索引数据库
索引数据库解决什么问题?
索引数据库 是一种存储大量结构化数据(甚至离线)的解决方案。它解决了 Web 应用程序的高效本地存储问题,使它们能够直接在客户端访问、更新和查询大型数据集。
索引数据库 解决的关键问题:
- 结构化数据的持久本地存储。
- 大型数据集的高效存储和检索。
- 跨离线和在线模式的功能。
- 异步访问数据,防止主线程阻塞。
代码示例:
// Open an 索引数据库 database let request = indexedDB.open("MyDatabase", 1); request.onupgradeneeded = function(event) { let db = event.target.result; // Create an object store let objectStore = db.createObjectStore("users", { keyPath: "id" }); objectStore.createIndex("name", "name", { unique: false }); }; request.onsuccess = function(event) { let db = event.target.result; console.log("Database opened successfully"); };
如何使用 索引数据库
打开数据库:使用indexedDB.open()函数打开索引数据库数据库。
创建对象存储:对象存储类似于关系数据库中的表。
执行事务:使用事务来添加、检索、更新或删除数据。
索引创建:索引用于在对象存储中搜索数据。
错误处理:使用事件监听器处理异步操作。
// Adding data to 索引数据库 let addData = function(db) { let transaction = db.transaction(["users"], "readwrite"); let objectStore = transaction.objectStore("users"); let user = { id: 1, name: "Alice", email: "alice@example.com" }; let request = objectStore.add(user); request.onsuccess = function() { console.log("User added to 索引数据库!"); }; }; // Retrieve data let getData = function(db) { let transaction = db.transaction(["users"], "readonly"); let objectStore = transaction.objectStore("users"); let request = objectStore.get(1); request.onsuccess = function(event) { console.log("User:", event.target.result); }; };
索引数据库 的优点
索引数据库 提供了几个关键优势,使其成为现代 Web 应用程序的理想选择
- 存储容量大:索引数据库 相比 localStorage 或 sessionStorage 可以存储更多数据
异步访问:通过使用非阻塞 I/O 操作防止阻塞 UI。
复杂数据处理:支持结构化数据,包括数组、对象和 blob。
离线支持:在线和离线工作,允许渐进式 Web 应用程序 (PWA) 用例。
索引搜索:可以通过索引高效地搜索数据。
// Create an index to improve search performance objectStore.createIndex("email", "email", { unique: true }); let emailIndex = objectStore.index("email"); let request = emailIndex.get("alice@example.com"); request.onsuccess = function(event) { console.log("User found by email:", event.target.result); };
*了解 索引数据库 索引
*
什么是 索引数据库 索引?
索引数据库 索引是对象存储中的附加数据结构,允许根据特定属性高效检索数据。索引由关键路径(一个或多个属性)和定义唯一性和排序顺序的选项组成。通过创建索引,您可以优化数据查询和过滤操作,从而提高性能。
索引数据库 中索引的重要性
索引在增强 索引数据库 的数据检索性能方面发挥着至关重要的作用。如果没有索引,根据特定属性查询数据将需要迭代所有记录,从而导致数据检索速度变慢且效率低下。索引可以根据索引属性直接访问数据,减少全表扫描的需要并显着提高查询执行速度。
let transaction = db.transaction("MyObjectStore", "readonly"); let objectStore = transaction.objectStore("MyObjectStore"); let index = objectStore.index("nameIndex"); let cursorRequest = index.openCursor(); cursorRequest.onsuccess = function(event) { let cursor = event.target.result; if (cursor) { // Access the current record console.log(cursor.value); // Move to the next record cursor.continue(); } };
使用范围查询过滤数据 索引数据库
索引还支持范围查询,允许您根据一系列值过滤数据。以下是检索特定年龄范围内的记录的示例:
let transaction = db.transaction("MyObjectStore", "readonly"); let objectStore = transaction.objectStore("MyObjectStore"); let index = objectStore.index("ageIndex"); let range = IDBKeyRange.bound(18, 30); // Records with age between 18 and 30 let cursorRequest = index.openCursor(range); cursorRequest.onsuccess = function(event) { let cursor = event.target.result; if (cursor) { // Process the record within the desired age range console.log(cursor.value); // Move to the next record cursor.continue(); } };
使用索引对数据进行排序
索引还可以用于对 索引数据库 中的数据进行排序。通过在索引上打开游标并指定所需的排序顺序,您可以按所需的顺序检索记录。
let transaction = db.transaction("MyObjectStore", "readonly"); let objectStore = transaction.objectStore("MyObjectStore"); let index = objectStore.index("nameIndex"); let cursorRequest = index.openCursor(null, "next"); // Sorted in ascending order cursorRequest.onsuccess = function(event) { let cursor = event.target.result; if (cursor) { // Process the record console.log(cursor.value); // Move to the next record cursor.continue(); } };
索引维护和架构升级
向现有对象存储添加新索引
要将新索引添加到现有对象存储中,您需要处理数据库升级过程。这是在数据库升级期间添加新索引的示例。
let request = indexedDB.open("MyDatabase", 2); request.onupgradeneeded = function(event) { let db = event.target.result; let objectStore = db.createObjectStore("MyObjectStore", { keyPath: "id" }); // Add a new index during upgrade objectStore.createIndex("newIndex", "newProperty", { unique: false }); };
在此示例中,我们将数据库版本从 1 升级到 2,并在“newProperty”属性上添加名为“newIndex”的新索引。
性能注意事项和最佳实践
选择正确的 索引数据库 索引
创建索引时,根据应用程序的数据访问模式仔细选择要建立索引的属性非常重要。应在常用于过滤、排序或搜索的属性上创建索引,以最大限度地发挥其优势。
限制索引大小和性能影响
大型索引会消耗大量存储空间并影响性能。建议限制索引的数量并选择高效数据检索所需的关键路径。避免过多的索引以保持最佳性能。
Monitoring Index Performance
Regularly monitor the performance of your 索引数据库 indexes using browser developer tools and profiling techniques. Identify any slow queries or bottlenecks and optimize your indexes accordingly. It may involve modifying the index structure or adding additional indexes to improve query execution speed.
Cursor in 索引数据库
let request = indexedDB.open("MyDatabase", 1); request.onsuccess = function(event) { let db = event.target.result; let transaction = db.transaction(["users"], "readonly"); let objectStore = transaction.objectStore("users"); let cursorRequest = objectStore.openCursor(); let batchSize = 10; let count = 0; cursorRequest.onsuccess = function(event) { let cursor = event.target.result; if (cursor) { console.log("Key:", cursor.key, "Value:", cursor.value); count++; // If batch size is reached, stop processing if (count <hr> <p><strong>索引数据库 Sharding</strong></p> <p>Sharding is a technique, normally used in server side databases, where the database is partitioned horizontally. Instead of storing all documents at one table/collection, the documents are split into so called shards and each shard is stored on one table/collection. This is done in server side architectures to spread the load between multiple physical servers which increases scalability.</p> <p>When you use 索引数据库 in a browser, there is of course no way to split the load between the client and other servers. But you can still benefit from sharding. Partitioning the documents horizontally into multiple 索引数据库 stores, has shown to have a big performance improvement in write- and read operations while only increasing initial pageload slightly.</p> <p><img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/172562768114980.jpg" class="lazy" alt="索引数据库"></p> <hr> <p><strong>Conclusion</strong><br> 索引数据库 represents a paradigm shift in browser-based storage, empowering developers to build powerful web applications with offline capabilities and efficient data management. By embracing 索引数据库 and adhering to best practices, developers can unlock new possibilities in web development, delivering richer and more responsive user experiences across a variety of platforms and devices. As the web continues to evolve, 索引数据库 stands as a testament to the innovative solutions driving the next generation of web applications.</p> <hr>
以上是索引数据库的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
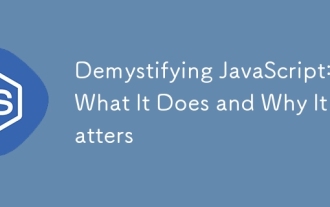
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
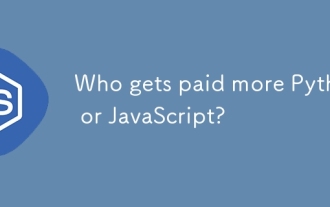
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
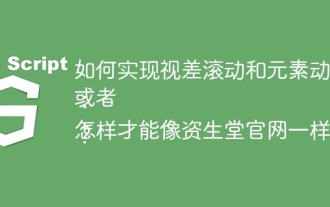
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
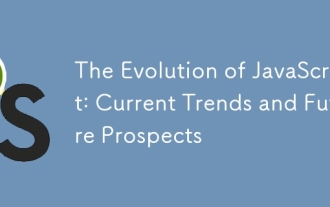
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
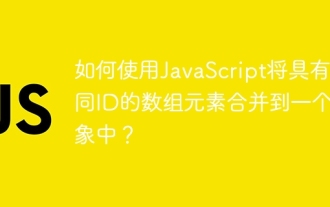
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
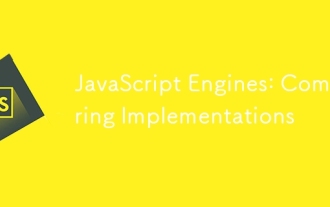
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
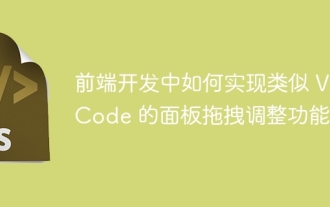
探索前端中类似VSCode的面板拖拽调整功能的实现在前端开发中,如何实现类似于VSCode...
