PHP 設計模式單元測試最佳實踐
PHP 設計模式單元測試最佳實務:隔離相依性: 使用依賴注入或 mock 對象,避免與外部元件的耦合。測試邊界條件: 考慮異常、錯誤處理和邊緣用例,確保設計模式在各種情況下都能正確運作。涵蓋多種場景: 測試不同變體和實現,以涵蓋所有可能的行為。遵循 SOLID 原則: 應用單一職責、鬆散耦合等原則,編寫可測試、可維護的程式碼。
PHP 設計模式單元測試最佳實踐
在編寫單元測試時,良好的實踐對於確保程式碼的可靠性和可維護性至關重要。對於 PHP 中的複雜設計模式而言,單元測試尤其關鍵。本文將介紹適用於 PHP 設計模式單元測試的最佳實踐,並透過實戰案例進行說明。
隔離依賴項
理想情況下,單元測試應針對單一類別或方法進行,而無需依賴其他元件。對於設計模式,這可能是一項艱鉅的任務,因為它們通常依賴多個類別和介面之間的相互作用。
使用依賴注入框架或 mock 物件可以隔離依賴項。例如,使用[PHPUnit\_MockObject](https://phpunit.readthedocs.io/en/latest/fixtures.html#creating-mocks) 建立一個mock 物件來取代外部依賴項:
use PHPUnit\Framework\TestCase; use PHPUnit\Framework\MockObject\MockObject; class MyClassTest extends TestCase { /** @var MockObject */ private $mockDependency; protected function setUp(): void { $this->mockDependency = $this->createMock(IDependency::class); } }
測試邊界條件
設計模式通常處理複雜的行為和狀態管理。單元測試應考慮所有可能的邊界條件,包括異常、錯誤處理和邊緣用例。
例如,測試觀察者模式的 attach
方法時,應確保僅附加有效的訂閱者。也可以測試當訂閱者嘗試附加到無效主題時的行為:
public function testAttachInvalidSubject() { $observer = new MyObserver(); $mode = 'invalid_mode'; $this->expectException(InvalidArgumentException::class); $observer->attach(new InvalidSubject(), $mode); }
覆蓋多種場景
設計模式通常具有多種變體和實作。單元測試應涵蓋所有這些不同的場景。
例如,測試策略模式的 execute
方法時,應考慮不同策略類別的行為。也可以測試將不同的策略類別傳遞給執行方法時發生的情況:
public function testExecuteDifferentStrategies() { $context = new Context(); $strategy1 = new Strategy1(); $strategy2 = new Strategy2(); $this->assertEquals('Strategy1 result', $context->execute($strategy1)); $this->assertEquals('Strategy2 result', $context->execute($strategy2)); }
遵循SOLID 原則
SOLID 原則是物件導向程式設計的五個準則,可以幫助編寫可測試、可維護的程式碼。對於設計模式的單元測試尤其重要。
例如,遵循單一職責原則來確保每個測試方法僅測試一個特定功能。另外,遵守鬆散耦合原則來確保測試與生產代碼的依賴關係保持在最低限度。
實戰案例
單例模式
class SingletonTest extends TestCase { public function testSingletonIsUnique() { $instance1 = Singleton::getInstance(); $instance2 = Singleton::getInstance(); $this->assertSame($instance1, $instance2); } public function testSingletonLazilyInitialized() { $this->assertNull(Singleton::getInstance()); Singleton::getInstance()->setValue('test'); $this->assertEquals('test', Singleton::getInstance()->getValue()); } }
觀察者模式
class ObserverTest extends TestCase { public function testObserverIsNotified() { $subject = new Subject(); $observer = new Observer(); $subject->attach($observer); $subject->setState('new state'); $this->assertEquals('new state', $observer->getState()); } public function testObserverIsDetached() { $subject = new Subject(); $observer = new Observer(); $subject->attach($observer); $subject->detach($observer); $subject->setState('new state'); $this->assertNotEquals('new state', $observer->getState()); } }
以上是PHP 設計模式單元測試最佳實踐的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
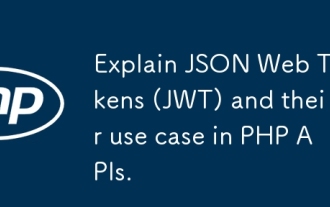
JWT是一種基於JSON的開放標準,用於在各方之間安全地傳輸信息,主要用於身份驗證和信息交換。 1.JWT由Header、Payload和Signature三部分組成。 2.JWT的工作原理包括生成JWT、驗證JWT和解析Payload三個步驟。 3.在PHP中使用JWT進行身份驗證時,可以生成和驗證JWT,並在高級用法中包含用戶角色和權限信息。 4.常見錯誤包括簽名驗證失敗、令牌過期和Payload過大,調試技巧包括使用調試工具和日誌記錄。 5.性能優化和最佳實踐包括使用合適的簽名算法、合理設置有效期、
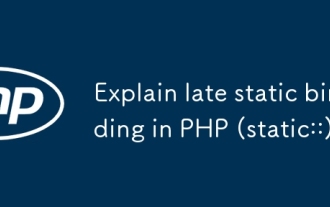
靜態綁定(static::)在PHP中實現晚期靜態綁定(LSB),允許在靜態上下文中引用調用類而非定義類。 1)解析過程在運行時進行,2)在繼承關係中向上查找調用類,3)可能帶來性能開銷。

PHP的魔法方法有哪些? PHP的魔法方法包括:1.\_\_construct,用於初始化對象;2.\_\_destruct,用於清理資源;3.\_\_call,處理不存在的方法調用;4.\_\_get,實現動態屬性訪問;5.\_\_set,實現動態屬性設置。這些方法在特定情況下自動調用,提升代碼的靈活性和效率。
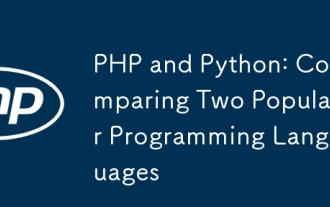
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
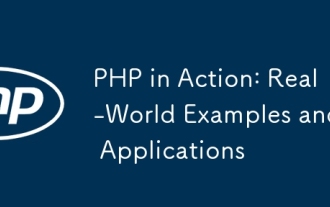
PHP在電子商務、內容管理系統和API開發中廣泛應用。 1)電子商務:用於購物車功能和支付處理。 2)內容管理系統:用於動態內容生成和用戶管理。 3)API開發:用於RESTfulAPI開發和API安全性。通過性能優化和最佳實踐,PHP應用的效率和可維護性得以提升。
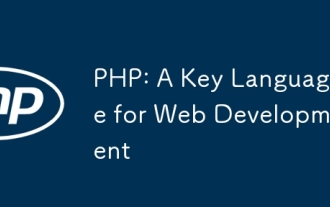
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
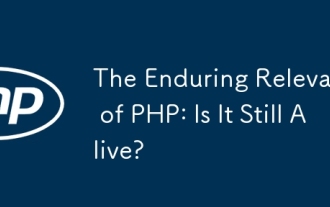
PHP仍然具有活力,其在現代編程領域中依然佔據重要地位。 1)PHP的簡單易學和強大社區支持使其在Web開發中廣泛應用;2)其靈活性和穩定性使其在處理Web表單、數據庫操作和文件處理等方面表現出色;3)PHP不斷進化和優化,適用於初學者和經驗豐富的開發者。
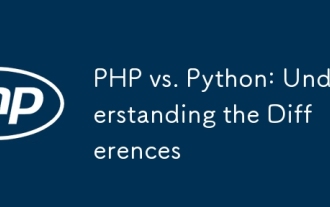
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
