学习 React.js 的综合指南
React.js 由 Facebook 开发和维护,已成为用于构建用户界面(尤其是单页应用程序 (SPA))的最流行的 JavaScript 库之一。 React 以其灵活性、高效和易用性而闻名,拥有庞大的社区和丰富的资源供各个级别的开发人员使用。无论您是初学者还是希望将 React 添加到您的技能组合中的经验丰富的开发人员,本教程都将指导您了解 React.js 的基础知识。
1.什么是 React.js?
React.js 是一个开源 JavaScript 库,用于构建用户界面,特别是对于需要快速、交互式用户体验的单页应用程序。 React 允许开发人员创建大型 Web 应用程序,这些应用程序可以有效地更新和渲染以响应数据更改。它是基于组件的,这意味着 UI 被分成小的、可重用的部分,称为组件。
2.设置你的 React 环境
开始编码之前,您需要设置开发环境。请按照以下步骤操作:
第 1 步:安装 Node.js 和 npm
- Node.js:React 需要 Node.js 作为其构建工具。
- npm:节点包管理器(npm)用于安装库和包。
您可以从官网下载并安装Node.js。 npm 与 Node.js 捆绑在一起。
第 2 步:安装 Create React App
Facebook 创建了一个名为 Create React App 的工具,可以帮助您快速高效地建立新的 React 项目。在终端中运行以下命令:
npx create-react-app my-app
此命令创建一个名为 my-app 的新目录,其中包含启动 React 项目所需的所有文件和依赖项。
第3步:启动开发服务器
导航到您的项目目录并启动开发服务器:
cd my-app npm start
您的新 React 应用程序现在应该在 http://localhost:3000 上运行。
3.了解 React 组件
React 就是组件。 React 中的组件是一个独立的模块,它呈现一些输出,通常是 HTML。组件可以定义为 功能组件 或 类组件.
功能组件
功能组件是一个返回 HTML 的简单 JavaScript 函数(使用 JSX)。
示例:
function Welcome(props) { return <h1>Hello, {props.name}</h1>; }
类组件
类组件是一种更强大的定义组件的方式,并允许您管理本地状态和生命周期方法。
示例:
class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}</h1>; } }
4. JSX – JavaScript XML
JSX 是 JavaScript 的语法扩展,看起来与 HTML 类似。它允许您直接在 JavaScript 中编写 HTML,然后 React 会将其转换为真正的 DOM 元素。
示例:
const element = <h1>Hello, world!</h1>;
JSX 使 UI 结构的编写和可视化变得更加容易。然而,在幕后,JSX 被转换为 React.createElement() 调用。
5.状态和道具
道具
Props(“属性”的缩写)用于将数据从一个组件传递到另一个组件。它们是不可变的,这意味着它们不能被接收组件修改。
示例:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; }
状态
State 与 props 类似,但它是在组件内管理的,并且可以随着时间的推移而改变。状态通常用于组件需要跟踪的数据,例如用户输入。
示例:
class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } increment = () => { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.increment}>Increment</button> </div> ); } }
6.处理事件
在 React 中处理事件类似于在 DOM 元素中处理事件。但是,存在一些语法差异:
- React 事件使用驼峰命名法命名,而不是小写。
- 使用 JSX,您可以传递一个函数作为事件处理程序,而不是一个字符串。
示例:
function Button() { function handleClick() { alert('Button clicked!'); } return ( <button onClick={handleClick}> Click me </button> ); }
7.生命周期方法
React 中的类组件具有特殊的生命周期方法,允许您在组件生命周期中的特定时间运行代码。其中包括:
- componentDidMount:组件挂载后调用。
- componentDidUpdate:组件更新后调用。
- componentWillUnmount:在组件卸载之前调用。
示例:
class Timer extends React.Component { componentDidMount() { this.timerID = setInterval( () => this.tick(), 1000 ); } componentWillUnmount() { clearInterval(this.timerID); } render() { return ( <div> <h1>{this.state.date.toLocaleTimeString()}</h1> </div> ); } }
8. Conditional Rendering
In React, you can create different views depending on the state of your component.
Example:
function Greeting(props) { const isLoggedIn = props.isLoggedIn; if (isLoggedIn) { return <h1>Welcome back!</h1>; } return <h1>Please sign up.</h1>; }
9. Lists and Keys
When you need to display a list of data, React can render each item as a component. It’s important to give each item a unique "key" prop to help React identify which items have changed.
Example:
function NumberList(props) { const numbers = props.numbers; const listItems = numbers.map((number) => <li key={number.toString()}>{number}</li> ); return ( <ul>{listItems}</ul> ); }
10. React Hooks
React Hooks allow you to use state and other React features in functional components. Some of the most commonly used hooks include:
- useState: Allows you to add state to a functional component.
- useEffect: Lets you perform side effects in your function components.
- useContext: Provides a way to pass data through the component tree without having to pass props down manually at every level.
Example of useState:
function Counter() { const [count, setCount] = useState(0); return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}> Click me </button> </div> ); }
11. Building and Deploying React Applications
Once your application is ready, you can build it for production. Use the following command:
npm run build
This will create an optimized production build of your React app in the build folder. You can then deploy it to any web server.
Conclusion
React.js is a powerful tool for building modern web applications. By understanding components, state management, event handling, and hooks, you can create dynamic and interactive user interfaces. This tutorial covers the basics, but React's ecosystem offers much more, including advanced state management with Redux, routing with React Router, and server-side rendering with Next.js.
As you continue your journey with React, remember to leverage the wealth of online resources, including the official React documentation, community forums, and tutorials. Happy coding!
以上是学习 React.js 的综合指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
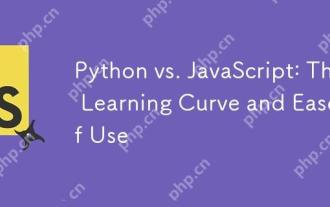
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
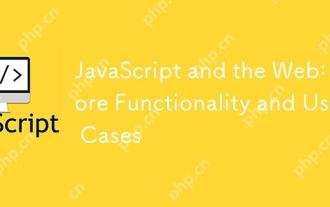
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
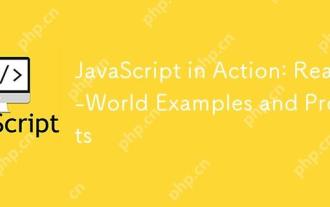
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
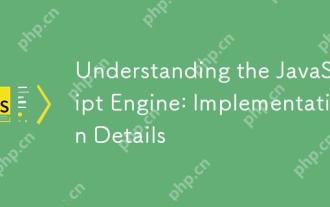
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
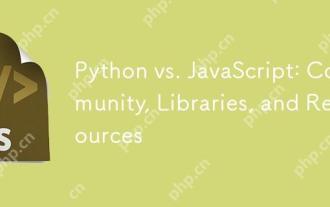
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
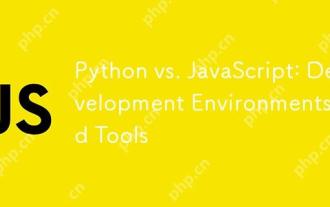
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
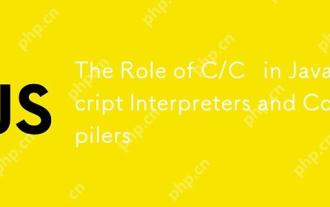
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
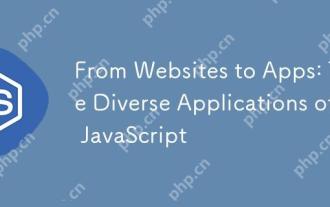
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
