Go 语言概述
介绍
Go,也称为 Golang,是一种静态类型、编译型编程语言,旨在简单和高效。它由 Robert Griesemer、Rob Pike 和 Ken Thompson 在 Google 开发,于 2009 年公开宣布,源于对一种编程语言的需求,该语言可以提高软件开发的生产力,同时解决 C++ 和 Java 等现有系统的缺点[5][ 7]。
Go 的设计哲学侧重于简单、高效和并发等关键原则。这些原则反映在 Go 的简洁语法及其对并发编程的强大支持中,允许开发人员构建可扩展的应用程序,而无需传统线程模型所带来的复杂性。该语言的并发模型以 goroutine 和通道为特色,是一个突出的方面,促进了可以有效地同时处理多个任务的高性能软件[3][18]。
在现代软件开发的背景下,Go 获得了极大的普及,特别是在云服务和分布式系统中。其简单的方法和强大的并发功能使其成为开发需要高可扩展性的微服务和应用程序的首选。与 Java 或 Python 等其他编程语言相比,Go 提供了卓越的性能和强大的标准库,使其特别适合当今技术环境中的高效应用程序[1][12][17]。
Go 入门
安装指南
要开始 Go 编程,第一步是在系统上下载并安装 Go 编程语言。 Go 官方网站提供了针对 Windows、macOS 和 Linux 的平台特定安装说明。对于 Windows,您可以访问官方安装程序页面,下载 .msi 文件,然后运行它来设置 Go。对于 macOS,您可以通过命令brew install go 使用 Homebrew,或者从 Go 网站下载软件包。对于 Linux,用户可以通过包管理器安装或下载 tarball 并将其解压到 /usr/local。安装后,您需要确保 PATH 环境变量设置正确,以允许从终端或命令提示符访问 Go 命令。
了解 Go 工作区和目录结构
了解 Go 工作区对于有效组织 Go 项目至关重要。在 Go 中,两个环境变量起着重要作用:GOPATH 和 GOROOT。 GOROOT 表示 Go SDK 的安装位置,而 GOPATH 是您自己的工作和 Go 项目所在的位置。 GOPATH目录通常包含三个子目录:src用于源文件,pkg用于编译后的包,bin用于编译后的可执行文件。通过为每个项目创建单独的目录来组织 GOPATH 中的项目,可以实现更好的结构和更轻松的管理。
第一个 Go 程序:编写、编译和运行一个简单的“Hello, World!”应用
设置 Go 环境后,您就可以编写第一个 Go 程序了。打开您喜欢的文本编辑器并创建一个名为 hello.go 的新文件。在此文件中,写入以下代码:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
此代码定义了一个简单的 Go 程序,用于打印“Hello, World!”到控制台。要编译并运行该程序,请导航到保存 hello.go 的目录并执行命令 go run hello.go。如果一切设置正确,您应该看到“Hello, World!”的输出。在您的终端中。当您深入研究 Go 语言时,这个基本程序可以作为理解 Go 语法和结构的构建块 [2][5][11]。
基本语法和结构
在Go编程中,基本语法被设计得简单直观。它使用一组保留关键字,例如 func、var、if 和 for,这些关键字对于定义函数、变量和控制结构至关重要。正确的缩进对于可读性至关重要,因为 Go 强制执行标准格式来增强代码的清晰度。最佳实践包括使用空格而不是制表符以及在整个代码库中保持一致的缩进级别,以确保其他开发人员可以轻松阅读和理解代码 [1][6]。
Go 支持各种内置数据类型,包括 int、float、string 和 bool。例如,int 用于表示整数值,而 float64 可以表示十进制数。除了内置类型之外,Go 还具有允许更复杂数据结构的复合类型。数组是固定大小的集合,切片提供动态集合,映射是键值存储,结构用于对相关数据进行分组。例如,定义一个简单的结构可以如下完成:
type Person struct { Name string Age int }
This struct can then be instantiated and used in the program [2][5].
Control structures in Go include conditional statements such as if, else, and switch, along with looping constructs like for loops and the range clause for iterating over collections. An example of an if statement is:
if age >= 18 { fmt.Println("You are an adult.") }
For looping, a for statement can iterate through a slice of numbers like this:
numbers := []int{1, 2, 3, 4, 5} for i, num := range numbers { fmt.Println(i, num) }
These control structures allow programmers to implement logic and handle data effectively in their applications [3][4][7].
Functions and Packages
Defining and calling functions in Go is a fundamental aspect of programming in this language. Functions in Go can accept parameters and return values, making them versatile. A function can be declared with various parameter types and can also return multiple values, which is a distinctive feature of Go[1]. Variadic functions allow for a variable number of arguments, which enhances flexibility in function definitions. Additionally, anonymous functions in Go are functions without a name, allowing for concise and functional programming styles[1][2]. Understanding the scope and lifetime of variables is crucial as well; local variables are confined to the function's scope, while global variables persist throughout the program's runtime[3].
The importance of packages in Go cannot be overstated as they facilitate code reuse and organization. Packages help in structuring code, making large programs more manageable by grouping related code. Go encourages the use of standard library packages, which contain a wealth of pre-built functionalities that improve development efficiency. Examples of commonly used standard library packages include fmt for formatted I/O and net/http for web capabilities[4]. Best practices for creating custom packages include maintaining a clear naming convention, avoiding circular dependencies, and adhering to Go's convention of using lowercase names for package imports[5]. Understanding how to import and use these packages effectively is essential for writing Go code that is clean, organized, and efficient.
Error Handling in Go
Go programming language incorporates a unique approach to error handling that promotes clarity and robustness in software development. Its error interface is a fundamental aspect, allowing developers to define custom error types and provide meaningful context. By convention, functions that can encounter an error return a value paired with an error type, enhancing the ability to detect issues immediately. This design significantly simplifies error checking, as developers are encouraged to inspect the error return value right after function calls[1][3].
In order to return and check errors effectively, Go employs specific techniques. After executing a function that returns an error, it is essential to verify whether the error is nil. For example:
result, err := someFunc() if err != nil { // handle the error }
This conditional approach allows for seamless error handling without relying on exceptions, aligning with Go's design philosophies of simplicity and clarity[2][5].
To ensure robust error handling, best practices emphasize gracefully handling errors and incorporating logging techniques. This includes consistent error messages that provide context and assistance for debugging, as well as logging errors at appropriate severity levels. Developers are encouraged to use structured logging tools to standardize the format of error logs, making it easier to track issues across the application[4][6]. By adopting these conventions, developers can create more resilient applications in Go that can better withstand unexpected behavior and facilitate easier maintenance.
Concurrency in Go
Concurrency is a fundamental feature of the Go programming language, primarily implemented through Goroutines. Goroutines are lightweight threads managed by the Go runtime, allowing developers to initiate concurrent execution of functions easily. Creating a Goroutine is as simple as prefixing a function call with the go keyword, which allows the function to run simultaneously with other Goroutines[1]. This model provides significant advantages over traditional threading models, including reduced overhead as Goroutines are cheaper to create and maintain, thus enhancing application performance and scalability[2].
Channels in Go are another essential component that facilitates communication between Goroutines. Channels act as conduits, enabling the transfer of data safely and efficiently. A channel must be created before it's used, and it can be defined using the make function. Go offers two types of channels: buffered and unbuffered. Unbuffered channels require both sending and receiving Goroutines to be ready simultaneously, whereas buffered channels allow for some level of asynchronous communication, accommodating multiple sends before blocking[3].
为了确保对共享资源的安全访问,Go 提供了先进的同步技术,例如 WaitGroups 和 Mutexes。 WaitGroup 用于等待 Goroutines 集合完成执行,而 Mutexes 用于管理对代码关键部分的并发访问,防止竞争条件。对于开发人员来说,了解避免竞争条件的重要性至关重要,因为它们可能会导致并发应用程序中出现不可预测的行为和难以跟踪的错误。通过利用这些同步工具,开发人员可以在 Go[2][3] 中编写健壮且高效的并发程序。
Go 中的面向对象编程
Go 采用了一种不同于传统 OOP 语言的独特的面向对象编程 (OOP) 方法。 Go 不依赖类和继承,而是利用 structs 和 interfaces 来封装数据和行为。结构是对相关字段进行分组的用户定义类型,而接口定义了类型必须实现的一组方法签名,从而实现多态性。这种设计强调组合而不是继承,允许开发人员通过组合更简单的类型来构建复杂的类型,而不是创建复杂的类层次结构。这种区别有助于 Go 在代码设计中保持简单性和可读性[1][2][6]。
在 Go 中实现方法非常简单。方法是具有接收器类型的函数,允许它们与结构关联。通过在结构上定义方法,开发人员可以将行为与数据一起封装,从而遵循面向对象的范例。另一方面,接口通过促进代码的灵活性和模块化发挥着至关重要的作用。任何实现接口所需方法的类型都可以说是实现了该接口,这允许泛化并使代码更具适应性[3][5]。这种 OOP 方法符合 Go 的设计理念,优先考虑简单性和效率,同时提供模块化编程的好处。
测试和文档
测试是软件开发的一个基本方面,可确保代码的可靠性和功能性。不同类型的测试有不同的目的:单元测试侧重于单个组件,而集成测试则评估系统的不同部分如何协同工作。在 Go 中,由于其内置的测试包,测试非常简单。该软件包允许开发人员高效地创建和运行测试,使用 go test 等命令来执行测试脚本并验证代码的行为是否符合预期[3][7]。
在 Go 中编写测试时,遵循基准测试和验证的最佳实践至关重要。测试包提供了用于测量性能和确保代码质量的实用程序。例如,您可以通过编写以Benchmark开头并使用testing.B类型的函数来定义基准测试,从而允许开发人员有效地评估其代码的速度和效率[1][2]。
文档在 Go 编程中同样重要,因为它增强了代码的可维护性和可用性。利用代码中的注释以及 GoDoc 等工具,开发人员可以直接从源代码生成全面的文档。 GoDoc 解析包声明和导出实体之前的注释,为与代码库交互的任何人提供清晰、用户友好的界面。对文档的关注不仅有助于个人理解,还支持更广泛的开发者社区内的协作[8][5][12]。
最佳实践和技巧
常见的 Go 习惯用法和编码约定
要在 Go 中编写惯用且可维护的代码,开发人员应遵守几个关键约定。首先,必须使用正确的命名约定,其中变量名称应该具有描述性且简洁。例如,使用驼峰命名法表示多字标识符(例如 userCount)符合 Go 约定。此外,开发人员应该利用Go强大的类型系统来定义清晰的接口和结构类型,促进代码重用并降低复杂性。在错误处理方面,建议从函数返回错误作为最后一个返回值,以便在函数调用后进行简单的错误检查[1][3][5]。
性能优化策略
识别Go应用程序中的性能瓶颈可以显着提高软件的整体效率。分析工具(例如 Go 的内置 pprof)可用于检测资源密集型函数并缩小需要优化的范围。此外,开发人员应尽可能通过重用对象来最大限度地减少内存分配和垃圾收集暂停。并发是 Go 的另一个强大功能,有效地使用 Goroutines 和通道可以通过在并行执行期间更好地利用系统资源来提高性能[2][4][8]。
进一步学习的资源
对于那些想要加深对 Go 的理解的人,推荐了一些优秀的资源。 Alan A. A. Donovan 和 Brian W. Kernighan 所著的《Go 编程语言》等书籍提供了对该语言的设计和功能的全面见解。 Coursera 和 Udemy 等平台的在线课程以及 DigitalOcean 和 W3Schools 等网站上提供的实用教程提供了结构化的学习路径。随着学习者继续练习和完善他们的 Go 编程技能,Reddit 或官方 Go Wiki 等论坛和网站上的社区参与也可以提供宝贵的支持和见解[10][11][19][20]。
结论
在本指南中,我们探索了 Go 编程语言,深入研究了它的定义、历史和设计理念,强调简单、高效和并发。在各个部分中,我们强调了 Go 的独特功能如何使其成为现代软件开发的有力竞争者,特别是在云服务和分布式系统中。现在您已经获得了基础知识,定期练习 Go 编码以增强您的技能并加深您的理解至关重要。
下一步,考虑深入研究诸如构建并发应用程序、利用 Go 广泛的标准库或为开源 Go 项目做出贡献以扩展您的经验等领域。有许多资源可以支持您的学习之旅,包括 Go 官方文档平台上的教程 [11]、编码挑战以及可以与其他 Go 开发人员联系的社区论坛 [4][8]。
最后,Go 的未来看起来很光明,因为它因其在创建高效且可扩展的应用程序方面的强大功能而在开发人员中越来越受欢迎。参与 Go 社区不仅可以提供支持,还可以帮助您及时了解编程领域不断发展的最佳实践和创新。迎接挑战,享受进入 Go 编程世界的旅程!
参考
- (PDF) Go 编程语言:概述 - ResearchGate
- Go Wiki:研究论文 - Go 编程语言
- 在实践中使用 Go 编程语言 - ResearchGate
- 过去 3 年来帮助我学习 golang 的一些资源...
- Go 编程语言和环境
- [PDF] Go 编程语言
- Go 编程语言(简介)-GeeksforGeeks
- 快速深入学习 Golang 的总体规划(2024 版)
- Go被推荐为第一编程语言? - 去论坛
- Go 编程语言和环境 - ACM 数字图书馆
- 教程 - Go 编程语言
- Go 编程语言 | IEEE 期刊和杂志
- 数据科学和 Go 编程语言 - Northwestern SPS
- karanpratapsingh/learn-go:掌握基础知识并...... - GitHub
- 如何在 Go 中编码 |数字海洋
- 学习 Golang 的最佳方式是什么? - 知乎
- Go 编程语言(Golang)真正有什么用处?
- 学习围棋 - Chico Pimentel - Medium
- dariubs/GoBooks:Golang 书籍列表 - GitHub
- Go 教程 - W3Schools
以上是Go 语言概述的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
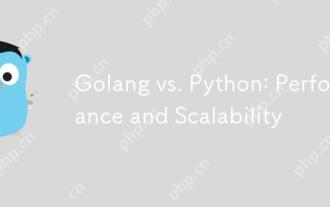
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
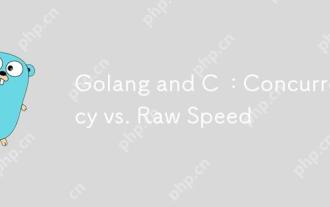
Golang在并发性上优于C ,而C 在原始速度上优于Golang。1)Golang通过goroutine和channel实现高效并发,适合处理大量并发任务。2)C 通过编译器优化和标准库,提供接近硬件的高性能,适合需要极致优化的应用。
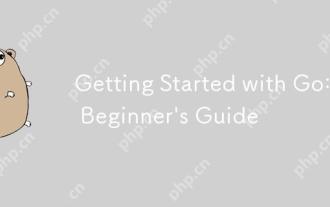
goisidealforbeginnersandsubableforforcloudnetworkservicesduetoitssimplicity,效率和concurrencyFeatures.1)installgromtheofficialwebsitealwebsiteandverifywith'.2)
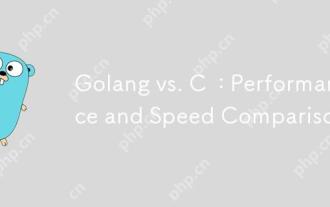
Golang适合快速开发和并发场景,C 适用于需要极致性能和低级控制的场景。1)Golang通过垃圾回收和并发机制提升性能,适合高并发Web服务开发。2)C 通过手动内存管理和编译器优化达到极致性能,适用于嵌入式系统开发。
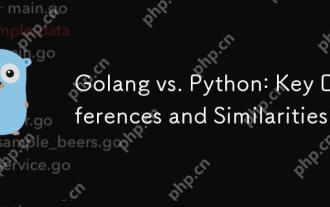
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。
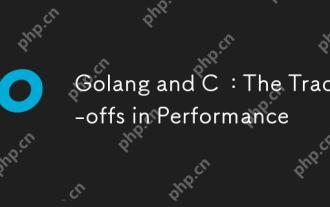
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
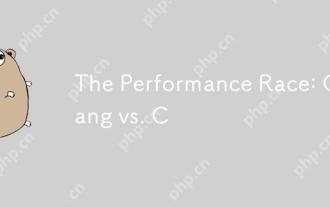
Golang和C 在性能竞赛中的表现各有优势:1)Golang适合高并发和快速开发,2)C 提供更高性能和细粒度控制。选择应基于项目需求和团队技术栈。
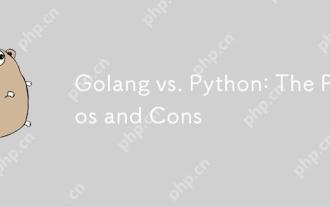
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
