无缝 React 学习的 JavaScript 先决条件
简介
React,一个用于构建用户界面的强大 JavaScript 库,已成为现代 Web 开发的必备条件。在深入研究 React 之前,深入了解 JavaScript 核心概念至关重要。这些基础技能将使您的学习曲线更加平滑,并帮助您构建更高效、更有效的 React 应用程序。本文将引导您了解学习 React 之前需要掌握的顶级 JavaScript 概念。
变量和数据类型
理解变量
变量是任何编程语言的基础,JavaScript 也不例外。在 JavaScript 中,变量是保存数据值的容器。您可以使用 var、let 或 const 声明变量。
var name = 'John'; let age = 30; const isDeveloper = true;
JavaScript 中的数据类型
JavaScript 有多种数据类型,包括:
- 基本类型:数字、字符串、布尔值、Null、未定义、符号和 BigInt。
- 引用类型:对象、数组和函数。
了解这些数据类型如何工作以及如何有效地使用它们对于使用 React 至关重要。
函数和箭头函数
传统功能
函数是执行特定任务的可重用代码块。传统的函数语法如下所示:
function greet(name) { return `Hello, ${name}!`; }
箭头函数
ES6 中引入的箭头函数提供了更短的语法并在词法上绑定了 this 值。以下是如何使用箭头语法编写相同的函数:
const greet = (name) => `Hello, ${name}!`;
在使用 React 组件和钩子时,理解函数,尤其是箭头函数是至关重要的。
ES6 语法
Let 和 Const
ES6 引入了 let 和 const 来声明块作用域的变量。与具有函数作用域的 var 不同,let 和 const 有助于避免由于作用域问题而导致的错误。
let count = 0; const PI = 3.14;
模板文字
模板文字允许您在字符串文字中嵌入表达式,使字符串连接更具可读性。
let name = 'John'; let greeting = `Hello, ${name}!`;
解构赋值
解构允许您将数组中的值或对象中的属性解压到不同的变量中。
let person = { name: 'John', age: 30 }; let { name, age } = person
掌握 ES6 语法对于编写现代 JavaScript 和使用 React 至关重要。
异步 JavaScript
回调
回调是作为参数传递给其他函数并在某些操作完成后执行的函数。
function fetchData(callback) { setTimeout(() => { callback('Data fetched'); }, 1000); }
承诺
Promise 提供了一种更简洁的方式来处理异步操作并且可以链接。
let promise = new Promise((resolve, reject) => { setTimeout(() => resolve('Data fetched'), 1000); }); promise.then((message) => console.log(message));
异步/等待
Async/await 语法允许您以同步方式编写异步代码,提高可读性。
async function fetchData() { let response = await fetch('url'); let data = await response.json(); console.log(data); }
理解异步 JavaScript 对于在 React 应用程序中处理数据获取至关重要。
文档对象模型 (DOM)
什么是 DOM?
DOM 是 Web 文档的编程接口。它代表页面,以便程序可以更改文档结构、样式和内容。
操作 DOM
您可以使用 JavaScript 来操作 DOM,选择元素并修改其属性或内容。
let element = document.getElementById('myElement'); element.textContent = 'Hello, World!';
React 抽象了直接的 DOM 操作,但理解它的工作原理对于调试和优化性能至关重要。
事件处理
添加事件监听器
JavaScript 中的事件处理涉及监听用户交互(例如单击和按键)并做出相应响应。
let button = document.getElementById('myButton'); button.addEventListener('click', () => { alert('Button clicked!'); });
事件冒泡和捕获
了解事件传播对于有效处理事件非常重要。事件冒泡和捕获决定了事件处理程序的执行顺序。
// Bubbling document.getElementById('child').addEventListener('click', () => { console.log('Child clicked'); }); // Capturing document.getElementById('parent').addEventListener( 'click', () => { console.log('Parent clicked'); }, true );
事件处理是 React 应用程序中用户交互的核心部分。
面向对象编程(OOP)
类和对象
JavaScript 通过类和对象支持面向对象编程。类是创建对象的蓝图。
class Person { constructor(name, age) { this.name = name; this.age = age; } greet() { return `Hello, my name is ${this.name}`; } } let john = new Person('John', 30); console.log(john.greet());
继承
继承允许您基于现有类创建新类,从而促进代码重用。
class Developer extends Person { constructor(name, age, language) { super(name, age); this.language = language; } code() { return `${this.name} is coding in ${this.language}`; } } let dev = new Developer('Jane', 25, 'JavaScript'); console.log(dev.code());
OOP concepts are valuable for structuring and managing complex React applications.
Modules and Imports
Importing and Exporting
Modules allow you to break your code into reusable pieces. You can export functions, objects, or primitives from a module and import them into other modules.
// module.js export const greeting = 'Hello, World!'; // main.js import { greeting } from './module'; console.log(greeting);
Understanding modules is essential for organizing your React codebase efficiently.
JavaScript Promises
Creating Promises
Promises represent the eventual completion or failure of an asynchronous operation.
let promise = new Promise((resolve, reject) => { setTimeout(() => resolve('Data fetched'), 1000); }); promise.then((message) => console.log(message));
Chaining Promises
Promises can be chained to handle multiple asynchronous operations in sequence.
promise .then((message) => { console.log(message); return new Promise((resolve) => setTimeout(() => resolve('Another operation'), 1000)); }) .then((message) => console.log(message));
Mastering promises is crucial for managing asynchronous data fetching and operations in React.
Destructuring and Spread Operator
Destructuring Arrays and Objects
Destructuring simplifies extracting values from arrays or properties from objects.
let [a, b] = [1, 2]; let { name, age } = { name: 'John', age: 30 };
Spread Operator
The spread operator allows you to expand elements of an iterable (like an array) or properties of an object.
let arr = [1, 2, 3]; let newArr = [...arr, 4, 5]; let obj = { a: 1, b: 2 }; let newObj = { ...obj, c: 3 };
Understanding destructuring and the spread operator is essential for writing concise and readable React code.
FAQ
What Are the Core JavaScript Concepts Needed for React?
The core concepts include variables, data types, functions, ES6 syntax, asynchronous JavaScript, DOM manipulation, event handling, OOP, modules, promises, and destructuring.
Why Is Understanding Asynchronous JavaScript Important for React?
React applications often involve data fetching and asynchronous operations. Mastering callbacks, promises, and async/await ensures smooth handling of these tasks.
How Do ES6 Features Enhance React Development?
ES6 features like arrow functions, template literals, and destructuring improve code readability and efficiency, making React development more streamlined and manageable.
What Is the Role of the DOM in React?
While React abstracts direct DOM manipulation, understanding the DOM is crucial for debugging, optimizing performance, and understanding how React manages UI updates.
How Do Modules and Imports Help in React?
Modules and imports allow for better code organization, making it easier to manage and maintain large React codebases by dividing code into reusable, independent pieces.
Conclusion
Before diving into React, mastering these JavaScript concepts will provide a solid foundation for building robust and efficient applications. Each concept plays a critical role in making your React development journey smoother and more productive. Happy coding!
以上是无缝 React 学习的 JavaScript 先决条件的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
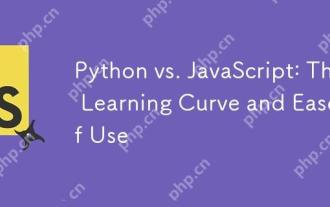
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
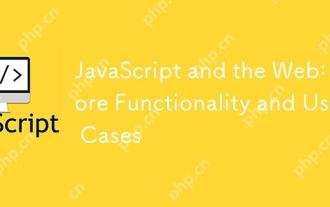
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
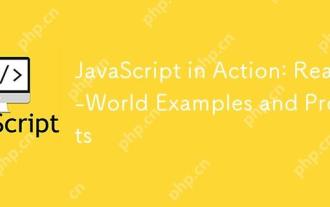
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
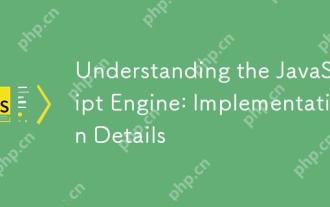
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
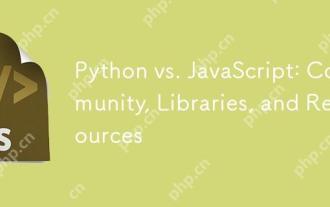
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
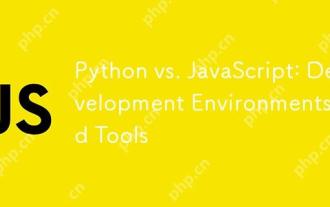
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
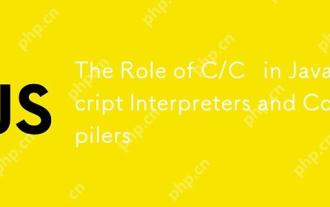
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。

Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
