使用 React Native 实现流畅的视频流
使用 React Native Video 构建流畅的视频流体验
在当今以移动为中心的世界中,视频流是许多应用程序的核心功能。无论是视频共享平台、教育应用程序还是多媒体丰富的社交网络,提供无缝的视频体验都是至关重要的。 React Native 是一种用于构建跨平台移动应用程序的流行框架,通过 React-native-video 库可以轻松集成视频流。
在本博客中,我们将逐步完成安装、配置和使用 React-native-video 的步骤,以便在 React Native 应用程序中创建流畅的视频流体验。
1. 安装
首先,您需要在 React Native 项目中安装react-native-video 库。
第 1 步:安装软件包
首先,使用npm或yarn安装库:
npm install react-native-video react-native-video-cache
或
yarn add react-native-video react-native-video-cache
对于 iOS,您可能需要安装必要的 pod:
cd ios pod install cd ..
第 2 步:针对 iOS/Android 的其他本机设置
一)安卓:
i) android/build.gradle
buildscript { ext { // Enable IMA (Interactive Media Ads) integration with ExoPlayer useExoplayerIMA = true // Enable support for RTSP (Real-Time Streaming Protocol) with ExoPlayer useExoplayerRtsp = true // Enable support for Smooth Streaming with ExoPlayer useExoplayerSmoothStreaming = true // Enable support for DASH (Dynamic Adaptive Streaming over HTTP) with ExoPlayer useExoplayerDash = true // Enable support for HLS (HTTP Live Streaming) with ExoPlayer useExoplayerHls = true } } allprojects { repositories { mavenLocal() google() jcenter() maven { url "$rootDir/../node_modules/react-native-video-cache/android/libs" } } }
此配置在 ExoPlayer 中启用各种流媒体协议(IMA、RTSP、Smooth Streaming、DASH、HLS),并设置存储库以包括本地、Google、JCenter 和用于react-native-video-的自定义 Maven 存储库缓存。
启用的每个功能都会增加 APK 的大小,因此仅启用您需要的功能。默认启用的功能有:useExoplayerSmoothStreaming、useExoplayerDash、useExoplayerHls
ii) AndroidManifest.xml
<application ... android:largeHeap="true" android:hardwareAccelerated="true">
这种组合确保应用程序有足够的内存来处理大型数据集(通过largeHeap),并可以高效地渲染图形(通过硬件加速),从而带来更流畅、更稳定的用户体验。但是,两者的使用都应考虑应用程序的性能及其功能的特定需求。
b) iOS:
i) 在 ios/your_app/AppDelegate.mm 里面 didFinishLaunchingWithOptions 添加:
#import <AVFoundation/AVFoundation.h> - (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { self.moduleName = @"your_app"; // --- You can add your custom initial props in the dictionary below. [[AVAudioSession sharedInstance] setCategory:AVAudioSessionCategoryPlayback error:nil]; // --- They will be passed down to the ViewController used by React Native. self.initialProps = @{}; return [super application:application didFinishLaunchingWithOptions:launchOptions]; }
即使应用程序处于后台或静音模式,也确保音频继续播放
ii) ios/Podfile:
... # ENABLE THIS FOR CACHEING THE VIDEOS platform :ios, min_ios_version_supported prepare_react_native_project! # -- ENABLE THIS FOR CACHEING THE VIDEOS $RNVideoUseVideoCaching=true ... target 'your_app' do config = use_native_modules! # ENABLE THIS FOR CACHEING THE VIDEOS pod 'SPTPersistentCache', :modular_headers => true; pod 'DVAssetLoaderDelegate', :modular_headers => true; ...
此配置通过添加处理缓存和资源加载的特定 CocoaPod(SPTPercientCache 和 DVAssetLoaderDelegate)来在 iOS 项目中启用视频缓存。标志 $RNVideoUseVideoCaching=true 表示项目应该使用这些缓存功能。此设置通过减少重新获取视频文件的需要来提高视频播放的性能。
2、使用方法:
import Video from 'react-native-video'; import convertToProxyURL from 'react-native-video-cache'; <Video // Display a thumbnail image while the video is loading poster={item.thumbUri} // Specifies how the thumbnail should be resized to cover the entire video area posterResizeMode="cover" // Sets the video source URI if the video is visible; otherwise, it avoids loading the video source={ isVisible ? { uri: convertToProxyURL(item.videoUri) } // Converts the video URL to a proxy URL if visible : undefined // Avoid loading the video if not visible } // Configures buffer settings to manage video loading and playback bufferConfig={{ minBufferMs: 2500, // Minimum buffer before playback starts maxBufferMs: 3000, // Maximum buffer allowed bufferForPlaybackMs: 2500, // Buffer required to start playback bufferForPlaybackAfterRebufferMs: 2500, // Buffer required after rebuffering }} // Ignores the silent switch on the device, allowing the video to play with sound even if the device is on silent mode ignoreSilentSwitch={'ignore'} // Prevents the video from playing when the app is inactive or in the background playWhenInactive={false} playInBackground={false} // Disables the use of TextureView, which can optimize performance but might limit certain effects useTextureView={false} // Hides the default media controls provided by the video player controls={false} // Disables focus on the video, which is useful for accessibility and UI focus management disableFocus={true} // Applies the defined styles to the video container style={styles.videoContainer} // Pauses the video based on the isPaused state paused={isPaused} // Repeats the video playback indefinitely repeat={true} // Hides the shutter view (a black screen that appears when the video is loading or paused) hideShutterView // Sets the minimum number of retry attempts when the video fails to load minLoadRetryCount={5} // Ensures the video maintains the correct aspect ratio by covering the entire view area resizeMode="cover" // Sets the shutter color to transparent, so the shutter view is invisible shutterColor="transparent" // Callback function that is triggered when the video is ready for display onReadyForDisplay={handleVideoLoad} />
3. 优化技巧
为了确保视频播放流畅:
- 使用 CDN:将视频托管在 CDN(内容交付网络)上以加快加载速度。
- 自适应流媒体:实施自适应流媒体(HLS 或 DASH)以根据网络条件调整视频质量。
- 预加载视频:预加载视频以避免播放过程中出现缓冲。
- 优化视频文件:在不损失质量的情况下压缩视频文件,以减少加载时间。
结论
react-native-video 库是一个强大的工具,用于向 React Native 应用程序添加视频功能。凭借其广泛的配置选项和事件处理功能,您可以为用户创建流畅且定制的视频流体验。无论您需要一个基本播放器还是完全定制的播放器,react-native-video 都能满足您的需求。
以上是使用 React Native 实现流畅的视频流的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
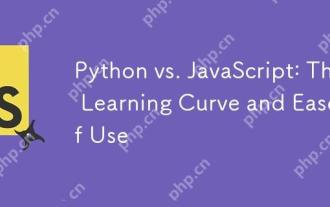
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
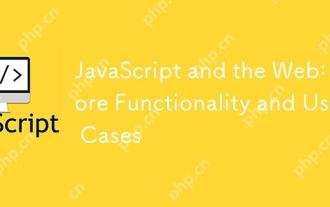
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
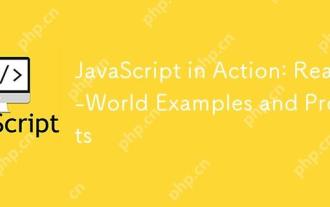
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
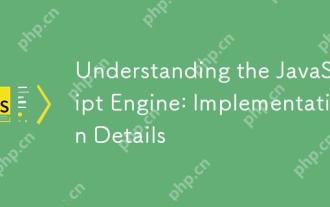
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
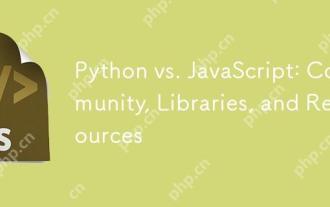
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
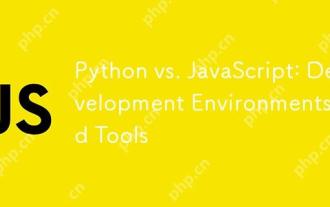
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
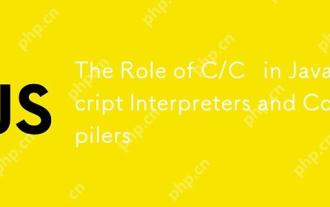
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。

Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
