PHP會傳回一個字串在另一個字串中最後一次出現位置開始到結尾的字串
在PHP中,如果需要傳回一個字串在另一個字串中最後一次出現位置開始到末尾的子字串,可以使用內建函數strrchr()來實現。 strrchr()函數會在目標字串中尋找最後一次出現的指定字串,並傳回該字串到結尾的部分。使用函數可以方便地截取所需的字串段落,提高程式碼的效率和可讀性。下面我們將詳細介紹如何在PHP中使用strrchr()函數來實現這項功能。
PHP 中取得字串最後一次出現的位置到最後的字串
問題: 如何使用 php 取得一個字串在另一個字串中最後一次出現的位置開始到末尾的子字串?
解決方案:
#PHP 中有兩種主要方法可以取得字串最後一次出現的位置到最後的子字串:
1. 使用 strrpos() 函數
strrpos()
函數傳回一個字串在另一個字串中最後一次出現的位置。我們可以使用此位置和 substr()
函數來提取子字串:
<?php $string = "Hello World, welcome to the world of PHP!"; $substring = "world"; // 取得 "world" 在 $string 中最後一次出現的位置 $pos = strrpos($string, $substring); // 如果字串中存在 "world",則提取子字串 if ($pos !== false) { $subString = substr($string, $pos); echo $subString; // 輸出 "world of PHP!" } else { echo "Substring not found."; } ?>
2. 使用 preg_match() 函數
#preg_match()
函數可以執行正規表示式搜尋。我們可以使用正規表示式來匹配字串最後一次出現的子字串:
<?php $string = "Hello World, welcome to the world of PHP!"; $pattern = "/.*$substring$/"; // 執行正規表示式搜尋並取得匹配項 preg_match($pattern, $string, $matches); // 如果找到匹配項,則提取子字串 if (isset($matches[0])) { $subString = $matches[0]; echo $subString; // 輸出 "world of PHP!" } else { echo "Substring not found."; } ?>
效能考量:
#strrpos()
函數的效能通常優於 preg_match()
函數,因為它不需要執行正規表示式匹配。但是,preg_match()
函數提供了更靈活的搜尋選項。
最佳實踐:
- #確保子字串在字串中存在,否則可能會產生意外結果。
- 使用
===
或!==
運算子嚴格比較$pos
的值,以避免誤將0
視為false
。 - 考慮使用
preg_replace()
函數取代字串中的子字串。
其他方法:
#除了上述方法外,還有其他方法可以取得字串最後一次出現的位置到末尾的子字串,例如:
- 使用字串切片(
$string[$pos:]
) - 使用
array_slice()
函數 - 使用自訂函數或第三方函式庫
以上是PHP會傳回一個字串在另一個字串中最後一次出現位置開始到結尾的字串的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
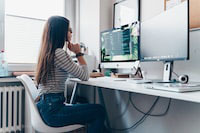
這篇文章將為大家詳細講解有關PHP將行格式化為CSV並寫入文件指針,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。將行格式化為CSV並寫入檔案指標步驟1:開啟檔案指標$file=fopen("path/to/file.csv","w");步驟2:將行轉換為CSV字串使用fputcsv( )函數將行轉換為CSV字串。此函數接受以下參數:$file:檔案指標$fields:作為陣列的CSV欄位$delimiter:欄位分隔符號(可選)$enclosure:欄位引號(
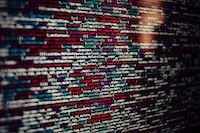
這篇文章將為大家詳細講解有關PHP建立一個具有唯一文件名的文件,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。在PHP中建立唯一檔案名稱的檔案簡介在php中建立具有唯一檔案名稱的檔案對於組織和管理檔案系統至關重要。唯一文件名稱可確保不會覆蓋現有文件,並便於尋找和檢索特定文件。本指南將介紹在PHP中產生唯一檔案名稱的幾種方法。方法1:使用uniqid()函數uniqid()函數產生一個基於當前時間和微秒的唯一字串。此字串可以作為檔案名稱的基礎。
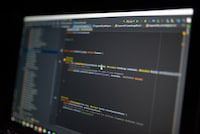
這篇文章將為大家詳細講解有關PHP改變當前的umask,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP更改目前的umask概述umask是一個用於設定新建立的檔案和目錄的預設檔案權限的php函數。它接受一個參數,這是一個八進制數字,表示要阻止的權限。例如,要阻止對新建立的檔案進行寫入權限,可以使用002。更改umask的方法有兩種方法可以更改PHP中的目前umask:使用umask()函數:umask()函數直接變更目前umask。其語法為:intumas
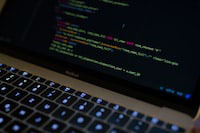
這篇文章將為大家詳細講解有關PHP返回一個鍵值翻轉後的數組,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP鍵值翻轉數組鍵值翻轉是一種對數組進行的操作,它將數組中的鍵和值進行交換,產生一個新的數組,其中原始鍵作為值,原始值作為鍵。實作方法在php中,可以透過以下方法對陣列進行鍵值翻轉:array_flip()函數:array_flip()函數專門用於鍵值翻轉操作。它接收一個數組作為參數,並傳回一個新的數組,其中鍵和值已交換。 $original_array=[
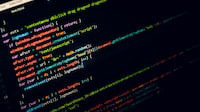
這篇文章將為大家詳細講解有關PHP計算文件的MD5散列,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP計算檔案的MD5雜湊MD5(MessageDigest5)是一種單向加密演算法,可將任意長度的訊息轉換為固定長度的128位元雜湊值。它廣泛用於確保文件完整性、驗證資料真實性和建立數位簽章。在PHP中計算檔案的MD5雜湊php提供了多種方法來計算檔案的MD5雜湊:使用md5_file()函數md5_file()函數直接計算檔案的MD5雜湊值,傳回一個32個字元的
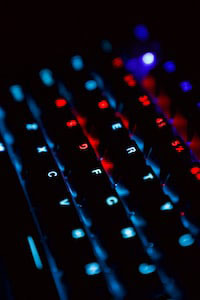
這篇文章將為大家詳細講解有關PHP將文件截斷到給定的長度,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP檔案截斷簡介php中的file_put_contents()函數可用來將檔案截斷到指定長度。截斷是指刪除檔案末端的部分內容,從而縮短檔案長度。語法file_put_contents($filename,$data,SEEK_SET,$offset);$filename:要截斷的檔案路徑。 $data:要寫入檔案的空字串。 SEEK_SET:指定為檔案開始處

這篇文章將為大家詳細講解有關PHP返回上一個Mysql操作中的錯誤訊息的數字編碼,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。利用PHP回傳MySQL錯誤訊息數字編碼引言在處理mysql查詢時,可能會遇到錯誤。為了有效處理這些錯誤,了解錯誤訊息數字編碼至關重要。本文將指導您使用php取得Mysql錯誤訊息數字編碼。取得錯誤訊息數字編碼的方法1.mysqli_errno()mysqli_errno()函數傳回目前MySQL連線的最近錯誤號碼。文法如下:$erro
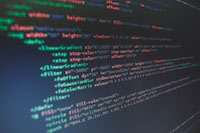
這篇文章將為大家詳細講解有關PHP判斷某個數組中是否存在指定的key,小編覺得挺實用的,因此分享給大家做個參考,希望大家閱讀完這篇文章後可以有所收穫。 PHP判斷某個陣列中是否存在指定的key:在php中,判斷某個陣列中是否存在指定的key的方法有多種:1.使用isset()函數:isset($array["key"])此函數傳回布林值,如果指定的key存在,則傳回true,否則傳回false。 2.使用array_key_exists()函數:array_key_exists("key",$arr
