探索AJAX請求:了解各種AJAX請求的方法
探索AJAX請求:了解各種AJAX請求的方法,需要具體程式碼範例
引言:
在現代Web開發中,AJAX(Asynchronous JavaScript and XML)被廣泛使用,可以實現網頁與伺服器之間的非同步資料交換,使用戶能夠在不刷新整個頁面的情況下獲得最新的資料。本文將介紹AJAX的基本原理,以及如何使用不同的AJAX請求方法,同時提供具體的程式碼範例。
一、AJAX的基本原理
AJAX的基本原理是透過瀏覽器內建的XMLHttpRequest物件與伺服器進行資料交換。它透過JavaScript在頁面上傳送請求並接收回應,然後使用DOM操作將資料插入頁面中對應的位置,實現頁面局部刷新。
二、使用AJAX的常見方法
- GET請求:
GET請求是最常見的AJAX請求方法之一,它用於從伺服器取得資料。以下是一個使用原生JavaScript實現的GET請求範例:
function getData(url, callback) { var xhr = new XMLHttpRequest(); xhr.open("GET", url, true); xhr.onreadystatechange = function() { if (xhr.readyState == 4 && xhr.status == 200) { var data = JSON.parse(xhr.responseText); callback(data); } }; xhr.send(); } getData("https://api.example.com/data", function(data) { console.log(data); });
- POST請求:
POST請求用於向伺服器發送數據,常用於表單提交等場景。以下是一個使用jQuery的POST請求範例:
$.ajax({ url: "https://api.example.com/post", type: "POST", data: { username: "example", password: "123456" }, success: function(response) { console.log(response); } });
- PUT請求:
PUT請求用於更新伺服器上的資料。以下是一個使用fetch API實作的PUT請求範例:
fetch("https://api.example.com/update", { method: "PUT", body: JSON.stringify({ id: 1, name: "example" }), headers: { "Content-Type": "application/json" } }) .then(function(response) { return response.json(); }) .then(function(data) { console.log(data); });
- DELETE請求:
DELETE請求用於刪除伺服器上的資料。以下是一個使用Axios實現的DELETE請求範例:
axios.delete("https://api.example.com/delete", { params: { id: 1 } }) .then(function(response) { console.log(response.data); }) .catch(function(error) { console.log(error); });
三、跨域請求的處理
由於同源策略的限制,AJAX請求在預設情況下只能傳送給相同域名下的伺服器。為了解決這個問題,可以使用JSONP、CORS、代理等方式。以下是使用JSONP實作跨域請求的範例:
function getData(callback) { var script = document.createElement("script"); script.src = "https://api.example.com/data?callback=" + callback; document.body.appendChild(script); } function handleData(data) { console.log(data); } getData("handleData");
四、AJAX請求的錯誤處理
在進行AJAX請求時,需要處理可能出現的錯誤。以下是使用fetch API實作錯誤處理的範例:
fetch("https://api.example.com/data") .then(function(response) { if (response.ok) { return response.json(); } else { throw new Error("请求失败,状态码:" + response.status); } }) .then(function(data) { console.log(data); }) .catch(function(error) { console.log("请求错误:" + error); });
結論:
透過本文的介紹,我們了解了AJAX的基本原理,並學習了使用不同的AJAX請求方法。希望這些具體的程式碼範例能幫助讀者更能掌握AJAX請求的使用方法,進而提高Web開發的效率和使用者體驗。
以上是探索AJAX請求:了解各種AJAX請求的方法的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
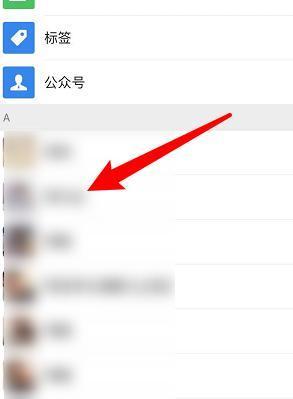
而後悔莫及、人們常常會因為一些原因不小心刪除某些聯絡人、微信作為一款廣泛使用的社群軟體。幫助用戶解決這個問題,本文將介紹如何透過簡單的方法找回被刪除的聯絡人。 1.了解微信聯絡人刪除機制這為我們找回被刪除的聯絡人提供了可能性、微信中的聯絡人刪除機制是將其從通訊錄中移除,但並未完全刪除。 2.使用微信內建「通訊錄恢復」功能微信提供了「通訊錄恢復」節省時間和精力,使用者可以透過此功能快速找回先前刪除的聯絡人,功能。 3.進入微信設定頁面點選右下角,開啟微信應用程式「我」再點選右上角設定圖示、進入設定頁面,,
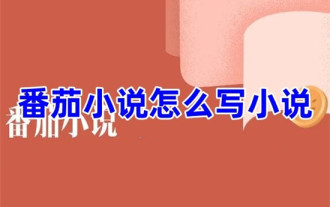
番茄小說是一款非常熱門的小說閱讀軟體,我們在番茄小說中經常會有新的小說和漫畫可以去閱讀,每一本小說和漫畫都很有意思,很多小伙伴也想著要去寫小說來賺取賺取零用錢,在把自己想要寫的小說內容編輯成文字,那麼我們要怎麼樣在這裡面去寫小說呢?小伙伴們都不知道,那就讓我們一起到本站本站中花點時間來看寫小說的方法介紹。分享番茄小說寫小說方法教學 1、先在手機上打開番茄免費小說app,點擊個人中心——作家中心 2、跳到番茄作家助手頁面——點擊創建新書在小說的結
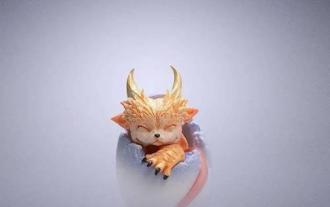
手機遊戲成為了人們生活中不可或缺的一部分,隨著科技的發展。它以其可愛的龍蛋形象和有趣的孵化過程吸引了眾多玩家的關注,而其中一款備受矚目的遊戲就是手機版龍蛋。幫助玩家們在遊戲中更好地培養和成長自己的小龍,本文將向大家介紹手機版龍蛋的孵化方法。 1.選擇合適的龍蛋種類玩家需要仔細選擇自己喜歡並且適合自己的龍蛋種類,根據遊戲中提供的不同種類的龍蛋屬性和能力。 2.提升孵化機的等級玩家需要透過完成任務和收集道具來提升孵化機的等級,孵化機的等級決定了孵化速度和孵化成功率。 3.收集孵化所需的資源玩家需要在遊戲中
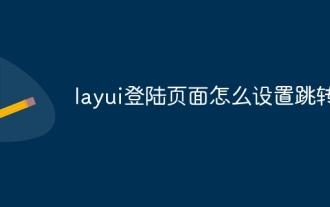
layui 登入頁面跳轉設定步驟:新增跳轉代碼:在登入表單提交按鈕點選事件中新增判斷,成功登入後透過 window.location.href 跳到指定頁面。修改 form 配置:在 lay-filter="login" 的 form 元素中新增 hidden 輸入字段,name 為 "redirect",value 為目標頁面位址。
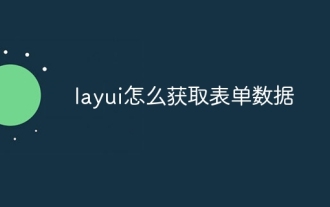
layui 提供了多種取得表單資料的方法,包括直接取得表單所有欄位資料、取得單一表單元素值、使用formAPI.getVal() 方法取得指定欄位值、將表單資料序列化並作為AJAX 請求參數,以及監聽表單提交事件獲取資料。
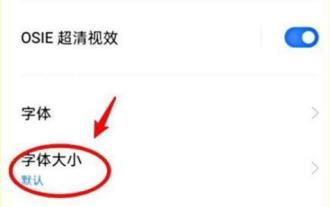
字體大小的設定成為了重要的個人化需求,隨著手機成為人們日常生活的重要工具。以滿足不同使用者的需求、本文將介紹如何透過簡單的操作,提升手機使用體驗,調整手機字體大小。為什麼需要調整手機字體大小-調整字體大小可以使文字更清晰易讀-適合不同年齡段用戶的閱讀需求-方便視力不佳的用戶使用手機系統自帶字體大小設置功能-如何進入系統設置界面-在在設定介面中找到並進入"顯示"選項-找到"字體大小"選項並進行調整第三方應用調整字體大小-下載並安裝支援字體大小調整的應用程式-開啟應用程式並進入相關設定介面-根據個人
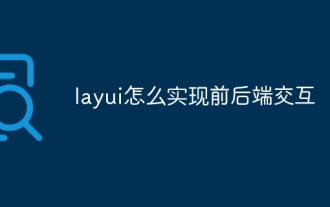
使用 layui 進行前後端互動有以下方法:$.ajax 方法:簡化非同步 HTTP 請求。自訂請求物件:允許發送自訂請求。 Form 控制項:處理表單提交和資料驗證。 Upload 控制項:輕鬆實作檔案上傳。
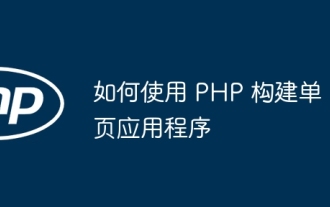
使用PHP建立單頁應用程式(SPA)的步驟:建立PHP文件,並載入Vue.js。定義Vue實例,並建立包含文字輸入和輸出文字的HTML介面。建立包含Vue組件的JavaScript框架檔案。將JavaScript框架檔案包含到PHP檔案中。
