PHP 演算法實作中常見的誤區
在 PHP 演算法實作中,常見的誤解包括:類型轉換不當、演算法選擇不正確、邊界條件處理不佳和效率最佳化忽視。解決方法包括:明確類型轉換、選擇合適的演算法、檢查邊界條件和利用最佳化技術。透過避免這些誤區,可以編寫高效且準確的演算法。
PHP演算法實作中常見的誤解
PHP是一種廣泛使用的程式語言,特別適用於Web開發。然而,在實作演算法時,PHP開發者可能會遇到一些常見的誤解。本文將探討這些誤區並提供解決方法,以協助您撰寫高效且準確的演算法。
誤區1:沒有考慮資料型別
PHP不支援強型別系統,這表示它可以自動將變數從一種資料型別轉換為另一種資料類型。雖然這可以提供靈活性,但它也可能導致演算法錯誤。例如,比較整數和字串可能會產生意外的結果:
$num = 10; $str = "10"; var_dump($num == $str); // 输出:true
解決方案:始終明確地轉換資料類型,以確保在演算法中進行正確的比較和操作。
誤解2:使用不正確的演算法
PHP提供了各種資料結構和演算法,但是選擇正確的演算法對於實現高效的解決方案至關重要。例如,使用線性搜尋演算法來尋找大型陣列中的元素可能非常低效:
function linearSearch($arr, $target) { for ($i = 0; $i < count($arr); $i++) { if ($arr[$i] === $target) { return $i; } } return -1; }
#解決方案:考慮資料的特性和演算法的複雜度,以選擇最合適的演算法.
誤解3:忽略邊界條件
演算法實作通常涉及處理邊界條件,例如空值、負數或特殊字元。忽略這些條件可能會導致執行時間錯誤或不準確的結果:
function countWords($str) { return str_word_count($str); } var_dump(countWords(null)); // 输出:0,期望:null
解決方案:總是檢查邊界條件並以適當的方式處理它們。
誤解4:沒有最佳化演算法效能
在某些情況下,演算法的效能可能會隨著資料量的增加而下降。 PHP提供了多種方法來最佳化演算法效能,例如快取、使用索引數組以及利用內建函數:
// 使用缓存以避免重复计算 $cache = []; function factorial($num) { if (isset($cache[$num])) { return $cache[$num]; } $result = $num; for ($i = $num - 1; $i > 1; $i--) { $result *= $i; } $cache[$num] = $result; return $result; } // 使用索引数组快速查找元素 $arr = [ 'key1' => 'value1', 'key2' => 'value2', ]; var_dump(isset($arr['key1'])); // 输出:true // 利用内置函数提高效率 $arr = range(1, 100); $sum = array_sum($arr); // 使用 array_sum() 代替循环累加
實戰案例:使用二元搜尋樹儲存和尋找資料
以下程式碼展示如何使用PHP實作二元搜尋樹,這是用於儲存和高效查找元素的資料結構:
class BinarySearchTree { private $root; public function insert($value) { if ($this->root === null) { $this->root = new Node($value); return; } $this->_insert($value, $this->root); } private function _insert($value, Node $node) { if ($value < $node->value) { if ($node->left === null) { $node->left = new Node($value); } else { $this->_insert($value, $node->left); } } else if ($value > $node->value) { if ($node->right === null) { $node->right = new Node($value); } else { $this->_insert($value, $node->right); } } } public function find($value) { if ($this->root === null) { return null; } return $this->_find($value, $this->root); } private function _find($value, Node $node) { if ($value === $node->value) { return $node; } else if ($value < $node->value) { if ($node->left === null) { return null; } else { return $this->_find($value, $node->left); } } else if ($value > $node->value) { if ($node->right === null) { return null; } else { return $this->_find($value, $node->right); } } } }
以上是PHP 演算法實作中常見的誤區的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
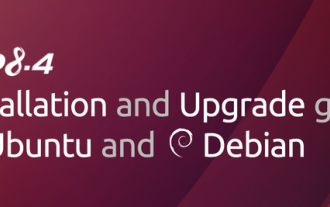
PHP 8.4 帶來了多項新功能、安全性改進和效能改進,同時棄用和刪除了大量功能。 本指南介紹如何在 Ubuntu、Debian 或其衍生版本上安裝 PHP 8.4 或升級到 PHP 8.4
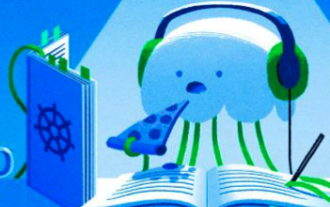
Visual Studio Code,也稱為 VS Code,是一個免費的原始碼編輯器 - 或整合開發環境 (IDE) - 可用於所有主要作業系統。 VS Code 擁有大量針對多種程式語言的擴展,可以輕鬆編寫
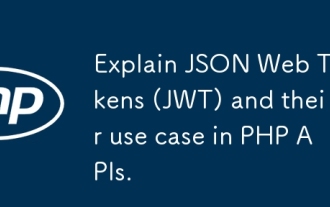
JWT是一種基於JSON的開放標準,用於在各方之間安全地傳輸信息,主要用於身份驗證和信息交換。 1.JWT由Header、Payload和Signature三部分組成。 2.JWT的工作原理包括生成JWT、驗證JWT和解析Payload三個步驟。 3.在PHP中使用JWT進行身份驗證時,可以生成和驗證JWT,並在高級用法中包含用戶角色和權限信息。 4.常見錯誤包括簽名驗證失敗、令牌過期和Payload過大,調試技巧包括使用調試工具和日誌記錄。 5.性能優化和最佳實踐包括使用合適的簽名算法、合理設置有效期、
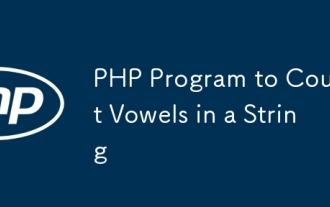
字符串是由字符組成的序列,包括字母、數字和符號。本教程將學習如何使用不同的方法在PHP中計算給定字符串中元音的數量。英語中的元音是a、e、i、o、u,它們可以是大寫或小寫。 什麼是元音? 元音是代表特定語音的字母字符。英語中共有五個元音,包括大寫和小寫: a, e, i, o, u 示例 1 輸入:字符串 = "Tutorialspoint" 輸出:6 解釋 字符串 "Tutorialspoint" 中的元音是 u、o、i、a、o、i。總共有 6 個元
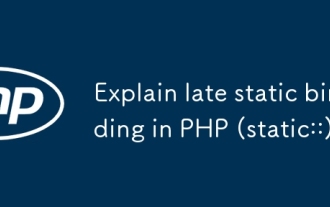
靜態綁定(static::)在PHP中實現晚期靜態綁定(LSB),允許在靜態上下文中引用調用類而非定義類。 1)解析過程在運行時進行,2)在繼承關係中向上查找調用類,3)可能帶來性能開銷。
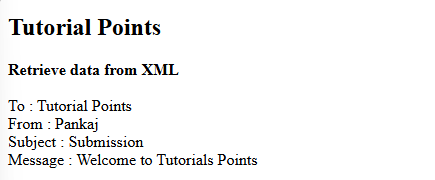
本教程演示瞭如何使用PHP有效地處理XML文檔。 XML(可擴展的標記語言)是一種用於人類可讀性和機器解析的多功能文本標記語言。它通常用於數據存儲

PHP的魔法方法有哪些? PHP的魔法方法包括:1.\_\_construct,用於初始化對象;2.\_\_destruct,用於清理資源;3.\_\_call,處理不存在的方法調用;4.\_\_get,實現動態屬性訪問;5.\_\_set,實現動態屬性設置。這些方法在特定情況下自動調用,提升代碼的靈活性和效率。
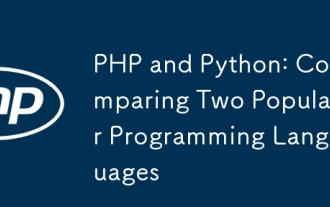
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
