C程式和子進程
我寫了這個簡單的 c 程式來解釋具有相同特徵的更困難的問題。
#include <stdio.h> int main(int argc, char *argv[]) { int n; while (1){ scanf("%d", &n); printf("%d\n", n); } return 0; }
它按預期工作。
我還編寫了一個子進程腳本來與該程式互動:
from subprocess import popen, pipe, stdout process = popen("./a.out", stdin=pipe, stdout=pipe, stderr=stdout) # sending a byte process.stdin.write(b'3') process.stdin.flush() # reading the echo of the number print(process.stdout.readline()) process.stdin.close()
問題是,如果我執行 python 腳本,執行會在 readline()
上凍結。事實上,如果我中斷腳本,我會得到:
/tmp » python script.py ^ctraceback (most recent call last): file "/tmp/script.py", line 10, in <module> print(process.stdout.readline()) ^^^^^^^^^^^^^^^^^^^^^^^^^ keyboardinterrupt
如果我更改我的 python 腳本:
from subprocess import popen, pipe, stdout process = popen("./a.out", stdin=pipe, stdout=pipe, stderr=stdout) with process.stdin as pipe: pipe.write(b"3") pipe.flush() # reading the echo of the number print(process.stdout.readline()) # sending another num: pipe.write(b"4") pipe.flush() process.stdin.close()
我得到了這個輸出:
» python script.py b'3\n' Traceback (most recent call last): File "/tmp/script.py", line 13, in <module> pipe.write(b"4") ValueError: write to closed file
這意味著第一個輸入已正確發送,並且讀取已完成。
我真的找不到解釋這種行為的東西;有人可以幫助我理解嗎? 提前致謝
[編輯]:由於有很多要點需要澄清,所以我添加了此編輯。我正在使用 rop
技術進行緩衝區溢出漏洞利用的培訓,並且我正在編寫 python 腳本來實現這一目標。為了利用這個漏洞,由於aslr,我需要發現libc
位址並使程式重新啟動而不終止。由於腳本將在目標機器上執行,我不知道哪些庫可用,那麼我將使用 subprocess,因為它是內建於 python 中的。不詳細說明,攻擊在第一個scanf
上發送一系列位元組,目的是洩漏libc
基底位址並重新啟動程式;然後發送第二個有效負載以獲得一個shell,我將透過它以互動模式進行通訊。
這就是為什麼:
- 我只能使用內建函式庫
- 我必須傳送位元組並且無法附加結尾
\n
:我的有效負載將無法對齊或可能導致失敗 - 我需要保持
stdin
打開 - 我無法更改 c 程式碼
正確答案
更改這些:
在 c 程式讀取的數字之間發送分隔符號。 scanf(3) 接受任何非數字位元組作為分隔符號。為了最簡單的緩衝,請從 python 發送換行符(例如
.write(b'42\n')
)。如果沒有分隔符,scanf(3) 將無限期地等待更多數字。每次寫入(c 和 python 中)後,刷新輸出。
這對我有用:
#include <stdio.h> int main(int argc, char *argv[]) { int n; while (1){ scanf("%d", &n); printf("%d\n", n); fflush(stdout); /* i've added this line only. */ } return 0; }
import subprocess p = subprocess.popen( ('./a.out',), stdin=subprocess.pipe, stdout=subprocess.pipe) try: print('a'); p.stdin.write(b'42 '); p.stdin.flush() print('b'); print(repr(p.stdout.readline())); print('c'); p.stdin.write(b'43\n'); p.stdin.flush() print('d'); print(repr(p.stdout.readline())); finally: print('e'); print(p.kill())
原始 c 程式在終端機視窗中交互運行時能夠正常工作的原因是,在 c 中,當將換行符 (\n
) 寫入終端時,輸出會自動刷新。因此 printf("%d\n", n);
最後會隱含執行 fflush(stdout);
。
當使用 subprocess
從 python 運行時,原始 c 程式無法工作的原因是它將輸出寫入管道(而不是終端),並且沒有自動刷新到管道。發生的情況是,python 程式正在等待字節,而c 程式不會將這些位元組寫入管道,但它正在等待更多位元組(在下一個scanf
中),因此兩個程式都在無限期地等待對方。 (但是,在輸出幾 kib(通常為 8192 位元組)後,將會出現部分自動刷新。但是單個十進制數太短,無法觸發該操作。)
如果無法更改 c 程序,那麼您應該使用終端設備而不是管道來在 c 和 python 程式之間進行通訊。 pty
python 模組可以建立終端設備,這對我來說適用於你的原始 c 程式:
import os, pty, subprocess master_fd, slave_fd = pty.openpty() p = subprocess.popen( ('./a.out',), stdin=slave_fd, stdout=slave_fd, preexec_fn=lambda: os.close(master_fd)) try: os.close(slave_fd) master = os.fdopen(master_fd, 'rb+', buffering=0) print('a'); master.write(b'42\n'); master.flush() print('b'); print(repr(master.readline())); print('c'); master.write(b'43\n'); master.flush() print('d'); print(repr(master.readline())); finally: print('e'); print(p.kill())
如果你不想從python發送換行符,這裡有一個沒有換行符的解決方案,它對我有用:
import os, pty, subprocess, termios master_fd, slave_fd = pty.openpty() ts = termios.tcgetattr(master_fd) ts[3] &= ~(termios.ICANON | termios.ECHO) termios.tcsetattr(master_fd, termios.TCSANOW, ts) p = subprocess.Popen( ('./a.out',), stdin=slave_fd, stdout=slave_fd, preexec_fn=lambda: os.close(master_fd)) try: os.close(slave_fd) master = os.fdopen(master_fd, 'rb+', buffering=0) print('A'); master.write(b'42 '); master.flush() print('B'); print(repr(master.readline())); print('C'); master.write(b'43\t'); master.flush() print('D'); print(repr(master.readline())); finally: print('E'); print(p.kill())
以上是C程式和子進程的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
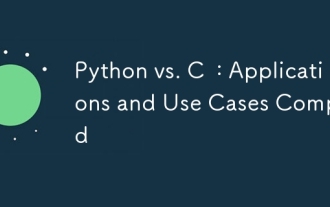
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
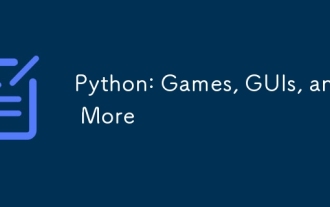
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
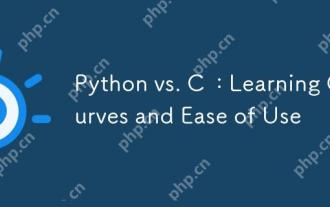
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
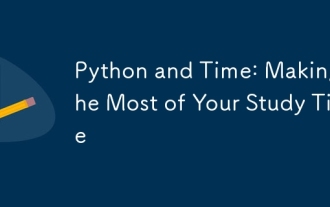
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
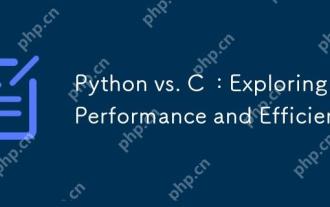
Python在開發效率上優於C ,但C 在執行性能上更高。 1.Python的簡潔語法和豐富庫提高開發效率。 2.C 的編譯型特性和硬件控制提升執行性能。選擇時需根據項目需求權衡開發速度與執行效率。
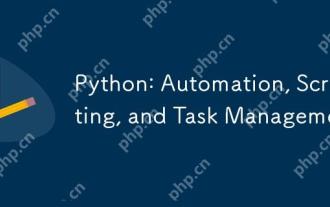
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
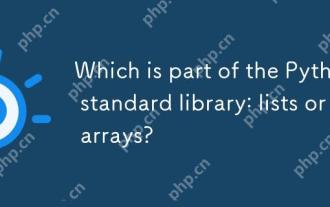
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
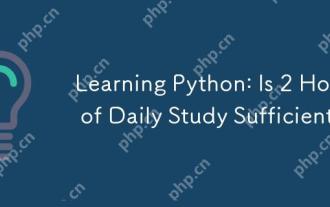
每天學習Python兩個小時是否足夠?這取決於你的目標和學習方法。 1)制定清晰的學習計劃,2)選擇合適的學習資源和方法,3)動手實踐和復習鞏固,可以在這段時間內逐步掌握Python的基本知識和高級功能。
