提升程式碼品質和開發效率的方法:掌握Spring AOP
學習如何利用Spring AOP提升程式碼品質和開發效率
#引言:
在大型軟體開發專案中,程式碼品質和開發效率是非常重要的考量因素。為了提高程式碼的質量,我們經常引入各種設計模式和編碼規範。而為了提高開發效率,我們通常會使用一些可以重複使用的程式碼片段或自動化工具。
在這篇文章中,我們將重點放在Spring AOP(Aspect-Oriented Programming)的使用,來提升程式碼品質和開發效率。我們將透過具體的程式碼範例來說明如何利用Spring AOP進行日誌記錄、異常處理和效能監控。
- 日誌記錄
在大部分軟體專案中,日誌記錄是不可或缺的。透過記錄系統的運作狀態和關鍵訊息,我們可以方便地進行故障排查和效能最佳化。在使用Spring AOP時,我們可以很方便地對程式碼進行日誌記錄。
首先,我們需要定義一個日誌切面類別(LoggingAspect),並使用@Aspect註解將其標記為切面:
@Aspect @Component public class LoggingAspect { @Before("execution(* com.example.service.*.*(..))") public void logBefore(JoinPoint joinPoint) { String methodName = joinPoint.getSignature().getName(); System.out.println("Before method: " + methodName); } @AfterReturning(pointcut = "execution(* com.example.service.*.*(..))", returning = "result") public void logAfterReturning(JoinPoint joinPoint, Object result) { String methodName = joinPoint.getSignature().getName(); System.out.println("After method: " + methodName); System.out.println("Result: " + result); } @AfterThrowing(pointcut = "execution(* com.example.service.*.*(..))", throwing = "ex") public void logAfterThrowing(JoinPoint joinPoint, Exception ex) { String methodName = joinPoint.getSignature().getName(); System.out.println("Exception occurred in method: " + methodName); System.out.println("Exception: " + ex.getMessage()); } }
在上述程式碼中,使用@Before、@AfterReturning和@AfterThrowing註解分別表示在方法執行前、方法正常返回後、方法拋出異常後執行的邏輯。
然後,我們需要在Spring設定檔中啟用AOP,並掃描日誌切面類別:
<aop:aspectj-autoproxy /> <context:component-scan base-package="com.example.aspect" />
最後,在需要記錄日誌的服務類別中新增@AspectJ註解:
@Service public class UserService { public void saveUser(User user) { // 保存用户 } }
有了上述配置,我們在呼叫UserService的方法時,就會自動觸發LoggingAspect中的切面邏輯,實現日誌的記錄。
- 異常處理
另一個常見的需求是對系統中的異常進行統一處理,例如記錄異常訊息、發送錯誤警報等。使用Spring AOP可以方便地實現這些功能。
首先,我們需要定義一個異常處理切面類別(ExceptionAspect),並使用@Aspect註解將其標記為切面:
@Aspect @Component public class ExceptionAspect { @AfterThrowing(pointcut = "execution(* com.example.service.*.*(..))", throwing = "ex") public void handleException(JoinPoint joinPoint, Exception ex) { String methodName = joinPoint.getSignature().getName(); System.out.println("Exception occurred in method: " + methodName); System.out.println("Exception: " + ex.getMessage()); // 发送错误报警等 } }
在上述程式碼中,我們使用@AfterThrowing註解指定了異常拋出後執行的邏輯。
然後,我們需要在Spring設定檔中啟用AOP,並掃描異常處理切面類別:
<aop:aspectj-autoproxy /> <context:component-scan base-package="com.example.aspect" />
最後,在需要異常處理的服務類別中新增@AspectJ註解。
- 效能監控
除了日誌記錄和異常處理外,效能監控也是提高程式碼品質和開發效率的關鍵因素之一。使用Spring AOP可以很方便地對方法的執行時間進行統計。
首先,我們需要定義一個效能監控切面類別(PerformanceAspect),並使用@Aspect註解將其標記為切面:
@Aspect @Component public class PerformanceAspect { @Around("execution(* com.example.service.*.*(..))") public Object measurePerformance(ProceedingJoinPoint proceedingJoinPoint) throws Throwable { long startTime = System.currentTimeMillis(); Object result = proceedingJoinPoint.proceed(); long endTime = System.currentTimeMillis(); String methodName = proceedingJoinPoint.getSignature().getName(); System.out.println("Method: " + methodName); System.out.println("Execution time: " + (endTime - startTime) + "ms"); return result; } }
在上述程式碼中,我們使用@Around註解來定義方法執行前後的切面邏輯。在方法開始前記錄開始時間,在方法結束後記錄結束時間並計算執行時間。
然後,我們需要在Spring設定檔中啟用AOP,並掃描效能監控切面類別:
<aop:aspectj-autoproxy /> <context:component-scan base-package="com.example.aspect" />
最後,在需要效能監控的服務類別中加入@AspectJ註解。
總結:
透過學習如何利用Spring AOP提升程式碼品質和開發效率,我們可以更方便地實現日誌記錄、異常處理和效能監控等功能。透過統一的切面配置,我們可以減少重複程式碼的編寫,並且可以非常方便地對關注點進行管理。希望本文的內容能幫助讀者更好地理解和使用Spring AOP,提高軟體開發專案的品質和效率。
以上是提升程式碼品質和開發效率的方法:掌握Spring AOP的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
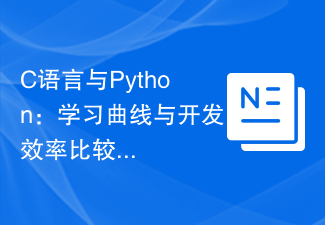
C语言与Python:学习曲线与开发效率比较C语言和Python是两种常用的编程语言,它们在学习曲线和开发效率上有着显著的区别。本文将从具体的代码示例入手,对这两种语言进行比较分析。首先,我们来看一段简单的计算两个数之和的程序。C语言示例:#includeintmain(){inta=5;in
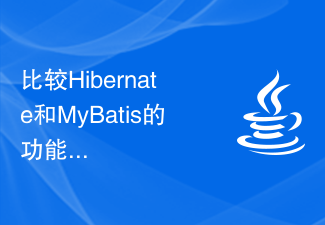
標題:探索Hibernate和MyBatis的功能差異及其對開發效率的影響引言:在Java開發領域中,ORM(物件關係映射)框架扮演著重要角色,它們簡化了資料庫操作,提高了開發效率。 Hibernate和MyBatis作為開發者最常使用的兩種ORM框架,具有不同的特點和適用場景。本文將對Hibernate和MyBatis的功能差異進行探討,並分析它們對開發效率的

在PHP開發中,維持程式碼品質至關重要,可提高軟體的可靠性、可維護性和安全性。持續監控程式碼品質可以主動發現問題,促進及早修復,並防止它們進入生產環境。在這篇文章中,我們將探討如何使用jenkins和SonarQube建立一個php專案的持續監控管道。 Jenkins:持續整合伺服器Jenkins是一個開源的持續整合伺服器,可自動化建置、測試和部署流程。它允許開發人員設定作業,這些作業將定期觸發並執行一系列任務。對於PHP項目,我們可以設定Jenkins作業來完成以下任務:從版本控制系統中檢出程式碼運
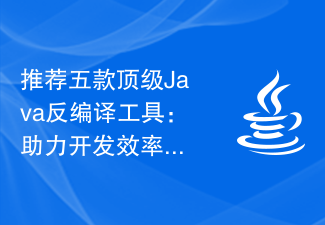
提升開發效率的利器:推薦五款頂尖Java反編譯工具身為Java開發人員,我們常常會遇到需要檢視或修改已編譯的Java類別的情況。儘管Java是一種編譯型語言,但在某些情況下,我們可能需要對已編譯的類別進行反編譯,以便分析原始程式碼或修改其中的某些部分。在這種情況下,Java反編譯工具就變得非常有用了。本文將介紹並推薦五款頂尖的Java反編譯工具,協助開發人員提升
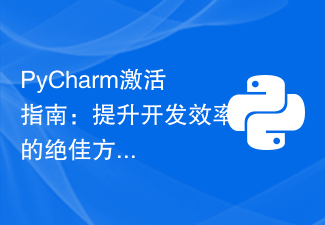
快速啟動PyCharm:讓你的開發效率倍增!引言:PyCharm作為一款功能強大的Python整合開發環境(IDE),可以大幅提高我們的開發效率。然而,在使用過程中,我們可能會遇到需要啟動PyCharm的問題。本文將為大家分享如何快速啟動PyCharm,讓你的開發效率倍增!同時,我們將提供具體的程式碼範例來幫助你更好地理解和操作。一、什麼是PyCharm? P
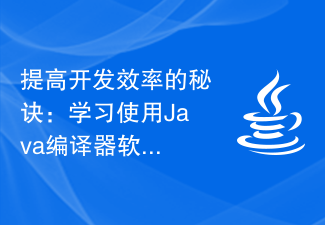
學習如何使用Java編譯器軟體來提高您的開發效率隨著軟體開發行業的發展,使用編譯器軟體來優化程式碼的速度和品質變得越來越重要。 Java作為一種廣泛使用的程式語言,在開發過程中也需要使用編譯器軟體來編譯和執行程式碼。本文將介紹一些常用的Java編譯器軟體,並提供一些使用它們來提高開發效率的技巧。 EclipseEclipse是一款十分流行的Java整合開發環境(ID
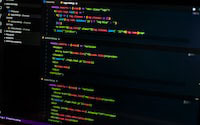
1.代碼自動補全:揮灑自如,舞步輕盈vscode整合了PHPIntelliSense功能,可在您輸入代碼時提供智慧建議。它自動補全函數、類別、常數和變量,減少了鍵入錯誤和語法錯誤,讓您在編碼時如同行雲流水,揮灑自如。範例:$name="JohnDoe";echo$name;//VSCode自動補全$name2.錯誤檢查:鷹眼掃描,步調嚴謹VSCode內建Linter,即時偵測程式碼中的語法錯誤和潛在問題。它在您鍵入時下劃線標註錯誤,幫助您及早發現並修復問題,避免盲目調試的困擾。範例://VSCode
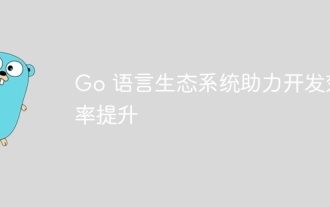
Go語言生態系統透過標準函式庫的強大功能和活躍的第三方函式庫社群來提升開發效率。標準庫功能卓越,包括面向並發程式設計、強大網路支援和豐富的容器類型。第三方庫生態系統為Go開發者提供了豐富的功能拓展,如Web框架、資料庫存取和機器學習等實戰案例展示如何使用Echo建立RESTfulAPI,進一步體現了Go語言生態系統的便利性和高效性。
