Slim和Phalcon的中间件实战指南
Jun 01, 2024 pm 03:59 PM
phalcon
slim
在 Slim 和 Phalcon 中使用中间件指南:Slim: 使用 slim/middleware 组件,创建一个自定义中间件函数,验证用户是否已登录,并根据结果重定向或继续执行。Phalcon: 创建一个实现 Phalcon\Mvc\UserInterface 接口的中间件类,并在类中定义在路由执行之前和之后执行的代码,然后在应用程序中注册中间件。实战示例: 在 Slim 中,创建中间件来缓存 API 响应,在 Phalcon 中,创建中间件来记录请求日志。
Slim 和 Phalcon 的中间件实战指南
在现代 Web 开发中,中间件是一种流行的技术,用于在应用程序处理 HTTP 请求和生成响应之前或之后执行自定义代码。通过使用中间件,您可以实现各种操作,如身份验证、缓存、日志记录和异常处理。
在 PHP 中,Slim 和 Phalcon 是两个流行的框架,提供了对中间件的强大支持。本文将提供一个实战指南,说明如何在这两个框架中使用中间件。
Slim
在 Slim 中,中间件可以使用 slim/middleware
组件轻松添加。要安装它:
composer require slim/middleware
登录后复制
以下是一个简单的身份验证中间件示例:
<?php $app->add(function ($request, $response, $next) { // 验证用户是否已登录 if (!isset($_SESSION['user_id'])) { return $response->withRedirect('/'); } // 继续执行下一个中间件 return $next($request, $response); });
登录后复制
Phalcon
Phalcon 具有开箱即用的中间件支持。要在 Phalcon 中创建中间件,您需要创建一个类并实现 Phalcon\Mvc\UserInterface
接口:
<?php use Phalcon\Mvc\UserInterface; class ExampleMiddleware implements UserInterface { public function beforeExecuteRoute($dispatcher) { // 在执行路由之前执行此代码 } public function afterExecuteRoute($dispatcher) { // 在执行路由之后执行此代码 } }
登录后复制
然后,您可以将中间件注册到应用程序:
<?php $middleware = new ExampleMiddleware(); $app->middleware->add( $middleware, Phalcon\Events\Manager::EVENT_BEFORE_EXECUTE_ROUTE, Phalcon\Events\Manager::PRIORITY_LOW );
登录后复制
实战案例
使用 Slim 缓存 API 响应
<?php $app->add(function ($request, $response, $next) { $cacheKey = 'api_response_' . $request->getUri()->getPath(); $response = $cache->get($cacheKey); if (!$response) { $response = $next($request, $response); $cache->set($cacheKey, $response, 3600); // 缓存 1 小时 } return $response; });
登录后复制
使用 Phalcon 记录请求日志
<?php use Phalcon\Logger; use Phalcon\Mvc\UserInterface; class LoggerMiddleware implements UserInterface { private $logger; public function __construct(Logger $logger) { $this->logger = $logger; } public function beforeExecuteRoute($dispatcher) { $this->logger->info('Request: ' . $dispatcher->getActionName() . ' - ' . $dispatcher->getParams()); } }
登录后复制
以上是Slim和Phalcon的中间件实战指南的详细内容。更多信息请关注PHP中文网其他相关文章!
本站声明
本文内容由网友自发贡献,版权归原作者所有,本站不承担相应法律责任。如您发现有涉嫌抄袭侵权的内容,请联系admin@php.cn

热门文章
击败分裂小说需要多长时间?
3 周前
By DDD
仓库:如何复兴队友
3 周前
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island冒险:如何获得巨型种子
3 周前
By 尊渡假赌尊渡假赌尊渡假赌
公众号网页更新缓存难题:如何避免版本更新后旧缓存影响用户体验?
3 周前
By 王林
R.E.P.O.能量晶体解释及其做什么(黄色晶体)
1 周前
By 尊渡假赌尊渡假赌尊渡假赌

热门文章
击败分裂小说需要多长时间?
3 周前
By DDD
仓库:如何复兴队友
3 周前
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island冒险:如何获得巨型种子
3 周前
By 尊渡假赌尊渡假赌尊渡假赌
公众号网页更新缓存难题:如何避免版本更新后旧缓存影响用户体验?
3 周前
By 王林
R.E.P.O.能量晶体解释及其做什么(黄色晶体)
1 周前
By 尊渡假赌尊渡假赌尊渡假赌

热门文章标签

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
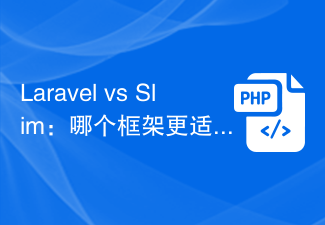
Laravel vs Slim:哪个框架更适合构建RESTful API?
