现代开发必须了解的 JavaScript ESFeatures
JavaScript 不断发展,随着 ES13 (ECMAScript 2022) 的引入,开发人员应该了解一些新功能,以便编写更高效、更现代的代码。在本文中,我们将深入探讨 ES13 中十个最有影响力的功能,这些功能可以改善您的开发工作流程。
1. 顶级等待
ES13 之前:
以前,您只能在异步函数中使用await。这意味着如果您需要使用await,则必须将代码包装在异步函数中,即使模块的其余部分不需要它。
示例:
// Without top-level await (Before ES13) async function fetchData() { const data = await fetch('/api/data'); return data.json(); } fetchData().then(console.log);
ES13特点:
使用 ES13,您现在可以在模块的顶层使用 wait,从而无需额外的异步包装函数。
// With top-level await (ES13) const data = await fetch('/api/data'); console.log(await data.json());
2. 私有实例方法和访问器
ES13 之前:
在 ES13 之前,JavaScript 类没有真正的私有字段或方法。开发人员经常使用下划线或闭包等命名约定来模拟隐私,但这些方法并不是真正的隐私。
示例:
// Simulating private fields (Before ES13) class Person { constructor(name) { this._name = name; // Conventionally "private" } _getName() { return this._name; } greet() { return `Hello, ${this._getName()}!`; } } const john = new Person('John'); console.log(john._getName()); // Accessible, but intended to be private
ES13特点:
ES13 引入了真正的私有实例方法和使用 # 前缀的访问器,确保它们无法在类外部访问。
// Private instance methods and fields (ES13) class Person { #name = ''; constructor(name) { this.#name = name; } #getName() { return this.#name; } greet() { return `Hello, ${this.#getName()}!`; } } const john = new Person('John'); console.log(john.greet()); // Hello, John! console.log(john.#getName()); // Error: Private field '#getName' must be declared in an enclosing class
3. 静态类字段和方法
ES13 之前:
在 ES13 之前,静态字段和方法通常在类体之外定义,导致代码内聚力较差。
示例:
// Static fields outside class body (Before ES13) class MathUtilities {} MathUtilities.PI = 3.14159; MathUtilities.calculateCircumference = function(radius) { return 2 * MathUtilities.PI * radius; }; console.log(MathUtilities.PI); // 3.14159 console.log(MathUtilities.calculateCircumference(5)); // 31.4159
ES13特点:
ES13 允许您直接在类体内定义静态字段和方法,从而提高可读性和组织性。
// Static fields and methods inside class body (ES13) class MathUtilities { static PI = 3.14159; static calculateCircumference(radius) { return 2 * MathUtilities.PI * radius; } } console.log(MathUtilities.PI); // 3.14159 console.log(MathUtilities.calculateCircumference(5)); // 31.4159
4. 逻辑赋值运算符
ES13 之前:
逻辑运算符(&&、||、??)和赋值通常在详细语句中手动组合,导致代码更加复杂。
示例:
// Manually combining logical operators and assignment (Before ES13) let a = 1; let b = 0; a = a && 2; // a = 2 b = b || 3; // b = 3 let c = null; c = c ?? 4; // c = 4 console.log(a, b, c); // 2, 3, 4
ES13特点:
ES13 引入了逻辑赋值运算符,它以简洁的语法将逻辑运算与赋值结合起来。
// Logical assignment operators (ES13) let a = 1; let b = 0; a &&= 2; // a = a && 2; // a = 2 b ||= 3; // b = b || 3; // b = 3 let c = null; c ??= 4; // c = c ?? 4; // c = 4 console.log(a, b, c); // 2, 3, 4
5. WeakRefs 和 FinalizationRegistry
ES13 之前:
JavaScript 本身不支持弱引用和终结器,这使得在某些情况下管理资源变得困难,尤其是处理昂贵对象的大型应用程序。
示例:
// No native support for weak references (Before ES13) // Developers often had to rely on complex workarounds or external libraries.
ES13特点:
ES13引入了WeakRef和FinalizationRegistry,为弱引用和垃圾回收后的清理任务提供原生支持。
// WeakRefs and FinalizationRegistry (ES13) let obj = { name: 'John' }; const weakRef = new WeakRef(obj); console.log(weakRef.deref()?.name); // 'John' obj = null; // obj is eligible for garbage collection setTimeout(() => { console.log(weakRef.deref()?.name); // undefined (if garbage collected) }, 1000); const registry = new FinalizationRegistry((heldValue) => { console.log(`Cleanup: ${heldValue}`); }); registry.register(obj, 'Object finalized');
6. 私人领域的人体工学品牌检查
ES13 之前:
检查对象是否具有私有字段并不简单,因为私有字段本身并不支持。开发人员必须依赖解决方法,例如检查公共属性或使用 instanceof 检查。
示例:
// Checking for private fields using workarounds (Before ES13) class Car { constructor() { this.engineStarted = false; // Public field } startEngine() { this.engineStarted = true; } static isCar(obj) { return obj instanceof Car; // Not reliable for truly private fields } } const myCar = new Car(); console.log(Car.isCar(myCar)); // true
ES13特点:
使用 ES13,您现在可以使用 # 语法直接检查对象是否具有私有字段,使其更简单、更可靠。
// Ergonomic brand checks for private fields (ES13) class Car { #engineStarted = false; startEngine() { this.#engineStarted = true; } static isCar(obj) { return #engineStarted in obj; } } const myCar = new Car(); console.log(Car.isCar(myCar)); // true
7. Array.prototype.at()
ES13 之前:
使用带索引的括号表示法访问数组中的元素,对于负索引,您必须手动计算位置。
示例:
// Accessing array elements (Before ES13) const arr = [1, 2, 3, 4, 5]; console.log(arr[arr.length - 1]); // 5 (last element)
ES13特点:
at() 方法允许您更直观地使用正索引和负索引访问数组元素。
// Accessing array elements with `at()` (ES13) const arr = [1, 2, 3, 4, 5]; console.log(arr.at(-1)); // 5 (last element) console.log(arr.at(0)); // 1 (first element)
8. Object.hasOwn()
ES13 之前:
要检查对象是否有自己的属性(不是继承的),开发人员通常使用 Object.prototype.hasOwnProperty.call() 或 obj.hasOwnProperty()。
示例:
// Checking own properties (Before ES13) const obj = { a: 1 }; console.log(Object.prototype.hasOwnProperty.call(obj, 'a')); // true console.log(obj.hasOwnProperty('a')); // true
ES13特点:
新的 Object.hasOwn() 方法简化了此检查,提供了更简洁和可读的语法。
// Checking own properties with `Object.hasOwn()` (ES13) const obj = { a: 1 }; console.log(Object.hasOwn(obj, 'a')); // true
9. Object.fromEntries()
ES13 之前:
将键值对(例如,从 Map 或数组)转换为对象需要循环和手动构造。
Example:
// Creating an object from entries (Before ES13) const entries = [['name', 'John'], ['age', 30]]; const obj = {}; entries.forEach(([key, value]) => { obj[key] = value; }); console.log(obj); // { name: 'John', age: 30 }
ES13 Feature:
Object.fromEntries() simplifies the creation of objects from key-value pairs.
// Creating an object with `Object.fromEntries()` (ES13) const entries = [['name', 'John'], ['age', 30]]; const obj = Object.fromEntries(entries); console.log(obj); // { name: 'John', age: 30 }
10. Global This in Modules
Before ES13:
The value of this in the top level of a module was undefined, leading to confusion when porting code from scripts to modules.
Example:
// Global `this` (Before ES13) console.log(this); // undefined in modules, global object in scripts
ES13 Feature:
ES13 clarifies that the value of this at the top level of a module is always undefined, providing consistency between modules and scripts.
// Global `this` in modules (ES13) console.log(this); // undefined
These ES13 features are designed to make your JavaScript code more efficient, readable, and maintainable. By integrating these into your development practices, you can leverage the latest advancements in the language to build modern, performant applications.
以上是现代开发必须了解的 JavaScript ESFeatures的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
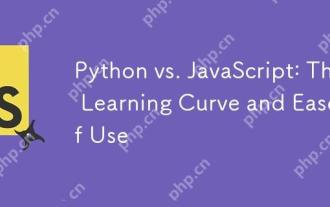
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
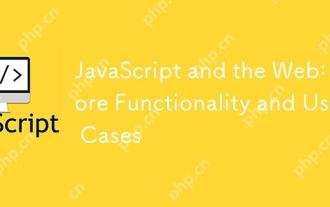
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
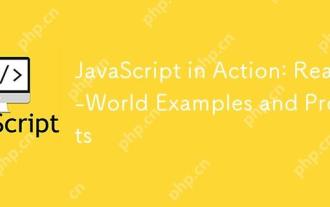
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
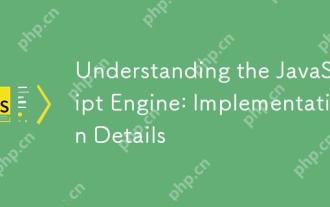
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
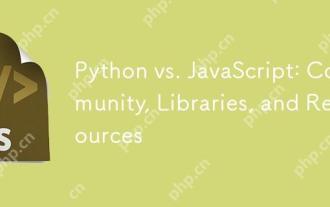
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
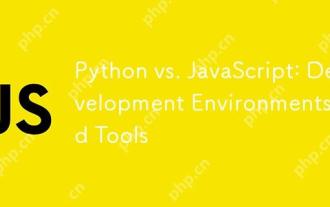
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
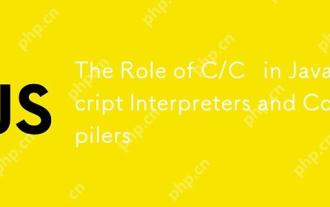
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。
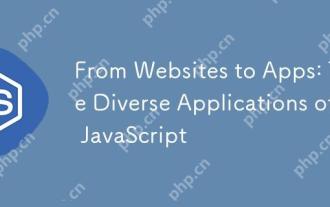
JavaScript在网站、移动应用、桌面应用和服务器端编程中均有广泛应用。1)在网站开发中,JavaScript与HTML、CSS一起操作DOM,实现动态效果,并支持如jQuery、React等框架。2)通过ReactNative和Ionic,JavaScript用于开发跨平台移动应用。3)Electron框架使JavaScript能构建桌面应用。4)Node.js让JavaScript在服务器端运行,支持高并发请求。
