Go語言開發的文字編輯器
Golang實作的檔案編輯器
概述:
在日常程式設計工作中,經常需要對檔案內容進行編輯、尋找、取代等操作。為了提高效率,可以使用Golang語言實作一個簡單的檔案編輯器,能夠實現常見的檔案操作功能。本文將介紹如何使用Golang編寫一個基於命令列的檔案編輯器,並提供具體的程式碼範例。
功能:
- 開啟檔案:使用者可以輸入檔案路徑,編輯器將開啟該檔案並顯示檔案內容。
- 查找:使用者輸入尋找關鍵字,編輯器會在檔案中尋找包含該關鍵字的行並顯示出來。
- 替換:使用者可以輸入要替換的關鍵字和替換後的內容,編輯器將取代檔案中所有包含該關鍵字的內容。
- 儲存:使用者可以儲存對檔案的修改。
- 退出:使用者可以退出編輯器。
具體實作:
以下是一個簡單的檔案編輯器的Golang程式碼範例:
package main import ( "bufio" "fmt" "io/ioutil" "os" "strings" ) func openFile(filePath string) string { file, err := ioutil.ReadFile(filePath) if err != nil { return "" } return string(file) } func searchFile(content string, keyword string) { scanner := bufio.NewScanner(strings.NewReader(content)) for scanner.Scan() { line := scanner.Text() if strings.Contains(line, keyword) { fmt.Println(line) } } } func replaceFile(filePath string, old string, new string) { file, err := ioutil.ReadFile(filePath) if err != nil { return } content := string(file) newContent := strings.ReplaceAll(content, old, new) err = ioutil.WriteFile(filePath, []byte(newContent), 0644) if err != nil { return } } func main() { var filePath, keyword, old, new string fmt.Println("请输入文件路径:") fmt.Scanln(&filePath) content := openFile(filePath) if content == "" { fmt.Println("文件打开失败") return } fmt.Println("请输入要查找的关键字:") fmt.Scanln(&keyword) searchFile(content, keyword) fmt.Println("请输入要替换的关键字:") fmt.Scanln(&old) fmt.Println("请输入替换后的内容:") fmt.Scanln(&new) replaceFile(filePath, old, new) fmt.Println("文件编辑完成,是否保存?(Y/N)") var choice string fmt.Scanln(&choice) if strings.ToUpper(choice) == "Y" { fmt.Println("文件保存成功") } else { fmt.Println("文件未保存") } }
以上程式碼實作了一個簡單的檔案編輯器,包括開啟檔案、尋找內容、取代內容、儲存檔案等功能。使用者可以根據需要擴展更多功能,如撤銷操作、編輯多個文件等。透過這個實例,希望讀者可以掌握如何使用Golang語言編寫檔案編輯器的基本想法和技巧。
以上是Go語言開發的文字編輯器的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
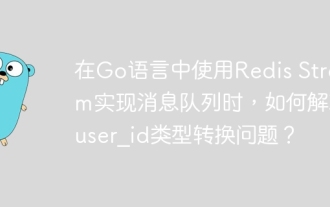
Go語言中使用RedisStream實現消息隊列時類型轉換問題在使用Go語言與Redis...
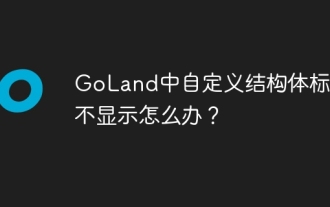
GoLand中自定義結構體標籤不顯示怎麼辦?在使用GoLand進行Go語言開發時,很多開發者會遇到自定義結構體標籤在�...
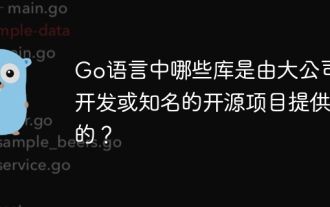
Go語言中哪些庫是大公司開發或知名開源項目?在使用Go語言進行編程時,開發者常常會遇到一些常見的需求,�...
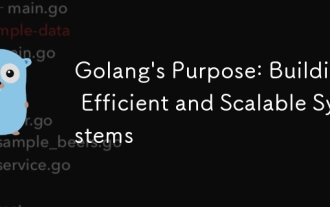
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
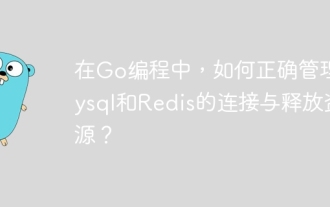
Go編程中的資源管理:Mysql和Redis的連接與釋放在學習Go編程過程中,如何正確管理資源,特別是與數據庫和緩存�...
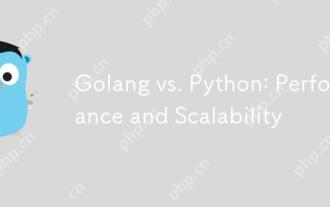
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
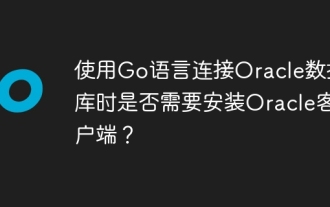
使用Go語言連接Oracle數據庫時是否需要安裝Oracle客戶端?在使用Go語言開發時,連接Oracle數據庫是一個常見需求�...
