C#终于
由于异常结束了当前正在运行的方法,并且该方法可能打开了一个文件或网络,需要在当前方法结束时关闭,因此可能会导致错误。为了克服这样的问题,我们在C#中有一个特殊的保留关键字,叫做Finally,当try和catch块的执行停止时,就会执行这个Finally代码块,无论什么情况导致执行停止,它都不会停止。不管try块的执行是正常停止还是异常停止。Finally代码块的主要目的是执行并释放所占用的资源,它写在try和catch代码块之后.
语法:
try { //Block of code } // this can be optional catch { //Block of code } finally { //Block of code }
C# 中finally 关键字的工作
- 在try块中分配的任何资源都可以通过使用Finally代码块来清理,并且无论try块中引发异常,Finally代码块都会运行。
- Finally 代码块中的语句在控件离开 try 代码块时执行。控制权作为正常执行或 goto 语句、break 语句、 continue 语句或 return 语句执行的一部分进行转移。
- 如果有异常处理,则与其关联的finally代码块肯定会运行;如果没有异常处理,则finally代码块的运行取决于异常展开操作的触发,而异常展开操作又取决于异常展开操作的触发设置计算机。
- C# 中同一程序中不能有多个 Final 代码块。
- 控件不会离开finally块,因为finally块中没有goto语句、break语句、continue语句或return语句。
最终实现 C# 的示例
下面是C#的例子最后:
示例#1
C# 程序,演示在程序中使用 Final 代码块。
代码:
using System; //a class called program is defined class program { // a method called ClassA() is defined static void ClassA() { try { Console.WriteLine("We are inside class A"); //An exception is thrown throw new Exception("An exception is thrown"); } //finally block is executed regardless of the exception is handled or not finally { Console.WriteLine("This is the finally block of Class A"); } } // a method called ClassB() is defined static void ClassB() { try { Console.WriteLine("We are Inside class B"); return; } //finally block is executed regardless of the exception is handled or not finally { Console.WriteLine("This is the finally block of class B"); } } // Main Method is called public static void Main(String[] args) { try { ClassA(); } catch (Exception) { Console.WriteLine("The exception that is thrown is caught"); } ClassB(); } }
输出:
说明:上面的程序中,程序就是定义的类。然后定义了一个名为 ClassA 的方法,其中编写了 try 和 finally 代码块。 try 块抛出一个稍后被捕获的异常。然后无论异常是否被处理,都会执行Finally块。然后定义了一个名为ClassB的方法。然后无论异常是否被处理,都会执行finally块。然后调用main方法。
示例#2
C# 程序,演示在具有异常处理的程序中使用finally关键字。
代码:
using System; //a class called program is defined public class program { // Main Method is called static public void Main() { // two integer variables are defined to store two integers intnum = 10; int div = 0; //try and catch block is defined in which an exception is raised by try block and is handled by catch block try { int op = num / div; } catch (DivideByZeroException) { Console.WriteLine("The divisor can not be zero because it is impossible to divide by 0"); } // finally block is executed regardless of the exception is handled or not finally { Console.WriteLine("We are in the finally block"); } } }
输出:
说明:在上面的程序中,定义了一个名为program的类。然后调用 main 方法。然后定义两个整型变量来存储两个整数。然后定义try和catch块,其中异常由try块引发并由catch块处理。然后无论异常是否被处理,都会执行finally块。
示例 #3
C# 程序,演示在没有异常处理的程序中使用finally关键字。
代码:
using System; //a class called program is defined public class program { // Main Method is called static public void Main() { // two integer variables are defined to store two integers intnum = 10; int div = 0; //try and catch block is defined in which an exception is raised by try block and is handled by catch block try { int op = num / div; } // finally block is executed regardless of the exception is handled or not finally { Console.WriteLine("We are in the finally block"); } } }
输出:
说明:在上面的程序中,定义了一个名为program的类。然后调用 main 方法。然后定义两个整型变量来存储两个整数。然后定义try块。然后无论异常是否被处理,都会执行finally块。程序的输出如上面的快照所示。
优点
- 最后,无论try块内发生什么,比如是否抛出异常,如果有return语句,都将执行该代码块。
- finally 代码块的主要用途是释放 try 块中所有分配的昂贵资源。
- 最后,block 确保无论抛出任何异常都会执行操作。
结论:在本教程中,我们通过定义、语法、编程示例及其输出来了解 C# 中的 finally 关键字的概念。
推荐文章
这是 C# 最终指南。在这里,我们最后讨论 C# 的简介及其优点以及示例和代码实现。您还可以浏览我们其他推荐的文章以了解更多信息 –
- C# 中的随机数生成器是什么?
- Java 中的静态构造函数 |工作|应用
- C# 中的 TextWriter |示例
- 如何在 C# 中使用静态构造函数?
以上是C#终于的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
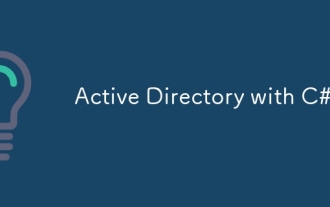
使用 C# 的 Active Directory 指南。在这里,我们讨论 Active Directory 在 C# 中的介绍和工作原理以及语法和示例。
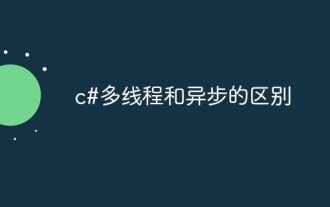
多线程和异步的区别在于,多线程同时执行多个线程,而异步在不阻塞当前线程的情况下执行操作。多线程用于计算密集型任务,而异步用于用户交互操作。多线程的优势是提高计算性能,异步的优势是不阻塞 UI 线程。选择多线程还是异步取决于任务性质:计算密集型任务使用多线程,与外部资源交互且需要保持 UI 响应的任务使用异步。

可以采用多种方法修改 XML 格式:使用文本编辑器(如 Notepad )进行手工编辑;使用在线或桌面 XML 格式化工具(如 XMLbeautifier)进行自动格式化;使用 XML 转换工具(如 XSLT)定义转换规则;或者使用编程语言(如 Python)进行解析和操作。修改时需谨慎,并备份原始文件。
