vue怎麼使用後端提供的接口
在 Vue 中使用后端接口
在 Vue.js 应用中使用后端提供的接口可以让你与服务器通信,获取和更新数据。本文将介绍如何在 Vue 中使用后端接口。
1. 安装 Axios
首先,你需要安装 Axios 库,这是一个用于发起 HTTP 请求的 JavaScript 库。在终端中执行以下命令:
<code>npm install axios</code>
然后,在你的 Vue.js 文件中导入 Axios:
import axios from 'axios'
2. 创建请求
要创建 HTTP 请求,请使用 axios
对象:
axios.get('api/todos') .then(response => { // 处理成功的响应 }) .catch(error => { // 处理请求错误 })
get
方法用于发送 GET 请求,post
方法用于发送 POST 请求,以此类推。
3.传递数据
要传递数据到后端,请使用 data
选项:
axios.post('api/todos', { title: '学习 Vue.js' }) .then(response => { // 处理成功的响应 }) .catch(error => { // 处理请求错误 })
4. 处理响应
成功响应中包含 data
属性,其中包含后端返回的数据。
axios.get('api/todos') .then(response => { const todos = response.data; // 使用 todos 数据 }) .catch(error => { // 处理请求错误 })
5. 使用 Vuex
Vuex 是一种状态管理库,可以帮助你在 Vue.js 应用中管理和共享数据。你可以使用 Vuex 来管理从后端获取的数据,并通过组件访问它。
要使用 Vuex,你需要创建一个 Vuex 存储:
import Vuex from 'vuex' import { createStore } from 'vuex' const store = createStore({ state: { todos: [] }, actions: { getTodos({ commit }) { axios.get('api/todos') .then(response => { commit('setTodos', response.data) }) .catch(error => { // 处理请求错误 }) } }, mutations: { setTodos(state, todos) { state.todos = todos } } })
然后,你可以在组件中使用 mapState
和 mapActions
辅助函数来访问 Vuex 存储:
import { mapState, mapActions } from 'vuex' export default { computed: { ...mapState(['todos']) }, methods: { ...mapActions(['getTodos']) } }
以上是vue怎麼使用後端提供的接口的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
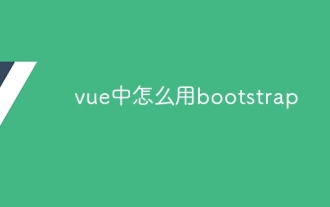
在 Vue.js 中使用 Bootstrap 分為五個步驟:安裝 Bootstrap。在 main.js 中導入 Bootstrap。直接在模板中使用 Bootstrap 組件。可選:自定義樣式。可選:使用插件。
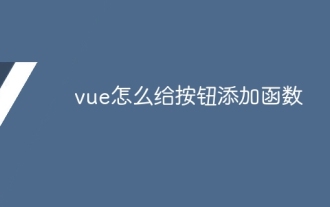
可以通過以下步驟為 Vue 按鈕添加函數:將 HTML 模板中的按鈕綁定到一個方法。在 Vue 實例中定義該方法並編寫函數邏輯。
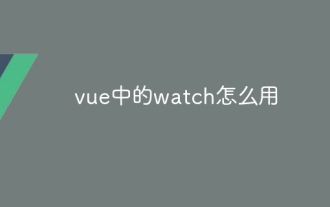
Vue.js 中的 watch 選項允許開發者監聽特定數據的變化。當數據發生變化時,watch 會觸發一個回調函數,用於執行更新視圖或其他任務。其配置選項包括 immediate,用於指定是否立即執行回調,以及 deep,用於指定是否遞歸監聽對像或數組的更改。
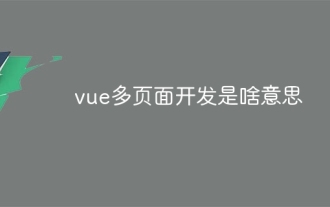
Vue 多頁面開發是一種使用 Vue.js 框架構建應用程序的方法,其中應用程序被劃分為獨立的頁面:代碼維護性:將應用程序拆分為多個頁面可以使代碼更易於管理和維護。模塊化:每個頁面都可以作為獨立的模塊,便於重用和替換。路由簡單:頁面之間的導航可以通過簡單的路由配置來管理。 SEO 優化:每個頁面都有自己的 URL,這有助於搜索引擎優化。
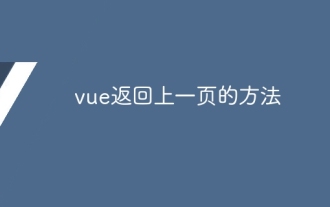
Vue.js 返回上一頁有四種方法:$router.go(-1)$router.back()使用 <router-link to="/"> 組件window.history.back(),方法選擇取決於場景。
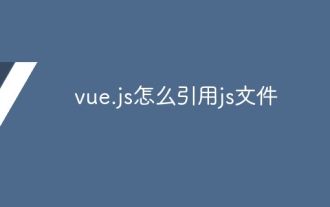
在 Vue.js 中引用 JS 文件的方法有三種:直接使用 <script> 標籤指定路徑;利用 mounted() 生命週期鉤子動態導入;通過 Vuex 狀態管理庫進行導入。
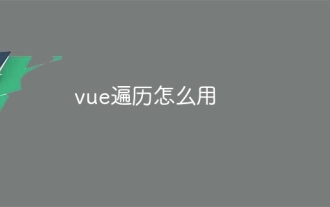
Vue.js 遍歷數組和對像有三種常見方法:v-for 指令用於遍歷每個元素並渲染模板;v-bind 指令可與 v-for 一起使用,為每個元素動態設置屬性值;.map 方法可將數組元素轉換為新數組。
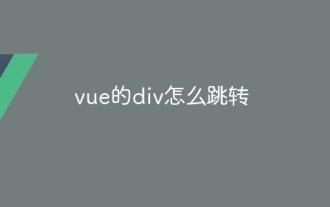
Vue 中 div 元素跳轉的方法有兩種:使用 Vue Router,添加 router-link 組件。添加 @click 事件監聽器,調用 this.$router.push() 方法跳轉。
