php短信接口代码,php短信接口
php短信接口代码,php短信接口
本文实例为大家分享了几个常用的php短信接口代码,供大家参考,具体内容如下
1. 短信调用class
<?php /** * User: Administrator * Date: 2016/5/8 0008 * Time: 下午 2:36 */ class Sms{ //Luosimao api key private $_api_key = ''; private $_last_error = array(); private $_use_ssl = FALSE; private $_ssl_api_url = array( 'send' => 'http://www.bkjia.com/v1/send.json', 'send_batch' => 'http://www.bkjia.com/v1/send_batch.json', 'status' => 'http://www.bkjia.com/v1/status.json', ); private $_api_url = array( 'send' => 'http://www.bkjia.com/v1/send.json', 'send_batch' => 'http://www.bkjia.com/send_batch.json', 'status' => 'http://www.bkjia.com/v1/status.json', ); /** * @param array $param 配置参数 * api_key api秘钥,在luosimao短信后台短信->触发发送下面可查看 * use_ssl 启用HTTPS地址,HTTPS有一定性能损耗,可选,默认不启用 */ public function __construct( $param = array() ){ if( !isset( $param['api_key'] ) ){ die("api key error."); } if( isset( $param['api_key'] ) ){ $this->_api_key = $param['api_key']; } if( isset( $param['use_ssl'] ) ){ $this->_use_ssl = $param['use_ssl']; } } //触发,单发,适用于验证码,订单触发提醒类 public function send( $mobile , $message = '' ){ $api_url = !$this->_use_ssl ? $this->_api_url['send'] : $this->_ssl_api_url['send']; $param = array( 'mobile' => $mobile , 'message' => $message, ); $res = $this->http_post( $api_url ,$param ); return @json_decode( $res ,TRUE ); } //批量发送,用于大批量发送 public function send_batch( $mobile_list = array() , $message = array() , $time = '' ){ $api_url = !$this->_use_ssl ? $this->_api_url['send_batch'] : $this->_ssl_api_url['send_batch']; $mobile_list = is_array( $mobile_list ) ? implode( ',' , $mobile_list ) : $mobile_list; $param = array( 'mobile_list' => $mobile_list , 'message' => $message, 'time' => $time, ); $res = $this->http_post( $api_url ,$param ); return @json_decode( $res ,TRUE ); } //获取短信账号余额 public function get_deposit(){ $api_url = !$this->_use_ssl ? $this->_api_url['status'] : $this->_ssl_api_url['status']; $res = $this->http_get( $api_url ); return @json_decode( $res ,TRUE ); } /** * @param string $type 接收类型,用于在服务器端接收上行和发送状态,接收地址需要在luosimao后台设置 * @param array $param 传入的参数,从推送的url中获取,官方文档:https://luosimao.com/docs/api/ */ public function recv( $type = 'status' , $param = array() ){ if( $type == 'status' ){ if( $param['batch_id'] && $param['mobile'] && $param['status'] ){ //状态 // do record } }elseif( $type == 'incoming' ){ //上行回复 if( $param['mobile'] && $param['message'] ){ // do record } } } /** * @param string $api_url 接口地址 * @param array $param post参数 * @param int $timeout 超时时间 * @return bool */ private function http_post( $api_url = '' , $param = array() , $timeout = 5 ){ if( !$api_url ){ die("error api_url"); } $ch = curl_init(); curl_setopt( $ch, CURLOPT_URL, $api_url ); curl_setopt( $ch, CURLOPT_HTTP_VERSION , CURL_HTTP_VERSION_1_0 ); curl_setopt( $ch, CURLOPT_CONNECTTIMEOUT, $timeout ); curl_setopt( $ch, CURLOPT_RETURNTRANSFER, TRUE); curl_setopt( $ch, CURLOPT_HEADER, FALSE); if( parse_url( $api_url )['scheme'] == 'https' ){ curl_setopt($ch, CURLOPT_SSL_VERIFYHOST , FALSE); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER , FALSE); } curl_setopt( $ch, CURLOPT_HTTPAUTH , CURLAUTH_BASIC); curl_setopt( $ch, CURLOPT_USERPWD , 'api:key-'.$this->_api_key ); curl_setopt( $ch, CURLOPT_POST, TRUE); curl_setopt( $ch, CURLOPT_POSTFIELDS, $param ); $res = curl_exec( $ch ); $error = curl_error( $ch ); curl_close( $ch ); if( $error ){ $this->_last_error[] = $error; return FALSE; } return $res; } /** * @param string $api_url 接口地址 * @param string $timeout 超时时间 * @return bool */ private function http_get( $api_url = '' , $timeout = '' ){ if( !$api_url ){ die("error api_url"); } $ch = curl_init(); curl_setopt( $ch, CURLOPT_URL, $api_url ); curl_setopt( $ch, CURLOPT_HTTP_VERSION , CURL_HTTP_VERSION_1_0 ); curl_setopt( $ch, CURLOPT_CONNECTTIMEOUT, $timeout ); curl_setopt( $ch, CURLOPT_RETURNTRANSFER, TRUE); curl_setopt( $ch, CURLOPT_HEADER, FALSE); if( parse_url( $api_url )['scheme'] == 'https' ){ curl_setopt( $ch, CURLOPT_SSL_VERIFYHOST , FALSE); curl_setopt( $ch, CURLOPT_SSL_VERIFYPEER , FALSE); } curl_setopt( $ch, CURLOPT_HTTPAUTH , CURLAUTH_BASIC); curl_setopt( $ch, CURLOPT_USERPWD , 'api:key-'.$this->_api_key ); $res = curl_exec( $ch ); $error = curl_error( $ch ); curl_close( $ch ); if( $error ){ $this->_last_error[] = curl_error( $ch ); return FALSE; } return $res; } public function last_error(){ return $this->_last_error; } }
2.短信发送示例
//send 单发接口 require 'sms.php'; $sms = new Sms( array('api_key' => '86f52f3ce0647dc24da53eafe29fadd4' , 'use_ssl' => FALSE ) ); $res = $sms->send_batch( array('13761428268') , '验证码:19272【帮客之家】'); if( $res ){ if( isset( $res['error'] ) && $res['error'] == 0 ){ echo 'success'; }else{ echo 'failed,code:'.$res['error'].',msg:'.$res['msg']; } }else{ var_dump( $sms->last_error() ); } exit;
3.批量发送示例
require 'sms.php'; $sms = new Sms( array('api_key' => '86f52f3ce0647dc24da53eafe29fadd4' , 'use_ssl' => FALSE ) ); //send 单发接口 $res = $sms->send_batch( array('13761428268') , '验证码:19272【帮客之家】'); if( $res ){ if( isset( $res['error'] ) && $res['error'] == 0 ){ echo 'success'; }else{ echo 'failed,code:'.$res['error'].',msg:'.$res['msg']; } }else{ var_dump( $sms->last_error() ); } exit;
4.获取余额示例
//deposit 余额查询 require 'sms.php'; $sms = new Sms( array('api_key' => '86f52f3ce0647dc24da53eafe29fadd4' , 'use_ssl' => FALSE ) ); $res = $sms->get_deposit(); if( $res ){ if( isset( $res['error'] ) && $res['error'] == 0 ){ echo 'desposit:'.$res['deposit']; }else{ echo 'failed,code:'.$res['error'].',msg:'.$res['msg']; } }else{ var_dump( $sms->last_error() ); } exit;
以上就是本文的全部内容,希望对大家的学习有所帮助。

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
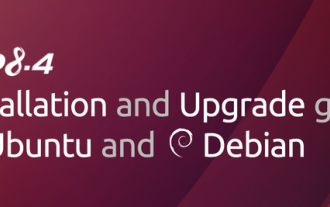
PHP 8.4 带来了多项新功能、安全性改进和性能改进,同时弃用和删除了大量功能。 本指南介绍了如何在 Ubuntu、Debian 或其衍生版本上安装 PHP 8.4 或升级到 PHP 8.4
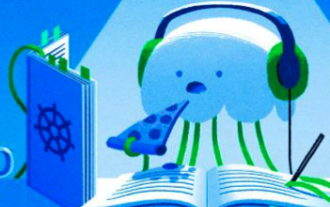
Visual Studio Code,也称为 VS Code,是一个免费的源代码编辑器 - 或集成开发环境 (IDE) - 可用于所有主要操作系统。 VS Code 拥有针对多种编程语言的大量扩展,可以轻松编写
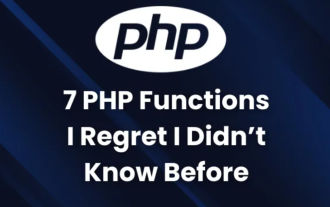
如果您是一位经验丰富的 PHP 开发人员,您可能会感觉您已经在那里并且已经完成了。您已经开发了大量的应用程序,调试了数百万行代码,并调整了一堆脚本来实现操作
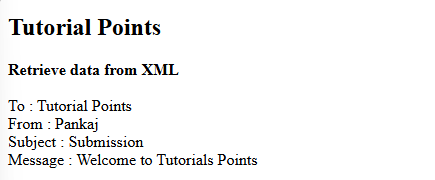
本教程演示了如何使用PHP有效地处理XML文档。 XML(可扩展的标记语言)是一种用于人类可读性和机器解析的多功能文本标记语言。它通常用于数据存储
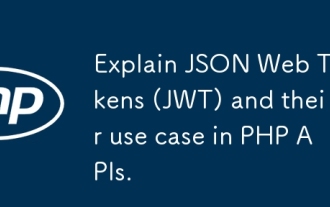
JWT是一种基于JSON的开放标准,用于在各方之间安全地传输信息,主要用于身份验证和信息交换。1.JWT由Header、Payload和Signature三部分组成。2.JWT的工作原理包括生成JWT、验证JWT和解析Payload三个步骤。3.在PHP中使用JWT进行身份验证时,可以生成和验证JWT,并在高级用法中包含用户角色和权限信息。4.常见错误包括签名验证失败、令牌过期和Payload过大,调试技巧包括使用调试工具和日志记录。5.性能优化和最佳实践包括使用合适的签名算法、合理设置有效期、
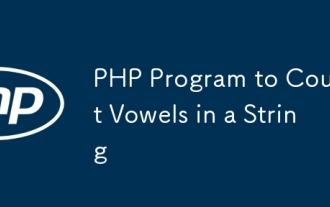
字符串是由字符组成的序列,包括字母、数字和符号。本教程将学习如何使用不同的方法在PHP中计算给定字符串中元音的数量。英语中的元音是a、e、i、o、u,它们可以是大写或小写。 什么是元音? 元音是代表特定语音的字母字符。英语中共有五个元音,包括大写和小写: a, e, i, o, u 示例 1 输入:字符串 = "Tutorialspoint" 输出:6 解释 字符串 "Tutorialspoint" 中的元音是 u、o、i、a、o、i。总共有 6 个元
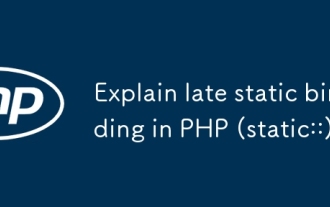
静态绑定(static::)在PHP中实现晚期静态绑定(LSB),允许在静态上下文中引用调用类而非定义类。1)解析过程在运行时进行,2)在继承关系中向上查找调用类,3)可能带来性能开销。

PHP的魔法方法有哪些?PHP的魔法方法包括:1.\_\_construct,用于初始化对象;2.\_\_destruct,用于清理资源;3.\_\_call,处理不存在的方法调用;4.\_\_get,实现动态属性访问;5.\_\_set,实现动态属性设置。这些方法在特定情况下自动调用,提升代码的灵活性和效率。
