就是实现这样的东西, 我在网上找的这段代码加入主页里面没有用呢\
请高手给我说下详细步骤,我加到APP的任何页面和任何位置都不行,
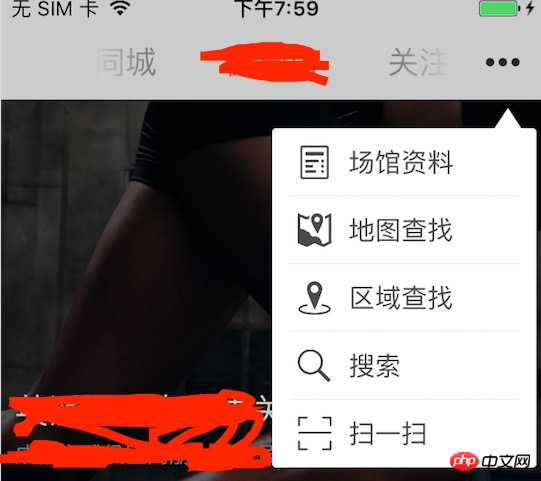
#import <UIKit/UIKit.h>
@interface CCNavigationBarMenuItem : NSObject
@property (nonatomic, strong) UIImage *image;// 图标
@property (nonatomic, copy ) NSString *title;// 标题
@property (nonatomic, strong) UIColor *titleColor;// 颜色 #4a4a4a
@property (nonatomic, strong) UIFont *titleFont;// 字体大小 system 15.5 17
(instancetype)navigationBarMenuItemWithImage:(UIImage )image title:(NSString )title;
@end
@interface CCNavigationBarMenu : NSObject
@property (nonatomic, strong) NSArray *items;// 菜单数据
@property (nonatomic, assign) CGRect triangleFrame;// 三角形位置 default : CGRectMake(width-25, 0, 12, 12)
@property (nonatomic, strong) UIColor *separatorColor;// 分割线颜色 #e8e8e8
@property (nonatomic, assign) CGFloat rowHeight;// 菜单条目高度
@property (nonatomic, copy) void(^didSelectMenuItem)(CCNavigationBarMenu menu, CCNavigationBarMenuItem item);// 点击条目
(instancetype)initWithOrigin:(CGPoint)origin width:(CGFloat)width;
(void)show;
(void)dismiss;
@end
#define ITEM_IMAGE_SIZE CGSizeMake(IS_IPHONE_5_OR_LESS ? 20 : 22, IS_IPHONE_5_OR_LESS ? 20 : 22)
#pragma mark - model
@implementation CCNavigationBarMenuItem
(instancetype)navigationBarMenuItemWithImage:(UIImage )image title:(NSString )title {
return [[CCNavigationBarMenuItem alloc] initWithImage:image title:title];
}
(instancetype)initWithImage:(UIImage )image title:(NSString )title {
self = [super init];
if (self) {
self.image = image;
self.title = title;
self.titleColor = [UIColor colorWithRGBHexString:@"#4a4a4a"];
self.titleFont = [UIFont appFontOfSize:IS_IPHONE_5_OR_LESS ? 15.5 : 17];
}
return self;
}
@end
#pragma mark - cell
@interface CCNavigationBarMenuTableViewCell : UITableViewCell
@property (nonatomic, strong) UIImageView *itemImageView;
@property (nonatomic, strong) UILabel *itemTitleLabel;
@property (nonatomic, strong) CCNavigationBarMenuItem *model;
@end
@implementation CCNavigationBarMenuTableViewCell
(instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier {
self = [super initWithStyle:style reuseIdentifier:reuseIdentifier];
if (self) {
[self _initSubviews];
}
return self;
}
(void)_initSubviews {
self.itemImageView = [[UIImageView alloc] init];
self.itemImageView.contentMode = UIViewContentModeScaleAspectFit;
[self.contentView addSubview:self.itemImageView];
[self.itemImageView mas_makeConstraints:^(MASConstraintMaker *make) {
make.left.equalTo(self.contentView).offset(15);
make.centerY.equalTo(self.contentView);
make.size.mas_equalTo(ITEM_IMAGE_SIZE);
}];
self.itemTitleLabel = [[UILabel alloc] init];
self.itemTitleLabel.font = [UIFont appFontOfSize:17];
self.itemTitleLabel.textColor = [UIColor colorWithRGBHexString:@"#4a4a4a"];
[self.contentView addSubview:self.itemTitleLabel];
[self.itemTitleLabel mas_makeConstraints:^(MASConstraintMaker *make) {
make.left.equalTo(self.itemImageView.mas_right).offset(10);
make.centerY.equalTo(self.contentView);
}];
}
#pragma mark - setter
(void)setModel:(CCNavigationBarMenuItem *)model {
self.itemImageView.image = model.image;
self.itemTitleLabel.text = model.title;
self.itemTitleLabel.font = model.titleFont;
self.itemTitleLabel.textColor = model.titleColor;
}
@end
@interface CCNavigationBarMenu ()<UITableViewDelegate, UITableViewDataSource>
@property (nonatomic, assign) CGPoint origin;
@property (nonatomic, assign) CGFloat width;
// 遮罩
@property (nonatomic, strong) UIView *maskView;
// 内容
@property (nonatomic, strong) UIView *contentView;
@property (nonatomic, strong) UIView *triangleView;
@property (nonatomic, strong) UITableView *tableView;
@end
@implementation CCNavigationBarMenu
(instancetype)initWithOrigin:(CGPoint)origin width:(CGFloat)width {
self = [super init];
if (self) {
_items = [NSArray array];
_separatorColor = [UIColor colorWithRGBHexString:@"#e8e8e8"];
_rowHeight = ITEM_IMAGE_SIZE.height + (IS_IPHONE_5_OR_LESS ? 20 : 26);
_triangleFrame = CGRectMake(width - 25, 0, IS_IPHONE_5_OR_LESS ? 16 : 18, IS_IPHONE_5_OR_LESS ? 12 : 14);
// 背景
self.maskView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, SCREEN_WIDTH, SCREEN_HEIGHT)];
self.maskView.backgroundColor = [UIColor blackColor];
self.maskView.alpha = .2f;
[self.maskView addGestureRecognizer:[[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(dismiss)]];
// 内容
self.origin = origin;
self.width = width;
self.contentView = [[UIView alloc] initWithFrame:CGRectMake(origin.x, origin.y, width, self.rowHeight * self.items.count + CGRectGetHeight(self.triangleFrame) + 5)];
self.contentView.backgroundColor = [UIColor clearColor];
// 三角形
[self _applytriangleView];
// item
self.tableView = [[UITableView alloc] initWithFrame:CGRectMake(0, CGRectGetHeight(self.triangleFrame) + 5, width, self.rowHeight * self.items.count) style:UITableViewStylePlain];
[self.tableView registerClass:[CCNavigationBarMenuTableViewCell class] forCellReuseIdentifier:NSStringFromClass([CCNavigationBarMenuTableViewCell class])];
self.tableView.delegate = self;
self.tableView.dataSource = self;
self.tableView.bounces = NO;
self.tableView.showsVerticalScrollIndicator = NO;
self.tableView.separatorColor = self.separatorColor;
self.tableView.layer.cornerRadius = 2;
self.tableView.tableFooterView = [[UIView alloc] init];
if ([self.tableView respondsToSelector:@selector(setSeparatorInset:)]) {
[self.tableView setSeparatorInset:UIEdgeInsetsMake(0, 10, 0, 10)];
}
if ([self.tableView respondsToSelector:@selector(setLayoutMargins:)]) {
[self.tableView setLayoutMargins:UIEdgeInsetsMake(0, 10, 0, 10)];
}
[self.contentView addSubview:self.tableView];
}
return self;
}
#pragma mark - UITableViewDataSource
(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.items.count;
}
(UITableViewCell )tableView:(UITableView )tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
CCNavigationBarMenuTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:NSStringFromClass([CCNavigationBarMenuTableViewCell class])];
cell.model = self.items[indexPath.row];
return cell;
}
#pragma mark - UITableViewDelegate
(CGFloat)tableView:(UITableView )tableView heightForRowAtIndexPath:(NSIndexPath )indexPath {
return self.rowHeight;
}
(void)tableView:(UITableView )tableView willDisplayCell:(UITableViewCell )cell forRowAtIndexPath:(NSIndexPath *)indexPath {
if ([cell respondsToSelector:@selector(setSeparatorInset:)]) {
cell.separatorInset = UIEdgeInsetsMake(0, 10, 0, 10);
}
if ([cell respondsToSelector:@selector(setLayoutMargins:)]) {
cell.layoutMargins = UIEdgeInsetsMake(0, 10, 0, 10);
}
}
(void)tableView:(UITableView )tableView didSelectRowAtIndexPath:(NSIndexPath )indexPath {
[tableView deselectRowAtIndexPath:indexPath animated:YES];
[self dismiss];
if (self.didSelectMenuItem) {
self.didSelectMenuItem(self, self.items[indexPath.row]);
}
}
(void)_applytriangleView {
if (self.triangleView == nil) {
self.triangleView = [[UIView alloc] init];
self.triangleView.backgroundColor = [UIColor whiteColor];
[self.contentView addSubview:self.triangleView];
}
self.triangleFrame = CGRectMake(CGRectGetMinX(self.triangleFrame), 5, CGRectGetWidth(self.triangleFrame), CGRectGetHeight(self.triangleFrame));
self.triangleView.frame = self.triangleFrame;
CAShapeLayer *shaperLayer = [CAShapeLayer layer];
CGMutablePathRef path = CGPathCreateMutable();
CGPathMoveToPoint(path, &CGAffineTransformIdentity, CGRectGetWidth(self.triangleFrame) / 2.0, 0);
CGPathAddLineToPoint(path, &CGAffineTransformIdentity, 0, CGRectGetHeight(self.triangleFrame));
CGPathAddLineToPoint(path, &CGAffineTransformIdentity, CGRectGetWidth(self.triangleFrame), CGRectGetHeight(self.triangleFrame));
shaperLayer.path = path;
self.triangleView.layer.mask = shaperLayer;
}
#pragma mark - setter
(void)setItems:(NSArray *)items {
_items = items;
self.contentView.height = self.rowHeight * self.items.count + CGRectGetHeight(self.triangleFrame) + 5;
self.tableView.height = self.rowHeight * self.items.count;
[self.tableView reloadData];
}
(void)settriangleFrame:(CGRect)triangleFrame {
_triangleFrame = triangleFrame;
self.contentView.height = self.rowHeight * self.items.count + CGRectGetHeight(self.triangleFrame) + 5;
self.tableView.height = self.rowHeight * self.items.count;
[self _applytriangleView];
}
(void)setSeparatorColor:(UIColor *)separatorColor {
_separatorColor = separatorColor;
self.tableView.separatorColor = separatorColor;
}
(void)setRowHeight:(CGFloat)rowHeight {
_rowHeight = rowHeight;
self.contentView.height = self.rowHeight * self.items.count + CGRectGetHeight(self.triangleFrame) + 5;
self.tableView.height = self.rowHeight * self.items.count;
[self.tableView reloadData];
}
#pragma mark - public
(void)show {
UIWindow *window = [UIApplication sharedApplication].keyWindow;
[window addSubview:self.maskView];
[window addSubview:self.contentView];
// self.contentView.transform = CGAffineTransformMakeScale(0.0, 0.0);
self.contentView.alpha = 0.0f;
self.maskView.alpha = .0f;
[UIView animateWithDuration:.35 animations:^{
self.contentView.alpha = 1.0;
// self.contentView.transform = CGAffineTransformMakeScale(1.0, 1.0);
self.maskView.alpha = .2f;
}];
}
(void)dismiss {
[UIView animateWithDuration:.15 animations:^{
// self.contentView.transform = CGAffineTransformMakeScale(.5f, .5f);
self.contentView.alpha = 0.0f;
self.maskView.alpha = .0f;
} completion:^(BOOL finished) {
if (finished) {
[self.maskView removeFromSuperview];
[self.contentView removeFromSuperview];
}
}];
}
// --------- 用法 --------
self.menuItems = [NSMutableArray array];
menuImages = @[@"icon_menu_venue", @"icon_menu_map", @"icon_menu_region", @"icon_menu_search", @"icon_menu_scan"];
menuTitles = @[@"场馆资料", @"地图查找", @"区域查找", @"搜索", @"扫一扫"];
for (int i = 0; i < menuImages.count; i++) {
CCNavigationBarMenuItem *item = [CCNavigationBarMenuItem navigationBarMenuItemWithImage:[UIImage imageNamed:menuImages[i]] title:menuTitles[i]];
[self.menuItems addObject:item];
}
self.menu = [[CCNavigationBarMenu alloc] initWithOrigin:CGPointMake(APP_WIDTH - (IS_IPHONE_5_OR_LESS ? 160 : 180), 64) width:IS_IPHONE_5_OR_LESS ? 156 : 176];
self.menu.items = self.menuItems;
认证高级PHP讲师