TreeMap 与抽象类一起使用,在 Java 中部署 Map 和 NavigableMap 接口。映射根据其键的自然顺序或通过取决于构建器的预构建比较器进行排序。这是排序和存储键值对的简单方法。树状图保留的存储顺序必须与任何其他分类图相同,无论特定的比较器如何。
开始您的免费软件开发课程
网络开发、编程语言、软件测试及其他
以下是TreeMap的一些属性。
- 它由独特的元素组成。
- 它不能有空键。
- 可以多次出现空值。
- 非同步。
声明:
public class TreeMap<Key, Value> extends AbstractMap<Key, Value>implements NavigableMap<Key, Value>, Cloneable, Serializable
登录后复制
这里,Key 和 Value 分别是这个映射中的键和映射值的类型。
TreeMap 的构造函数
以下是TreeMap的构造函数。
-
TreeMap():将通过自然排序键来构造一个新的空树形图。
-
TreeMap(比较器 Key> 比较器):将通过对比较器中指定的键进行排序来构造一个新的空树形图。
-
TreeMap( 地图Key,?Ext Value> m): 将使用映射 m 中的映射构造一个新的树形图,并自然地对键进行排序。
-
TreeMap( SortedMapKey,? 扩展 Value> m): 将使用映射 m 中的映射构造一个新的树状图,并对比较器中指定的键进行排序。
TreeMap 的方法
TreeMap 提供了广泛的方法来帮助执行不同的功能。他们是:
-
clear(): All the mapping in the map will be removed.
-
clone(): A shallow copy will be returned for the TreeMap instance.
-
containsKey(Objectk): If there is mapping available for the specified key, true will be returned.
-
containsValue(Objectv): If there is mapping available for one or more keys for the value v, true will be returned.
-
ceilingKey(Kkey): Least key which is larger than or equal to the specified key will be returned. If there is no key, then null will be returned.
-
ceilingEntry(Kkey): Key-value pair for the least key, which is larger than or equal to the specified key will be returned. If there is no key, then null will be returned.
-
firstKey(): First or last key in the map will be returned.
-
firstEntry(): Key-value pair for the least or first key in the map will be returned. If there is no key, then null will be returned.
-
floorKey(Kkey): The Largest key, which is less than or equal to the specified key, will be returned. If there is no key, then null will be returned.
-
floorEntry(Kkey): Key-value pair for the largest key, which is less than or equal to the specified key, will be returned. If there is no key, then null will be returned.
-
lastKey(): Highest or last key in the map will be returned.
-
lastEntry(): Key-value pair for the largest key in the map will be returned. If there is no key, then null will be returned.
-
lowerKey(Kkey): The Largest key, which is strictly smaller than the specified key, will be returned. If there is no key, then null will be returned.
-
lowerEntry(Kkey): Key-value pair for the largest key, which is strictly smaller than the specified key, will be returned. If there is no key, then null will be returned.
-
remove(Objectk): Mapping for the specified key in the map will be removed.
-
size(): Count of key-value pairs in the map will be returned.
-
higherEntry(Kkey): Key-value pair for the smallest key, which is strictly larger than the specified key, will be returned. If there is no key, then null will be returned.
-
higherKey(Kkey): The Smallest key, which is strictly higher than the specified key, will be returned. If there is no key, then null will be returned.
-
descendingMap(): Reverse order will be returned for the mappings.
-
entrySet(): Set view will be returned for the mappings.
-
get(Objectk): The value of the specified key will be returned. If the key does not contain any mapping, then null will be returned.
-
keySet(): Set view will be returned for the keys.
-
navigableKeySet(): NavigableSet view will be returned for the keys.
-
pollFirstEntry(): Key-value pair for the least or first key in the map will be removed and returned. If the map is empty, then null will be returned.
-
pollLastEntry(): Key-value pairs for the greatest key in the map will be removed and returned. If the map is empty, then null will be returned.
-
values(): Collection view will be returned for the values in the map.
Example to Implement TreeMap in Java
Now, let us see a sample program to create a treemap and add elements to it.
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
public class TreeMapExample {
public static void main(String args[]) {
// Tree Map creation
TreeMap tmap = new TreeMap();
// Add elements to the treemap tmap
tmap.put("Anna Jeshua", new Double(3459.70));
tmap.put("Annamu Jeshua", new Double(321.56));
tmap.put("Izanorah Denan", new Double(1234.87));
tmap.put("Adam Jeshua", new Double(89.35));
tmap.put("Anabeth Jeshua", new Double(-20.98));
// Retrieve the entry set of treemap
Set set = tmap.entrySet();
// Create an iterator itr
Iterator itr = set.iterator();
// Display the elements in treemap using while loop
while(itr.hasNext()) {
Map.Entry mp = (Map.Entry)itr.next();
System.out.print(" Key : " + mp.getKey() + ";");
System.out.println(" Value : "+ mp.getValue());
}
System.out.println();
// Add 2500 to Anabeth's value
double val = ((Double)tmap.get("Anabeth Jeshua")).doubleValue();
tmap.put("Anabeth Jeshua", new Double(val + 2500));
System.out.println("Anabeth's new value: " + tmap.get("Anabeth Jeshua"));
} }
登录后复制
Output:
Keys and corresponding values of the TreeMap will be displayed on executing the code.
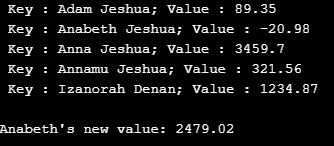
Explanation:
- 首先,创建一个TreeMap并向其中添加元素。
- 为了显示元素,必须创建一个迭代器。
- 使用迭代器,显示所有键及其对应的值。
- 要将键值加 2500,还可以使用 put() 方法。
结论
Java TreeMap 是红黑树的实现,有助于按排序顺序存储键值对。本文档详细讨论了Java TreeMap的声明、构造函数、方法和示例程序等几个细节。
以上是Java中的TreeMap是什么?的详细内容。更多信息请关注PHP中文网其他相关文章!