Java 中的私有
Java中的关键字“private”用于建立java代码块中类、方法或变量的可访问性限制。如果一个类、方法或变量在程序中被称为私有,则意味着特定的类、方法或变量不能被该类或方法的外部访问,这与公共方法不同。 Private 关键字通常在 Java 中完全封装的类中使用。
私有关键字在 Java 中如何工作?
Java 中的私有关键字在特定类中工作。在课堂之外无法访问它。它在类/类和接口/接口之外不起作用。如果类的成员是 PRIVATE 并且在完全封装的类中也是如此,则 Private 关键字效果很好。 Private 关键字或变量或方法也可以使用某些访问修饰符来覆盖子类/类,以调用类外部的 PRIVATE METHOD。这样,私有关键字也可以仅使用私有访问修饰符在类之外工作。
开始您的免费软件开发课程
网络开发、编程语言、软件测试及其他
示例
下面是一些私有修饰符的示例,如下:
示例#1
这里我们以 Private Access Modifier 为例,由于从类 AB 访问私有数据成员,该示例显示编译错误,如下例所示。私有方法或私有成员只能在特定类中访问。
代码:
class AB{ private double number = 100; private int squares(int a){ return a*a; } } public class Main{ public static void main(String args[]){ AB obj = new AB(); System.out.println(obj.number); System.out.println(obj.squares(10)); } }
输出:
示例#2
这是说明在以下程序中使用 PRIVATE 关键字的示例:
代码:
class Emp{ private int id1; private String name14; private int age14; public int getId1() { return id1; } public void setId(int id1) { this.id1 = id1; } public String getName14() { return name14; } public void setName14(String name14) { this.name14 = name14; } public int getAge14() { return age14; } public void setAge14(int age14) { this.age14 = age14; } } public class Main{ public static void main(String args[]){ Emp e=new Emp(); e.setId(1473); e.setName14("Pavan Kumar Sake"); e.setAge14(24); System.out.println(e.getId1()+" "+e.getName14()+" "+e.getAge14()); } }
输出:
示例 #3
在此示例中,您可以看到如何使用默认的访问修饰符将 PRIVATE METHOD 覆盖到子类。我们甚至不允许从子类调用父类方法。
代码:
class ABC{ private void msg() { System.out.println("Hey Buddy this is the parent class method"); //Output of the Private Method } } public class Main extends ABC{ // Private method is overridden using the access modifier void msg() { System.out.println("Buddy this is the child class method"); } public static void main(String args[]){ Main obj=new Main(); obj.msg(); } }
输出:
示例#4
在此示例中,我说明不能在类外部调用/调用 PRIVATE METHOD。现在,私有方法通过更改类运行时行为从外部类调用。
代码:
import java.lang.reflect.Method; class ABC { private void display() { System.out.println("Hey Now private method is invoked/Called"); } } public class Main{ public static void main(String[] args)throws Exception{ Class d = Class.forName("ABC"); Object p= d.newInstance(); Method n =d.getDeclaredMethod("display", null); n.setAccessible(true); n.invoke(p, null); } }
输出:
示例#5
这是 Java 编程语言中私有方法和字段的示例。这里私有方法在编译时使用静态绑定,甚至不能被覆盖。不要与 Private 变量输出混淆,因为 Private 变量实际上可以在内部类内部访问。如果在类外部调用 PRIVATE 变量,编译器肯定会产生错误。
代码:
public class Main { private String i_m_private1 = " \n Hey Buddy I am a private member and i am not even accessible outside of this Class"; private void privateMethod1() { System.out.println("Outer Class Private Method"); } public static void main(String args[]) { Main outerClass = new Main(); NestedClass nestc = outerClass.new NestedClass(); nestc.showPrivate(); //This syntax shows private method/methods are accessible inside the class/inner class. outerClass = nestc; nestc.privateMethod1(); //It don't call/invoke private method from the inner class because // you can not override the private method inside the inner class. } class NestedClass extends Main { public void showPrivate() { System.out.println("Now we are going to access Outer Class Private Method: " + i_m_private1); privateMethod1(); } private void privateMethod1() { System.out.println("Nested Class's Private Method"); } } }
输出:
优点
下面我们将解释在 Java 中使用私有方法/字段的优点。
- 它也可以应用于Java编程语言中的方法、字段和内部类。
- 我们不能将 private 分配给外部接口或类。
- 在 Java 编程语言中,顶级类始终不能是 Private。
- 当然,我们知道私有变量或私有方法不能在 java 类之外访问,但可以通过使用 setAccessible(true) 的反射并通过更改 PRIVATE 可见性以另一种方式访问它们。
- 在 Java 编程语言中,此方法不能被重写,即使不在内部类中也是如此。
- PRIVATE 成员允许 Java 虚拟机和编译器对其进行优化以获得更好的性能。
Java 私有构造函数的规则和规定
以下是您应该了解的一些私人规则和规定。
- 如果类包含私有构造函数,则无法从外部类创建该特定类的任何对象。
- 您不应该在类之外访问私有关键字。如果您尝试,它将产生该行为的编译错误。为此,您必须修改代码。
- 在该 java 语言中顶级类不能是私有的。
结论
主题即将结束,我们实际上很高兴知道在 Java 中使用 Private Keyword 是多么有用和简单。在本文中,我希望您了解 private 关键字、私有变量、访问修饰符、私有构造函数,以及如何在程序中使用这些私有关键字。
以上是Java 中的私有的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
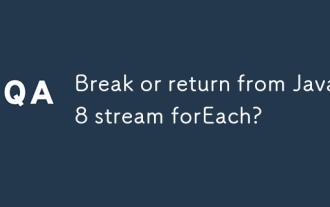
Java 8引入了Stream API,提供了一种强大且表达力丰富的处理数据集合的方式。然而,使用Stream时,一个常见问题是:如何从forEach操作中中断或返回? 传统循环允许提前中断或返回,但Stream的forEach方法并不直接支持这种方式。本文将解释原因,并探讨在Stream处理系统中实现提前终止的替代方法。 延伸阅读: Java Stream API改进 理解Stream forEach forEach方法是一个终端操作,它对Stream中的每个元素执行一个操作。它的设计意图是处
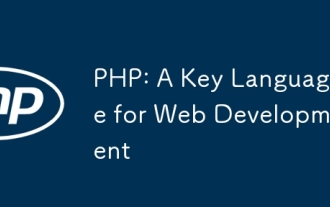
PHP是一种广泛应用于服务器端的脚本语言,特别适合web开发。1.PHP可以嵌入HTML,处理HTTP请求和响应,支持多种数据库。2.PHP用于生成动态网页内容,处理表单数据,访问数据库等,具有强大的社区支持和开源资源。3.PHP是解释型语言,执行过程包括词法分析、语法分析、编译和执行。4.PHP可以与MySQL结合用于用户注册系统等高级应用。5.调试PHP时,可使用error_reporting()和var_dump()等函数。6.优化PHP代码可通过缓存机制、优化数据库查询和使用内置函数。7
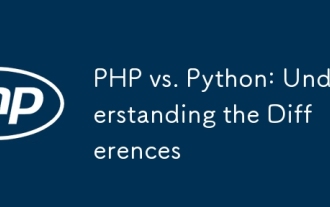
PHP和Python各有优势,选择应基于项目需求。1.PHP适合web开发,语法简单,执行效率高。2.Python适用于数据科学和机器学习,语法简洁,库丰富。
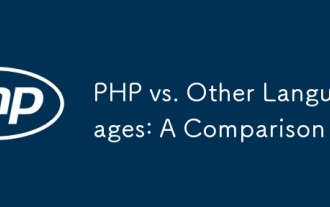
PHP适合web开发,特别是在快速开发和处理动态内容方面表现出色,但不擅长数据科学和企业级应用。与Python相比,PHP在web开发中更具优势,但在数据科学领域不如Python;与Java相比,PHP在企业级应用中表现较差,但在web开发中更灵活;与JavaScript相比,PHP在后端开发中更简洁,但在前端开发中不如JavaScript。
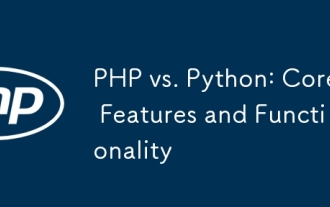
PHP和Python各有优势,适合不同场景。1.PHP适用于web开发,提供内置web服务器和丰富函数库。2.Python适合数据科学和机器学习,语法简洁且有强大标准库。选择时应根据项目需求决定。
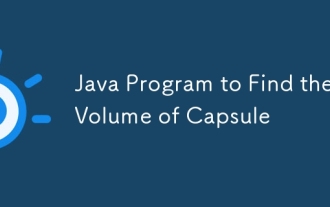
胶囊是一种三维几何图形,由一个圆柱体和两端各一个半球体组成。胶囊的体积可以通过将圆柱体的体积和两端半球体的体积相加来计算。本教程将讨论如何使用不同的方法在Java中计算给定胶囊的体积。 胶囊体积公式 胶囊体积的公式如下: 胶囊体积 = 圆柱体体积 两个半球体体积 其中, r: 半球体的半径。 h: 圆柱体的高度(不包括半球体)。 例子 1 输入 半径 = 5 单位 高度 = 10 单位 输出 体积 = 1570.8 立方单位 解释 使用公式计算体积: 体积 = π × r2 × h (4
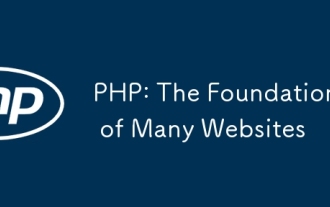
PHP成为许多网站首选技术栈的原因包括其易用性、强大社区支持和广泛应用。1)易于学习和使用,适合初学者。2)拥有庞大的开发者社区,资源丰富。3)广泛应用于WordPress、Drupal等平台。4)与Web服务器紧密集成,简化开发部署。
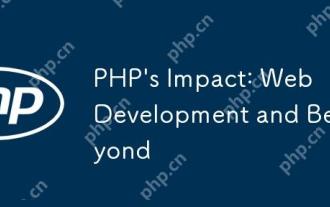
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
