JS 中的弱集?
JavaScript 中的 WeakSet 是一种特殊的集合,其中的对象具有“弱”引用。这意味着如果没有其他对存储在 WeakSet 中的对象的引用,则该对象可以被垃圾收集。与常规 Set 不同,WeakSet 只接受对象作为元素,并且这些对象是弱保存的。这使得 WeakSet 对于您想要跟踪对象但又不想阻止它们在其他地方不再需要时被垃圾回收的情况非常有用。
WeakSet的特点:
1 仅限对象: WeakSet 只能包含对象,不能包含数字或字符串等原始值。
2 弱引用: WeakSet 中对对象的引用是弱引用,这意味着如果没有其他对象引用,则该对象可以被垃圾回收。
3 无 Size 属性: 您无法获取 WeakSet 的大小,因为它不公开其元素的数量。
4。 无迭代: 你不能迭代 WeakSet,因为它没有像 forEach 这样的方法或像 Set 那样的迭代器。
基本用法:
let weakset = new WeakSet(); let obj1 = {name: "object1"}; let obj2 = {name: "object2"}; weakset.add(obj1); weakset.add(obj2); console.log(weakset.has(obj1)); // true console.log(weakset.has(obj2)); // true obj1 = null; // obj1 is eligible for garbage collection console.log(weakset.has(obj1)); // false
有趣的示例:
让我们想象一个场景,WeakSet 就像间谍的秘密俱乐部。这个俱乐部非常隐秘,如果间谍不再活跃,他们就会消失得无影无踪。俱乐部从不记录其成员的数量,并且您无法获得当前在俱乐部中的成员的列表。您只能询问俱乐部中是否有特定间谍。
// The Secret Spy Club class Spy { constructor(name) { this.name = name; } introduce() { console.log(`Hi, I am Agent ${this.name}`); } } let spy1 = new Spy("007"); let spy2 = new Spy("008"); let spyClub = new WeakSet(); // Adding spies to the secret club spyClub.add(spy1); spyClub.add(spy2); console.log(spyClub.has(spy1)); // true console.log(spyClub.has(spy2)); // true spy1.introduce(); // Hi, I am Agent 007 spy2.introduce(); // Hi, I am Agent 008 // Spy 007 finishes his mission and disappears spy1 = null; // Now Agent 007 is no longer in the club and is eligible for garbage collection // Let's see if spies are still in the club console.log(spyClub.has(spy1)); // false, because Agent 007 is no longer referenced console.log(spyClub.has(spy2)); // true, because Agent 008 is still active // Agent 008 finishes his mission too spy2 = null; // Now Agent 008 is also eligible for garbage collection // Checking club members again console.log(spyClub.has(spy2)); // false, no active spies left
在这个有趣的例子中,WeakSet 是秘密间谍俱乐部,而间谍是对象。当间谍(对象)完成其任务并且没有其他引用它们时,它们就会消失(被垃圾收集),没有任何痕迹,就像当对象不再在代码中的其他地方引用时从 WeakSet 中删除它们一样。
以上是JS 中的弱集?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
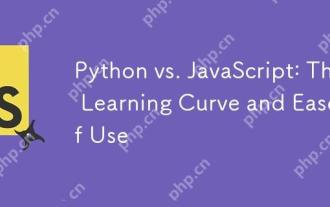
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
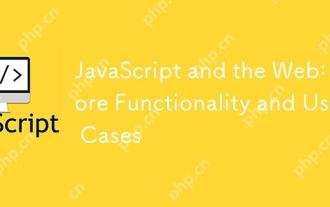
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
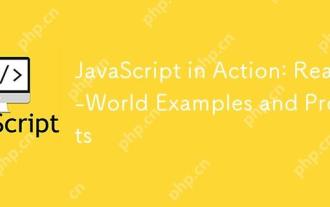
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
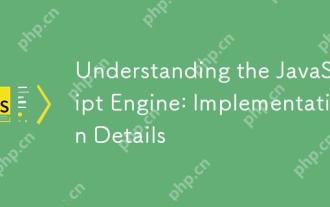
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
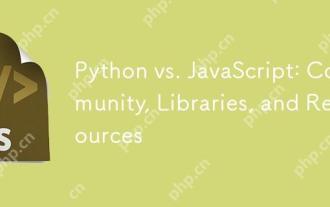
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
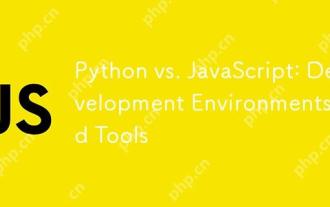
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
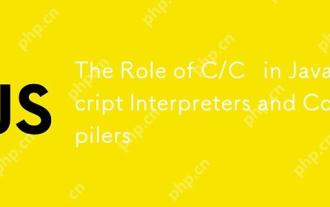
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
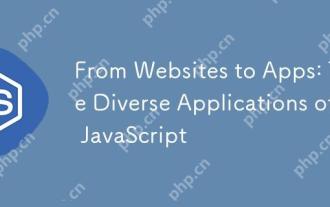
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
