Golang Docker Selenium Chrome
Golang Docker Selenium Chrome是一种强大的组合,为开发人员提供了便捷且高效的工具链。Golang是一种高性能的编程语言,具有简洁的语法和强大的并发性能。Docker是一种容器化技术,可以将应用程序与其依赖项打包成一个独立的运行环境。Selenium是一个自动化测试工具,可以模拟用户在浏览器中的操作。而Chrome是一款流行的Web浏览器。通过结合使用这些工具,开发人员可以更方便地进行Web应用程序的开发和测试。在本文中,我们将深入探讨如何使用Golang Docker Selenium Chrome来加速开发过程,并分享一些实用技巧和最佳实践。
问题内容
我在 golang 中有一个 api,它尝试连接到在 docker 镜像中运行的 selenium 服务器:
package main import ( "fmt" "time" "github.com/tebeka/selenium" "github.com/tebeka/selenium/chrome" ) func main() { // configuração webdriver (simulador) caps := selenium.capabilities{ "browsername": "chrome", "browserversion": "114.0", "se:novncport": 7900, "se:vncenabled": true, } chromecaps := chrome.capabilities{ args: []string{ "--headless", }, } caps.addchrome(chromecaps) // iniciando o servidor, conectado ao eu webdriver que esta rodando wd, err := selenium.newremote(caps, "http://localhost:4444/wd/hub") if err != nil { fmt.printf("falha ao iniciar o servidor selenium: %s\n", err.error()) return } defer wd.quit() time.sleep(10 * time.second) // inicia a pagina inicial kk err = wd.get("https://www.csonline.com.br/") if err != nil { fmt.printf("falha ao abrir a página de login: %s\n", err.error()) return } time.sleep(10 * time.second) // selecionar o elemento por id usernamefield, err := wd.findelement(selenium.byid, "dfgd") if err != nil { fmt.printf("falha ao encontrar o campo de usuário: %s\n", err) return } //populando os campos, ai é só repetir os passos err = usernamefield.sendkeys("dfglkdf) if err != nil { fmt.printf("falha ao preencher o campo de usuário: %s\n", err.error()) } passwordfield, err := wd.findelement(selenium.byid, "dfg") if err != nil { fmt.printf("falha ao encontrar o campo de senha: %s\n", err.error()) } err = passwordfield.sendkeys("dlfknvkxjcn ") if err != nil { fmt.printf("falha ao preencher o campo de senha: %s\n", err.error()) } // envia o formulário de login loginbutton, err := wd.findelement(selenium.bycssselector, "#next") if err != nil { fmt.printf("falha ao encontrar o botão de login: %s\n", err.error()) } err = loginbutton.click() if err != nil { fmt.printf("falha ao clicar no botão de login: %s\n", err.error()) } fmt.println("sucess") //aguarda um tempo para realizar login time.sleep(10 * time.second) access, err := wd.findelement(selenium.byid, "btnselectlogin") if err != nil { fmt.printf("falha ao encontrar o botão de acesso: %s\n", err) } err = access.click() if err != nil { fmt.printf("falha ao clicar no botão de acesso: %s\n", err) } fmt.println("sucess") localstoragescript := `return localstorage.getitem("cs.token");` // execute o script no contexto do navegador localstoragedata, err := wd.executescript(localstoragescript, nil) if err != nil { fmt.println("falha ao obter dados do localstorage:", err) } // imprima os dados do localstorage fmt.println("bearer", localstoragedata) }
我在docker中安装了selenium,如下所示:
docker pull selenium/standalone-chrome
我运行此命令在 docker 中启动 selenium:
docker run -d -p 4444:4444 -v /dev/shm:/dev/shm selenium/standalone-chrome
运行命令时 go run main.go 我在 go 终端中收到此错误:
invalid session id: unable to execute request for an existing session: unable to find session with id: build info: version: '4.10.0', revision: 'c14d967899' system info: os.name: 'linux', os.arch: 'amd64', os.version: '5.10.16.3-microsoft-standard-wsl2', java.version: '11.0.19' driver info: driver.version: unknown
在 docker 中的 selenium 日志中我得到:
WARN [SeleniumSpanExporter$1.lambda$export$3] - {"traceId": "171e16c9402c8f85639418c7b458f388","eventTime": 1687963769139610379,"eventName": "exception","attributes": {"exception.message": "Unable to execute request for an existing session: Unable to find session with ID: \nBuild info: version: '4.10.0', revision: 'c14d967899'\nSystem info: os.name: 'Linux', os.arch: 'amd64', os.version: '5.10.16.3-microsoft-standard-WSL2', java.version: '11.0.19'\nDriver info: driver.version: unknown","exception.stacktrace": "org.openqa.selenium.NoSuchSessionException: Unable to find session with ID: \nBuild info: version: '4.10.0', revision: 'c14d967899'\nSystem info: os.name: 'Linux', os.arch: 'amd64', os.version: '5.10.16.3-microsoft-standard-WSL2', java.version: '11.0.19'\nDriver info: driver.version: unknown\n\tat org.openqa.selenium.grid.sessionmap.local.LocalSessionMap.get(LocalSessionMap.java:137)\n\tat org.openqa.selenium.grid.router.HandleSession.lambda$loadSessionId$4(HandleSession.java:172)\n\tat io.opentelemetry.context.Context.lambda$wrap$2(Context.java:224)\n\tat org.openqa.selenium.grid.router.HandleSession.execute(HandleSession.java:125)\n\tat org.openqa.selenium.remote.http.Route$PredicatedRoute.handle(Route.java:384)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.remote.http.Route$CombinedRoute.handle(Route.java:347)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.grid.router.Router.execute(Router.java:87)\n\tat org.openqa.selenium.grid.web.EnsureSpecCompliantResponseHeaders.lambda$apply$0(EnsureSpecCompliantResponseHeaders.java:34)\n\tat org.openqa.selenium.remote.http.Filter$1.execute(Filter.java:63)\n\tat org.openqa.selenium.remote.http.Route$CombinedRoute.handle(Route.java:347)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.remote.http.Route$NestedRoute.handle(Route.java:271)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.remote.http.Route$CombinedRoute.handle(Route.java:347)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.remote.http.Route$CombinedRoute.handle(Route.java:347)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.remote.http.Route$CombinedRoute.handle(Route.java:347)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.remote.http.Route$CombinedRoute.handle(Route.java:347)\n\tat org.openqa.selenium.remote.http.Route.execute(Route.java:69)\n\tat org.openqa.selenium.remote.AddWebDriverSpecHeaders.lambda$apply$0(AddWebDriverSpecHeaders.java:35)\n\tat org.openqa.selenium.remote.ErrorFilter.lambda$apply$0(ErrorFilter.java:44)\n\tat org.openqa.selenium.remote.http.Filter$1.execute(Filter.java:63)\n\tat org.openqa.selenium.remote.ErrorFilter.lambda$apply$0(ErrorFilter.java:44)\n\tat org.openqa.selenium.remote.http.Filter$1.execute(Filter.java:63)\n\tat org.openqa.selenium.netty.server.SeleniumHandler.lambda$channelRead0$0(SeleniumHandler.java:44)\n\tat java.base\u002fjava.util.concurrent.Executors$RunnableAdapter.call(Executors.java:515)\n\tat java.base\u002fjava.util.concurrent.FutureTask.run(FutureTask.java:264)\n\tat java.base\u002fjava.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1128)\n\tat java.base\u002fjava.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:628)\n\tat java.base\u002fjava.lang.Thread.run(Thread.java:829)\n","exception.type": "org.openqa.selenium.NoSuchSessionException","http.flavor": 1,"http.handler_class": "org.openqa.selenium.grid.router.HandleSession","http.host": "localhost:4444","http.method": "POST","http.request_content_length": "38","http.scheme": "HTTP","http.target": "\u002fsession\u002f\u002furl","http.user_agent": "Go-http-client\u002f1.1","session.id": ""}}
为什么会发生这种情况?有人可以提供帮助吗?
解决方法
要使您的演示与 selenium/standalone-chrome:latest
(目前为 selenium/standalone-chrome:4.10.0
)一起使用,您需要将 w3c
设置为 true
:
chromeCaps := chrome.Capabilities{ Args: []string{ "--headless", }, W3C: true, }
如果你仔细查看容器的日志,你应该会看到类似这样的内容:
警告 [seleniumspanexporter$1.lambda$export$1] - org.openqa.selenium.sessionnotcreatedexception:无法启动新会话。与驱动程序服务创建会话时出错。停止驱动程序服务:无法启动新会话。握手响应与任何支持的协议不匹配。响应负载:{"sessionid":"4ef27eb4d2fbb1ebfe5cf2fb51df731b","status":33,"value":{"message":"会话未创建:缺少或无效的功能\n(驱动程序信息:chromedriver =114.0.5735.90 (386bc09e8f4f2e025eddae123f36f6263096ae49-refs/branch-heads/5735@{#1052}),platform=linux 5.19.0-43-通用 x86_64)"}}
这可能是由删除 json wire 协议支持引入的重大更改引起的,在 selenium 4.9.0
中提供。
我从演示中删除了 "browserversion": "114.0"
,然后针对 selenium/standalone-chrome:4.8.3
和 selenium/standalone-chrome:4.9.0
进行了测试。结果是,它适用于 4.8.3
,但不适用于 4.9.0
。这至少证明 4.9.0
引入了重大更改。
以上是Golang Docker Selenium Chrome的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

熱門話題
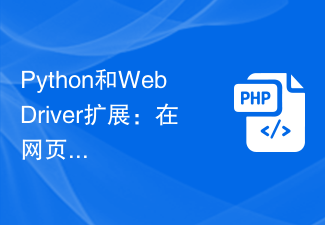
Python和WebDriver擴充:在網頁中模擬滑鼠滾輪操作引言:隨著網頁互動設計的不斷發展,模擬使用者操作在自動化測試中變得越來越重要。在一些網頁上,滑鼠滾輪的使用已經成為了常見的操作之一。然而,對於使用Python編寫自動化測試腳本的開發人員來說,如何在WebDriver中模擬滑鼠滾輪操作可能會成為一個挑戰。本文將介紹一種使用Python和WebDriv
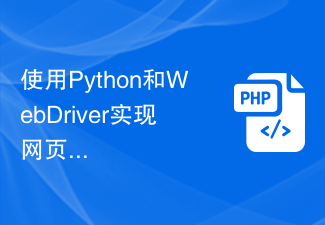
使用Python和WebDriver實作網頁截圖並儲存為PDF文件摘要:在Web開發和測試過程中,經常需要對網頁進行截圖以便進行分析、記錄和報告。本文將介紹如何使用Python和WebDriver來實現網頁截圖,並將截圖儲存為PDF文件,以方便分享和存檔。一、安裝與設定SeleniumWebDriver:安裝Python:造訪Python官網(https:
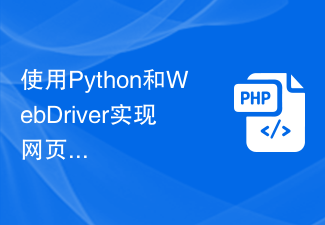
使用Python和WebDriver實現網頁自動填寫驗證碼隨著網路的發展,越來越多的網站在用戶註冊、登入等操作中引入了驗證碼機制,以提高安全性和防止自動化攻擊。然而,手動輸入驗證碼不僅麻煩,還增加了使用者體驗的複雜度。那麼,有沒有一種方法能夠自動填入驗證碼呢?答案是肯定的。本文將介紹如何使用Python和WebDriver實作網頁自動填入驗證碼的方法。首先,我
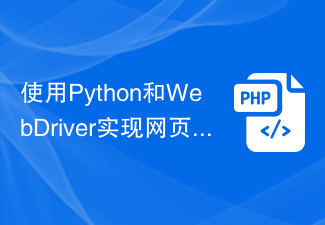
使用Python和WebDriver實現網頁自動刷新引言:在日常的網頁瀏覽中,我們常常會遇到需要頻繁刷新網頁的場景,例如監控即時資料、自動刷新動態頁面等。手動刷新網頁會浪費大量的時間和精力,因此我們可以使用Python和WebDriver來實現自動刷新網頁的功能,並提高我們的工作效率。一、安裝和配置環境在開始之前,我們需要安裝和配置對應的環境。安裝Python
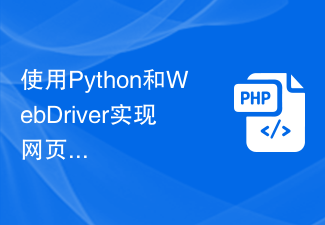
使用Python和WebDriver實現網頁自動填充表格資料自動化測試是軟體開發過程中重要的一環,其中之一是網頁表單的自動填充。對於開發人員來說,手動填寫表單是一個枯燥且容易出錯的過程。而使用Python和WebDriver,在自動測試過程中實現自動填入表格數據,能夠減少人工重複勞動,並提高測試效率。在這篇文章中,我將介紹如何使用Python的Selenium
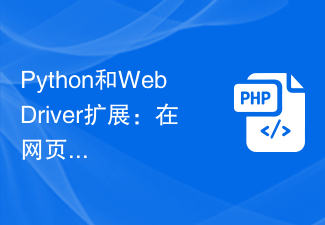
Python和WebDriver擴充:在網頁中模擬滑鼠右鍵點擊在使用Python和WebDriver進行網頁自動化測試時,我們經常需要模擬使用者的滑鼠行為,例如點擊、拖曳和右鍵選單等操作。 WebDriver會提供一些基本的滑鼠行動函數,如click、drag_and_drop等,但卻沒有直接提供模擬滑鼠右鍵點擊的函數。本文將介紹如何使用Python和WebD
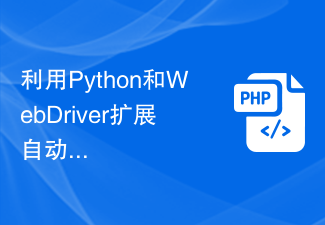
利用Python和WebDriver擴充自動化處理網頁的拖放操作在實際的Web應用中,拖放(DragandDrop)是一個常見的互動操作,它可以增強使用者的體驗和便利性。對測試人員而言,自動化處理網頁的拖放操作是一項重要且常見的任務。本文將介紹如何利用Python和WebDriver擴充功能自動化處理網頁的拖放作業。一、準備工作在開始前,我們需要安裝Pyt
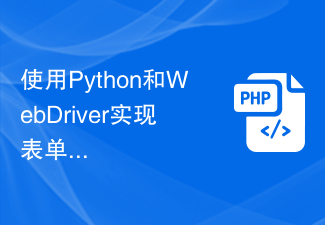
使用Python和WebDriver實作表單自動填寫功能在日常的網站瀏覽中,我們經常會遇到需要填寫表單的情況。當我們需要頻繁填寫相同或類似的表單時,手動填寫顯得很繁瑣而且耗時。幸運的是,我們可以藉助Python和WebDriver來實現自動填寫表單的功能,提高我們的工作效率。首先,我們要安裝selenium庫。 Selenium是一個自動化測試工具,可以模擬
