使用 Gin、FerretDB 和 oapi-codegen 构建博客 API
在本教程中,我们将逐步介绍使用 Go 为简单博客应用程序创建 RESTful API 的过程。我们将使用以下技术:
- Gin:Go 的 Web 框架
- FerretDB:兼容 MongoDB 的数据库
- oapi-codegen:根据 OpenAPI 3.0 规范生成 Go 服务器样板的工具
目录
- 设置项目
- 定义 API 规范
- 生成服务器代码
- 实现数据库层
- 实现 API 处理程序
- 运行应用程序
- 测试 API
- 结论
设置项目
首先,让我们设置 Go 项目并安装必要的依赖项:
mkdir blog-api cd blog-api go mod init github.com/yourusername/blog-api go get github.com/gin-gonic/gin go get github.com/deepmap/oapi-codegen/cmd/oapi-codegen go get github.com/FerretDB/FerretDB
定义API规范
在项目根目录中创建一个名为 api.yaml 的文件,并为我们的博客 API 定义 OpenAPI 3.0 规范:
openapi: 3.0.0 info: title: Blog API version: 1.0.0 paths: /posts: get: summary: List all posts responses: '200': description: Successful response content: application/json: schema: type: array items: $ref: '#/components/schemas/Post' post: summary: Create a new post requestBody: required: true content: application/json: schema: $ref: '#/components/schemas/NewPost' responses: '201': description: Created content: application/json: schema: $ref: '#/components/schemas/Post' /posts/{id}: get: summary: Get a post by ID parameters: - name: id in: path required: true schema: type: string responses: '200': description: Successful response content: application/json: schema: $ref: '#/components/schemas/Post' put: summary: Update a post parameters: - name: id in: path required: true schema: type: string requestBody: required: true content: application/json: schema: $ref: '#/components/schemas/NewPost' responses: '200': description: Successful response content: application/json: schema: $ref: '#/components/schemas/Post' delete: summary: Delete a post parameters: - name: id in: path required: true schema: type: string responses: '204': description: Successful response components: schemas: Post: type: object properties: id: type: string title: type: string content: type: string createdAt: type: string format: date-time updatedAt: type: string format: date-time NewPost: type: object required: - title - content properties: title: type: string content: type: string
生成服务器代码
现在,让我们使用 oapi-codegen 根据我们的 API 规范生成服务器代码:
oapi-codegen -package api api.yaml > api/api.go
此命令将创建一个名为 api 的新目录,并生成包含服务器接口和模型的 api.go 文件。
实施数据库层
创建一个名为 db/db.go 的新文件,以使用 FerretDB 实现数据库层:
package db import ( "context" "time" "go.mongodb.org/mongo-driver/bson" "go.mongodb.org/mongo-driver/bson/primitive" "go.mongodb.org/mongo-driver/mongo" "go.mongodb.org/mongo-driver/mongo/options" ) type Post struct { ID primitive.ObjectID `bson:"_id,omitempty"` Title string `bson:"title"` Content string `bson:"content"` CreatedAt time.Time `bson:"createdAt"` UpdatedAt time.Time `bson:"updatedAt"` } type DB struct { client *mongo.Client posts *mongo.Collection } func NewDB(uri string) (*DB, error) { client, err := mongo.Connect(context.Background(), options.Client().ApplyURI(uri)) if err != nil { return nil, err } db := client.Database("blog") posts := db.Collection("posts") return &DB{ client: client, posts: posts, }, nil } func (db *DB) Close() error { return db.client.Disconnect(context.Background()) } func (db *DB) CreatePost(title, content string) (*Post, error) { post := &Post{ Title: title, Content: content, CreatedAt: time.Now(), UpdatedAt: time.Now(), } result, err := db.posts.InsertOne(context.Background(), post) if err != nil { return nil, err } post.ID = result.InsertedID.(primitive.ObjectID) return post, nil } func (db *DB) GetPost(id string) (*Post, error) { objectID, err := primitive.ObjectIDFromHex(id) if err != nil { return nil, err } var post Post err = db.posts.FindOne(context.Background(), bson.M{"_id": objectID}).Decode(&post) if err != nil { return nil, err } return &post, nil } func (db *DB) UpdatePost(id, title, content string) (*Post, error) { objectID, err := primitive.ObjectIDFromHex(id) if err != nil { return nil, err } update := bson.M{ "$set": bson.M{ "title": title, "content": content, "updatedAt": time.Now(), }, } var post Post err = db.posts.FindOneAndUpdate( context.Background(), bson.M{"_id": objectID}, update, options.FindOneAndUpdate().SetReturnDocument(options.After), ).Decode(&post) if err != nil { return nil, err } return &post, nil } func (db *DB) DeletePost(id string) error { objectID, err := primitive.ObjectIDFromHex(id) if err != nil { return err } _, err = db.posts.DeleteOne(context.Background(), bson.M{"_id": objectID}) return err } func (db *DB) ListPosts() ([]*Post, error) { cursor, err := db.posts.Find(context.Background(), bson.M{}) if err != nil { return nil, err } defer cursor.Close(context.Background()) var posts []*Post for cursor.Next(context.Background()) { var post Post if err := cursor.Decode(&post); err != nil { return nil, err } posts = append(posts, &post) } return posts, nil }
实施 API 处理程序
创建一个名为 handlers/handlers.go 的新文件来实现 API 处理程序:
package handlers import ( "net/http" "time" "github.com/gin-gonic/gin" "github.com/yourusername/blog-api/api" "github.com/yourusername/blog-api/db" ) type BlogAPI struct { db *db.DB } func NewBlogAPI(db *db.DB) *BlogAPI { return &BlogAPI{db: db} } func (b *BlogAPI) ListPosts(c *gin.Context) { posts, err := b.db.ListPosts() if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } apiPosts := make([]api.Post, len(posts)) for i, post := range posts { apiPosts[i] = api.Post{ Id: post.ID.Hex(), Title: post.Title, Content: post.Content, CreatedAt: post.CreatedAt, UpdatedAt: post.UpdatedAt, } } c.JSON(http.StatusOK, apiPosts) } func (b *BlogAPI) CreatePost(c *gin.Context) { var newPost api.NewPost if err := c.ShouldBindJSON(&newPost); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } post, err := b.db.CreatePost(newPost.Title, newPost.Content) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } c.JSON(http.StatusCreated, api.Post{ Id: post.ID.Hex(), Title: post.Title, Content: post.Content, CreatedAt: post.CreatedAt, UpdatedAt: post.UpdatedAt, }) } func (b *BlogAPI) GetPost(c *gin.Context) { id := c.Param("id") post, err := b.db.GetPost(id) if err != nil { c.JSON(http.StatusNotFound, gin.H{"error": "Post not found"}) return } c.JSON(http.StatusOK, api.Post{ Id: post.ID.Hex(), Title: post.Title, Content: post.Content, CreatedAt: post.CreatedAt, UpdatedAt: post.UpdatedAt, }) } func (b *BlogAPI) UpdatePost(c *gin.Context) { id := c.Param("id") var updatePost api.NewPost if err := c.ShouldBindJSON(&updatePost); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } post, err := b.db.UpdatePost(id, updatePost.Title, updatePost.Content) if err != nil { c.JSON(http.StatusNotFound, gin.H{"error": "Post not found"}) return } c.JSON(http.StatusOK, api.Post{ Id: post.ID.Hex(), Title: post.Title, Content: post.Content, CreatedAt: post.CreatedAt, UpdatedAt: post.UpdatedAt, }) } func (b *BlogAPI) DeletePost(c *gin.Context) { id := c.Param("id") err := b.db.DeletePost(id) if err != nil { c.JSON(http.StatusNotFound, gin.H{"error": "Post not found"}) return } c.Status(http.StatusNoContent) }
运行应用程序
在项目根目录中创建一个名为 main.go 的新文件来设置和运行应用程序:
package main import ( "log" "github.com/gin-gonic/gin" "github.com/yourusername/blog-api/api" "github.com/yourusername/blog-api/db" "github.com/yourusername/blog-api/handlers" ) func main() { // Initialize the database connection database, err := db.NewDB("mongodb://localhost:27017") if err != nil { log.Fatalf("Failed to connect to the database: %v", err) } defer database.Close() // Create a new Gin router router := gin.Default() // Initialize the BlogAPI handlers blogAPI := handlers.NewBlogAPI(database) // Register the API routes api.RegisterHandlers(router, blogAPI) // Start the server log.Println("Starting server on :8080") if err := router.Run(":8080"); err != nil { log.Fatalf("Failed to start server: %v", err) } }
测试 API
现在我们已经启动并运行了 API,让我们使用curl 命令来测试它:
- 创建一个新帖子:
curl -X POST -H "Content-Type: application/json" -d '{"title":"My First Post","content":"This is the content of my first post."}' http://localhost:8080/posts
- 列出所有帖子:
curl http://localhost:8080/posts
- 获取特定帖子(将 {id} 替换为实际帖子 ID):
curl http://localhost:8080/posts/{id}
- 更新帖子(将 {id} 替换为实际帖子 ID):
curl -X PUT -H "Content-Type: application/json" -d '{"title":"Updated Post","content":"This is the updated content."}' http://localhost:8080/posts/{id}
- 删除帖子(将 {id} 替换为实际帖子 ID):
curl -X DELETE http://localhost:8080/posts/{id}
结论
在本教程中,我们使用 Gin 框架、FerretDB 和 oapi-codegen 构建了一个简单的博客 API。我们已经介绍了以下步骤:
- 设置项目并安装依赖项
- 使用 OpenAPI 3.0 定义 API 规范
- 使用 oapi-codegen 生成服务器代码
- 使用FerretDB实现数据库层
- 实现 API 处理程序
- 运行应用程序
- 使用curl命令测试API
该项目演示了如何利用代码生成和 MongoDB 兼容数据库的强大功能,使用 Go 创建 RESTful API。您可以通过添加身份验证、分页和更复杂的查询功能来进一步扩展此 API。
请记住在将此 API 部署到生产环境之前适当处理错误、添加适当的日志记录并实施安全措施。
需要帮助吗?
您是否面临着具有挑战性的问题,或者需要外部视角来看待新想法或项目?我可以帮忙!无论您是想在进行更大投资之前建立技术概念验证,还是需要解决困难问题的指导,我都会为您提供帮助。
提供的服务:
- 解决问题:通过创新的解决方案解决复杂问题。
- 咨询:为您的项目提供专家建议和新观点。
- 概念验证:开发初步模型来测试和验证您的想法。
如果您有兴趣与我合作,请通过电子邮件与我联系:hungaikevin@gmail.com。
让我们将挑战转化为机遇!
以上是使用 Gin、FerretDB 和 oapi-codegen 构建博客 API的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
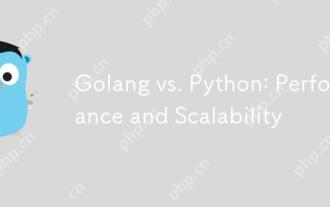
Golang在性能和可扩展性方面优于Python。1)Golang的编译型特性和高效并发模型使其在高并发场景下表现出色。2)Python作为解释型语言,执行速度较慢,但通过工具如Cython可优化性能。
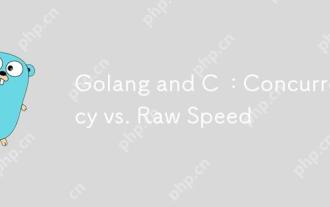
Golang在并发性上优于C ,而C 在原始速度上优于Golang。1)Golang通过goroutine和channel实现高效并发,适合处理大量并发任务。2)C 通过编译器优化和标准库,提供接近硬件的高性能,适合需要极致优化的应用。
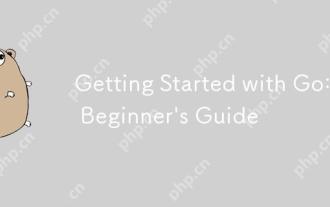
goisidealforbeginnersandsubableforforcloudnetworkservicesduetoitssimplicity,效率和concurrencyFeatures.1)installgromtheofficialwebsitealwebsiteandverifywith'.2)
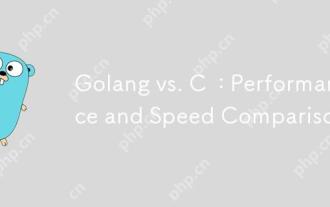
Golang适合快速开发和并发场景,C 适用于需要极致性能和低级控制的场景。1)Golang通过垃圾回收和并发机制提升性能,适合高并发Web服务开发。2)C 通过手动内存管理和编译器优化达到极致性能,适用于嵌入式系统开发。
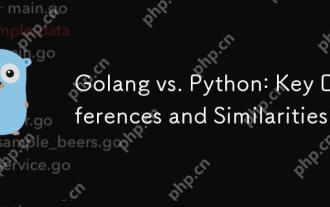
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。 Golang以其并发模型和高效性能着称,Python则以简洁语法和丰富库生态系统着称。
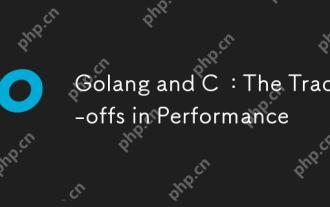
Golang和C 在性能上的差异主要体现在内存管理、编译优化和运行时效率等方面。1)Golang的垃圾回收机制方便但可能影响性能,2)C 的手动内存管理和编译器优化在递归计算中表现更为高效。
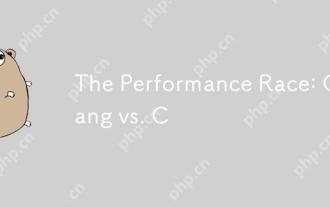
Golang和C 在性能竞赛中的表现各有优势:1)Golang适合高并发和快速开发,2)C 提供更高性能和细粒度控制。选择应基于项目需求和团队技术栈。
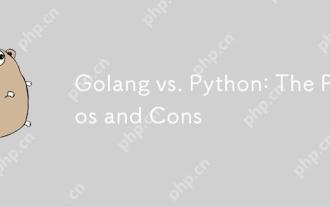
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
