释放实时 UI 的力量:使用 React.js、gRPC、Envoy 和 Golang 流式传输数据的初学者指南
作者:Naveen M
背景
作为 Kubernetes 平台团队的一员,我们面临着提供用户工作负载实时可见性的持续挑战。从监控资源使用情况到跟踪 Kubernetes 集群活动和应用程序状态,每个特定类别都有大量开源解决方案可用。然而,这些工具往往分散在不同的平台上,导致用户体验支离破碎。为了解决这个问题,我们利用了服务器端流的力量,使我们能够在用户访问我们的平台门户时立即提供实时资源使用情况、Kubernetes 事件和应用程序状态。
介绍
通过实现服务器端流式传输,我们可以将数据无缝流式传输到用户界面,提供最新信息,而无需手动刷新或不断的 API 调用。这种方法彻底改变了用户体验,使用户能够以统一、简化的方式即时可视化其工作负载的运行状况和性能。无论是监控资源利用率、随时了解 Kubernetes 事件还是密切关注应用程序状态,我们的服务器端流解决方案都将所有关键信息汇集在一个实时仪表板中,但这适用于任何想要向用户界面提供实时流数据。
通过多种工具和平台来收集重要见解的日子已经一去不复返了。通过我们简化的方法,用户在登陆我们的平台门户时就可以全面了解其 Kubernetes 环境。通过利用服务器端流的力量,我们改变了用户与其交互和监控其工作负载的方式,使他们的体验更加高效、直观和富有成效。
通过我们的博客系列,我们旨在引导您了解使用 React.js、Envoy、gRPC 和 Golang 等技术设置服务器端流的复杂过程。
这个项目涉及三个主要组件:
1.后端,使用Golang开发,利用gRPC服务器端流式传输数据。
2. Envoy 代理,负责让后端服务能够被外界访问。
3.前端,使用 React.js 构建,并使用 grpc-web 与后端建立通信。
该系列分为多个部分,以适应开发人员不同的语言偏好。如果您对 Envoy 在流媒体中的作用特别感兴趣,或者想了解如何在 Kubernetes 中部署 Envoy 代理,您可以跳到第二部分(Envoy 作为 Kubernetes 中的前端代理)并探索该方面,或者只是对前端部分,那么你可以直接查看博客的前端部分。
在最初的部分中,我们将重点关注该系列中最简单的部分:“如何使用 Go 设置 gRPC 服务器端流”。我们将展示具有服务器端流的示例应用程序。幸运的是,互联网上有大量针对该主题的内容,这些内容是根据您喜欢的编程语言量身定制的。
第 1 部分:如何使用 Go 设置 gRPC 服务器端流
是时候将我们的计划付诸行动了!假设您对以下概念有基本的了解,让我们直接进入实现:
- gRPC:它是一种通信协议,允许客户端和服务器有效地交换数据。
- 服务器端流:当服务器需要向客户端发送大量数据时,此功能特别有用。通过使用服务器端流式传输,服务器可以将数据分割成更小的部分,然后将它们一一发送。如果客户端接收到的数据足够多或者等待时间太长,则可以选择停止接收数据。
现在,让我们开始代码实现。
第 1 步:创建原型文件
首先,我们需要定义一个客户端和服务器端都会使用的 protobuf 文件。这是一个简单的例子:
syntax = "proto3"; package protobuf; service StreamService { rpc FetchResponse (Request) returns (stream Response) {} } message Request { int32 id = 1; } message Response { string result = 1; }
在此原型文件中,我们有一个名为 FetchResponse 的函数,它接受请求参数并返回响应消息流。
Step 2: Generate the Protocol Buffer File
Before we proceed, we need to generate the corresponding pb file that will be used in our Go program. Each programming language has its own way of generating the protocol buffer file. In Go, we will be using the protoc library.
If you haven't installed it yet, you can find the installation guide provided by Google.
To generate the protocol buffer file, run the following command:
protoc --go_out=plugins=grpc:. *.proto
Now, we have the data.pb.go file ready to be used in our implementation.
Step 3: Server side implementation
To create the server file, follow the code snippet below:
package main import ( "fmt" "log" "net" "sync" "time" pb "github.com/mnkg561/go-grpc-server-streaming-example/src/proto" "google.golang.org/grpc" ) type server struct{} func (s server) FetchResponse(in pb.Request, srv pb.StreamService_FetchResponseServer) error { log.Printf("Fetching response for ID: %d", in.Id) var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go func(count int) { defer wg.Done() time.Sleep(time.Duration(count) time.Second) resp := pb.Response{Result: fmt.Sprintf("Request #%d for ID: %d", count, in.Id)} if err := srv.Send(&resp); err != nil { log.Printf("Error sending response: %v", err) } log.Printf("Finished processing request number: %d", count) }(i) } wg.Wait() return nil } func main() { lis, err := net.Listen("tcp", ":50005") if err != nil { log.Fatalf("Failed to listen: %v", err) } s := grpc.NewServer() pb.RegisterStreamServiceServer(s, server{}) log.Println("Server started") if err := s.Serve(lis); err != nil { log.Fatalf("Failed to serve: %v", err) } }
In this server file, I have implemented the FetchResponse function, which receives a request from the client and sends a stream of responses back. The server simulates concurrent processing using goroutines. For each request, it streams five responses back to the client. Each response is delayed by a certain duration to simulate different processing times.
The server listens on port 50005 and registers the StreamServiceServer with the created server. Finally, it starts serving requests and logs a message indicating that the server has started.
Now you have the server file ready to handle streaming requests from clients.
Part 2
Stay tuned for Part 2 where we will continue to dive into the exciting world of streaming data and how it can revolutionize your user interface.
以上是释放实时 UI 的力量:使用 React.js、gRPC、Envoy 和 Golang 流式传输数据的初学者指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
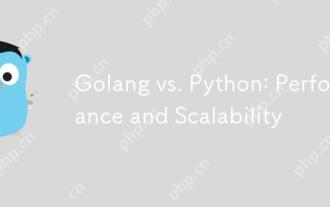
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
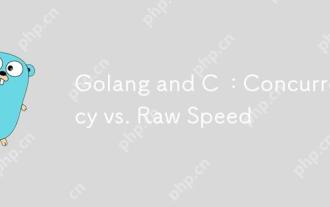
Golang在并发性上优于C ,而C 在原始速度上优于Golang。1)Golang通过goroutine和channel实现高效并发,适合处理大量并发任务。2)C 通过编译器优化和标准库,提供接近硬件的高性能,适合需要极致优化的应用。
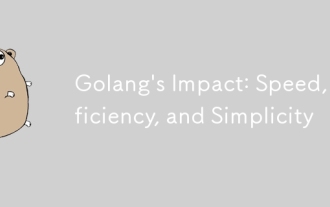
GoimpactsdevelopmentPositationalityThroughSpeed,效率和模拟性。1)速度:gocompilesquicklyandrunseff,ifealforlargeprojects.2)效率:效率:ITScomprehenSevestAndArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增强开发的简单性:3)SimpleflovelmentIcties:3)简单性。
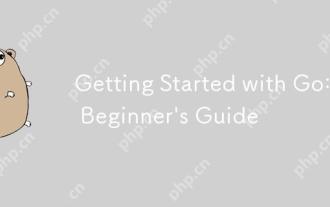
goisidealforbeginnersandsubableforforcloudnetworkservicesduetoitssimplicity,效率和concurrencyFeatures.1)installgromtheofficialwebsitealwebsiteandverifywith'.2)
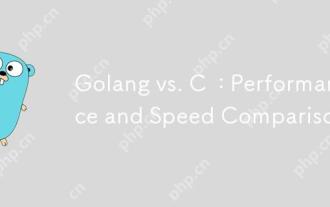
Golang适合快速开发和并发场景,C 适用于需要极致性能和低级控制的场景。1)Golang通过垃圾回收和并发机制提升性能,适合高并发Web服务开发。2)C 通过手动内存管理和编译器优化达到极致性能,适用于嵌入式系统开发。
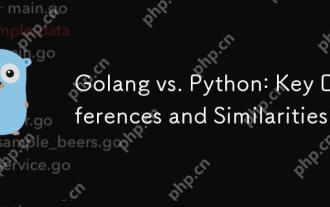
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。
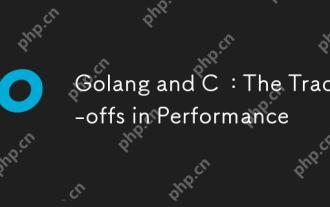
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
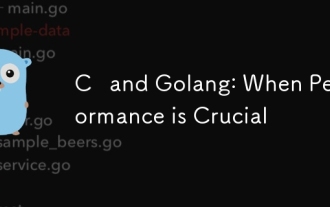
C 更适合需要直接控制硬件资源和高性能优化的场景,而Golang更适合需要快速开发和高并发处理的场景。1.C 的优势在于其接近硬件的特性和高度的优化能力,适合游戏开发等高性能需求。2.Golang的优势在于其简洁的语法和天然的并发支持,适合高并发服务开发。
