运行不受信任的 JavaScript 代码
重要:这仅与运行 JavaScript 和 TypeScript 代码有关。话虽如此,写作也可能是用其他语言运行其他代码的方向。
允许用户在您的应用程序中执行他们的代码打开了一个自定义和功能的世界,但它也使您的平台面临重大的安全威胁。
鉴于它是用户代码,一切都在预料之中,从停止服务器(可能是无限循环)到窃取敏感信息。
本文将探讨缓解运行用户代码的各种策略,包括 Web Workers、静态代码分析等等......
你应该关心
有很多场景需要运行用户提供的代码,从 CodeSandbox 和 StackBiltz 这样的协作开发环境到像 January 这样的可定制 API 平台。即使是代码游乐场也容易受到风险的影响。
也就是说,安全运行用户提供的代码的两个基本优势是:
- 获得用户的信任:即使用户值得信赖,他们也可能会执行从其他故意坏人那里复制的代码。
- 保护您的环境:您最不需要的就是一段代码来停止您的服务器。边思考 (true) {}
定义“敏感信息”
运行用户代码并没有什么害处,除非您担心这可能会导致某些数据被盗。无论您关心什么数据,都将被视为敏感信息。例如,在大多数情况下,JWT 是敏感信息(也许用作身份验证机制时)
可能会出现什么问题
考虑 JWT 存储在随每个请求发送的 cookie 中的潜在风险。用户可能会无意中触发将 JWT 发送到恶意服务器的请求,并且...
- 跨站脚本 (XSS)。
- 拒绝服务 (DoS) 攻击。
- 数据泄露。如果没有适当的保护措施,这些威胁可能会损害应用程序的完整性和性能。
方法
邪恶的评估
最简单,但风险最大。
eval('console.log("I am dangerous!")');
当您运行此代码时,它会记录该消息。本质上,eval 是一个能够访问全局/窗口范围的 JS 解释器。
const res = await eval('fetch(`https://jsonplaceholder.typicode.com/users`)'); const users = await res.json();
此代码使用在全局范围内定义的 fetch。解释器不知道这一点,但由于 eval 可以访问窗口,所以它知道。这意味着在浏览器中运行 eval 与在服务器环境或工作线程中运行它不同。
eval(`document.body`);
这个怎么样...
eval(`while (true) {}`);
此代码将停止浏览器选项卡。您可能会问为什么用户会对自己这样做。好吧,他们可能会从互联网上复制代码。这就是为什么首选进行静态分析并/或对执行进行时间限制。
您可能想查看有关 eval 的 MDN 文档
时间框执行可以通过在 Web Worker 中运行代码并使用 setTimeout 来限制执行时间来完成。
async function timebox(code, timeout = 5000) { const worker = new Worker('user-runner-worker.js'); worker.postMessage(code); const timerId = setTimeout(() => { worker.terminate(); reject(new Error('Code execution timed out')); }, timeout); return new Promise((resolve, reject) => { worker.onmessage = event => { clearTimeout(timerId); resolve(event.data); }; worker.onerror = error => { clearTimeout(timerId); reject(error); }; }); } await timebox('while (true) {}');
函数构造器
这与 eval 类似,但它更安全一些,因为它无法访问封闭的范围。
const userFunction = new Function('param', 'console.log(param);'); userFunction(2);
此代码将记录 2。
注意:第二个参数是函数体。
函数构造函数无法访问封闭范围,因此以下代码将抛出错误。
function fnConstructorCannotUseMyScope() { let localVar = 'local value'; const userFunction = new Function('return localVar'); return userFunction(); }
但它可以访问全局范围,因此上面的 fetch 示例可以工作。
网络工作者
您可以在 WebWorker 上运行“函数构造函数和 eval”,由于没有 DOM 访问,这会更安全。
要实施更多限制,请考虑禁止使用全局对象,例如 fetch、XMLHttpRequest、sendBeacon 查看本文以了解如何做到这一点。
隔离虚拟机
Isolated-VM 是一个库,允许您在单独的 VM 中运行代码(v8 的 Isolate 接口)
import ivm from 'isolated-vm'; const code = `count += 5;`; const isolate = new ivm.Isolate({ memoryLimit: 32 /* MB */ }); const script = isolate.compileScriptSync(code); const context = isolate.createContextSync(); const jail = context.global; jail.setSync('log', console.log); context.evalSync('log("hello world")');
此代码将记录 hello world
网络组装
这是一个令人兴奋的选项,因为它提供了运行代码的沙盒环境。需要注意的是,您需要一个具有 Javascript 绑定的环境。然而,一个名为 Extism 的有趣项目促进了这一点。您可能想遵循他们的教程。
它的迷人之处在于,您将使用 eval 来运行代码,但考虑到 WebAssembly 的性质,DOM、网络、文件系统和对主机环境的访问都是不可能的(尽管它们可能会根据不同的环境而有所不同) wasm 运行时)。
function evaluate() { const { code, input } = JSON.parse(Host.inputString()); const func = eval(code); const result = func(input).toString(); Host.outputString(result); } module.exports = { evaluate };
You'll have to compile the above code first using Extism, which will output a Wasm file that can be run in an environment that has Wasm-runtime (browser or node.js).
const message = { input: '1,2,3,4,5', code: ` const sum = (str) => str .split(',') .reduce((acc, curr) => acc + parseInt(curr), 0); module.exports = sum; `, }; // continue running the wasm file
Docker
We're now moving to the server-side, Docker is a great option to run code in an isolation from the host machine. (Beware of container escape)
You can use dockerode to run the code in a container.
import Docker from 'dockerode'; const docker = new Docker(); const code = `console.log("hello world")`; const container = await docker.createContainer({ Image: 'node:lts', Cmd: ['node', '-e', code], User: 'node', WorkingDir: '/app', AttachStdout: true, AttachStderr: true, OpenStdin: false, AttachStdin: false, Tty: true, NetworkDisabled: true, HostConfig: { AutoRemove: true, ReadonlyPaths: ['/'], ReadonlyRootfs: true, CapDrop: ['ALL'], Memory: 8 * 1024 * 1024, SecurityOpt: ['no-new-privileges'], }, });
Keep in mind that you need to make sure the server has docker installed and running. I'd recommend having a separate server dedicated only to this that acts as a pure-function server.
Moreover, you might benefit from taking a look at sysbox, a VM-like container runtime that provides a more secure environment. Sysbox is worth it, especially if the main app is running in a container, which means that you'll be running Docker in Docker.
This was the method of choice at January but soon enough, the language capabilities mandated more than passing the code through the container shell. Besides, for some reason, the server memory spikes frequently; we run the code inside self-removable containers on every 1s debounced keystroke. (You can do better!)
Other options
- Web Containers
- MicroVM (Firecraker)
- Deno subhosting
- Wasmer
- ShadowRealms
Safest option
I'm particularly fond of Firecracker, but it’s a bit of work to set up, so if you cannot afford the time yet, you want to be on the safe side, do a combination of static analysis and time-boxing execution. You can use esprima to parse the code and check for any malicious act.
How to run TypeScript code?
Well, same story with one (could be optional) extra step: Transpile the code to JavaScript before running it. Simply put, you can use esbuild or typescript compiler, then continue with the above methods.
async function build(userCode: string) { const result = await esbuild.build({ stdin: { contents: `${userCode}`, loader: 'ts', resolveDir: __dirname, }, inject: [ // In case you want to inject some code ], platform: 'node', write: false, treeShaking: false, sourcemap: false, minify: false, drop: ['debugger', 'console'], keepNames: true, format: 'cjs', bundle: true, target: 'es2022', plugins: [ nodeExternalsPlugin(), // make all the non-native modules external ], }); return result.outputFiles![0].text; }
Notes:
- Rust-based bundlers usually offer a web assembly version, which means you can transpile the code in the browser. Esbuild does have a web assembly version.
- Don't include user specified imports into the bundle unless you've allow-listed them.
Additionally, you can avoid transpiling altogether by running the code using Deno or Bun in a docker container since they support TypeScript out of the box.
Conclusion
Running user code is a double-edged sword. It can provide a lot of functionality and customization to your platform, but it also exposes you to significant security risks. It’s essential to understand the risks and take appropriate measures to mitigate them and remember that the more isolated the environment, the safer it is.
References
- January instant compilation
- Running untrusted JavaScript in Node.js
- How do languages support executing untrusted user code at runtime?
- Safely Evaluating JavaScript with Context Data
以上是运行不受信任的 JavaScript 代码的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
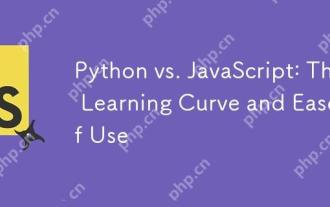
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
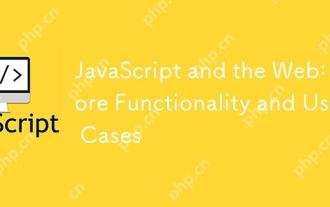
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
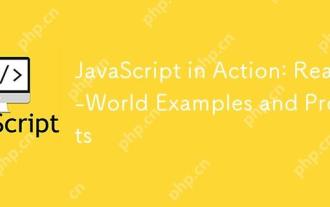
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
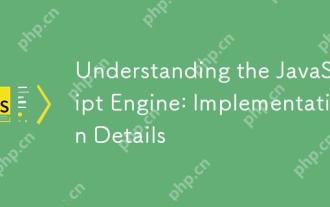
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
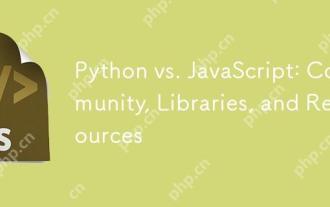
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
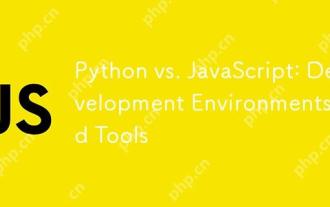
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
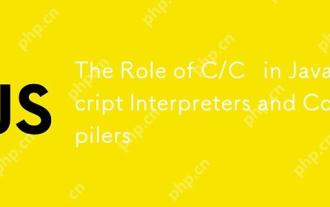
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。

Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
