如何在 Go 语言中测试包?
通过使用 Go 语言的内置测试框架,开发者可以轻松地为他们的代码编写和运行测试。测试文件以 _test.go 结尾,并包含 Test 开头的测试函数,其中 *testing.T 参数表示测试实例。错误信息使用 t.Error() 记录。可以通过运行 go test 命令来运行测试。子测试允许将测试函数分解成更小的部分,并通过 t.Run() 创建。实战案例包括针对 utils 包中 IsStringPalindrome() 函数编写的测试文件,该文件使用一系列输入字符串和预期输出来测试该函数的正确性。
如何在 Go 语言中测试包?
Go 语言提供了强大的内置测试框架,让开发者轻松地为其代码编写和运行测试。下面将介绍如何使用 Go 测试包对你的程序进行测试。
编写测试
在 Go 中,测试文件以 _test.go 结尾,并放在与要测试的包所在的目录中。测试文件包含一个或多个测试函数,它们以 Test
开头,后面跟要测试的功能。
以下是一个示例测试函数:
import "testing" func TestAdd(t *testing.T) { if Add(1, 2) != 3 { t.Error("Add(1, 2) returned an incorrect result") } }
*testing.T
参数表示测试实例。错误信息使用 t.Error()
记录。
运行测试
可以通过运行以下命令来运行测试:
go test
如果测试成功,将显示诸如 "PASS" 之类的消息。如果出现错误,将显示错误信息。
使用子测试
子测试允许将一个测试函数分解成更小的部分。这有助于组织测试代码并提高可读性。
以下是如何编写子测试:
func TestAdd(t *testing.T) { t.Run("PositiveNumbers", func(t *testing.T) { if Add(1, 2) != 3 { t.Error("Add(1, 2) returned an incorrect result") } }) t.Run("NegativeNumbers", func(t *testing.T) { if Add(-1, -2) != -3 { t.Error("Add(-1, -2) returned an incorrect result") } }) }
实战案例
假设我们有一个名为 utils
的包,里面包含一个 IsStringPalindrome()
函数,用于检查一个字符串是否是回文字符串。
下面是如何编写一个测试文件来测试这个函数:
package utils_test import ( "testing" "utils" ) func TestIsStringPalindrome(t *testing.T) { tests := []struct { input string expected bool }{ {"", true}, {"a", true}, {"bb", true}, {"racecar", true}, {"level", true}, {"hello", false}, {"world", false}, } for _, test := range tests { t.Run(test.input, func(t *testing.T) { if got := utils.IsStringPalindrome(test.input); got != test.expected { t.Errorf("IsStringPalindrome(%s) = %t; want %t", test.input, got, test.expected) } }) } }
在这个测试文件中:
-
tests
数组定义了一系列输入字符串和预期的输出。 -
for
循环遍历tests
数组,并使用t.Run()
创建子测试。 - 每个子测试调用
utils.IsStringPalindrome()
函数并将其结果与预期结果进行比较。如果结果不一致,它使用t.Errorf()
记录错误。
以上是如何在 Go 语言中测试包?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
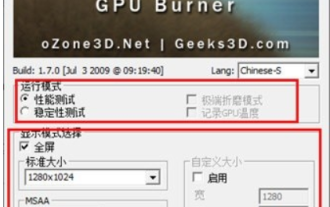
furmark怎么看?1、在主界面中设置“运行模式”和“显示模式”,还能调整“测试模式”,点击“开始”按钮。2、等待片刻后,就会看到测试结果,包含了显卡各种参数。furmark怎么算合格?1、用furmark烤机,半个小时左右看一下结果,基本上在85度左右徘徊,峰值87度,室温19度。大号机箱,5个机箱风扇口,前置两个,上置两个,后置一个,不过只装了一个风扇。所有配件都没有超频。2、一般情况下,显卡的正常温度应该在“30-85℃”之间。3、就算是大夏天周围环境温度过高,正常温度也是“50-85℃
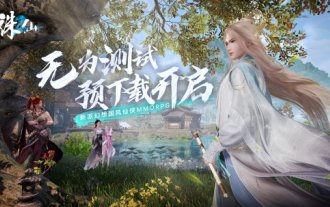
新派幻想仙侠MMORPG《诛仙2》“无为测试”即将于4月23日开启,在原著千年后的诛仙大陆,会发生怎样的全新仙侠冒险故事?六境仙侠大世界,全日制修仙学府,自由自在的修仙生活,仙界中的万般妙趣都在等待着仙友们亲自前往探索!“无为测试”预下载现已开启,仙友们可前往官网下载,开服前无法登录游戏服务器,激活码可在预下载安装完成后使用。《诛仙2》“无为测试”开放时间:4月23日10:00——5月6日23:59诛仙正统续作全新仙侠冒险篇章《诛仙2》以《诛仙》小说为蓝图,在继承原著世界观的基础上,将游戏背景设
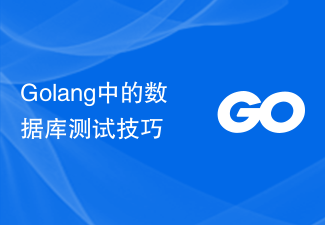
Golang中的数据库测试技巧引言:在开发应用程序时,数据库测试是一个非常重要的环节。合适的测试方法可以帮助我们发现潜在的问题并确保数据库操作的正确性。本文将介绍Golang中的一些常用数据库测试技巧,并提供相应的代码示例。一、使用内存数据库进行测试在编写数据库相关的测试时,我们通常会面临一个问题:如何在不依赖外部数据库的情况下进行测试?这里我们可以使用内存
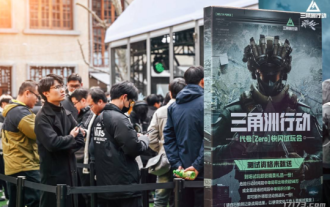
《三角洲行动》于今日(3月7日)将开启一场名为“代号:ZERO”的大规模PC测试。而在上周末,这款游戏在上海举办了一次线下快闪体验活动,17173也有幸受邀参与其中。此次测试距离上一次仅仅相隔四个多月,这不禁让我们好奇,在这么短的时间内,《三角洲行动》将会带来哪些新的亮点与惊喜?四个多月前,我已先行在线下品鉴会和首测版本中体验了《三角洲行动》。当时,游戏仅开放了“危险行动”这一模式。然而,《三角洲行动》在当时的表现已然令人瞩目。在各大厂商纷纷涌向手游市场的背景下,如此一款与国际水准相媲美的FPS
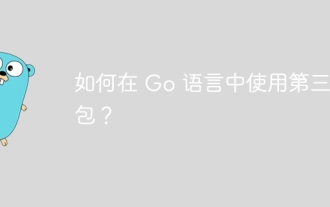
在Go中使用第三方包:使用goget命令安装包,如:gogetgithub.com/user/package。导入包,如:import("github.com/user/package")。示例:使用encoding/json包解析JSON数据:安装:gogetencoding/json导入:import("encoding/json")解析:json.Unmarshal([]byte(jsonString),&data)
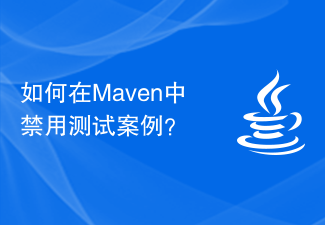
Maven是一个开源的项目管理工具,常用于Java项目的构建、依赖管理及文档发布等任务。在使用Maven进行项目构建时,有时候我们希望在执行mvnpackage等命令时忽略测试阶段,这在某些情况下会提高构建速度,尤其是在需要快速构建原型或测试环境时。本文将详细介绍如何在Maven中忽略测试阶段,并附有具体的代码示例。为什么要忽略测试在项目开发过程中,通常会
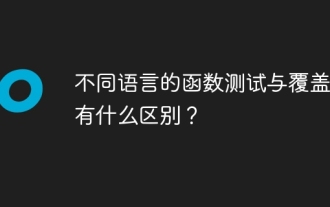
函数测试通过黑盒和白盒测试验证函数功能,而代码覆盖率衡量了测试用例覆盖的代码部分。不同语言(如Python和Java)的测试框架、覆盖率工具和特性不同。实战案例展示了如何使用Python的Unittest和Coverage以及Java的JUnit和JaCoCo进行函数测试和覆盖率评估。
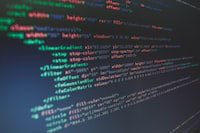
简介持续集成(CI)和持续部署(CD)是现代软件开发中的关键实践,它们可以帮助团队更快、更可靠地交付高质量的软件。jenkins是一个流行的开源CI/CD工具,它可以自动化构建、测试和部署流程。本文将介绍如何使用PHP与Jenkins一起设置CI/CD管道。设置Jenkins安装Jenkins:从Jenkins官网下载并安装Jenkins。创建项目:从Jenkins仪表板中创建一个新的项目,并将其命名为与您的php项目相匹配的名称。配置源代码管理:将您的PHP项目的git存储库配置为Jenkin
