Javascript 中的展开和休息运算符及其示例
其余和展开运算符是 JavaScript 中强大的功能,允许您更有效地处理数组、对象和函数参数。它们都使用相同的语法 (...),但用途不同。
休息操作员 (...)
剩余运算符用于将所有剩余元素收集到数组中。它通常用在函数参数中来处理可变数量的参数。
休息运算符示例:
function sum(...numbers) { return numbers.reduce((acc, curr) => acc + curr, 0); } console.log(sum(1, 2, 3, 4)); // Output: 10
这里,...numbers 将传递给 sum 函数的所有参数收集到一个名为 number 的数组中,然后可以对其进行处理。
扩展运算符 (...)
扩展运算符用于将数组或对象的元素扩展为单个元素或属性。
扩展运算符示例:
const arr1 = [1, 2, 3]; const arr2 = [4, 5, 6]; const combinedArray = [...arr1, ...arr2]; console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
在此示例中,...arr1 和 ...arr2 将 arr1 和 arr2 的元素扩展为新的组合数组。
概括
- 剩余运算符:将所有剩余元素收集到数组中。
- 扩展运算符:将数组或对象的元素扩展为单个元素或属性。
这些运算符对于以干净简洁的方式处理数组、对象和函数参数非常有用。
.
.
.
.
.
更多关于 Spread 和 Rest 运算符
.
.
.
.
.
当然!让我们更深入地了解其余和展开运算符,通过更详细的解释和示例探索它们的概念和各种用例。
休息操作员 (...)
剩余运算符允许您收集多个元素并将它们捆绑到一个数组中。它通常在函数中用于处理可变数量的参数或在解构数组或对象时收集“其余”元素。
使用案例
- 处理多个函数参数: 当您事先不知道函数将接收多少个参数时,通常会使用剩余运算符。
function multiply(factor, ...numbers) { return numbers.map(number => number * factor); } console.log(multiply(2, 1, 2, 3, 4)); // Output: [2, 4, 6, 8]
说明:
- Factor 是第一个参数。
- ...numbers 将剩余参数收集到数组 [1, 2, 3, 4] 中。
- 然后,map 函数将每个数字乘以因子 (2)。
- 解构数组: 解构数组时,可以使用剩余运算符来收集剩余元素。
const [first, second, ...rest] = [10, 20, 30, 40, 50]; console.log(first); // Output: 10 console.log(second); // Output: 20 console.log(rest); // Output: [30, 40, 50]
说明:
- 首先获取值 10。
- 第二个获取值 20。
- ...rest 将剩余元素 [30, 40, 50] 收集到一个数组中。
- 解构对象: 类似地,剩余运算符可用于捕获对象中的剩余属性。
const {a, b, ...rest} = {a: 1, b: 2, c: 3, d: 4}; console.log(a); // Output: 1 console.log(b); // Output: 2 console.log(rest); // Output: {c: 3, d: 4}
说明:
- a和b直接提取出来。
- ...rest 将剩余属性(c: 3 和 d: 4)捕获到一个新对象中。
扩展运算符 (...)
扩展运算符用于将数组、对象或可迭代对象的元素扩展为单个元素或属性。它与剩余运算符相反,对于合并、复制和传递元素非常有用。
使用案例
- 组合数组: 扩展运算符可用于组合或连接数组。
const arr1 = [1, 2]; const arr2 = [3, 4]; const arr3 = [5, 6]; const combined = [...arr1, ...arr2, ...arr3]; console.log(combined); // Output: [1, 2, 3, 4, 5, 6]
说明:
- ...arr1、...arr2 和 ...arr3 将它们的元素分散到组合数组中。
- 复制数组: 您可以使用扩展运算符创建数组的浅表副本。
const original = [1, 2, 3]; const copy = [...original]; console.log(copy); // Output: [1, 2, 3] console.log(copy === original); // Output: false (different references)
说明:
- ...original 将original 的元素传播到新的数组副本中,创建浅副本。
- 合并对象: 扩展运算符对于合并对象或向现有对象添加属性非常有用。
const obj1 = {x: 1, y: 2}; const obj2 = {y: 3, z: 4}; const merged = {...obj1, ...obj2}; console.log(merged); // Output: {x: 1, y: 3, z: 4}
说明:
- ...obj1 将 obj1 的属性传播到新对象中。
- ...obj2 然后将其属性传播到新对象中,覆盖 obj1 中的 y 属性。
- 函数参数: 扩展运算符还可用于将数组元素作为单独的参数传递给函数。
function add(a, b, c) { return a + b + c; } const numbers = [1, 2, 3]; console.log(add(...numbers)); // Output: 6
说明:
- ...numbers 将numbers 数组的元素分散到各个参数中(a、b、c)。
概括
-
休息运算符 (...):
- Collects multiple elements into an array or object.
- Often used in function parameters, array destructuring, or object destructuring.
-
Spread Operator (...):
- Expands or spreads elements from an array, object, or iterable.
- Useful for merging, copying, and passing elements in a concise manner.
Both operators enhance code readability and maintainability by reducing boilerplate code and providing more flexible ways to handle data structures.
.
.
.
.
.
.
Real world Example
.
.
.
.
Let's consider a real-world scenario where the rest and spread operators are particularly useful. Imagine you are building an e-commerce platform, and you need to manage a shopping cart and process user orders. Here's how you might use the rest and spread operators in this context:
Rest Operator: Managing a Shopping Cart
Suppose you have a function to add items to a user's shopping cart. The function should accept a required item and then any number of optional additional items. You can use the rest operator to handle this:
function addToCart(mainItem, ...additionalItems) { const cart = [mainItem, ...additionalItems]; console.log(`Items in your cart: ${cart.join(', ')}`); return cart; } // User adds a laptop to the cart, followed by a mouse and keyboard const userCart = addToCart('Laptop', 'Mouse', 'Keyboard'); // Output: Items in your cart: Laptop, Mouse, Keyboard
Explanation:
- mainItem is a required parameter, which in this case is the 'Laptop'.
- ...additionalItems collects the rest of the items passed to the function ('Mouse' and 'Keyboard') into an array.
- The cart array then combines all these items, and they are logged and returned as the user's cart.
Spread Operator: Processing an Order
Now, let's say you want to process an order and send the user's cart items along with their shipping details to a function that finalizes the order. The spread operator can be used to merge the cart items with the shipping details into a single order object.
const shippingDetails = { name: 'John Doe', address: '1234 Elm Street', city: 'Metropolis', postalCode: '12345' }; function finalizeOrder(cart, shipping) { const order = { items: [...cart], ...shipping, orderDate: new Date().toISOString() }; console.log('Order details:', order); return order; } // Finalizing the order with the user's cart and shipping details const userOrder = finalizeOrder(userCart, shippingDetails); // Output: // Order details: { // items: ['Laptop', 'Mouse', 'Keyboard'], // name: 'John Doe', // address: '1234 Elm Street', // city: 'Metropolis', // postalCode: '12345', // orderDate: '2024-09-01T12:00:00.000Z' // }
Explanation:
- ...cart spreads the items in the cart array into the items array inside the order object.
- ...shipping spreads the properties of the shippingDetails object into the order object.
- The orderDate property is added to capture when the order was finalized.
Combining Both Operators
Let's say you want to add a feature where the user can add multiple items to the cart, and the first item is considered a "featured" item with a discount. The rest operator can handle the additional items, and the spread operator can be used to create a new cart with the updated featured item:
function addItemsWithDiscount(featuredItem, ...otherItems) { const discountedItem = { ...featuredItem, price: featuredItem.price * 0.9 }; // 10% discount return [discountedItem, ...otherItems]; } const laptop = { name: 'Laptop', price: 1000 }; const mouse = { name: 'Mouse', price: 50 }; const keyboard = { name: 'Keyboard', price: 70 }; const updatedCart = addItemsWithDiscount(laptop, mouse, keyboard); console.log(updatedCart); // Output: // [ // { name: 'Laptop', price: 900 }, // { name: 'Mouse', price: 50 }, // { name: 'Keyboard', price: 70 } // ]
Explanation:
- The featuredItem (the laptop) receives a 10% discount by creating a new object using the spread operator, which copies all properties and then modifies the price.
- ...otherItems collects the additional items (mouse and keyboard) into an array.
- The final updatedCart array combines the discounted featured item with the other items using the spread operator.
Real-World Summary
- Rest Operator: Used to manage dynamic input like adding multiple items to a shopping cart. It gathers remaining arguments or properties into an array or object.
- Spread Operator: Useful for processing and transforming data, such as merging arrays, copying objects, and finalizing orders by combining item details with user information.
These examples demonstrate how the rest and spread operators can simplify code and improve readability in real-world scenarios like managing shopping carts and processing e-commerce orders.
Here's a breakdown of what's happening in your code:
const [first, second, third, ...rest] = [10, 20, 30, 40, 50]; console.log(first); // Output: 10 console.log(second); // Output: 20 console.log(third); // Output: 30 console.log(rest); // Output: [40, 50]
Explanation:
-
Destructuring:
- first is assigned the first element of the array (10).
- second is assigned the second element of the array (20).
- third is assigned the third element of the array (30).
-
Rest Operator:
- ...rest collects all the remaining elements of the array after the third element into a new array [40, 50].
Output:
- first: 10
- second: 20
- third: 30
- rest: [40, 50]
This code correctly logs the individual elements first, second, and third, and also captures the remaining elements into the rest array, which contains [40, 50].
Let me know if you have any further questions or if there's anything else you'd like to explore!
以上是Javascript 中的展开和休息运算符及其示例的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
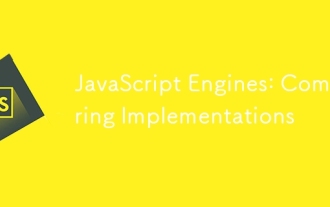
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
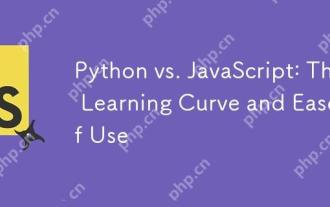
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
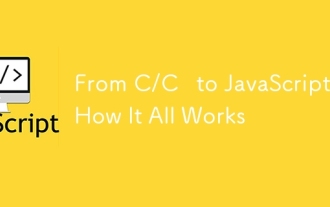
从C/C 转向JavaScript需要适应动态类型、垃圾回收和异步编程等特点。1)C/C 是静态类型语言,需手动管理内存,而JavaScript是动态类型,垃圾回收自动处理。2)C/C 需编译成机器码,JavaScript则为解释型语言。3)JavaScript引入闭包、原型链和Promise等概念,增强了灵活性和异步编程能力。
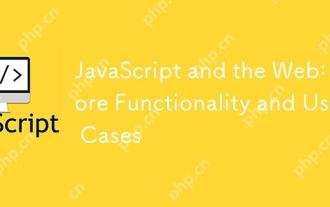
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
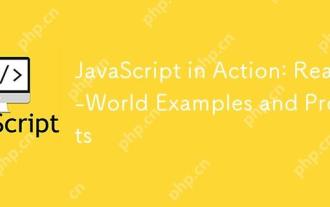
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
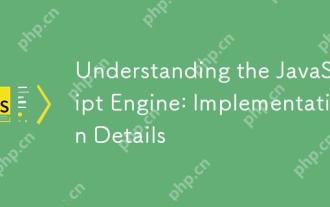
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
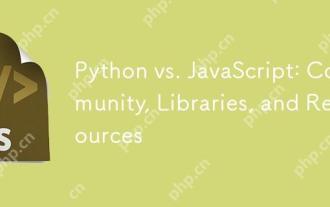
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
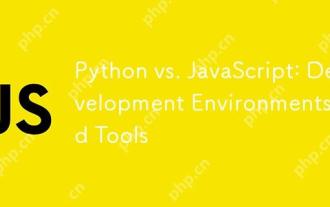
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
