Spring Boot 中的异常处理
异常处理是构建健壮且用户友好的应用程序的关键部分。在 Spring Boot 中,我们可以通过多种方式处理异常,以确保我们的应用程序保持稳定并向用户提供有意义的反馈。本指南将涵盖异常处理的不同策略,包括自定义异常、全局异常处理、验证错误和生产最佳实践。
1. 异常处理基础知识
异常是破坏程序正常流程的事件。它们可以分为:
- 检查异常: 在编译时检查的异常。
- 未经检查的异常(运行时异常):运行时发生的异常。
- 错误:应用程序不应处理的严重问题,例如 OutOfMemoryError。
2. 自定义异常类
创建自定义异常类有助于处理应用程序中的特定错误情况。
package com.example.SpringBootRefresher.exception; public class DepartmentNotFoundException extends RuntimeException { public DepartmentNotFoundException(String message) { super(message); } }
3. 控制器中的异常处理
@ExceptionHandler 注解:
您可以在控制器类中定义处理异常的方法。
package com.example.SpringBootRefresher.controller; import com.example.SpringBootRefresher.exception.DepartmentNotFoundException; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class DepartmentController { @GetMapping("/department") public String getDepartment() { // Simulate an exception throw new DepartmentNotFoundException("Department not found!"); } @ExceptionHandler(DepartmentNotFoundException.class) public ResponseEntity<String> handleDepartmentNotFoundException(DepartmentNotFoundException ex) { return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND); } }
4. 使用@ControllerAdvice进行全局异常处理
要全局处理异常,您可以使用@ControllerAdvice和集中式异常处理程序。
package com.example.SpringBootRefresher.error; import com.example.SpringBootRefresher.entity.ErrorMessage; import com.example.SpringBootRefresher.exception.DepartmentNotFoundException; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.ResponseStatus; import org.springframework.web.context.request.WebRequest; import org.springframework.web.servlet.mvc.method.annotation.ResponseEntityExceptionHandler; @ControllerAdvice @ResponseStatus public class CustomResponseEntityExceptionHandler extends ResponseEntityExceptionHandler { @ExceptionHandler(DepartmentNotFoundException.class) public ResponseEntity<ErrorMessage> handleDepartmentNotFoundException(DepartmentNotFoundException exception, WebRequest request) { ErrorMessage message = new ErrorMessage( HttpStatus.NOT_FOUND.value(), exception.getMessage(), request.getDescription(false) ); return ResponseEntity.status(HttpStatus.NOT_FOUND) .body(message); } @ExceptionHandler(Exception.class) public ResponseEntity<ErrorMessage> handleGlobalException(Exception exception, WebRequest request) { ErrorMessage message = new ErrorMessage( HttpStatus.INTERNAL_SERVER_ERROR.value(), exception.getMessage(), request.getDescription(false) ); return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR) .body(message); } }
5. 创建标准错误响应
定义标准错误响应类来构建错误消息。
package com.example.SpringBootRefresher.entity; public class ErrorMessage { private int statusCode; private String message; private String description; public ErrorMessage(int statusCode, String message, String description) { this.statusCode = statusCode; this.message = message; this.description = description; } // Getters and setters public int getStatusCode() { return statusCode; } public void setStatusCode(int statusCode) { this.statusCode = statusCode; } public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } }
6. 处理验证错误
Spring Boot 与 Bean Validation (JSR-380) 集成良好。要全局处理验证错误,请使用@ControllerAdvice。
package com.example.SpringBootRefresher.error; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.validation.FieldError; import org.springframework.web.bind.MethodArgumentNotValidException; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.ResponseStatus; import org.springframework.web.context.request.WebRequest; import java.util.HashMap; import java.util.Map; @ControllerAdvice @ResponseStatus public class ValidationExceptionHandler extends ResponseEntityExceptionHandler { @ExceptionHandler(MethodArgumentNotValidException.class) public ResponseEntity<Map<String, String>> handleValidationExceptions(MethodArgumentNotValidException ex) { Map<String, String> errors = new HashMap<>(); ex.getBindingResult().getAllErrors().forEach((error) -> { String fieldName = ((FieldError) error).getField(); String errorMessage = error.getDefaultMessage(); errors.put(fieldName, errorMessage); }); return new ResponseEntity<>(errors, HttpStatus.BAD_REQUEST); } }
7. 使用@ResponseStatus处理简单异常
对于简单的情况,可以用@ResponseStatus注解异常类来指定HTTP状态码。
package com.example.SpringBootRefresher.exception; import org.springframework.http.HttpStatus; import org.springframework.web.bind.annotation.ResponseStatus; @ResponseStatus(HttpStatus.NOT_FOUND) public class DepartmentNotFoundException extends RuntimeException { public DepartmentNotFoundException(String message) { super(message); } }
8. 生产最佳实践
- 一致的错误响应: 确保您的应用程序返回一致且结构化的错误响应。使用标准错误响应类。
- 日志记录: 记录异常以用于调试和监控目的。确保敏感信息不会在日志中暴露。
- 用户友好的消息:提供用户友好的错误消息。避免向用户暴露内部细节或堆栈跟踪。
- 安全性: 请谨慎对待错误响应中包含的信息,以避免暴露敏感数据。
- 文档:为您的团队和未来的维护人员记录您的异常处理策略。
概括
Spring Boot 中的异常处理涉及使用 @ExceptionHandler、@ControllerAdvice 和 @ResponseStatus 等注释来有效地管理错误。通过创建自定义异常、处理验证错误并遵循最佳实践,您可以构建强大的应用程序,以优雅地处理错误并向用户提供有意义的反馈。使用 Java 17 功能可确保您的应用程序利用 Java 生态系统中的最新改进。
以上是Spring Boot 中的异常处理的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
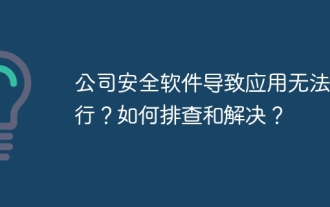
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
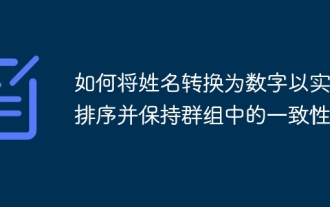
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
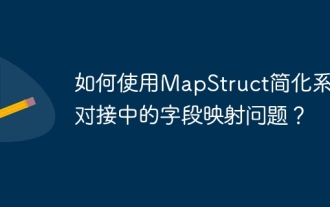
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
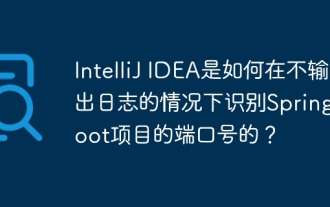
在使用IntelliJIDEAUltimate版本启动Spring...
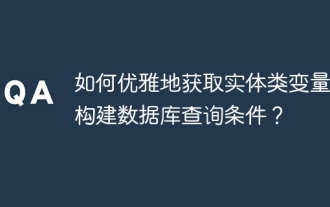
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
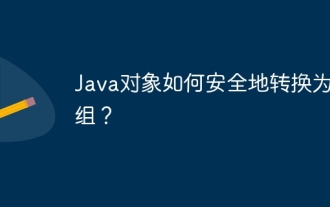
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
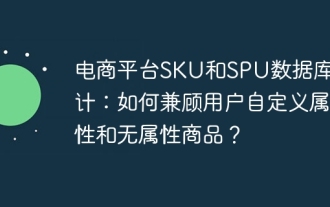
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
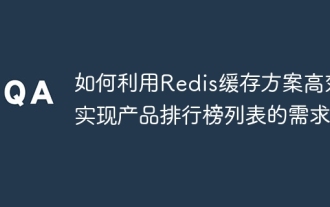
Redis缓存方案如何实现产品排行榜列表的需求?在开发过程中,我们常常需要处理排行榜的需求,例如展示一个�...
