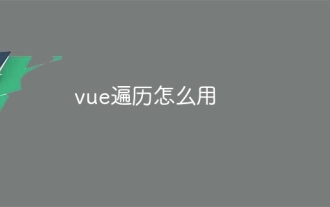
Vue.js 遍歷數組和對像有三種常見方法:v-for 指令用於遍歷每個元素並渲染模板;v-bind 指令可與 v-for 一起使用,為每個元素動態設置屬性值;.map 方法可將數組元素轉換為新數組。
