Java 中的 Servlet
Java 中的 Servlet 可以用多种方式描述。作为一种技术,Servlet 用于创建网页;作为 API,提供接口等。它用于扩展在请求-响应编程模型上托管应用程序的服务器的功能。 Servlet 提供基于组件和独立于平台的方法来构建基于 Web 的应用程序,而没有任何性能限制。 Java 中的 Servlet 可以通过 Java API 和 JDBC 完全访问企业数据库。我们将详细了解这些 Servlet 是什么、为什么使用它们、它的优点和局限性,以及 Servlet 在 Java 中实际如何工作。
广告 该类别中的热门课程 JAVA SERVLET - 专业化 | 18 门课程系列 | 6 次模拟测试开始您的免费软件开发课程
网络开发、编程语言、软件测试及其他
Servlet 可以用许多其他方式来描述,
- Servlet 是一种用于创建 Web 应用程序的技术
- Servlet 也是一个 API,提供许多接口和类以及文档
- 这是一个用Java实现的用于创建Servlet的接口
- 它是一个扩展服务器功能并响应传入请求的类。它可以是任何类型的请求。
- 它也是一个部署在服务器上的Web组件,用于创建动态网页。
Java 中为什么需要 Servlet?
随着技术的不断发展,我们需要每天熟悉最新的更新或最新的技术堆栈。 Servlet 充当接口、技术、Web 组件、类或 API。 借助 servlet,我们可以通过网页/表单、数据库以及任何其他数据源收集用户信息并创建网页。
- Java 中的 Servlet 与使用通用网关接口 (CGI) 实现的程序类似,但 Servlet 比 CGI 具有更多优势。
- 在性能方面,Servlet 明显优于 CGI。
- 与平台无关,因为 servlet 是用 Java 编写的。
- 它们在 Web 服务器空间内执行。我们不需要创建单独的流程来处理客户请求。
- Java 安全性在保护服务器计算机的资源方面实施了一组严格的限制,因此 Servlet 是可信的。
- Servlet 可以通过套接字、RMI 机制与数据库、小程序或其他一些软件进行通信。
Servlet 在 Java 中如何工作?
Java 中的 Servlet 检查通信接口、客户端和服务器的要求、使用的协议、编程语言以及涉及的软件。 Servlet 按以下步骤执行,
第 1 步: 客户端向 Web 服务器发送请求,读取客户端发送的显式数据,这些数据可以是 HTML 表单、小程序或自定义 HTTP 客户端程序。
第 2 步: Web 服务器接收请求。
第3步:Web服务器将请求传递给相应的servlet,处理请求可能包括与数据库通信、调用Web服务或直接响应。
第 4 步: Servlet 然后处理请求并以输出的形式生成响应。它可以是任何格式,HTML 或 XML、GIF(如果是图像)或 Excel。
第 5 步: 然后这些 servlet 将响应发送回服务器
第 6 步: 然后 Web 服务器将响应发送回客户端和客户端,如浏览器显示在 UI 上一样。
Servlet 架构
上面的 servlet 架构使用了一些 Java 方法,例如:
- Servlet.init(): 由 Servlet 调用,表示 Servlet 实例执行成功并调用服务。然后Servlet调用service()方法来处理客户端的请求。然后使用 destroy() 方法完成服务后终止
- Servlet.destroy():方法在整个生命周期中只会运行一次,这给我们一个信号,表明Servlet实例已经结束。
用 Java 创建 Servlet 的示例
首先,我们需要安装Java、Eclipse和Tomcat:
1.我们将使用 File-> 创建一个动态 Web 项目。新->动态网络项目。
2.输入Project Name并选择Target Runtime,点击Next,需要勾选“Generate web.xml”然后Finish
3.项目结构如下所示。
4.然后,点击文件->创建新的 Servlet。
5.单击上面的“完成”。现在 Eclipse 将根据前面步骤中完成的输入或配置生成 Servlet 类。
代码:
FirstProgram.java
package com.srccode.example; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class FirstProgram */ @WebServlet("/FirstProgram") public class FirstProgram extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public FirstProgram() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub response.getWriter().append("Served at: ").append(request.getContextPath()); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub doGet(request, response); } }
我们将修改 Servlet 类的代码如下,
包 com.srccode.example;
import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class FirstProgram */ @WebServlet("/FirstProgram") public class FirstProgram extends HttpServlet { private static final long <em>serialVersionUID</em> = 1L; /** * @see HttpServlet#HttpServlet() */ public FirstProgram() { super(); // TODO Auto-generated constructor stub } private String mymsg; public void init() throws ServletException { mymsg = "Hi eduCBA Team! We are working on Java Servlet Tutorial! This is the first Servlet Program!"; } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub response.setContentType("text/html"); PrintWriter printWriter = response.getWriter(); printWriter.println("<h1>" + mymsg + "</h1>"); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub doGet(request, response); } }
在 web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <display-name>ServletExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> </web-app>
在index.html
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>BeginnersBook Servlet Demo</title> </head> <body> <a href="welcome">Click to call Servlet</a> </body> </html>
输出:
右键,点击项目并选择运行方式->在服务器上运行。
现在打开浏览器,我们可以看到以下输出,服务器将在本地主机上运行:
http://localhost:8080/ServletExample/FirstProgram
Java Servlet 的优点
Java中的Servlet有很多优点。 Servlet 可以看作是运行在服务器端的小程序:
- Servlet 是持久的:Servlet 保留在内存中,直到使用 destroy() 方法销毁,这有助于服务传入的请求。它只与数据库建立一次连接,并且可以处理同一数据库上的多个请求。减少用于连接到数据库或任何其他源的时间和资源
- Servlet 是可移植的:这意味着 Servlet 与所有操作系统兼容,即在一个操作系统中编写的程序可以在其他操作系统上执行
- Servlet 独立于服务器:Servlet 与市场上可用的任何 Web 服务器兼容
- Servlet 与协议无关:Servlet 可以支持任何协议,如 FTP、Telnet 等。它提供了对 HTTP 协议的扩展支持
- Servlet 是安全的:由于这些 Servlet 是服务器端程序,仅由 Web 服务器调用,因此继承了 Web 服务器采取的所有安全措施
- Servlet 是可扩展的:Servlet 可以根据用户需求扩展并变形为对象
- Servlet 速度很快:由于这些 Servlet 被编译为字节码,因此与其他脚本语言相比执行速度更快。并且还提供类型检查和强错误。
- Servlet 价格低廉:有许多免费的 Web 服务器可用于任何类型的用途,无论是个人用途还是商业用途。
至此,我们结束了“Java Servlet”主题。我们已经通过示例了解了 Java 中的 Servlet 是什么以及如何使用它们。我们也看到了它的优点,并通过Servlet架构和使用的Servlet方法一步步了解了Servlet是如何使用的。还看到了为什么在 Java 中使用 Servlet 以及它相对于 CGI 的优点。关于 Servlet,我们还有很多值得探索的地方,还有多种类型的 Servlet 可供使用,我们将在进一步的教程中进行更深入的研究。
以上是Java 中的 Servlet的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
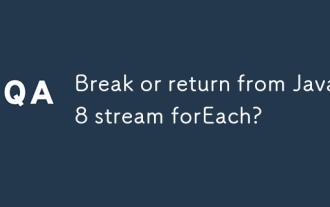
Java 8引入了Stream API,提供了一种强大且表达力丰富的处理数据集合的方式。然而,使用Stream时,一个常见问题是:如何从forEach操作中中断或返回? 传统循环允许提前中断或返回,但Stream的forEach方法并不直接支持这种方式。本文将解释原因,并探讨在Stream处理系统中实现提前终止的替代方法。 延伸阅读: Java Stream API改进 理解Stream forEach forEach方法是一个终端操作,它对Stream中的每个元素执行一个操作。它的设计意图是处
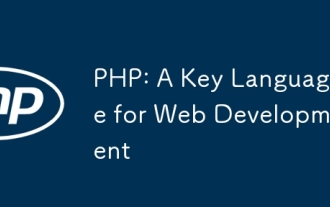
PHP是一种广泛应用于服务器端的脚本语言,特别适合web开发。1.PHP可以嵌入HTML,处理HTTP请求和响应,支持多种数据库。2.PHP用于生成动态网页内容,处理表单数据,访问数据库等,具有强大的社区支持和开源资源。3.PHP是解释型语言,执行过程包括词法分析、语法分析、编译和执行。4.PHP可以与MySQL结合用于用户注册系统等高级应用。5.调试PHP时,可使用error_reporting()和var_dump()等函数。6.优化PHP代码可通过缓存机制、优化数据库查询和使用内置函数。7
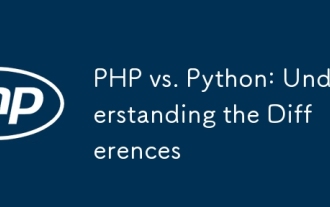
PHP和Python各有优势,选择应基于项目需求。1.PHP适合web开发,语法简单,执行效率高。2.Python适用于数据科学和机器学习,语法简洁,库丰富。
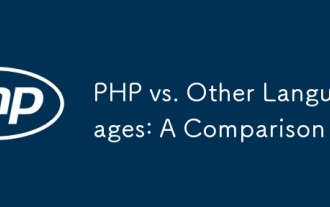
PHP适合web开发,特别是在快速开发和处理动态内容方面表现出色,但不擅长数据科学和企业级应用。与Python相比,PHP在web开发中更具优势,但在数据科学领域不如Python;与Java相比,PHP在企业级应用中表现较差,但在web开发中更灵活;与JavaScript相比,PHP在后端开发中更简洁,但在前端开发中不如JavaScript。
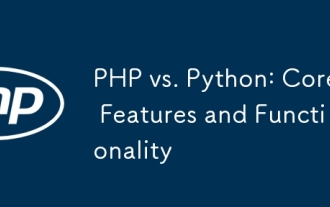
PHP和Python各有优势,适合不同场景。1.PHP适用于web开发,提供内置web服务器和丰富函数库。2.Python适合数据科学和机器学习,语法简洁且有强大标准库。选择时应根据项目需求决定。
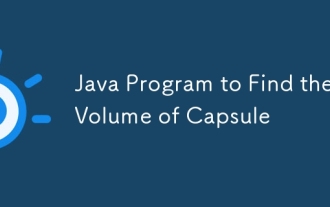
胶囊是一种三维几何图形,由一个圆柱体和两端各一个半球体组成。胶囊的体积可以通过将圆柱体的体积和两端半球体的体积相加来计算。本教程将讨论如何使用不同的方法在Java中计算给定胶囊的体积。 胶囊体积公式 胶囊体积的公式如下: 胶囊体积 = 圆柱体体积 两个半球体体积 其中, r: 半球体的半径。 h: 圆柱体的高度(不包括半球体)。 例子 1 输入 半径 = 5 单位 高度 = 10 单位 输出 体积 = 1570.8 立方单位 解释 使用公式计算体积: 体积 = π × r2 × h (4
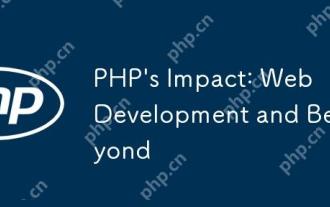
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
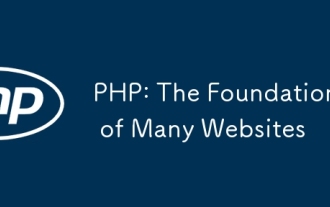
PHP成为许多网站首选技术栈的原因包括其易用性、强大社区支持和广泛应用。1)易于学习和使用,适合初学者。2)拥有庞大的开发者社区,资源丰富。3)广泛应用于WordPress、Drupal等平台。4)与Web服务器紧密集成,简化开发部署。
