PHP 中的平方根
Aug 29, 2024 pm 01:12 PM计算其他根,例如数字的 n 次方根或数字的立方根,类似地,我们需要在 PHP 中求数字的平方根。我们通过使用不同的函数(如 pow()、log() 等)来计算这些根。
广告 该类别中的热门课程 PHP 开发人员 - 专业化 | 8 门课程系列 | 3次模拟测试开始您的免费软件开发课程
网络开发、编程语言、软件测试及其他
在 PHP 这样的编程语言中,与内置函数一起使用时计算平方根很简单。这个函数就是sqrt()。我们还将了解如何在不使用 sqrt() 的情况下查找数字的平方根,以及如何使用带有用户输入的表单来计算平方根。
sqrt() 函数用于计算给定数字的平方根。该函数是 PHP 中使用的内置数学函数,如 pow()、rand()、is_nan() 等
平方根逻辑
平方根逻辑的语法和描述在下面详细解释,
语法:
sqrt($num)
其中 $num 是传递给 sqrt 函数的单个参数。
描述:sqrt() 函数计算并返回给定数字的平方根。返回值是float类型。此外,我们对给定函数有不同类型的输入数字,在这些数字上执行平方根函数并计算结果。
这里我们会看到输入的数字可以是正数、负数或小数(浮点数),也可以是零。正数返回正数作为输出,负数返回 NAN(非数字)作为输出,十进制数的平方根是浮点数作为输出,一的平方根是 1。另外,请记住零的平方根为零。
求给定数的平方根
给定数字的平方根如下,
如果输入数字是81,则该数字的平方根将为9。如果输入数字为49,则该数字的平方根将为7,依此类推。
让我们通过一个例子来学习:
我们还将学习使用不同类型的输入求平方根。
示例#1
代码:
<?php // simple example to find how sqrt() function works on numbers echo sqrt(16); echo '<br>'; // output is 4 echo sqrt(7); echo '<br>'; //output is 2.6457513110646 ?>
输出:
在上面的程序中,输出是 4,我们知道 4*4 是 16,因此 16 的平方根是 4。在计算 7 的平方根时,我们看到小数点后有很多位,数字是小数点后的位数取决于用户。
类似于 sqrt 函数,计算给定数字的平方根。为了计算给定数字的任意根,我们使用 pow() 函数,它代表幂。
示例#2
代码 :
<?php // example to calculate any root echo '<br>'.'Result of : pow(16, 1/2) ====== '. pow(16, 1/2); // example to calculate the cube root of 27 echo '<br>'.'Result of : pow(27, 1/3) ====== '. pow(27, 1/3); //example to calculate the fourth root of 12 echo '<br>'.'Result of : pow(12, 1/4) ====== '. pow(12, 1/4); //example to calculate the fifth root of 76 echo '<br>'.'Result of : pow(76, 1/5) ====== '. pow(76, 1/5); //example to calculate the sixth root of 88 echo '<br>'.'Result of : pow(88, 1/6) ====== '. pow(88, 1/6); ?>
输出:
示例#3
代码:
<?php echo '<br>'.'Result of : sqrt(625) ====== '. sqrt(625); echo '<br>'.'Result of : sqrt(49) ====== '. sqrt(49); echo '<br>'.'Result of : sqrt(-36) ====== '. sqrt(-36); echo '<br>'.'Result of : sqrt(0) ====== '. sqrt(0); echo '<br>'.'Result of : sqrt(121) ====== '. sqrt(121); echo '<br>'.'Result of : sqrt(22) ====== '. sqrt(22); echo '<br>'.'Result of : sqrt(12.34) ====== '. sqrt(12.34); echo '<br>'.'Result of : sqrt(-16) ====== '. sqrt(-16); ?>
输出:
示例#4
求用户通过表单输入的数字的平方根:在下面的程序中,我们用 PHP 创建了一个程序来计算用户通过表单输入的数字的平方根。假设用户输入了 16,那么我们可以找到 16 的平方根,并期望结果为 4,如果用户输入 49,我们可以期望结果为 7,依此类推。
此外,我们还使用内置数学函数 sqrt() 来求平方根。
代码:
<!---program to calculate square root of input number using form --> <html> <head> <title>Square root of a number using form</title> </head> <body> <!--- input form with text box ---> <form method="post" action=""> <label>Enter a number</label> <input type="text" name="input" value="" /> <input type="submit" name="submit" value="Submit" /> </form> <?php if(isset($_POST['submit'])) { //storing the number in a variable $input $input = $_POST['input']; //storing the square root of the number in a variable $ans $ans = sqrt($input); //printing the result echo 'The square root of '.$input.'====='.$ans; } ?> </body> </html>
输出 – 1:
输出 – 2:输入 100。
示例#5
不使用内置 sqrt() 函数求数字的平方根:在下面的程序中,我们在 PHP 中创建了一个程序来计算数字的平方根,而不使用内置函数sqrt() 函数。
代码:
function squareroot($input) { //if the input number is 0 then return 0 as result if($input == 0) { return 0; } //if the input number is 1 then return 1 as result if($input == 1) { return 1; } // assigning $input value to a variable $a $a = $input; $b = 1; while($a > $b) { // calculating the middle number $a= ($a + $b)/2; // dividing the input number with the middle number $b = $input/$a; } return $a; } echo '<br>'.'Square root of 0 is '.squareroot(0); echo '<br>'.'Square root of 20 is '.squareroot(20); echo '<br>'.'Square root of 49 is '.squareroot(49); echo '<br>'.'Square root of 81 is '.squareroot(81); echo '<br>'.'Square root of 1 is '.squareroot(1);
输出:
结论
在本文中,我们学习了什么是平方根,以及如何使用和不使用 sqrt()、pow() 等内置函数来计算平方根。 sqrt() 和 pow() 函数的作用是什么?如何在程序中使用它来求平方根?我们学习了如何对数字、浮点数、负数等进行平方根。我们还学习了如何使用表单通过用户定义的输入计算平方根。
以上是PHP 中的平方根的详细内容。更多信息请关注PHP中文网其他相关文章!

热门文章

热门文章

热门文章标签

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
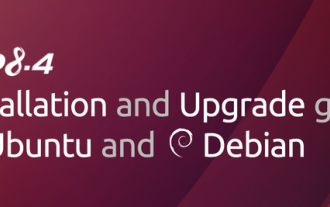
适用于 Ubuntu 和 Debian 的 PHP 8.4 安装和升级指南
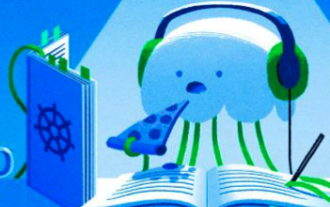
如何设置 Visual Studio Code (VS Code) 进行 PHP 开发
